Record page is a UI element that stores the information about Creatio business objects organized as fields, tabs, details, and dashboards. The name of the record page corresponds to the name of the Creatio object. For example, account page, contact page, etc. The purpose of the record page is management of section list records. Each Creatio section includes one or more record pages.
Each record page is represented by a client module schema. For example, the ContactPageV2 schema of the Uiv2 package configures the contact page. The BasePageV2 schema of the NUI package implements the functionality of a base record page. Record page schemas must inherit the BasePageV2 schema.
The record page types are as follows:
- The page that adds records. Creates a section list record.
- The page that edits records. Modifies an existing section list record.
Record page containers
Creatio stores the UI elements related to the record page in the corresponding containers. Configure the containers in the base schema of the record page or the schema of the replacing record page. The record page type does not affect the containers.
View the main record page containers in the figure below.
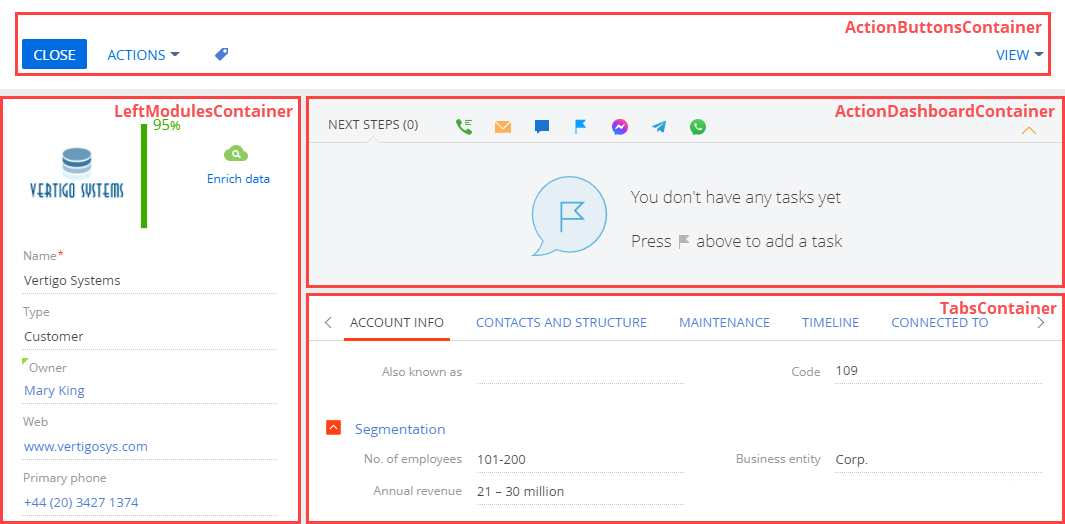
- ActionButtonsContainer stores the action buttons of the record page.
- LeftModulesContainer stores the main data input and edit fields.
- ActionDashboardContainer stores the action panel and workflow bar.
- TabsContainer stores the tabs that group input and edit fields by a category. For example, current employment.
Create a record page
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add on the section list toolbar and select the type of the view model schema.
-
Select Page view model to create a new record page.
-
Select Replacing view model to replace an existing record page.
-
-
Fill out the following schema properties.
- Code is the schema name. Required. The code must contain a prefix specified in the Prefix for object name (the SchemaNamePrefix code) system setting. The default prefix is Usr.
- Title is the localizable schema title. Required.
-
Parent object is the record page schema whose functionality to inherit.
- Select "BasePageV2" to create a new record page.
- Select the schema of the needed record page to replace an existing record page. For example, select the AccountPageV2 schema of the Uiv2 package to display a custom account page in Creatio.
- Implement the record page logic.
- Click Save on the Module Designer’s toolbar.
Set up the record page
Use controls to set up the record page.
Creatio lets you set up the record page in the following ways:
- Add standard page controls.
- Edit standard page controls.
- Add custom page controls.
The main page controls are as follows:
- Action dashboard. Learn more about setting up the action dashboard in a separate article: Action dashboard.
- Field. Learn more about setting up page fields in a separate article: Field.
