Set up action handling using a lookup for multiple section records
Set up action handling in the Activities section list. The action must open the Contacts lookup box. Set the selected contact as the owner of the selected activities.
Create a schema of the replacing section view model
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "ActivitySectionV2".
- Set Title to "Activities section".
- Set Parent object to "ActivitySectionV2".
-
Add a localizable string that contains the menu item caption.
-
Implement the menu item behavior. To do this, implement the following methods in the
methods
property:isCustomActionEnabled()
. Determines whether the menu item is available.setOwner()
. The action handler method that opens the Contacts lookup.lookupCallback()
. The callback method that sets the contact selected from the lookup as the owner of the selected activities.getSectionActions()
. The overloaded parent schema method that retrieves the section action collection.
View the source code of the replacing view model schema of the section below.
ActivitySectionV2define("ActivitySectionV2", ["ConfigurationConstants"], function(ConfigurationConstants) {
return {
/* The name of the section object schema. */
entitySchemaName: "Activity",
/* The methods of the section view model. */
methods: {
/* Determine whether the menu item is available. */
isCustomActionEnabled: function() {
/* Attempt to retrieve the array of the selected record IDs. */
var selectedRows = this.get("SelectedRows");
/* Return true if the array contains items (at least one list record is selected), return false otherwise. */
return selectedRows ? (selectedRows.length > 0) : false;
},
/* The action handler method. Opens the [Contacts] lookup. */
setOwner: function() {
/* Define the lookup configuration. */
var config = {
/* The [Contact] schema. */
entitySchemaName: "Contact",
/* Disable multiple selection. */
multiSelect: false,
/* Display the [Name] column. */
columns: ["Name"]
};
/* Open the lookup with a particular configuration and the callback function that triggers when a user clicks the [Select] button. */
this.openLookup(config, this.lookupCallback, this);
},
/* Set the contact selected from the lookup as the owner of the selected activities. */
lookupCallback: function(args) {
/* The ID of the selected lookup record. */
var activeRowId;
/* Retrieve the selected lookup records. */
var lookupSelectedRows = args.selectedRows.getItems();
if (lookupSelectedRows && lookupSelectedRows.length > 0) {
/* Retrieve the ID of the first selected lookup record. */
activeRowId = lookupSelectedRows[0].Id;
}
/* Retrieve the array of the selected record IDs. */
var selectedRows = this.get("SelectedRows");
/* Run handling if at least one record was selected and the owner was selected in the lookup. */
if ((selectedRows.length > 0) && activeRowId) {
/* Create an instance of the batch query class. */
var batchQuery = this.Ext.create("Terrasoft.BatchQuery");
/* Update each of the selected records. */
selectedRows.forEach(function(selectedRowId) {
/* Create an UpdateQuery class instance with the Activity root schema. */
var update = this.Ext.create("Terrasoft.UpdateQuery", {
rootSchemaName: "Activity"
});
/* Apply the filter to determine the record to update. */
update.enablePrimaryColumnFilter(selectedRowId);
/* Set the [Owner] column to the ID of the contact selected from the lookup. */
update.setParameterValue("Owner", activeRowId, this.Terrasoft.DataValueType.GUID);
/* Add a record update query to the batch query. */
batchQuery.add(update);
}, this);
/* Execute the batch server query. */
batchQuery.execute(function() {
/* Update the list. */
this.reloadGridData();
}, this);
}
},
/* Overload the base virtual method that returns the section action collection. */
getSectionActions: function() {
/* Call the parent method implementation that retrieves the collection of the initialized section actions. */
var actionMenuItems = this.callParent(arguments);
/* Add a separator line. */
actionMenuItems.addItem(this.getButtonMenuItem({
Type: "Terrasoft.MenuSeparator",
Caption: ""
}));
/* Add a menu item to the section action index. */
actionMenuItems.addItem(this.getButtonMenuItem({
/* Bind the menu item caption to the localizable schema string. */
"Caption": { bindTo: "Resources.Strings.SetOwnerCaption" },
/* Bind the action handler method. */
"Click": { bindTo: "setOwner" },
/* Bind the property of the menu item availability to the value returned by the isCustomActionEnabled method. */
"Enabled": { bindTo: "isCustomActionEnabled" },
/* Support the multiple selection mode. */
"IsEnabledForSelectedAll": true
}));
/* Return the expanded section action collection. */
return actionMenuItems;
}
}
};
}); -
Click Save on the Designer&'s toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Activities section page.
The section single record mode is used by default. To select multiple list records, click Select multiple records in the menu of the Actions button. This changes the visual view of the list. You will see record selection elements appear.
As a result, Creatio will add the Assign Owner action to the order page.
