Add a chat routing mechanism
To set up chat routing, select a routing rule from the drop-down list in the queue settings. This determines the mechanism that distributes chats to agents.
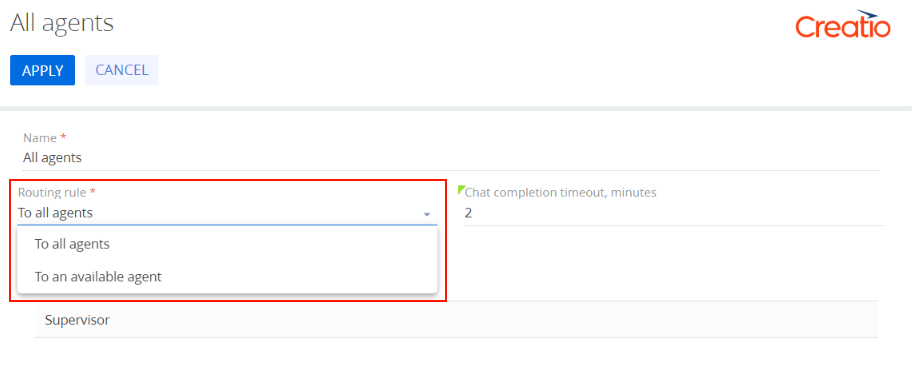
View an example that adds a custom distribution mechanism below.
Add a custom chat distribution mechanism. Grant the system user (Supervisor) access to new chats. Grant agents access to distributed chats.
1. Add a new chat routing rule
-
Click the
button to open the System Designer.
-
Go to the System setup block → Lookups.
-
Use the filter at the top to find the "Rules for routing chat queue operators" lookup.
-
Open the lookup content and add a new rule:
- Set Name to "Test rule."
- Set Code to "TestRule."
2. Create a class that implements the IOperatorRoutingRule interface
On this step, you can create a class that inherits from the BaseOperatorRoutingRule
class or new class that implements the IOperatorRoutingRule
interface.
The BaseOperatorRoutingRule
class contains abstract PickUpFreeQueueOperators
and GetChatOperator
methods you must implement.
/// <summary>
/// Pick up and return queue agent IDs.
/// </summary>
/// <param name="chatId"><see cref="OmniChat"/> The agent ID.</param>
/// <param name="queueId"><see cref="ChatQueue"/> The instance ID.</param>
/// <returns>The queue agent IDs.</returns>
protected abstract List<Guid> PickUpFreeQueueOperators(Guid chatId, Guid queueId);
/// <summary>
/// Returns the <see cref="OmniChat"/> agent ID.
/// </summary>
/// <param name="chatId"><see cref="OmniChat"/> The agent ID.</param>
/// <returns>The chat agent.</returns>
protected abstract Guid GetChatOperator(Guid chatId);
Moreover, the BaseOperatorRoutingRule
already contains the implementation of the IOperatorRoutingRule
interface. View the implementation logic below.
public List <Guid> GetOperatorIds(string chatId, Guid queueId) {
var parsedChatId = Guid.Parse(chatId);
var chatOperator = GetChatOperator(parsedChatId);
return chatOperator.IsNotEmpty() ? new List <Guid> {
chatOperator
}: PickUpFreeQueueOperators(parsedChatId, queueId);
}
If the chat is already assigned to an agent (the user ID is specified in the [OperatorId]
column), select the agent. If the chat is not assigned to an agent, retrieve the agent or agents as List<Guid>
using the PickUpFreeQueueOperators
method. In this case, Guid
are agent IDs in the [SysAdminUnit]
table. The agents that have the corresponding IDs receive new chat notifications and can access the chat in the communication panel.
Create a TestOperatorRoutingRule
class that implements the basic logic that routes the chat to the system user (Supervisor).
To create a class:
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Source code on the section list toolbar.
-
Go to the Schema Designer and fill out the schema properties:
- Set Code to "UsrTestOperatorRoutingRule."
- Set Title to "TestOperatorRoutingRule."
Click Apply to apply the properties.
-
Add the source code in the Schema Designer.
namespace Terrasoft.Configuration.Omnichannel.Messaging {
using System;
using System.Collections.Generic;
using Terrasoft.Core;
using Terrasoft.Core.DB;
#region Class: ForEveryoneOperatorRoutingRule
/// <summary>
/// Retrieve chat agents.