- Button. Learn more about setting up buttons in a separate article: Button.
Add a custom action to a record page
Creatio lets you add a custom action to the Actions drop-down menu of the record page. The BasePageV2 schema of the base record page implements the menu.
To add a custom action to the record page:
- Create a record page or replacing record page. To do this, take steps 1-3 in the instruction to create a record page.
- Override the protected getActions() virtual method that returns the page action list. The page action list is an instance of the Terrasoft.BaseViewModelCollection class. Each action is a view model.
- Pass the getButtonMenuItem() method to the addItem() method as a parameter. The addItem() method adds a custom action to the collection.
- Pass the configuration object to the getButtonMenuItem() callback method as a parameter. The getButtonMenuItem() method creates an instance of the action view model.
- Implement the action configuration object that lets you set the properties of the action view model explicitly or use the base binding mechanism.
View the template to add a custom action to a record page below.
1. Create a replacing view model schema of the order page
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the following schema properties.
- Set Code to "OrderPageV2."
- Set Title to "Order edit page."
- Set Parent object to "OrderPageV2."
-
Add a localizable string that contains the menu item caption.
- Click the
button in the context menu of the Localizable strings node.
-
Fill out the localizable string properties:
- Set Code to "InfoActionCaption."
- Set Value to "Show execution date."
- Click Add to add a localizable string.
- Click the
-
Implement the menu item logic.
To do this, implement the following methods in the methods property:
- isRunning() – checks whether the order is at the In progress stage and the menu item is available.
- showOrderInfo() – the action handler method. Displays the scheduled order execution date in the notification box. The record page action applies to a specific object opened on the page. To access the field values of the record page object in the action handler method, use the get() (retrieve the value) and set() (assign the value) view model methods.
- getActions() – an overridden base method. Returns the action collection of the replacing page.
View the source code of the order page’s replacing view model below.
- Click Save on the Designer’s toolbar.
As a result, Creatio will add the Show execution date action to the page of the order at the In progress stage.
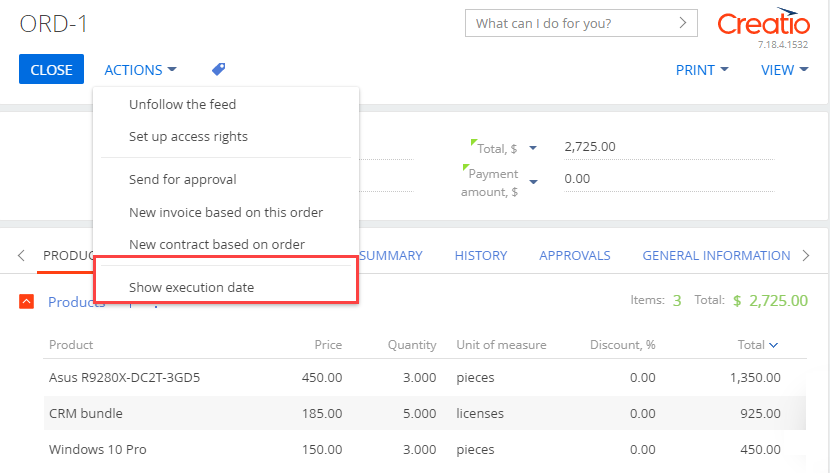
Creatio does not display the custom page action in the vertical list view.
