Run the business process from a client module
Add the Schedule a meeting custom action to the account page and Accounts section list. The action must run the Holding a meeting custom business process. The action must be active if the account has a primary contact, i. e., the Primary contact field of the account page is filled out. Pass the primary contact of the account to the business process as a parameter.
1. Implement the Holding a meeting custom business process
-
Create the Holding a meeting business process. To do this, implement the example from a separate article: Send emails via business processes.
-
Add the business process parameter.
Fill out the values of the business process parameter.
- Set Title to "Meeting contact."
- Set Code to "ProcessSchemaContactParameter."
- Set Data type to "Unique identifier."
-
Add the "[#Meeting contact#]" incoming parameter to the Contact property of the Call customer process action.
2. Implement the custom action on the account page
-
Create a replacing view model schema of the account page To do this, follow the guide in a separate article: Add an action to the record page.
-
Add a localizable string that contains the menu item caption.
-
Click the
button in the context menu of the Localizable strings node.
-
Fill out the localizable string properties.
- Set Code to "CallProcessCaption."
- Set Value to "Schedule a meeting."
-
Click Add to add a localizable string.
-
-
Implement the menu item behavior.
-
Add the
ProcessModuleUtilities
module as a dependency. -
Implement the following methods in the
methods
property:callCustomProcess()
. Action handler method. Implements theexecuteProcess()
method of theProcessModuleUtilities
module. Pass the name of the business process and object that contains the initialized incoming process parameters to the module as a parameter.isAccountPrimaryContactSet()
. Checks whether the primary contact of the account is filled out.getActions()
. An overloaded base method. Returns the action collection of the replacing page.
View the source code of the replacing view model schema of the account page below.
AccountPageV2define("AccountPageV2", ["ProcessModuleUtilities"], function(ProcessModuleUtilities) {
return {
/* The name of the record page object’s schema. */
entitySchemaName: "Account",
/* The methods of the record page’s view model. */
methods: {
/* Verifies the [Primary contact] field of the record page is filled in. */
isAccountPrimaryContactSet: function() {
return this.get("PrimaryContact") ? true : false;
},
/* Overrides the base virtual method that returns the action collection of the record page. */
getActions: function() {
/* Calls the parent method implementation to retrieve the collection of the base page’s initialized actions. */
var actionMenuItems = this.callParent(arguments);
/* Adds a separator line. */
actionMenuItems.addItem(this.getActionsMenuItem({
Type: "Terrasoft.MenuSeparator",
Caption: ""
}));
/* Adds a menu item to the action list of the record page. */
actionMenuItems.addItem(this.getActionsMenuItem({
/* Binds the menu item caption to the localizable schema string. */
"Caption": {
bindTo: "Resources.Strings.CallProcessCaption"
},
/* Binds the action handler method. */
"Tag": "callCustomProcess",
/* Binds the property of the menu item visibility to the value returned by the isAccountPrimaryContactSet() method. */
"Visible": {
bindTo: "isAccountPrimaryContactSet"
}
}));
return actionMenuItems;
},
/* The action handler method. */
callCustomProcess: function() {
/* Receives the ID of the account primary contact. */
var contactParameter = this.get("PrimaryContact");
/* The object that transferred to the executeProcess() method as a parameter. */
var args = {
/* The name of the process that needs to be run. */
sysProcessName: "UsrCustomProcess",
/* The object with the ContactParameter incoming parameter value for the CustomProcess process. */
parameters: {
ProcessSchemaContactParameter: contactParameter.value
}
};
/* Runs the custom business process. */
ProcessModuleUtilities.executeProcess(args);
}
}
};
}); -
-
Click Save on the Module Designer’s toolbar.
3. Implement the custom action of the section page
-
Create a replacing view model schema of the section. To do this, follow the guide in a separate article: Add an action to the record page.
-
Add a localizable string that contains the menu item caption. To do this, take step 2 of the procedure to implement a custom action of the account page.
-
Implement the menu item behavior. To do this, implement the
isAccountPrimaryContactSet()
method in themethods
property. The method checks whether the primary contact of the account is filled out.View the source code of the replacing view model schema of the section page below.
AccountSectionV2define("AccountSectionV2", [], function() {
return {
/* The name of the section page schema. */
entitySchemaName: "Account",
/* The methods of the section page’s view model. */
methods: {
/* Verifies the [Primary contact] field of the selected account is filled in. */
isAccountPrimaryContactSet: function() {
/* Defines the active record. */
var activeRowId = this.get("ActiveRow");
if (!activeRowId) {
return false;
}
/* Retrieves the data collection of the section list’s list view. Retrieves the selected account model by the specified primary column value. */
var selectedAccount = this.get("GridData").get(activeRowId);
if (selectedAccount) {
/* Receives the model property – the primary contact. */
var selectedPrimaryContact = selectedAccount.get("PrimaryContact");
/* Returns true if the primary contact is filled in, return false otherwise. */
return selectedPrimaryContact ? true : false;
}
return false;
}
}
};
}); -
Click Save on the Module Designer’s toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Accounts section page.
