Create an editable list detail using Configuration section
Add a custom Courier service detail to the Delivery tab of the order page. Display the index of courier services (i. e., the values of the Account lookup) available for the current order on the detail.
1. Create a detail object schema
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Object in the section list toolbar.
-
Fill out the schema properties.
- Set Code to "UsrCourierService."
- Set Title to "Courier service."
- Select "BaseEntity" in the Parent object property.
-
Add a column that stores the order to the schema.
-
Add a column that stores the account to the schema in a similar way.
-
Click Save then Publish on the Object Designer’s toolbar.
2. Create a view model schema of the detail
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Detail (list) view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "UsrCourierServiceDetail."
- Set Title to "Courier service in Order detail schema."
- Select "BaseGridDetailV2" in the Parent object property.
-
Add a localizable string.
-
Implement an editable detail list.
- Add the
ConfigurationGrid
,ConfigurationGridGenerator
,ConfigurationGridUtilitiesV2
module schemas as dependencies. - Add the
ConfigurationGridUtilitiesV2
mixin to themixins
property. - Add the
IsEditable
attribute to theattributes
property. Set thevalue
property of the attribute totrue
. - Add the configuration object to the
diff array of modifications
. This object must have the property settings and binding of the detail list events' handler methods.
View the source code of the
UsrCourierServiceDetail
view model schema of the detail below.UsrCourierServiceDetail/* Define the schema and set its dependencies on other modules. */
define("UsrCourierServiceDetail", ["ConfigurationGrid", "ConfigurationGridGenerator", "ConfigurationGridUtilitiesV2"], function() {
return {
/* The name of the detail object schema. */
entitySchemaName: "UsrCourierService",
/* The index of schema attributes. */
attributes: {
/* The flag that enables editability. */
"IsEditable": {
/* Set the data type to boolean. */
dataValueType: Terrasoft.DataValueType.BOOLEAN,
/* Set the attribute type to a virtual column of the view model. */
type: Terrasoft.ViewModelColumnType.VIRTUAL_COLUMN,
/* The value to set. */
value: true
}
},
/* The mixins used. */
mixins: {
ConfigurationGridUtilities: "Terrasoft.ConfigurationGridUtilitiesV2"
},
/* The array of view model modifications. */
diff: /**SCHEMA_DIFF*/[
{
/* Set the operation type to merge. */
"operation": "merge",
/* The name of the schema element on which to execute the operation. */
"name": "DataGrid",
/* The object whose properties to merge with the schema element properties. */
"values": {
/* The class name. */
"className": "Terrasoft.ConfigurationGrid",
/* The view generator must generate only a part of the view. */
"generator": "ConfigurationGridGenerator.generatePartial",
/* Bind the active string edit element’s configuration retrieval event to the handler method. */
"generateControlsConfig": {"bindTo": "generateActiveRowControlsConfig"},
/* Bind the active record change event to the handler method. */
"changeRow": {"bindTo": "changeRow"},
/* Bind the record’s selection cancel event to the handler method. */
"unSelectRow": {"bindTo": "unSelectRow"},
/* Bind the list click event to the handler method. */
"onGridClick": {"bindTo": "onGridClick"},
/* Bind the field caption to the localizable schema string. */
"caption": {"bindTo": "Resources.Strings.CourierServiceCaption"},
/* The operations to execute on the active record. */
"activeRowActions": /* Set up the Save] action. */
{
/* The name of the control class to which the action is connected. */
"className": "Terrasoft.Button",
/* Set the button style to transparent. */
"style": this.Terrasoft.controls.ButtonEnums.style.TRANSPARENT,
/* The tag. */
"tag": "save",
/* The marker value. */
"markerValue": "save",
/* Bind to the button image. */
"imageConfig": {"bindTo": "Resources.Images.SaveIcon"}
},
/* Set up the [Edit on page] action. */
{
"className": "Terrasoft.Button",
"style": this.Terrasoft.controls.ButtonEnums.style.TRANSPARENT,
"tag": "card",
"markerValue": "card",
"imageConfig": {"bindTo": "Resources.Images.CardIcon"}
},
/* Set up the [Copy] action. */
{
"className": "Terrasoft.Button",
"style": Terrasoft.controls.ButtonEnums.style.TRANSPARENT,
"tag": "copy",
"markerValue": "copy",
"imageConfig": {"bindTo": "Resources.Images.CopyIcon"}
},
/* Set up the [Cancel] action. */
{
"className": "Terrasoft.Button",
"style": this.Terrasoft.controls.ButtonEnums.style.TRANSPARENT,
"tag": "cancel",
"markerValue": "cancel",
"imageConfig": {"bindTo": "Resources.Images.CancelIcon"}
},
/* Set up the [Delete] action. */
{
"className": "Terrasoft.Button",
"style": this.Terrasoft.controls.ButtonEnums.style.TRANSPARENT,
"tag": "remove",
"markerValue": "remove",
"imageConfig": {"bindTo": "Resources.Images.RemoveIcon"}
}
],
/* Bind the method that initializes listening to button click events in the active row. */
"initActiveRowKeyMap": {"bindTo": "initActiveRowKeyMap"},
/* Bind the active record’s action execution event to the handler method. */
"activeRowAction": {"bindTo": "onActiveRowAction"},
/* Flag that enables selection of multiple records. */
"multiSelect": {"bindTo": "MultiSelect"}
}
}
]/**SCHEMA_DIFF*/
};
}); - Add the
-
Click Save on the Module Designer’s toolbar.
