How to create a mini-page
Glossary Item Box
Introduction
Bpm'online mini-pages were introduced in version 7.7. System users see mini-pages as an improved pop-up tool tip that contains additional section-specific functions. Mini-pages contain shortcuts to a number of actions, such as viewing the location of an account on the map, sending emails and making calls. Mini-pages are displayed when a user places their cursor over the hyperlinks that open edit pages in the [Accounts] and [Contacts] sections.
The primary purpose of mini-pages is:
- Give users the ability to get information about a record without opening the edit page.
- Give users the ability to quickly add records in sections by filling out only required fields as opposed to filling out the entire edit page.
The structure of the mini-page view model schema is the same as the general structure of the system schema modules. The required parameters in the structure of the mini-page are:
- entitySchemaName, which contains the schema name of the object to which the mini-page will be bound,
- diff array that contains modifications.
These parameters are used to build the module view in the system UI.
Other items of the general schema structure, such as attributes, methods, mixins and messages can be used to implement the required functions. Use them to add custom controls, register messages, and implement business logic in various mini-pages. The appearance of the mini-page items can be customized.
How to add a mini-page in an existing section
To add a custom mini-page in an existing bpm'online section:
- Add the view model schema for the card in the custom package. Set the following required properties for the created schema: [Title], [Name], [Package] and [Parent object]. Select "BaseMiniPage" as the parent object.
- Use an SQL query to modify the SysModuleEdit system table in the bpm'online database.
- Add necessary data and functions in the schema source code. Specify schema name of the object to which the mini-page will be bound in the entitySchemaName item and make at least one modification in the diff array.
- Apply styles to the mini-page.
![]() |
Attention! To bind the created mini-page to the objects of a certain section, add the unique Id of the mini-page to the [Unique identifier of mini page schema] (MiniPageSchemaUId) column of these objects. Currently, the only way to do this is by modifying the SysModuleEdit system table in the bpm'online database using an SQL query. Please double-check any custom SQL queries made to the bpm'online database. Executing an invalid SQL query may damage existing data and disrupt system operation. |
You can add a mini-page to any system object. Below is an example of how to create a mini-page for the [Knowledge Base] (KnowledgeBase) object.
Case description
Create a custom mini-page for the [Knowledge base] section. The mini-page will be used to view a set of fields and download attachments of knowledge base articles.
Case implementation
1. Creating a model view schema of the mini-page
In the setup mode, go to the [Configuration] section and select the [Add] > [
Fig. 1. Adding card view schema
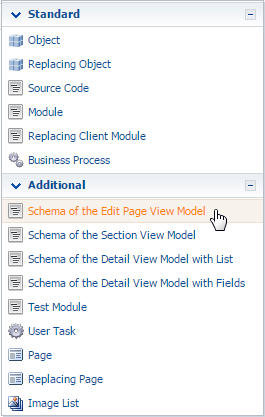
Fill out the properties of the mini-page view schema as shown on fig. 2.
Fig. 2. Fill out the mini-page properties.
2. Registering a mini-page in the database.
Execute the following SQL query to make changes in the database:
DECLARE -- Name of the view schema of the created mini-page. @ClientUnitSchemaName NVARCHAR(100) = 'KnowledgeBaseArticleMiniPage', -- Name of the object schema to which the mini-page is bound. @EntitySchemaName NVARCHAR(100) = 'KnowledgeBase' UPDATE SysModuleEdit SET MiniPageSchemaUId = ( SELECT TOP 1 UId FROM SysSchema WHERE Name = @ClientUnitSchemaName ) WHERE SysModuleEntityId = ( SELECT TOP 1 Id FROM SysModuleEntity WHERE SysEntitySchemaUId = ( SELECT TOP 1 UId FROM SysSchema WHERE Name = @EntitySchemaName AND ExtendParent = 0 ) );
As a result of this query, a unique Id for the mini-page is added to the MiniPageSchemaUId field of the SysModuleEdit table (fig. 3).
Fig. 3. Unique Id value of mini-page in the SysModuleEdit database table
3. Displaying fields of the primary object
The mini-page code structure is identical to that of the common edit page. Before adding fields, specify the object schema, as you would for any common edit page. In this case the object schema name is "KnowledgeBase".
Adding fields to the mini-page is similar to adding fields to an edit page: all necessary changes are added to the diff array.
The only difference that results from the limited space of a mini-page, is the need to hide field titles and specify the display mode.
"values": { "labelConfig": { // Hiding field title "visible": false }, // Specifying display mode in mini-page. "isMiniPageModelItem": true }
A base mini-page contains the following items:
- MiniPage — Terrasoft.GridLayout — card field.
- HeaderContainer — Terrasoft.Container — card title (is placed in the first row of card field).
Add the [Name] field
On this stage, the mini-page is already functional and the remaining steps are optional.
Source code of the mini-page view model schema:
define("KnowledgeBaseArticleMiniPage", [], function() { return { entitySchemaName: "KnowledgeBase", details: /**SCHEMA_DETAILS*/{}/**SCHEMA_DETAILS*/, messages: {}, mixins: {}, attributes: {}, methods: {}, diff: /**SCHEMA_DIFF*/[ { "operation": "insert", "name": "Name", "parentName": "HeaderContainer", "propertyName": "items", "index": 0, "values": { "labelConfig": { "visible": false }, "isMiniPageModelItem": true } }, { "operation": "insert", "name": "Notes", "parentName": "MiniPage", "propertyName": "items", "values": { "labelConfig": { "visible": false }, "isMiniPageModelItem": true, "layout": { "column": 0, "row": 1, "colSpan": 24 } } } ]/**SCHEMA_DIFF*/ }; });
4. How to add a functional button to a mini-page
One of the case requirements is the ability to download knowledge base article attachments using mini-pages.