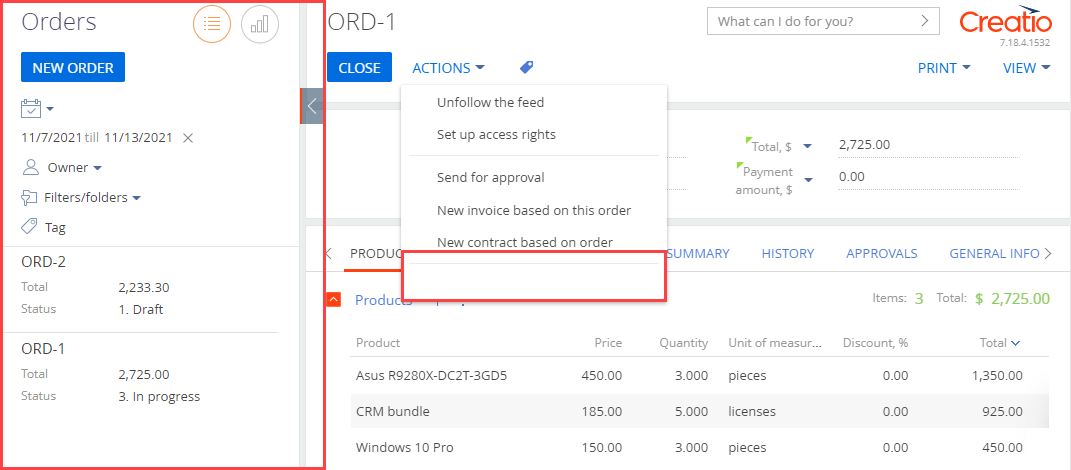
To ensure the page action is displayed correctly, add the following to the replacing view model schema of the section:
- The localizable string that contains the menu item caption.
- The method that determines the menu item availability.
2. Create a replacing view model schema of the section
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the following schema properties.
- Set Code to "OrderSectionV2."
- Set Title to "Order section."
- Set Parent object to "OrderSectionV2."
- Add a localizable string that contains the menu item caption. To do this, take step 4 of the procedure to create a replacing view model schema of the order page.
-
Implement the menu item logic. To do this, implement the isRunning() method in the methods property. The method checks if the order is at In progress stage and determines the menu item availability.
View the source code of the section page’s replacing view model schema below.
- Click Save on the Designer’s toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Orders section page.
As a result, Creatio will add the Show execution date action to the order page.
If the order is at the In progress stage, the Show execution date action will be active.
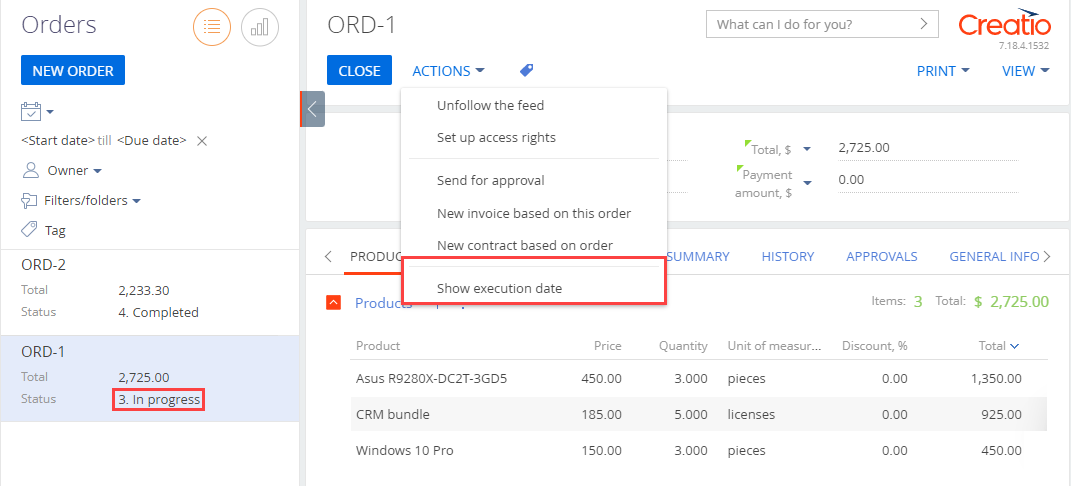
Run the Show execution date action to bring up the notification box that displays the scheduled order execution date.
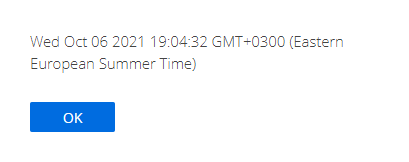
If the order is not at the In progress stage, the Show execution date action will be inactive.
