Add an action for multiple records to the section
The example is relevant to Sales Creatio products.
Add an action for records of the Orders section list. The action must display the index of accounts for the selected orders in the message box.
Create a schema of the replacing section view model
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "OrderSectionV2".
- Set Title to "Order section".
- Set Parent object to "OrderSectionV2".
-
Add a localizable string that contains the menu item caption.
-
Implement the menu item behavior. To do this, implement the following methods in the
methods
property:isCustomActionEnabled()
. Determines whether the menu item is available.showOrderInfo()
. The action handler method that displays the index of accounts for the selected orders in the message box.getSectionActions()
. The overloaded parent schema method that retrieves the section's action collection.
The primary column values of the selected records are stored in the
SelectedRows
property of the section view model. You can use these values to get the values uploaded to the field list of the selected objects. For example, from a regular list's data collection stored in theGridData
view model property of the list.View the source code of the replacing view model schema of the section below.
OrderSectionV2define("OrderSectionV2", ["OrderConfigurationConstants"], function(OrderConfigurationConstants) {
return {
/* The name of the section object schema. */
entitySchemaName: "Order",
/* The methods of the section view model. */
methods: {
/* Determine whether the menu item is available. */
isCustomActionEnabled: function() {
/* Attempt to retrieve the array of the selected record IDs. */
var selectedRows = this.get("SelectedRows");
/* Return true if the array contains items (at least one list record is selected), return false otherwise. */
return selectedRows ? (selectedRows.length > 0) : false;
},
/* The action handler method. Displays the index of accounts in the message box. */
showOrdersInfo: function() {
/* Retrieve the array of the selected record IDs. */
var selectedRows = this.get("SelectedRows");
/* Retrieve the data collection of the list records. */
var gridData = this.get("GridData");
/* The variable that stores the object model of the selected order. */
var selectedOrder = null;
/* The variable that stores the account name for the selected order. */
var selectedOrderAccount = "";
/* The variable that generates the message box text. */
var infoText = "";
/* Process the array of the selected record IDs. */
selectedRows.forEach(function(selectedRowId) {
/* Retrieve the object model of the selected order. */
selectedOrder = gridData.get(selectedRowId);
/* Retrieve the account name for the selected order. The column must be added to the list. */
selectedOrderAccount = selectedOrder.get("Account").displayValue;
/* Add the account name to the message box text. */
infoText += "\n" + selectedOrderAccount;
});
/* Display the message box. */
this.showInformationDialog(infoText);
},
/* Overload the base virtual method that returns the section action collection. */
getSectionActions: function() {
/* Call the parent method implementation that retrieves the collection of the initialized section actions. */
var actionMenuItems = this.callParent(arguments);
/* Add a separator line. */
actionMenuItems.addItem(this.getButtonMenuItem({
Type: "Terrasoft.MenuSeparator",
Caption: ""
}));
/* Add a menu item to the section action index. */
actionMenuItems.addItem(this.getButtonMenuItem({
/* Bind the menu item caption to the localizable schema string. */
"Caption": {bindTo: "Resources.Strings.AccountsSectionAction"},
/* Bind the action handler method. */
"Click": {bindTo: "showOrdersInfo"},
/* Bind the property of the menu item availability to the value returned by the isCustomActionEnabled method. */
"Enabled": {bindTo: "isCustomActionEnabled"},
/* Support the multiple selection mode. */
"IsEnabledForSelectedAll": true
}));
/* Return the expanded section action collection. */
return actionMenuItems;
}
}
};
}); -
Click Save on the Designer's toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Orders section page.
The section single record mode is used by default. To select multiple list records, click Select multiple records in the menu of the Actions button. This changes the visual view of the list. You will see record selection elements appear.
As a result, Creatio will add the Accounts for the selected orders action to the order page.
