Display the time zone of a contact on a communication panel tab
Display the time zone of a contact on the CTI panel tab of the communication panel when you search for a contact. Use the current time of a contact.
1. Create a replacing view model schema of the page for found subscribers
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "SubscriberSearchResultItem."
- Set Title to "Found subscriber schema."
- Select "SubscriberSearchResultItem" in the Parent object property.
-
Add the
TimezoneGenerator
andTimezoneMixin
modules as dependencies to the declaration of the view model class. TheTimezoneGenerator
module creates an element that displays the time zone of the contact. TheTimezoneMixin
module searches for the time zone of the contact. -
Implement a mechanism that displays the time zone.
-
Add the
IsShowTimeZone
attribute to theattributes
property. The attribute determines the visibility of the time zone element. -
Add the
TimezoneMixin
mixin to themixins
property. To run the search for the contact time zone, pass the contact ID to theinit
method of theTimezoneMixin
mixin. As a result, Creatio will set the following attributes:TimeZoneCaption
. The name of the contact's time zone and current time.TimeZoneCity
. The name of the city in the time zone.
-
Implement the following methods in the
methods
property:constructor()
. The class constructor.isContactType()
. Returns the flag that indicates that the subscriber is a contact.
-
Add a configuration object with the display settings of the contact's time zone to the
diff
array of modifications.-
index
property. Configures the element layout.SubscriberSearchResultItemContainer
container elements:- 0 index: subscriber photo.
- 1 index: subscriber info.
- 2 index: subscriber phones.
Assign 2 to the
index
property of the array of modifications to display the contact time zone between the subscriber info and the index of phone numbers. -
wrapClass
property. Controls the styles. An element generator. Use thesubscriber-data
CSS class to define styles for text elements in the schema.
-
View the source code of the replacing view model schema of the page for found subscribers below.
SubscriberSearchResultItemdefine("SubscriberSearchResultItem", ["TimezoneGenerator", "TimezoneMixin"], function() {
return {
/* The attributes of the page view model. */
attributes: {
/* The name of the attribute that determines the visibility of the time zone element. */
"IsShowTimeZone": {
/* The data type of the view model column. */
"dataValueType": Terrasoft.DataValueType.BOOLEAN,
/* The attribute type. */
"type": Terrasoft.ViewModelColumnType.VIRTUAL_COLUMN,
/* The default value. */
"value": true
}
},
/* The mixins of the page view model. */
mixins: {
/* Enable the mixin. */
TimezoneMixin: "Terrasoft.TimezoneMixin"
},
/* The methods of the page view model. */
methods: {
/* The class constructor. */
constructor: function() {
/* Call the base constructor. */
this.callParent(arguments);
/* The flag that indicates that the subscriber is a contact. */
var isContact = this.isContactType();
/* If the subscriber is a contact, display the element. */
this.set("IsShowTimeZone", isContact);
/* If the subscriber is a contact. */
if (isContact) {
/* The contact ID. */
var contactId = this.get("Id");
/* Search for the contact time zone. */
this.mixins.TimezoneMixin.init.call(this, contactId);
}
},
/* Return the flag that indicates that the subscriber is a contact. */
isContactType: function() {
/* The subscriber type. */
var type = this.get("Type");
/* Return the comparison result. */
return type === "Contact";
}
},
/* The array of modifications of the page view model. */
diff: [
{
/* Add the element to the page. */
"operation": "insert",
/* The meta name of the parent container to add the element. */
"parentName": "SubscriberSearchResultItemContainer",
/* Add the element to the parent element's collection of elements. */
"propertyName": "items",
/* The meta name of the element to add. */
"name": "TimezoneContact",
/* The properties to pass to the element constructor. */
"values": {
/* The element type. */
"itemType": Terrasoft.ViewItemType.CONTAINER,
/* Call the method of the generator to create the view configuration. */
"generator": "TimezoneGenerator.generateTimeZone",
/* Bind the container visibility to the attribute. */
"visible": {"bindTo": "IsShowTimeZone"},
/* The name of the CSS class. */
"wrapClass": ["subscriber-data", "timezone"],
/* Bind the caption to the attribute. */
"timeZoneCaption": {"bindTo": "TimeZoneCaption"},
/* Bind the city to the attribute. */
"timeZoneCity": {"bindTo": "TimeZoneCity"}
},
/* The element layout in the parent container. */
"index": 2
}
]
};
}); -
-
Click Save on the Designer's toolbar.
As a result, Creatio will display the current time and city of the contact.
2. Add display styles of the time zone
The view model schema of a page for found subscribers does not support visual styles. As such, take the following steps to add the styles:
- Create a module schema. Define the styles in it.
- Add the style module to the dependencies of the page for found subscribers.
1. Create a module schema
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Module on the section list toolbar.
-
Fill out the schema properties:
- Set Code to "UsrSubscriberSearchResultItemCSS."
- Set Title to "SubscriberSearchResultItemCSS."
Click Apply to apply the properties.
-
Go to the LESS node of the object structure and set up the needed visual styles of the time zone.
