Modify sales pipeline calculations
You can modify the way values are calculated for the sales pipeline dashboard element in the Opportunities section. To do this, you need to create a new module for calculations and replace the sales pipeline display client schema.
To modify the sales pipeline calculations:
- Create a new class inherited from
FunnelBaseDataProvider
and specify the calculation logic. - Create a replacing
FunnelChartSchema
client schema and use the new calculation class in it.
Example of modifying the calculations displayed in the "Number of opportunities" view of the sales pipeline
Case description
Modify the sales pipeline calculation algorithms by replacing the number of opportunities with the number of products added to opportunities.
Case implementation algorithm
1. Create a new module in the custom package
Create a new calculation provider client module in the custom package. Calculation provider is a class responsible for selecting, filtering and processing data for sales pipeline chart.
Specify a name and caption for the new module, for example, UsrFunnelByProductCountDataProvider
(Fig. 1).
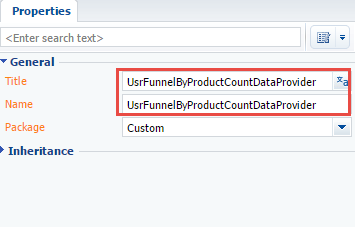
2. Add localizable strings
Add a string with the Number of products
value to the collection of localizable strings of the created module. To do this, right-click the LocalizableStrings structure node and select Add from the context menu. Set the properties for the new string as shown on Fig. 2.
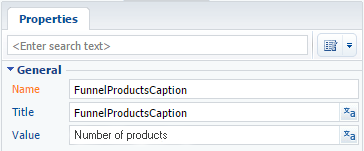
Add CntOpportunity
localizable string with the Number of opportunities
value in the similar way.
3. Add implementation to the provider module
To modify sales pipeline calculations, override the following methods:
addQueryColumns
column generation method for data selection- methods for selection data processing.
To process one record from the selection, define the getSeriesDataConfigByItem
method. To process the whole collection, define the prepareFunnelResponseCollection
method. To filter the records, define the applyFunnelPeriodFilters
method.
Below is the source code of the new calculation provider module for the sales pipeline.
define("UsrFunnelByProductCountDataProvider", ["ext-base", "terrasoft", "UsrFunnelByProductCountDataProviderResources", "FunnelBaseDataProvider"], function(Ext, Terrasoft, resources) {
// Defining a new calculation provider.
Ext.define("Terrasoft.configuration.UsrFunnelByProductCountDataProvider", {
// Inheriting from the basic provider.
extend: "Terrasoft.FunnelBaseDataProvider",
// New provider short name
alternateClassName: "Terrasoft.UsrFunnelByProductCountDataProvider",
// Collection processing method
prepareFunnelResponseCollection: function(collection) {
this.callParent(arguments);
},
// Extending the FunnelBaseDataProvider base model method.
// Sets the column number of products for data sampling
addQueryColumns: function(entitySchemaQuery) {
// Parent method calling
this.callParent(arguments);
// Adds the number of products column to the sample
entitySchemaQuery.addAggregationSchemaColumn("[OpportunityProductInterest:Opportunity].Quantity",
Terrasoft.AggregationType.SUM, "ProductsAmount");
},
// Extending the FunnelBaseDataProvider base class method.
// Sets sample filtration
applyFunnelPeriodFilters: function(filterGroup) {
// Parent method calling
this.callParent(arguments);
// Creates a filter group.
var endStageFilterGroup = Terrasoft.createFilterGroup();
// Sets the group operator type.
endStageFilterGroup.logicalOperation = Terrasoft.LogicalOperatorType.OR;
// Sets the filter that shows whether the sale stage is over yet.
endStageFilterGroup.addItem(
Terrasoft.createColumnIsNullFilter(this.getDetailColumnPath("DueDate")));
// Sets the filter that shows whether the sale stage is final.
endStageFilterGroup.addItem(
Terrasoft.createColumnFilterWithParameter(Terrasoft.ComparisonType.EQUAL,
this.getDetailColumnPath("Stage.End"), true, Terrasoft.DataValueType.BOOLEAN));
filterGroup.addItem(endStageFilterGroup);
},
// Extending the FunnelBaseDataProvider base model method.
