Implement the bulk addition of records to the detail
The functionality is relevant to Classic UI.
Implement the bulk addition of records to the Contacts detail on the record page of the Opportunities section.
1. Create the view model schema of the detail
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties in the Module Designer:
- Set Code to "OpportunityContactDetailV2."
- Set Title to "OpportunityContactDetailV2."
- Set Parent object to "OpportunityContactDetailV2."
2. Implement the business logic of the detail
-
Add the
LookupMultiAddMixin
mixin to themixins
property of the detail schema. -
Initialize the
LookupMultiAddMixin
mixin in the overriddeninit()
method of the detail schema. Learn more about theinit()
module: Module types and their specificities. -
Override the
getAddRecordButtonVisible()
method that displays the addition button. -
Override the
onCardSaved()
method that saves the detail page.Use the
openLookupWithMultiSelect()
method that calls the multiple selection dialog box. -
Implement the
getMultiSelectLookupConfig()
method that configures the dialog box. The method is connected to theopenLookupWithMultiSelect()
method and returns the configuration object for the dialog box.The object properties are as follows:
rootEntitySchemaName
is the root object schema.rootColumnName
is the connected column that indicates the root schema record.relatedEntitySchemaName
is the connected schema.relatedColumnName
is the column that indicates the connected schema record.
-
Override the
addRecord()
method that adds records to the detail. Use theopenLookupWithMultiSelect()
, similar toonCardSaved()
method. Set the value totrue
to run a check if the record is new.In this example, the dialog box uses the data of the
[OpportunityContact]
table connected to the[Opportunity]
column of the[Opportunity]
root schema and the[Contact]
column of the[Contact]
connected schema.View the source code of the detail schema below.
OpportunityContactDetailV2define("OpportunityContactDetailV2", ["LookupMultiAddMixin"], function() {
return {
mixins: {
/* Enable the mixin in the schema. */
LookupMultiAddMixin: "Terrasoft.LookupMultiAddMixin"
},
methods: {
/* Override the base method that initializes the schema. */
init: function() {
this.callParent(arguments);
/* Initialize the mixin. */
this.mixins.LookupMultiAddMixin.init.call(this);
},
/* Override the base method that displays the addition button. */
getAddRecordButtonVisible: function() {
/* Display the addition button if the detail is expanded regardless of whether you implemented the record page for the detail. */
return this.getToolsVisible();
},
/* Override the base method.
Handles the save event of the detail record page. */
onCardSaved: function() {
/* Open the multiple selection dialog box. */
this.openLookupWithMultiSelect();
},
/* Override the base method that adds records to the detail. */
addRecord: function() {
/* Open the multiple selection dialog box. */
this.openLookupWithMultiSelect(true);
},
/* The method that returns the configuration object for the dialog box. */
getMultiSelectLookupConfig: function() {
return {
/* Set the root schema to [Opportunity]. */
rootEntitySchemaName: "Opportunity",
/* The root schema column. */
rootColumnName: "Opportunity",
/* Set the connected schema to [Contact]. */
relatedEntitySchemaName: "Contact",
/* The connected schema column. */
relatedColumnName: "Contact"
};
}
}
};
}); -
Save the changes.
Outcome of the example
-
Refresh the Creatio page.
-
Click the
button on the Contacts detail on the record page of the Opportunities section.
As a result, you will be able to select multiple records from the lookup.
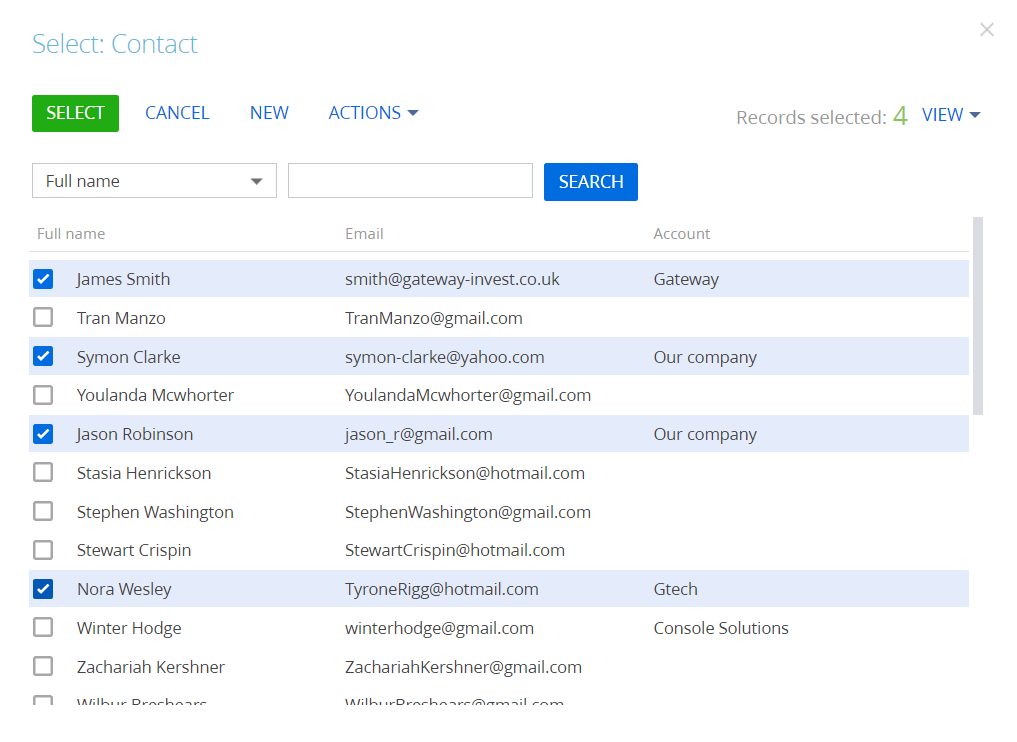
Once you confirm the selection, Creatio will add the records to the Contacts detail on the record page of the Opportunities section.
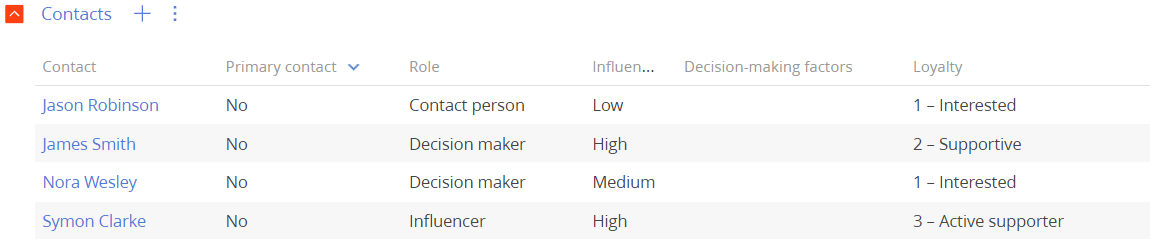