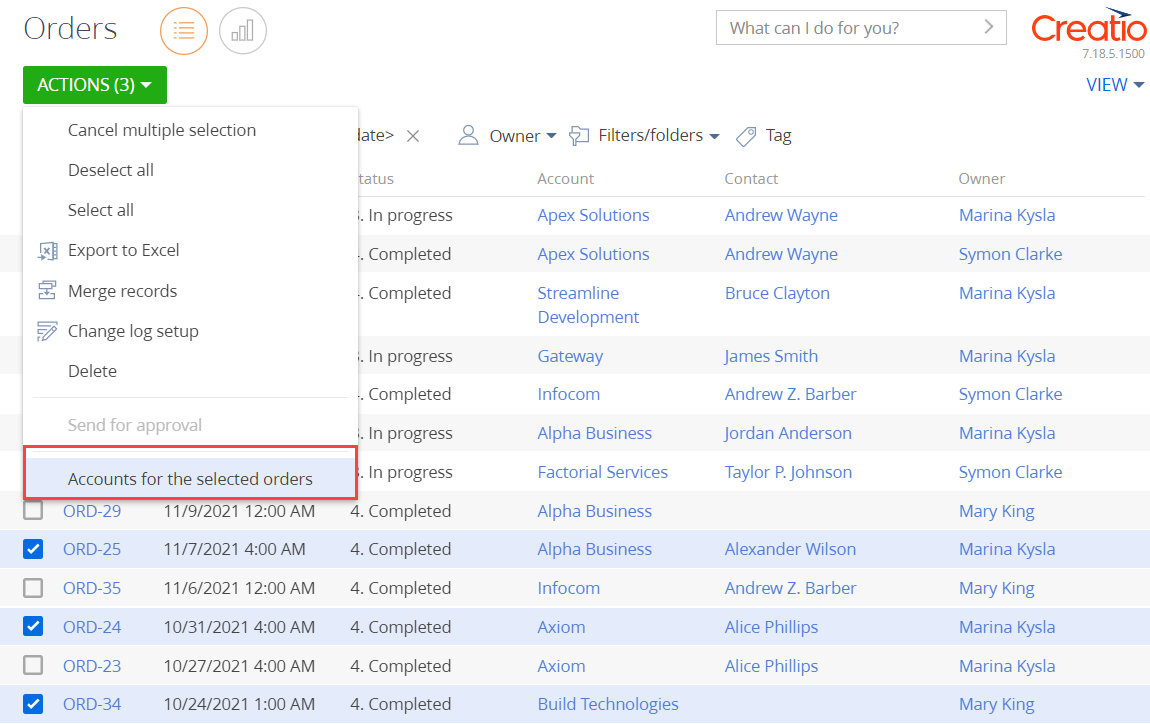
Run the Accounts for the selected orders action to bring up the message box that displays the account list for the selected orders.
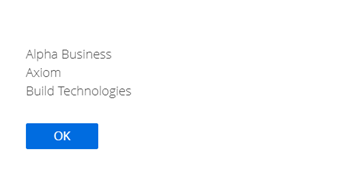
To cancel the multiple selection mode, click Cancel multiple selection in the menu of the Actions button.
If you do not select any records in the Orders section list, the Accounts for the selected orders action will be inactive.
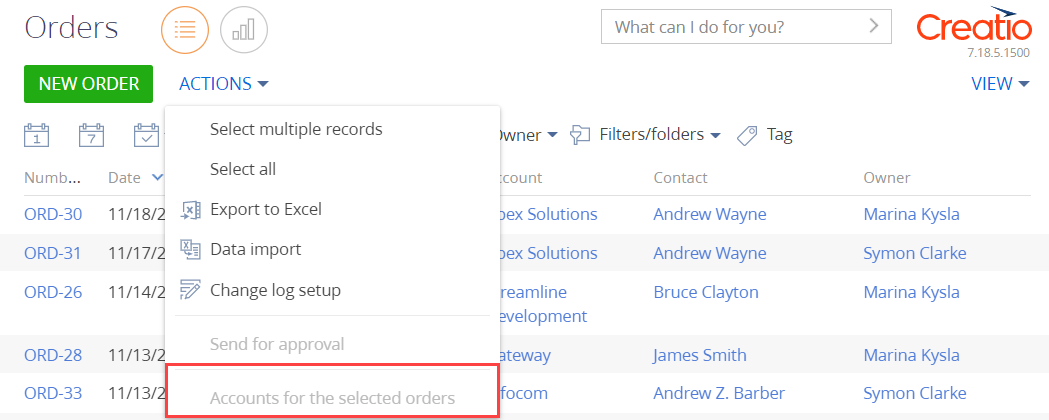
Resources
Package with example implementation
Tech Hour - How to use buttons and actions to empower Creatio pages