Implement a custom validator using remote module
The example is relevant to Creatio version 8.0.8 and later.
Add a validator that ensures the Name field of the custom Requests section starts from the REQ-
prefix.
Implement the validator using a remote module created in Angular framework.
1. Create an Angular project to develop a custom validator using remote module
To create an Angular project to develop a custom validator using remote module, follow the instruction in a separate article: Implement custom UI component using remote module.
2. Create a custom validator using remote module
-
Create an Angular class in the project. To do this, run the
ng g class verify-request-name.validator
command at the command line terminal of Microsoft Visual Studio Code.As a result, Microsoft Visual Studio Code will add the
VerifyRequestNameValidator
class files to thesrc/app/
project directory. -
Implement the validator.
- Open the
verify-request-name.validator.ts
file. - Flag the
VerifyRequestNameValidator
class using the@CrtValidator
decorator. - Inherit the
BaseValidator
class from the@creatio-devkit/common
library. - Implement the field
async
. - Implement the abstract method
validate()
that validates data in the field. - Import the required functionality from the libraries into the class.
- Save the file.
verify-request-name.validator.ts file/* Import the required functionality from the libraries. */
import { BaseValidator, CrtControlState, CrtInject, CrtValidationErrors, CrtValidator, ValidatorParametersValues } from '@creatio-devkit/common';
/* Add the CrtValidator decorator to the VerifyRequestNameValidator interface. */
@CrtValidator({
type: 'usr.VerifyRequestNameValidator',
})
export class VerifyRequestNameValidator extends BaseValidator {
constructor(private _prefix: string) {
super();
}
protected override async = false;
public validate(
control: CrtControlState,
params?: ValidatorParametersValues
): CrtValidationErrors | null {
const value = control.value as string;
const maxLength = (params as any).maxLength;
if (value && (!value.startsWith(this._prefix) || value.length > maxLength)) {
return {
error: {
message: 'Add "REQ-" prefix'
},
};
}
return null;
}
} - Open the
-
Inject dependencies.
- Open the
verify-request-name.validator.ts
file. - Create the
REQUEST_NAME_PREFIX
instance of theInjectionToken
class. Use the token to work with Angular DI (dependency injection). - Mark DI in the constructor of the
VerifyRequestNameValidator
class using the@CrtInject
decorator. - Import the required functionality from the libraries into the class.
- Save the file.
verify-request-name.validator.ts file/* Import the required functionality from the libraries. */
...
import { InjectionToken } from "@angular/core";
/* The REQUEST_NAME_PREFIX token that works with Angular DI (dependency injection). */
export const REQUEST_NAME_PREFIX = new InjectionToken<string>('REQUEST_NAME_PREFIX');
...
export class VerifyRequestNameValidator extends BaseValidator {
/* Mark DI in the constructor using the @CrtInject decorator. */
constructor(@CrtInject(REQUEST_NAME_PREFIX) private _prefix: string) {
super();
}
...
} - Open the
-
Register the
VerifyRequestNameValidator
validator as a validator and theREQUEST_NAME_PREFIX
token.-
Open the
app.module.ts
file. -
Add the
VerifyRequestNameValidator
validator to thevalidators
section in theCrtModule
decorator. -
Add the configuration object to the
providers
section in theNgModule
decorator.- Set the
provide
property toREQUEST_NAME_PREFIX
. - Set the
useValue
property to theREQ
.
- Set the
-
Implement the
resolveDependency()
method in thebootstrapCrtModule()
method of theAppModule
root module. ThebootstrapCrtModule()
method registers theVerifyRequestNameValidator
validator flagged using theCrtModule
decorator. TheresolveDependency()
method receives the dependencies of theVerifyRequestNameValidator
converter. -
Import the required functionality from the libraries into the class.