As a result, Creatio will add the Schedule a meeting action to the account page. This action will be active if the primary contact of the account is filled out.
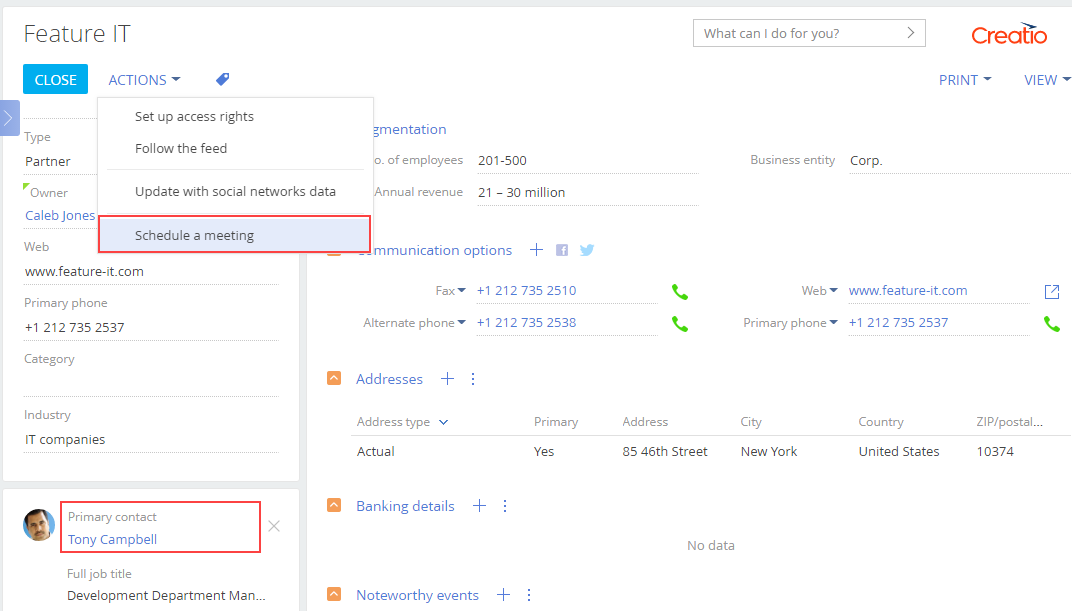
Select the action to run the Holding a meeting custom business process . The primary contact of the account will be passed to the business process as a parameter.
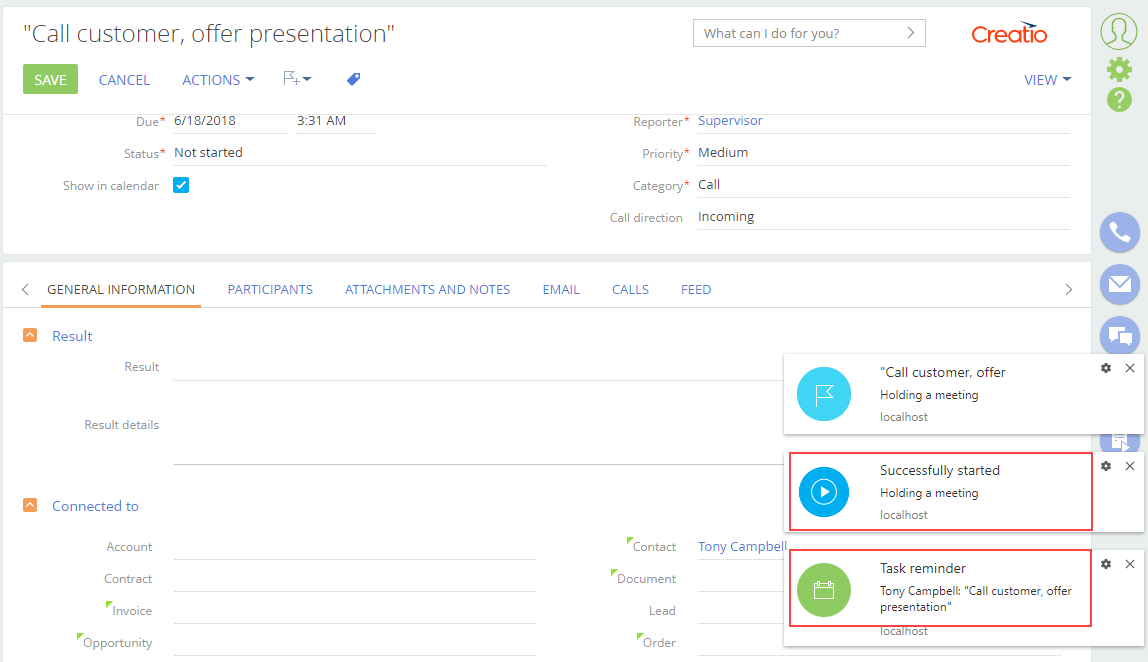