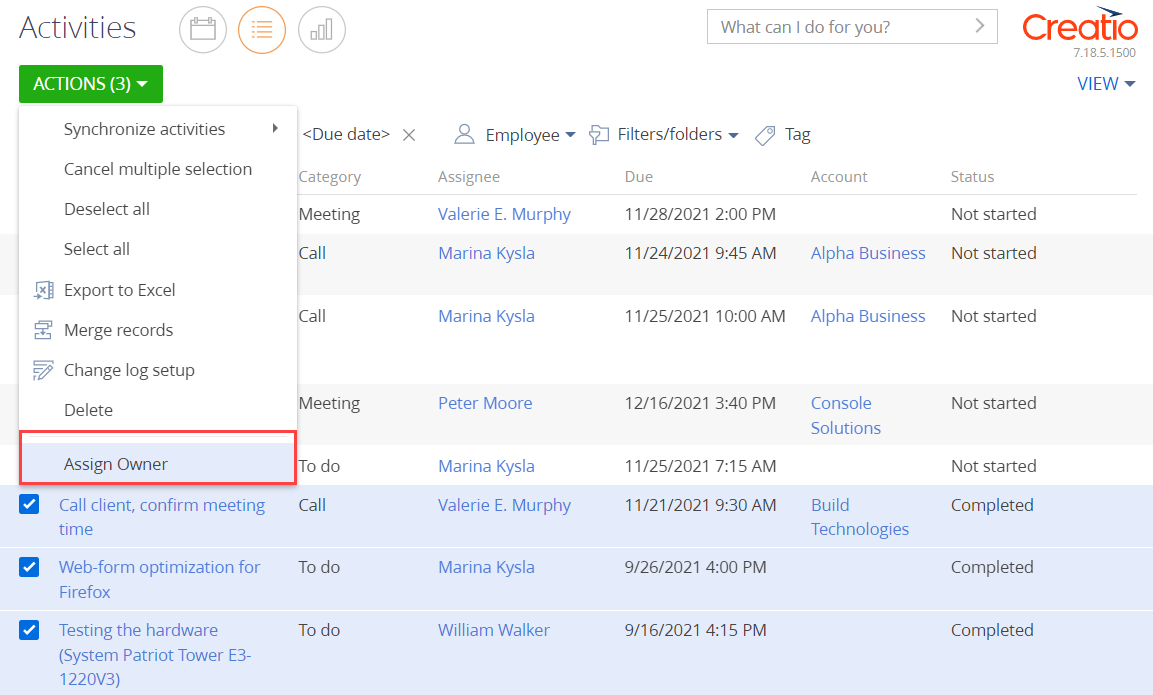
Run the Assign Owner action to open the Contacts lookup box. Creatio sets the selected contact as the owner of the selected activities in the Assignee column.
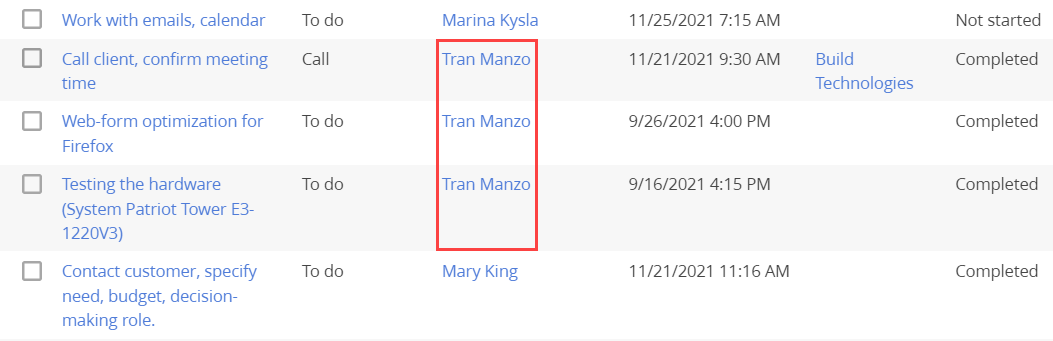
To cancel the multiple selection mode, click Cancel multiple selection in the menu of the Actions button.
If you do not select any records in the Activities section list, the Assign Owner action will be inactive.
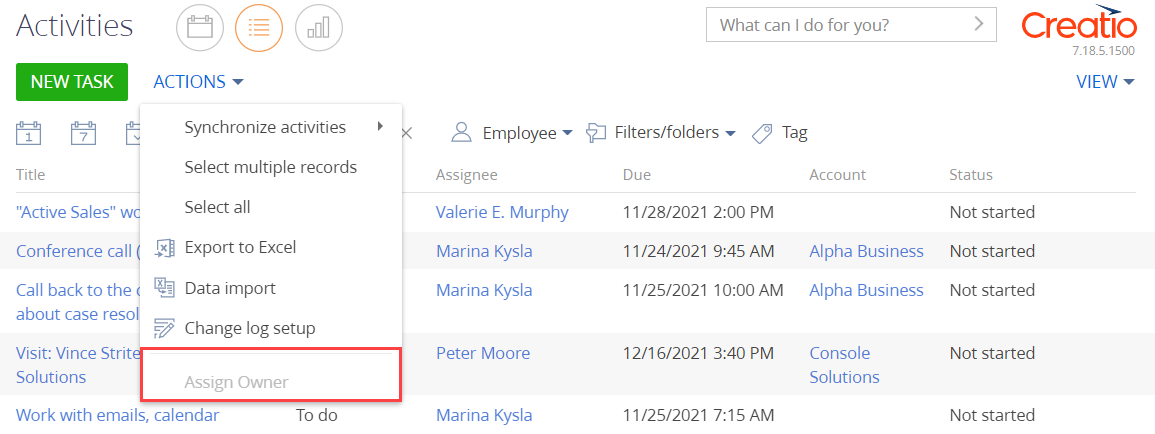