Add a button to a section record row
Add the Show age button to the row of the active record in the Contacts section. Display the age of the selected contact in a pop-up box on a button click.
Create a schema of the replacing section view model
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "ContactSectionV2."
- Set Title to "Contact section."
- Set Parent object to "ContactSectionV2."
-
Add a localizable string.
-
Implement the button logic.
-
Implement the following methods in the
methods
property:onActiveRowAction()
– assigns a handler method to the button located in the row of the active section record.onShowAgeButtonClicked()
– the button click handler method. Returns the contact age in the pop-up box.
-
Add a configuration object with the settings that determine the button position to the
diff
array of modifications.
Reference the selected record via the
ActiveRow
attribute of the section view model. The attribute returns the value of the primary column of the selected record. In turn, you can use the value of the primary column of the selected record to get the values loaded into the field list of the selected object.View the source code of the replacing view model of the section page below:
ContactSectionV2define("ContactSectionV2", ["ContactSectionV2Resources"], function (resources) {
return {
/* The name of the section entity schema. */
entitySchemaName: "Contact",
/* The methods of the section view model. */
methods: {
onActiveRowAction: function (buttonTag) {
switch (buttonTag) {
case "showAgeButton":
this.onShowAgeButtonClicked();
break;
default:
this.callParent(arguments);
break;
}
},
/* The button click handler method. */
onShowAgeButtonClicked: function () {
var message = "";
var activeRow = this.getActiveRow();
var recordId = activeRow.get("Id");
/* Create a Terrasoft.EntitySchemaQuery class instance with the Contact root schema. */
var esq = this.Ext.create("Terrasoft.EntitySchemaQuery", {
rootSchemaName: "Contact"
});
/* Add the age column. */
esq.addColumn("Age", "Age");
/* Get the record from the selection by ID. */
esq.getEntity(recordId, function(result) {
if (!result.success) {
this.showInformationDialog("Error");
return;
}
message += "Age of contact is "+ result.entity.get("Age");
this.showInformationDialog(message);
}, this);
}
},
/* Display the button in the section. */
diff: /**SCHEMA_DIFF*/[
/* Properties to add the custom button to the section. */
{
/* Run the operation that inserts the element to the page. */
"operation": "insert",
/* The meta name of the added button. */
"name": "DataGridActiveRowShowAgeButton",
/* The meta name of the parent container to add the button. */
"parentName": "DataGrid",
/* Add the button to the element collection of the parent element. */
"propertyName": "activeRowActions",
/* The properties to pass to the element’s constructor. */
"values": {
"className": "Terrasoft.Button",
"style": Terrasoft.controls.ButtonEnums.style.GREEN,
/* Bind the button title to the localizable schema string. */
"caption": resources.localizableStrings.ShowAgeOfContactButtonCaption,
"tag": "showAgeButton"
}
}
]/**SCHEMA_DIFF*/
};
}); -
-
Click Save on the Designer’s toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Contacts section page.
As a result, Creatio will add the Show Age button to the row of the active record in the Contacts section. Click Show Age to open a pop-up box that displays the contact age.
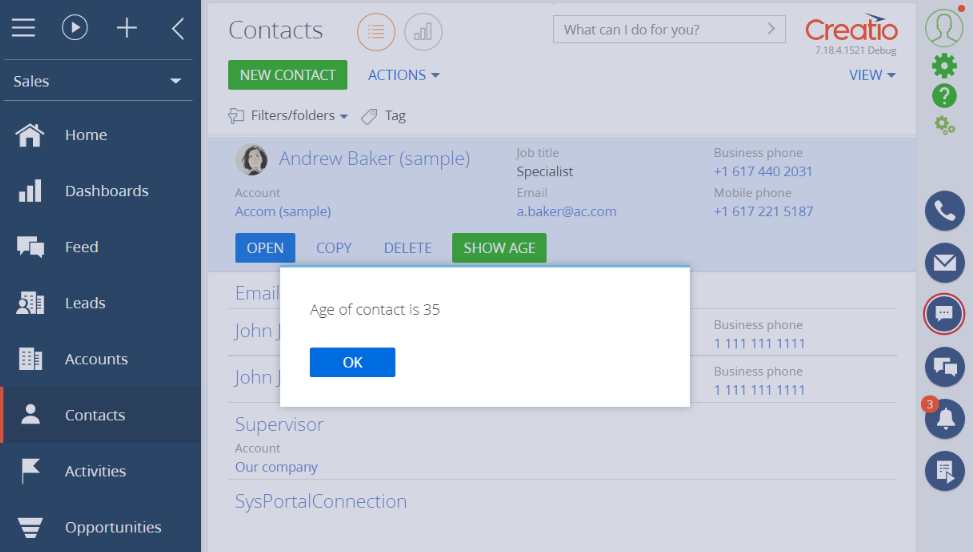