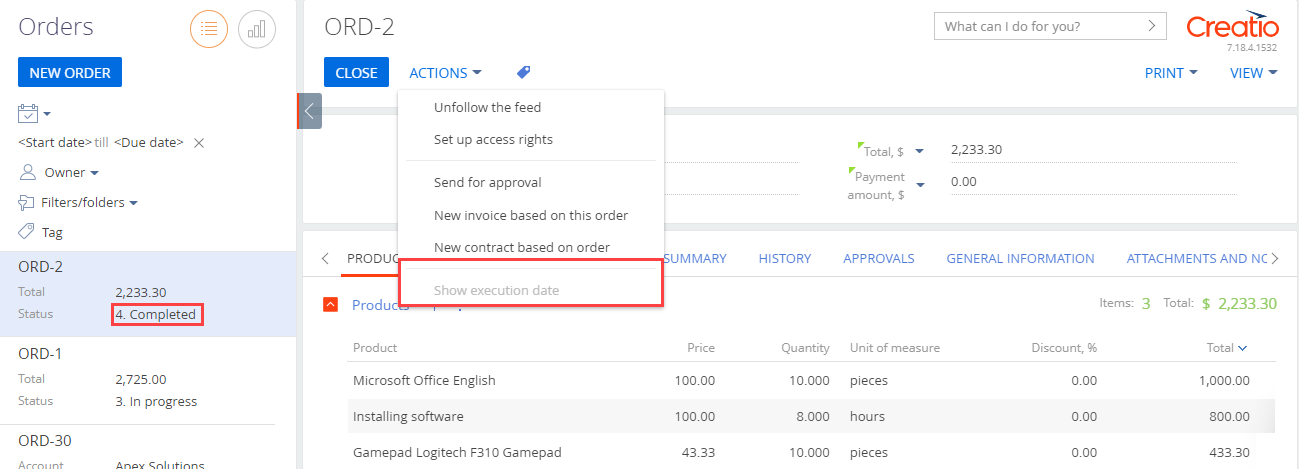
BaseEntityPage is the base schema of the record page. Implemented in the NUI package. This is a view model schema. Learn more about the schema properties in a separate article: Client schema structure. Record page schemas must inherit the BaseEntityPage schema.
Messages
Name | Mode | Direction | Description |
---|---|---|---|
Update |
Address | Publish | Communicates the change details of the record page. |
Card |
Address | Bidirectional | Communicates the record page change. |
Card |
Broadcasting | Bidirectional | Communicates the record page processing. |
Entity |
Broadcasting | Bidirectional | Communicates the object initialization and sends the object information. |
Show |
Address | Publish | Displays the process page. |
The Terrasoft.core.enums.MessageMode enumeration represents the message modes, and the Terrasoft.core.enums.MessageDirectionType enumeration represents the message directions. Learn more about the MessageMode enumeration in the JS class library. Learn more about the MessageDirectionType enumeration in the JS class library.
Attributes
Initializes the entity.
The array of default values for the object.
The availability flag for model elements.
The configuration details.
The Terrasoft.core.enums.DataValueType enumeration represents the attribute types. Learn more about the DataValueType enumeration in the JS class library.
Methods
Initializes the record page.
{Function} callback | The callback function. |
{Object} scope | The method execution context. |
Saves details to a chain.
{Function} next | The callback function. |
Returns the detail ID.
{String} detailName | The detail name. |
Loads the detail. If the detail is loaded, displays it once more.
{Object} config | The detail configuration. |
Checks whether the user has edit permission.
{Function} callback | The callback function. |
{Object} scope | The method execution context. |
Generates a search query for the display value.
{Object} config | The detail configuration. |
Populates the lookup fields.
{String} name | The object schema name. |
{String} value | The object value. |
{Function} callback | The callback function. |
{Object} scope | The method execution context. |
Returns true if a business rule can clear the object column.
BasePageV2 is a base schema of a mini page. Implemented in the NUI package. This is a view model schema. Learn more about the schema properties in a separate article: Client schema.