Set up the visual styles of the time zone/* Set up the visual styles of the element to add. */
.ctiPanelMain .search-result-items-list-container .timezone {
/* Top padding. */
padding-top: 13px;
/* Bottom margin. */
margin-bottom: -10px;
}
/* Set up the visual styles of the contact time. */
.ctiPanelMain .search-result-items-list-container .timezone-caption {
/* Left padding. */
padding-left: 10px;
/* Text color. */
color: rgb(255, 174, 0);
/* Set the text font to bold. */
font-weight: bold;
}
/* Set up the visual styles of the contact city. */
.ctiPanelMain .search-result-items-list-container .timezone-city {
/* Left padding. */
padding-left: 10px;
} -
Go to the JS node of the object structure and add the module code.
UsrSubscriberSearchResultItemCSSdefine("UsrSubscriberSearchResultItemCSS", [], function() {
return {};
}); -
Click Save on the Designer's toolbar.
2. Edit the view model schema of the page for found subscribers
To use the module and its styles in the schema of the page for found subscribers:
-
Open the
SubscriberSearchResultItem
view model schema of the page for found subscribers. -
Add the
UsrSubscriberSearchResultItemCSS
module to the dependencies of theSubscriberSearchResultItem
schema.View the source code of the edited schema of the page for found subscribers.
SubscriberSearchResultItemdefine("SubscriberSearchResultItem", ["TimezoneGenerator", "TimezoneMixin", "css!UsrSubscriberSearchResultItemCSS"], function() {
return {
/* The attributes of the page view model. */
attributes: {
/* The name of the attribute that controls the visibility of the time zone element. */
"IsShowTimeZone": {
/* The data type of the view model column. */
"dataValueType": Terrasoft.DataValueType.BOOLEAN,
/* The attribute type. */
"type": Terrasoft.ViewModelColumnType.VIRTUAL_COLUMN,
/* The default value. */
"value": true
}
},
/* The mixins of the page view model. */
mixins: {
/* Enable the mixin. */
TimezoneMixin: "Terrasoft.TimezoneMixin"
},
/* The methods of the page view model. */
methods: {
/* The class constructor. */
constructor: function() {
/* Call the base constructor. */
this.callParent(arguments);
/* The flag that indicates that the subscriber is a contact. */
var isContact = this.isContactType();
/* If the subscriber is a contact, display the element. */
this.set("IsShowTimeZone", isContact);
/* If the subscriber is a contact. */
if (isContact) {
/* The contact ID. */
var contactId = this.get("Id");
/* Search for the contact time zone. */
this.mixins.TimezoneMixin.init.call(this, contactId);
}
},
/* Return the flag that indicates that the subscriber is a contact. */
isContactType: function() {
/* The subscriber type. */
var type = this.get("Type");
/* Return the comparison result. */
return type === "Contact";
}
},
/* The array of modifications of the page view model. */
diff: [
{
/* Add the element to the page. */
"operation": "insert",
/* The meta name of the parent container to add the element. */
"parentName": "SubscriberSearchResultItemContainer",
/* Add the element to the parent element's collection of elements. */
"propertyName": "items",
/* The meta name of the element to add. */
"name": "TimezoneContact",
/* The properties to pass to the element constructor. */
"values": {
/* The element type. */
"itemType": Terrasoft.ViewItemType.CONTAINER,
/* Call the method of the generator to create the view configuration. */
"generator": "TimezoneGenerator.generateTimeZone",
/* Bind the container visibility to the attribute. */
"visible": {"bindTo": "IsShowTimeZone"},
/* The name of the CSS class. */
"wrapClass": ["subscriber-data", "timezone"],
/* Bind the caption to the attribute. */
"timeZoneCaption": {"bindTo": "TimeZoneCaption"},
/* Bind the city to the attribute. */
"timeZoneCity": {"bindTo": "TimeZoneCity"}
},
/* The element layout in the parent container. */
"index": 2
}
]
};
}); -
Click Save on the Designer's toolbar.
Outcome of the example
To view the outcome of the example:
- Open the CTI panel tab of the communication panel.
- Search for a subscriber.
As a result, when you search for a contact, Creatio will display their time zone on the CTI panel tab of the communication panel. The current time of the contact will be used.
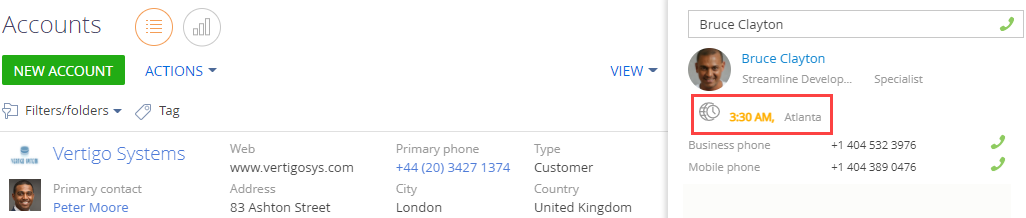