Populate a field using an install script
The example is relevant to Sales Creatio products.
Populate the Delivery type field on the order page using an install script.
- Set the field to "Courier" for products that have the "Software" category.
- Set the field to "Customer pickup" for products that have the "Hardware" and "Services" category.
- Leave the field empty for products that have other categories.
Populate the Delivery type field for orders that contain a single product.
1. Implement an install script
-
Open the Configuration section and select a custom package to add the schema.
-
Click Add → Source code on the section list toolbar.
-
Fill out the schema properties in the Source Code Designer:
- Set Code to "UsrPopulateDeliveryType."
- Set Title to "Populate the delivery type."
Click Apply.
-
Implement the business logic of the delivery type filling.
UsrFillDeliveryTypenamespace Terrasoft.Configuration {
using System;
using System.Collections.Generic;
using Terrasoft.Core;
using Terrasoft.Core.Entities;
public class UsrPopulateDeliveryType: IInstallScriptExecutor {
private static object GetDeliveryIdByName(string deliveryName) {
/* The unique ID of the delivery type. Delivery types are stored in the [DeliveryType] configuration element of the [Data] type in the [Order] package. */
switch (deliveryName) {
case "Courier":
return new Guid("50df77d0-7b1f-4dbc-a02d-7b6ebb95dfd0");
case "Customer pickup":
return new Guid("ab31305f-7c6d-4158-bd0a-760ac7897755");
default:
return Guid.Empty;
}
}
private static object GetDeliveryTypeByProductCategory(string category) {
/* The category of the product. Product categories are stored in the [ProductCategory] configuration element of the [Data] type in the [ProductCatalogue] package. */
switch (category) {
case "Software":
return GetDeliveryIdByName("Courier");
case "Hardware":
return GetDeliveryIdByName("Customer pickup");
case "Services":
return GetDeliveryIdByName("Customer pickup");
default:
return GetDeliveryIdByName("");
}
}
public void Execute(UserConnection userConnection) {
/* Create an ESQ that contains the [Id] column of the product and [Name] column from the [OrderProduct] database table. The [Name] column is the name of the product category. */
EntitySchemaQuery esqResult = new EntitySchemaQuery(userConnection.EntitySchemaManager, "OrderProduct");
EntitySchemaQueryColumn orderIdColumn = esqResult.AddColumn("Order.Id");
EntitySchemaQueryColumn productCategoryColumn = esqResult.AddColumn("Product.Category.Name");
/* Retrieve the collection of products in the order. */
EntityCollection productsInOrder = esqResult.GetEntityCollection(userConnection);
EntitySchemaManager entitySchemaManager = userConnection.EntitySchemaManager;
Entity orderEntity = entitySchemaManager.GetEntityByName("Order", userConnection);
foreach(Entity entity in productsInOrder) {
/* Retrieve the order object using the ID. */
Guid id = entity.GetTypedColumnValue <Guid> (orderIdColumn.Name);
string category = entity.GetTypedColumnValue <string> (productCategoryColumn.Name);
var conditions = new Dictionary <string,
object> {
{
"Id",
id
}
};
orderEntity.FetchFromDB(conditions);
/* Populate the delivery type based on the product category. */
orderEntity.SetColumnValue("DeliveryTypeId", GetDeliveryTypeByProductCategory(category));
/* Save the order. */
orderEntity.Save();
}
}
}
} -
Click Publish on the Source Code Designer’s toolbar to apply the changes to the database level.
2. Add the install script to the installation steps
-
Open the Configuration section and select a custom package.
-
Select Properties in the package menu.
-
Go to the Installation steps tab to set up the execution order of steps when installing the package.
-
Add the
UsrFillDeliveryType
install script to the After package installation start event.- Click + Add in the After package installation block. This opens a window.
- Select the
UsrPopulateDeliveryType
schema. - Click Select.
-
Click Apply.
Outcome of the example
To view the outcome of the example for the current Creatio instance:
- Click Actions → Run as install script on the Source Code Designer’s toolbar to execute the Source code schema as install script.
- Open the
Sales Enterprise
app page and click Run app. - Open the
Orders
section. - Open the order page → Products tab to check the category of the product.
- Go to the Delivery tab.
As a result, Creatio will set the "Courier" delivery type for products that have the "Software" category.
