Implement the validation of a Date/Time type field on a record page
Validate the Created on field of the Date/Time type on the opportunity page. The date value of the Created on field must be earlier than the Closed on date.
Create a replacing view model schema of the opportunity page
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "OpportunityPageV2."
- Set Title to "Opportunity edit page."
- Select "OpportunityPageV2" in the Parent object property.
-
Add a localizable string.
-
Implement validation of the Date/Time type field.
To do this, implement the following methods in the
methods
property:dueDateValidator()
. The validator method that checks whether the condition is true.setValidationConfig()
. The overloaded base method that binds the validator method to the DueDate and CreatedOn columns.
View the source code of the replacing view model schema of the opportunity page below.
OpportunityPageV2define("OpportunityPageV2", [], function() {
return {
/* The name of the record page object's schema. */
entitySchemaName: "Opportunity",
/* The methods of the section page's view model. */
methods: {
/* The method that validates the [DueDate] and [CreatedOn] column values. */
dueDateValidator: function() {
/* The variable that stores the validation error message. */
var invalidMessage = "";
/* Check the [DueDate] and [CreatedOn] column values. */
if (this.get("DueDate") < this.get("CreatedOn")) {
/* If the [DueDate] column value is earlier than the [CreatedOn] column value, put the localizable string that contains the validation error message in the invalidMessage variable. */
invalidMessage = this.get("Resources.Strings.CreatedOnLessDueDateMessage");
}
/* The object whose property contains the validation error message. If the validation is successful, the object returns an empty string. */
return {
/* The validation error message. */
invalidMessage: invalidMessage
};
},
/* Overload the base method that initalizes the custom validators. */
setValidationConfig: function() {
/* Call the initialization of the parent view model's validators. */
this.callParent(arguments);
/* Add the dueDateValidator() validator method for the [DueDate] column. */
this.addColumnValidator("DueDate", this.dueDateValidator);
/* Add the dueDateValidator() validator method for the [CreatedOn] column. */
this.addColumnValidator("CreatedOn", this.dueDateValidator);
}
}
};
}); -
Click Save on the Designer's toolbar.
Outcome of the example
To view the outcome of the example:
- Refresh the Opportunities section page.
- Enter the date earlier than the Created on field value in the Closed on field.
As a result, Creatio will display the corresponding warning on the opportunity page.
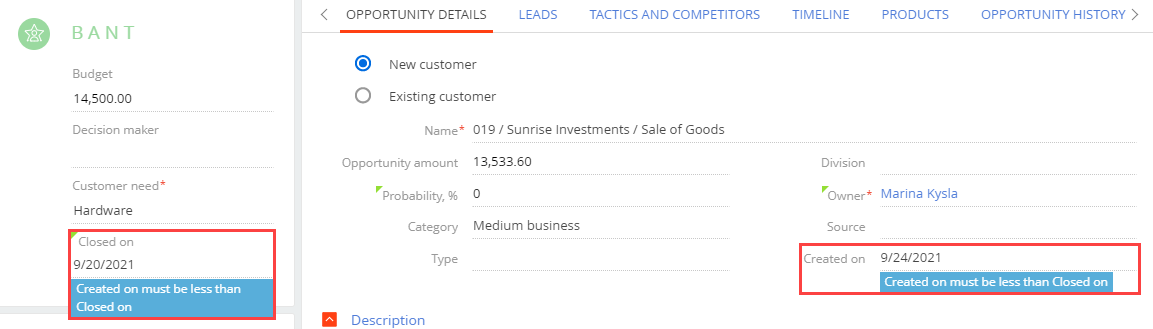
If you try to save an opportunity where the date value of the Closed on field is earlier than the Created on date, a message box will open.
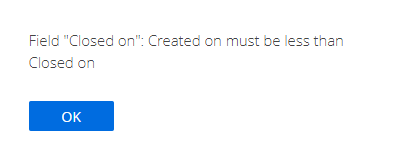