Set up filtering of bound lookup field values on a record page
Set up filtering of Country, State/province, City fields on the contact page. The index of selectable states/provinces must depend on the country selected in the Country field. The index of selectable cities must depend on the state/province selected in the State/province field.
Create a replacing view model schema of the contact page
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "ContactPageV2."
- Set Title to "Display schema - Contact card."
- Select "ContactPageV2" in the Parent object property.
-
Add the
BusinessRuleModule
module as a dependency to the declaration of the view model class. -
Implement the value filtering for connected lookup fields.
-
Take the following steps in the
rules
property for the City and Region columns:- Set the
ruleType
property toFILTRATION
. The value sets the business rule type. TheBusinessRuleModule.enums.RuleType
enumeration represents the rule types. - Set the
autocomplete
property totrue
. The property performs inverse filtering, i. e., populates the Country and State/province fields automatically depending on the selected city.
- Set the
-
Add the configuration object with the layout settings of the Country, State/province, City fields to the
diff
array of modifications.The base schema of the contact page has a rule for filtering cities by the country specified for the contact. To enable users to select a city from a country not specified for the contact, add a Country field.
View the source code of the replacement view model of the contact page below.
ContactPageV2/* Specify the BusinessRuleModule module as a dependency. */
define("ContactPageV2", ["BusinessRuleModule"], function(BusinessRuleModule) {
return {
/* The name of the record page object's schema. */
entitySchemaName: "Contact",
/* The business rules of the record page's view model. */
rules: {
/* The set of rules for the [City] column of the view model. */
"City": {
/* The filtering rule by the [Region] column value for the [City] column. */
"FiltrationCityByRegion": {
/* The FILTRATION rule type. */
"ruleType": BusinessRuleModule.enums.RuleType.FILTRATION,
/* Perform the inverse filtering. */
"autocomplete": true,
/* Clean the value when the [Region] column value changes. */
"autoClean": true,
/* The path to the filtering column in the [City] lookup schema referenced in the [City] column of the record page's view model. */
"baseAttributePatch": "Region",
/* The comparison operation type in the filter. */
"comparisonType": Terrasoft.ComparisonType.EQUAL,
/* Set the expression type to attribute (column) of the view model. */
"type": BusinessRuleModule.enums.ValueType.ATTRIBUTE,
/* The name of the view model column whose value to compare in the expression. */
"attribute": "Region"
}
},
/* The set of rules for the [Region] column of the view model. */
"Region": {
"FiltrationRegionByCountry": {
"ruleType": BusinessRuleModule.enums.RuleType.FILTRATION,
"autocomplete": true,
"autoClean": true,
"baseAttributePatch": "Country",
"comparisonType": Terrasoft.ComparisonType.EQUAL,
"type": BusinessRuleModule.enums.ValueType.ATTRIBUTE,
"attribute": "Country"
}
}
},
/* Display the fields on the record page. */
diff: [
/* The properties to add a [Country] field to the record page. */
{
/* Add the element to the page. */
"operation": "insert",
/* The meta name of the parent container to add the field. */
"parentName": "ProfileContainer",
/* Add the field to the parent element's collection of elements. */
"propertyName": "items",
/* The meta name of the field to add. */
"name": "Country",
/* The properties to pass to the element's constructor. */
"values": {
/* Set the field type to lookup. */
"contentType": Terrasoft.ContentType.LOOKUP,
/* Set up the field layout. */
"layout": {
/* The column number. */
"column": 0,
/* The row number. */
"row": 6,
/* The column span. */
"colSpan": 24
}
}
},
/* The properties to add a [Region] field to the record page. */
{
"operation": "insert",
"parentName": "ProfileContainer",
"propertyName": "items",
"name": "Region",
"values": {
"contentType": Terrasoft.ContentType.LOOKUP,
"layout": {
"column": 0,
"row": 7,
"colSpan": 24
}
}
},
/* The properties to add a [City] field to the record page. */
{
"operation": "insert",
"parentName": "ProfileContainer",
"propertyName": "items",
"name": "City",
"values": {
"contentType": Terrasoft.ContentType.LOOKUP,
"layout": {
"column": 0,
"row": 8,
"colSpan": 24
}
}
}
]
};
}); -
-
Click Save on the Designer's toolbar.
Outcome of the example
To view the outcome of the example, refresh the Contacts section page.
