Keep fields on a record page unlocked
Set up the conditions that lock the fields on the invoice page. Lock the fields of completely paid invoices, i. e., invoices that have "Paid" selected in the Payment status field. Keep the Payment status field and Activities detail unlocked.
Create a replacing view model schema of the invoice page
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "InvoicePageV2."
- Set Title to "Invoice edit page."
- Select "InvoicePageV2" in the Parent object property.
-
Add the
InvoiceConfigurationConstants
module as a dependency to the declaration of the view model class. -
Set up the conditions that lock fields and keep fields unlocked.
-
Add the
IsModelItemsEnabled
attribute to theattributes
property. The attribute includes the mechanism that locks the fields. -
Implement the following methods in the
methods
property:getDisableExclusionsColumnTags()
. Keeps the column unlocked.getDisableExclusionsDetailSchemaNames()
. Keeps the detail unlocked.setCardLockoutStatus()
. Sets up the conditions that lock fields.onEntityInitialized()
. Overloads the base virtual method. Called after Creatio initializes the object schema of the record page.
-
Add a configuration object to the
diff
array of modifications. The object must contain the settings of theCardContentWrapper
container that locks the fields.
View the source code of the replacing view model schema of the invoice page below.
InvoicePageV2/* Specify the InvoiceConfigurationConstants module as a dependency. */
define("InvoicePageV2", ["InvoiceConfigurationConstants"], function(InvoiceConfigurationConstants) {
return {
/* The name of the record page object's schema. */
entitySchemaName: "Invoice",
/* The attributes of the view model. */
attributes: {
"IsModelItemsEnabled": {
/* The data type of the view model column. */
dataValueType: Terrasoft.DataValueType.BOOLEAN,
value: true,
/* The array of configuration objects that define the [IsModelItemsEnabled] attribute dependencies. */
dependencies: [{
/* The [IsModelItemsEnabled] column value depends on the [PaymentStatus] column value. */
columns: ["PaymentStatus"],
/* The handler method. */
methodName: "setCardLockoutStatus"
}]
}
},
/* The methods of the record page's view model. */
methods: {
/* Keep the [PaymentStatus] column unlocked. */
getDisableExclusionsColumnTags: function() {
return ["PaymentStatus"];
},
/* Keep the [ActivityDetailV2] detail unlocked. */
getDisableExclusionsDetailSchemaNames: function() {
return ["ActivityDetailV2"];
},
/* Set up the conditions that lock the fields. */
setCardLockoutStatus: function() {
var state = this.get("PaymentStatus");
if (state.value === InvoiceConfigurationConstants.Invoice.PaymentStatus.Paid) {
this.set("IsModelItemsEnabled", false);
} else {
this.set("IsModelItemsEnabled", true);
}
},
/* Overload the Terrasoft.BasePageV2.onEntityInitialized() base method. */
onEntityInitialized: function() {
/* Call the parent implementation of the method. */
this.callParent(arguments);
this.setCardLockoutStatus();
}
},
details: /**SCHEMA_DETAILS*/{}/**SCHEMA_DETAILS*/,
/* Display the locking container on the record page. */
diff: /**SCHEMA_DIFF*/[
{
/* Execute the operation that modifies the existing element. */
"operation": "merge",
/* The meta name of the parent container to lock the fields. */
"name": "CardContentWrapper",
/* The properties to pass to the element's constructor. */
"values": {
/* The view generator of the control. */
"generator": "DisableControlsGenerator.generatePartial"
}
}
]/**SCHEMA_DIFF*/
};
}); -
-
Click Save on the Designer's toolbar.
Outcome of the example
To view the outcome of the example, refresh the Invoices section page.
As a result, Creatio will lock most fields on the page of the invoice that has "Paid" selected in the Payment status field. The following remain unlocked:
- the Payment status field
- the Activities detail
- the fields that have the
enabled
property of thediff
array of modifications set totrue
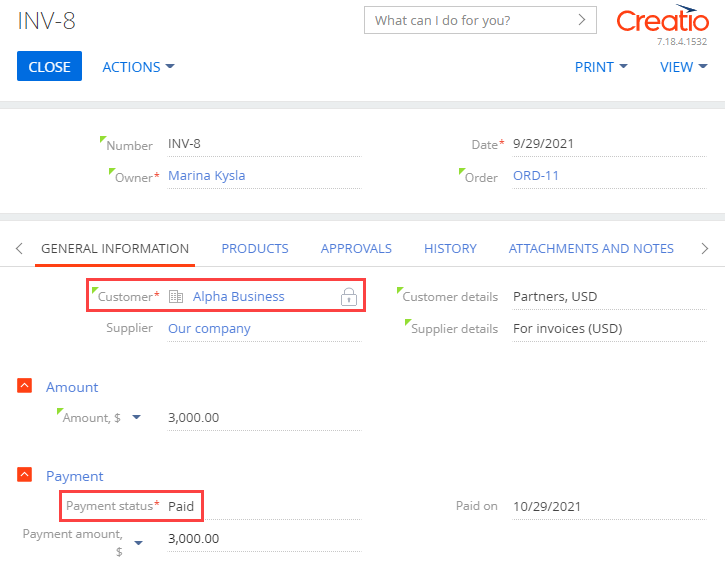