/// </summary>
public class TestOperatorRoutingRule: BaseOperatorRoutingRule {
#region Constructors: Public
/// <summary>
/// Initialize a new instance of <see cref="TestOperatorRoutingRule"/>.
/// </summary>
/// <param name="userConnection"><see cref="UserConnection"/> the instance.</param>
public TestOperatorRoutingRule(UserConnection userConnection) : base(userConnection) {}
#endregion
#region Methods: Private
#endregion
#region Methods: Protected
/// <inheritdoc cref="BaseOperatorRoutingRule.PickUpFreeQueueOperators(Guid, Guid)"/>
protected override List < Guid > PickUpFreeQueueOperators(Guid chatId, Guid queueId) {
return new List < Guid > {
Guid.Parse("7F3B869F-34F3-4F20-AB4D-7480A5FDF647")
};
}
/// <inheritdoc cref="BaseOperatorRoutingRule.GetChatOperator(Guid)"/>
protected override Guid GetChatOperator(Guid chatId) {
Guid operatorId = (new Select(UserConnection).Column("OperatorId").From("OmniChat", "oc").Where("oc", "Id").IsEqual(Column.Parameter(chatId))
as Select).ExecuteScalar < Guid > ();
return operatorId;
}
#endregion
}
#endregion
}
3. Link the interface and lookup rule code
To link the interface implementation and alphabetic code of the rule you specified in the lookup on step 1, create a TestAppEventListener
class (inherit the class from the AppEventListenerBase
class) and link the interfaces in it.
To create a class:
-
Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Source code on the section list toolbar.
-
Go to the Schema Designer and fill out the schema properties:
- Set Code to "UsrTestAppEventListener."
- Set Title to "TestAppEventListener."
-
Add the source code in the Schema Designer.
namespace Terrasoft.Configuration.Omnichannel.Messaging {
using Common;
using Core;
using Terrasoft.Core.Factories;
using Web.Common;
#region Class: TestAppEventListener
/// <summary>
/// The class that runs prerequisites for OmnichannelMessaging on the application start.
/// </summary>
public class TestAppEventListener: AppEventListenerBase {
#region Fields: Protected
protected UserConnection UserConnection {
get;
private set;
}
#endregion
#region Methods: Protected
/// <summary>
/// Retrieve the user connection from the application event scope.
/// </summary>
/// <param name="context">The application event scope.</param>
/// <returns>User connection.</returns>
protected UserConnection GetUserConnection(AppEventContext context) {
var appConnection = context.Application["AppConnection"] as AppConnection;
if (appConnection == null) {
throw new ArgumentNullOrEmptyException("AppConnection");
}
return appConnection.SystemUserConnection;
}
protected void BindInterfaces() {
ClassFactory.Bind < IOperatorRoutingRule,
TestOperatorRoutingRule > ("TestRule");
}
#endregion
#region Methods: Public
/// <summary>
/// Handle the application start.
/// </summary>
/// <param name="context">The application event scope.</param>
public override void OnAppStart(AppEventContext context) {
base.OnAppStart(context);
UserConnection = GetUserConnection(context);
BindInterfaces();
}
#endregion
}
#endregion
}
4. Restart Creatio in IIS
Restart Creatio in IIS to apply the changes. After you restart Creatio and select "Test rule" in the queue settings, Creatio will distribute chats based on the specified conditions.
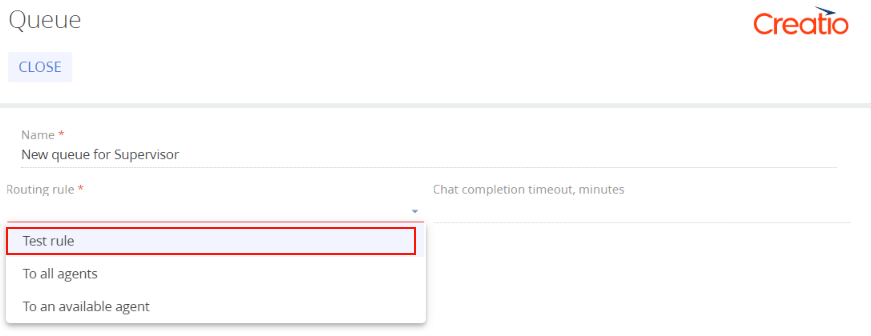