As a result, Creatio will add the Country, State/province, City connected lookup fields to the contact profile on the contact page.
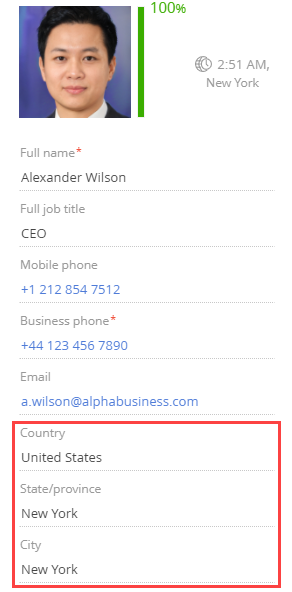
You can change the contact country in the contact profile.
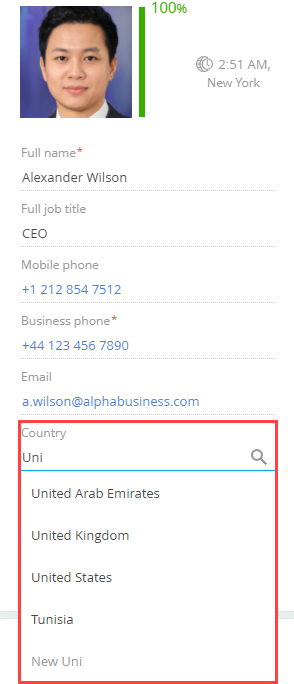
Creatio also filters the country selection box.
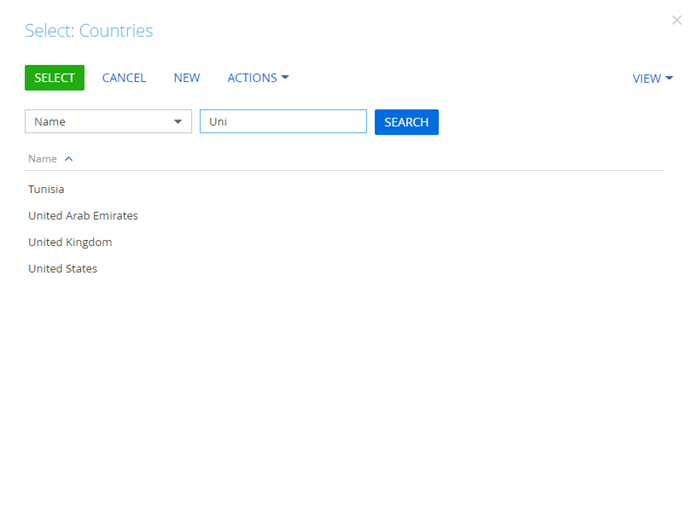
The index of selectable states/provinces depends on the country selected in the Country field. Creatio filters both the input field and state/province selection box.
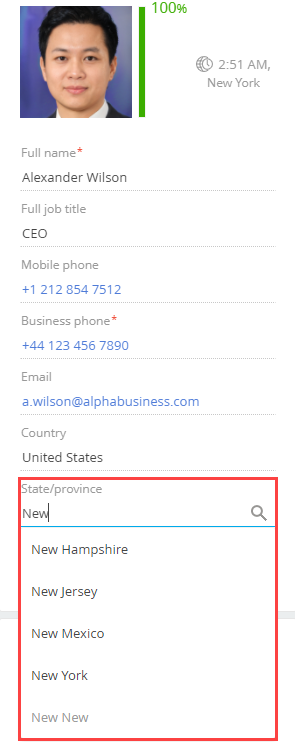
The index of selectable cities depends on the state/province selected in the State/province field. Creatio filters both the input field and city selection box.
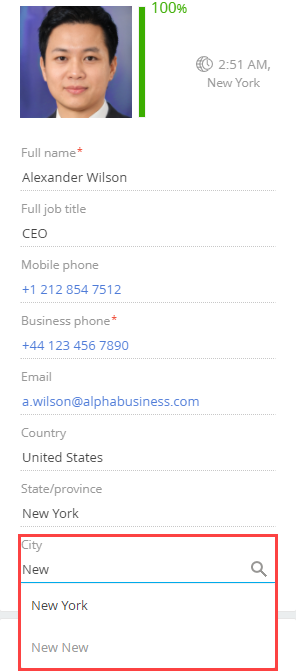