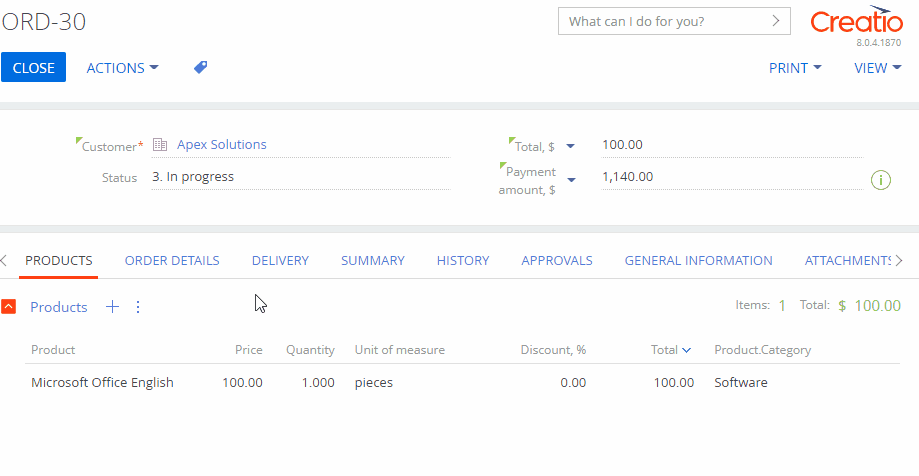
Creatio will set the "Customer pickup" delivery type for products that have "Hardware" category.
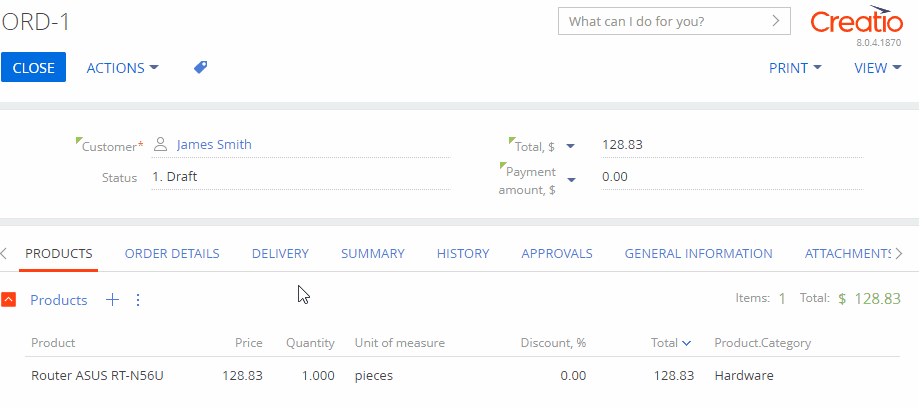
Creatio will set the "Customer pickup" delivery type for products that have "Services" category.
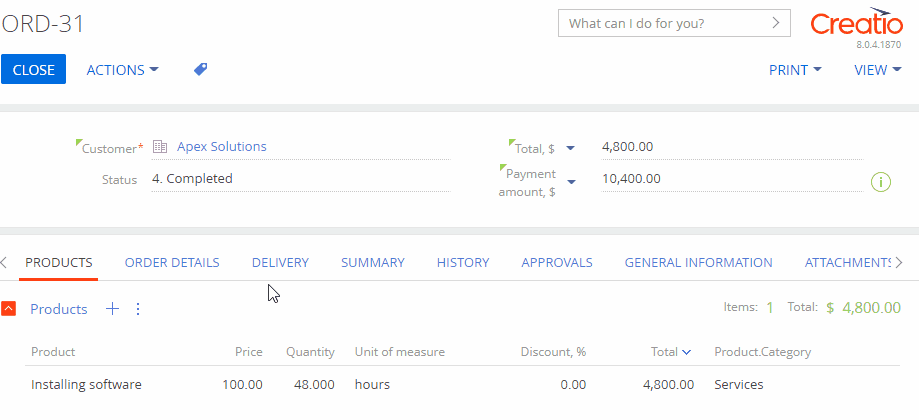
Creatio will leave the Delivery type field empty for products that have other categories.
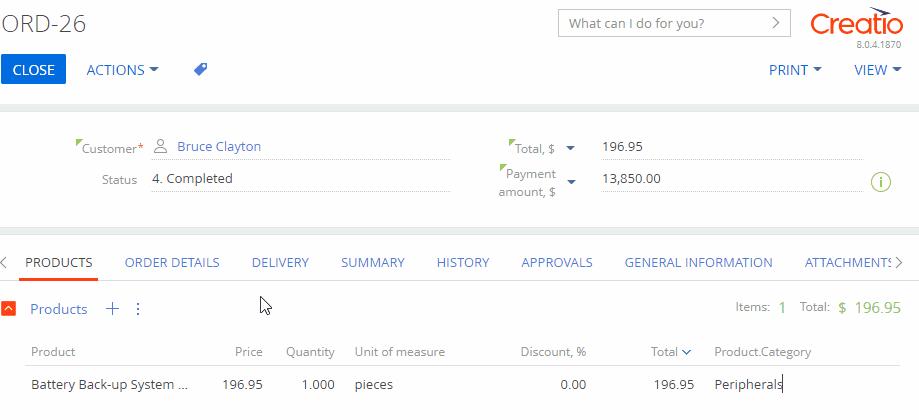
To view the outcome of the example for other Creatio instances:
- Select Export in the package menu.
- Install the package to the needed Creatio instance. To do this, follow the guide in a separate article: Install apps from the Marketplace.
- Repeat steps 2-5 of the procedure to view the outcome of the example for the current Creatio instance.