Messages
Name | Mode | Direction | Description |
---|---|---|---|
Update |
Address | Publishing | Updates the page header. |
Grid |
Address | Subscription | Retrieves the ID of the selected list record when the record changes. |
Update |
Address | Subscription | Changes the model parameter value. |
Update |
Address | Subscription | Updates the add page header. |
Close |
Address | Publishing | Closes the add page. |
Open |
Address | Subscription | Opens the add page. |
Open |
Address | Publishing | Opens an add page chain. |
Get |
Address | Subscription | Returns the add page status. |
Is |
Address | Publishing | Informs of the add page change. |
Get |
Address | Publishing | Retrieves the name of the active view. |
Get |
Address | Subscription | Retrieves data about the master entity of the mini add page. |
Get |
Address | Subscription | Retrieves page tooltips. |
Get |
Address | Subscription | Retrieves column data. |
Get |
Broadcasting | Publishing | Sends entity column data when the column changes. |
Reload |
Address | Publishing | Reloads the section row depending on the primary column value. |
Validate |
Address | Subscription | Launches the add page validation. |
Re |
Address | Publishing | Relaunches the action dashboard validation. |
Re |
Address | Subscription | Updates the action dashboard configuration. |
Reload |
Broadcasting | Publishing | Reloads the dashboard elements. |
Reload |
Address | Publishing | Reloads the dashboard elements on the current page. |
Can |
Broadcasting | Subscription | Allows or prohibits changing the current history state. |
Is |
Address | Subscription | Returns the changed entity. |
Is |
Address | Subscription | Returns true if the filtered columns changed. |
Update |
Broadcasting | Bidirectional | Updates the parent lookup record in accordance with the configuration. |
Update |
Broadcasting | Bidirectional | States that data must be reloaded on the following launch. |
The Terrasoft.core.enums.MessageMode enumeration represents the message modes, and the Terrasoft.core.enums.MessageDirectionType enumeration represents the message directions. Learn more about the MessageMode enumeration in the JS class library. Learn more about the MessageDirectionType enumeration in the JS class library.
Attributes
The visibility flag of LeftModulesContainer.
The visibility flag of ActionDashboardContainer.
The visibility flag of DcmActionsDashboardContainer.
Custom attributes of DcmActionsDashboardContainer.
The visibility flag of the page title.
Saves the name of the active tab.
The name of the GridData view.
The name of the AnalyticsData view.
Attribute of the add page body when the add page is displayed or hidden.
Attribute of the add page body when the main header is displayed or hidden.
The array of page header column names.
Flag that marks the page as not available.
Flag that marks the page as customizable.
Operations of the add page.
Flag that forces entity reload on the following launch.
The Terrasoft.core.enums.DataValueType enumeration represents the attribute data types. Learn more about the DataValueType enumeration in the JS class library.
Methods
Handles the Discard button click event.
{String} [callback] | The callback function. |
{Terrasoft. |
The method execution scope. |
Adds switchpoint views to the drop-down list of the View button.
{Terrasoft. |
Items in the drop-down list of the View button. |
Adds the Open section wizard item to the drop-down list of the View button.
{Terrasoft. |
Items in the drop-down list of the View button. |
Returns the query filter collection.
Initializes the column names of the page header.
Returns the values of ViewModel parameters.
{Array} parameters | Parameter names. |
Sets the ViewModel parameters.
{Object} parameters | Parameter values. |
Returns the ID of the lookup page module.
ReloadCard message handler. Reloads the data of the add page entity.
{Object[]} defaultValues | Array of default values. |
Returns the column information.
{String} columnName | The name of the column. |
Returns the visibility status of container tabs.
Returns the visibility status of the Print button’s drop-down list items (i. e., reports).
{String} reportId | Report ID. |
Retrieves the section view.
Runs a business process using the start button for global business processes.
{Object} tag | ID of the business process schema. |
Runs a business process with parameters.
{Object} config | The configuration object. |
Checks for unsaved data. Edit the cached config.result if the data was changed but not saved.
{String} cacheKey | The key of the cached configuration object. |
Displays an error message if the add page is invalid.