Working with additional data is enabled via the mechanism that displays it as the drop-down list of a preconfigured button. To add a button for selecting attachments of a knowledge base article:
- Add the button description to the diff array – the [FilesButton] item.
- Add the attribute that connects primary and additional records – the [Article] virtual button.
- Add methods for working with the drop-down list of the file selection button:
- override the init() method;
- override the EntityInitialized() method;
- set the [Article] attribute value using the setArticleInfo() method;
- get data about the attachments of the current knowledge base article using the getFiles(callback, scope) method;
- populate the drop-down list collection of the file select button using the initFilesMenu(files) method;
- initiate file loading and adding to the drop-down list of the file select button using the fillFilesExtendedMenuData() method;
- initiate file download
5. How to apply style to a mini-page
Create the KnowledgeBaseArticleMiniPageCss module on the LESS tab and specify a style.
div[data-item-marker='KnowledgeBaseArticleMiniPageContainer'] > div { width: 34em; }
In the designer of the mini-page schema, specify the dependency of the style module and add loading of this module in the source code.
Example of complete code of a mini-page:
define("KnowledgeBaseArticleMiniPage", ["terrasoft", "KnowledgeBaseFile", "ConfigurationConstants", "css!KnowledgeBaseArticleMiniPageCss"], function(Terrasoft, KnowledgeBaseFile, ConfigurationConstants) { return { entitySchemaName: "KnowledgeBase", details: /**SCHEMA_DETAILS*/{}/**SCHEMA_DETAILS*/, messages: {}, mixins: {}, attributes: { Article: { type: Terrasoft.ViewModelColumnType.VIRTUAL_COLUMN, referenceSchemaName: "KnowledgeBase" } }, methods: { /** * Initializes drop-down list collection of the file select button. */ init: function() { this.callParent(arguments); this.initExtendedMenuButtonCollections("File", ["Article"], this.close); }, /** * Initializes value of the attribute that binds primary and additional records. * Fills out drop-down list collection of the file select button. */ onEntityInitialized: function() { this.callParent(arguments); this.setArticleInfo(); this.fillFilesExtendedMenuData(); }, /** * Initializes loading of the files and adds them to the drop-down list of the file select button. */ fillFilesExtendedMenuData: function() { this.getFiles(this.initFilesMenu, this); }, /** * Sets value of the attribute that binds primary and additional records. */ setArticleInfo: function() { this.set("Article", { value: this.get(this.primaryColumnName), displayValue: this.get(this.primaryDisplayColumnName) }); }, /** * Gets information about files of the current knowledge base article. */ getFiles: function(callback, scope) { var esq = this.Ext.create("Terrasoft.EntitySchemaQuery", { rootSchema: KnowledgeBaseFile }); esq.addColumn("Name"); var articleFilter = this.Terrasoft.createColumnFilterWithParameter( this.Terrasoft.ComparisonType.EQUAL, "KnowledgeBase", this.get(this.primaryColumnName)); var typeFilter = this.Terrasoft.createColumnFilterWithParameter( this.Terrasoft.ComparisonType.EQUAL, "Type", ConfigurationConstants.FileType.File); esq.filters.addItem(articleFilter); esq.filters.addItem(typeFilter); esq.getEntityCollection(function(response) { if (!response.success) { return; } callback.call(scope, response.collection); }, this); }, /** * Fills out the drop-down list collection of the file select button. */ initFilesMenu: function(files) { if (files.isEmpty()) { return; } var data = []; files.each(function(file) { data.push({ caption: file.get("Name"), tag: file.get("Id") }); }, this); var recipientInfo = this.fillExtendedMenuItems("File", ["Article"]); this.fillExtendedMenuData(data, recipientInfo, this.downloadFile); }, /** * Initiates downloading of the selected file. */ downloadFile: function(id) { var element = document.createElement("a"); element.href = "../rest/FileService/GetFile/" + KnowledgeBaseFile.uId + "/" + id; document.body.appendChild(element); element.click(); document.body.removeChild(element); } }, diff: /**SCHEMA_DIFF*/[ { "operation": "insert", "name": "Name", "parentName": "HeaderContainer", "propertyName": "items", "index": 0, "values": { "labelConfig": { "visible": false }, "isMiniPageModelItem": true } }, { "operation": "insert", "name": "Notes", "parentName": "MiniPage", "propertyName": "items", "values": { "labelConfig": { "visible": false }, "isMiniPageModelItem": true, "layout": { "column": 0, "row": 1, "colSpan": 24 } } }, { "operation": "insert", "parentName": "HeaderContainer", "propertyName": "items", "name": "FilesButton", "values": { "itemType": Terrasoft.ViewItemType.BUTTON, // Button image setup. "imageConfig": { // The image must first be added to the mini-page resources. "bindTo": "Resources.Images.FilesImage" }, // Drop-down list setup. "extendedMenu": { // Drop-down list item name. "Name": "File", // Name of the mini-page attribute that binds the primary and additional records "PropertyName": "Article", // Button click handler setup. "Click": { "bindTo": "fillFilesExtendedMenuData" } } }, "index": 1 } ]/**SCHEMA_DIFF*/ }; });
6. How to save a mini-page schema
After saving the schema and updating the [Knowledge base] section page, the mini-page with the list of attachments will be displayed If a user hovers their cursor over the knowledge base article title (fig. 4).
Fig. 4. Functional mini-page