3. Create a replacing view model schema of the order page
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "OrderPageV2."
- Set Title to "Order edit page."
- Select "OrderPageV2" in the Parent object property.
-
Add the custom
UsrCourierServiceDetail
detail to the order page.- Add the
UsrCourierServiceDetail
detail to thedetails
property. - Add a configuration object with the settings that determine the detail layout to the
diff
array of modifications.
View the source code of the order page’s replacing view model below.
OrderPageV2define("OrderPageV2", [], function() {
return {
/* The name of the record page object’s schema. */
entitySchemaName: "Order",
/* The details of the record page. */
details: /**SCHEMA_DETAILS*/{
/* Set up the [Courier service] detail. */
"UsrCourierServiceDetail": {
/* The name of the detail schema. */
"schemaName": "UsrCourierServiceDetail",
/* The name of the detail object schema. */
"entitySchemaName": "UsrCourierService",
/* Display only the contacts relevant to the current order. */
"filter": {
/* The column of the detail object schema. */
"detailColumn": "UsrOrder",
/* The column of the section object schema. */
"masterColumn": "Id"
}
}
}/**SCHEMA_DETAILS*/,
/* Display the detail on the record page. */
diff: /**SCHEMA_DIFF*/[
/* The properties to add the custom detail to the page. */
{
/* Add the element to the page. */
"operation": "insert",
/* The meta name of the detail to add. */
"name": "UsrCourierServiceDetail",
/* The meta name of the parent container to add the detail. */
"parentName": "OrderDeliveryTab",
/* Add the detail to the parent element’s collection of elements. */
"propertyName": "items",
/* The index in the list of elements to add. */
"index": 3,
/* The properties to pass to the element’s constructor. */
"values": {
/* Set the type of the added element to detail. */
"itemType": Terrasoft.core.enums.ViewItemType.DETAIL,
"markerValue": "added-detail"
}
}
]/**SCHEMA_DIFF*/
};
}); - Add the
-
Click Save on the Module Designer’s toolbar.
4. Register the custom detail in the database
To make the detail visible in the Section Wizard and Detail Wizard, register it in the database. To do this, execute the SQL query to the [SysDetails]
database table.
DECLARE
-- The name of the detail schema.
@ClientUnitSchemaName NVARCHAR(100) = 'UsrCourierServiceDetail',
-- The name of the detail object schema.
@EntitySchemaName NVARCHAR(100) = 'UsrCourierService',
-- The name of the detail.
@DetailCaption NVARCHAR(100) = 'CourierService'
INSERT INTO SysDetail(
Caption,
DetailSchemaUId,
EntitySchemaUId
)
VALUES(
@DetailCaption,
(SELECT TOP 1 UId
from SysSchema
WHERE Name = @ClientUnitSchemaName),
(SELECT TOP 1 UId
from SysSchema
WHERE Name = @EntitySchemaName)
)
Outcome of the example
To view the outcome of the example:
- Refresh the Orders section page.
- Open an order page and go to the Delivery tab.
As a result, Creatio will add the custom Courier service detail to the Delivery tab of the order page. The detail displays the index of available courier services (the values of the Account lookup) for the current order (the Order column). The order is populated automatically.
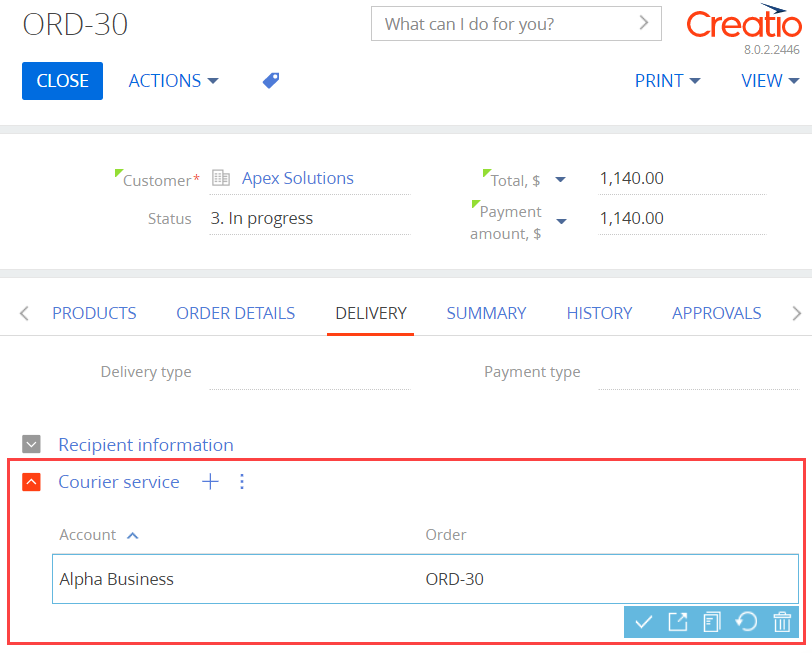