// Processes data for the stages in the pipeline.
getSeriesDataConfigByItem: function(responseItem) {
// Object that stores localizable strings.
var lcz = resources.localizableStrings;
// Receives a stage data object from the parent method.
var config = this.callParent(arguments);
// Receives data about the number of products in an opportunity from the sample result.
var products = responseItem.get("ProductsAmount");
products = Ext.isNumber(products) ? products : 0;
// Formats the strings.
var name = Ext.String.format("{0}<br/>{1}: {2}<br/>{3}: {4}",
config.menuHeaderValue, lcz.CntOpportunity, config.y, lcz.FunnelProductsCaption, products);
var displayValue = Ext.String.format("<br/>{0}: {1}", lcz.FunnelProductsCaption, products);
// Installs new data in the data object and returns it.
return Ext.apply(config, {
name: name,
displayValue: displayValue
});
}
});
});
4. Create a sales pipeline replacing schema
To use the new provider module in the calculations, override the sales pipeline calculation provider generator method.
To do this, create a replacing client module and specify FunnelChartSchema
as a parent (Fig. 3).
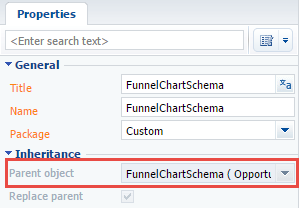
Add the new calculation module to dependencies (the Dependencies
section), by specifying its name in the Dependency field and the UsrFunnelByProductCountDataProvider
value in the Name field (Fig. 4).
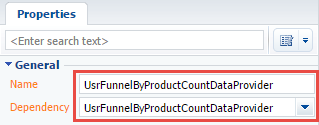
5. Specify the new calculation provider in the sales pipeline replacing schema
To do this, override the getProvidersCollectionConfig method in the replacing schema that gets the configuration object with the collection of providers.
define("FunnelChartSchema", ["UsrFunnelByProductCountDataProvider"], function() {
return {
entitySchemaName: "Opportunity",
methods: {
getProvidersCollectionConfig: function() {
// Calls parent method.
// Gets array of providers.
var config = this.callParent();
// Searches data provider in the measurement by the number of opportunities.
var byCount = Terrasoft.findItem(config, {tag: "byNumberConversion"});
// Replaces with new class.
byCount.item.className = "Terrasoft.UsrFunnelByProductCountDataProvider";
return config;
}
}
};
});
After saving the schema, the new calculation module will be used in the sales pipeline and the sales pipeline itself will display the total number of products by stages (Fig. 5).
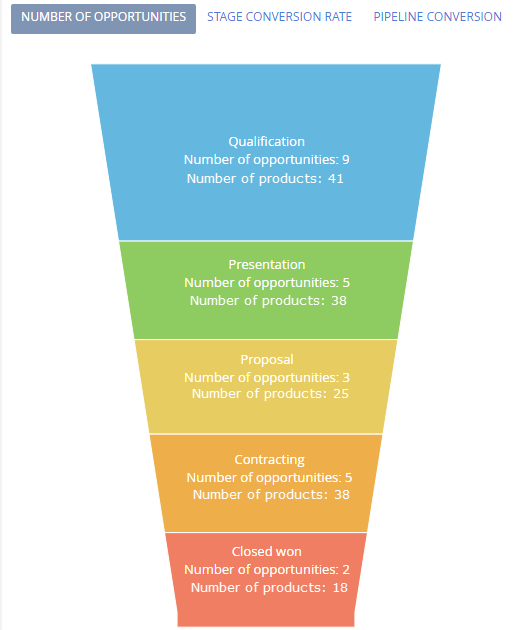