-
Save the file.
app.module.ts file/* Import the required functionality from the libraries. */
import { DoBootstrap, Injector, NgModule, ProviderToken } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { bootstrapCrtModule, CrtModule } from '@creatio-devkit/common';
import { VerifyRequestNameValidator, REQUEST_NAME_PREFIX } from "./verify-request-name.validator";
@CrtModule({
/* Specify that VerifyRequestNameValidator is a validator. */
validators: [VerifyRequestNameValidator],
})
@NgModule({
...
providers: [{
provide: REQUEST_NAME_PREFIX,
useValue: 'REQ-'
}],
})
export class AppModule implements DoBootstrap {
constructor(private _injector: Injector) { }
ngDoBootstrap(): void {
/* Bootstrap CrtModule definitions. */
bootstrapCrtModule('sdk_remote_module_package', AppModule, {
resolveDependency: (token) => this._injector.get(<ProviderToken<unknown>>token)
});
}
} -
-
Build the project. To do this, run the
npm run build
command at the command line terminal of Microsoft Visual Studio Code.
As a result, Microsoft Visual Studio Code will add the build to the dist
directory of the Angular project. The build will have the sdk_remote_module_package
name.
3. Add the validator implemented using remote module to the Freedom UI page
-
Set up the Freedom UI page.
- Repeat steps 1-7 of the procedure to implement custom UI component using remote module.
- Click the
button in the action panel of the Freedom UI Designer. After you save the page settings, Creatio opens the source code of the Freedom UI page.
-
Bind the
usr.VerifyRequestNameValidator
validator to the model attribute in theviewModelConfig
schema section.viewModelConfig schema sectionviewModelConfig: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
"UsrName": {
"modelConfig": {
"path": "PDS.UsrName"
},
"validators": {
"usr.VerifyRequestNameValidator": {
"type": "usr.VerifyRequestNameValidator",
"params": {
"maxLength": 10
}
}
}
},
...
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/, -
Click Save on the Client Module Designer’s toolbar.
As a result, Creatio will add the validator to the Freedom UI page.
Outcome of the example
To view the outcome of the example:
- Open the
Requests
app page and click Run app. - Click New on the
Requests
app toolbar. - Enter "123" in the Name input.
As a result, Creatio will display an error notification in a pop-up box. The validator is implemented using a remote module created in Angular framework.
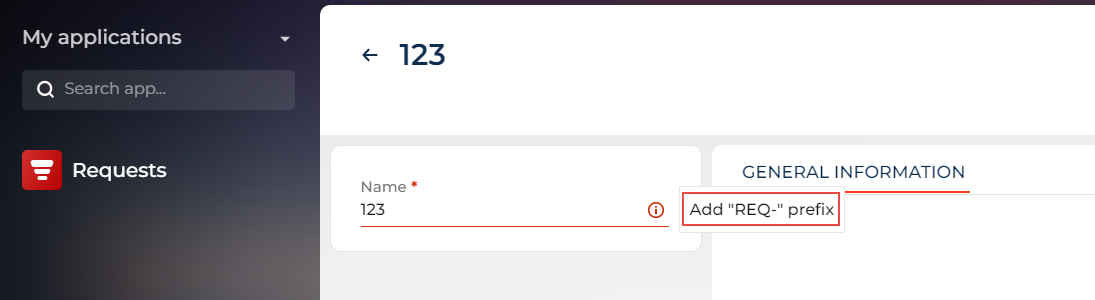
Source code
- verify-request-name.validator.ts file
- app.module.ts file
import {
BaseValidator, CrtControlState, CrtInject,
CrtValidationErrors,
CrtValidator,
ValidatorParametersValues
} from '@creatio-devkit/common';
import { InjectionToken } from "@angular/core";
export const REQUEST_NAME_PREFIX =
new InjectionToken<string>('REQUEST_NAME_PREFIX');
@CrtValidator({
type: 'usr.VerifyRequestNameValidator',
})
export class VerifyRequestNameValidator extends BaseValidator {
constructor(@CrtInject(REQUEST_NAME_PREFIX) private _prefix: string) {
super();
}
protected override async = false;
public validate(
control: CrtControlState,
params?: ValidatorParametersValues
): CrtValidationErrors | null {
const value = control.value as string;
const maxLength = (params as any).maxLength;
if (value && (!value.startsWith(this._prefix) || value.length > maxLength)) {
return {
error: {
message: 'Add "REQ-" prefix'
},
};
}
return null;
}
}
import { DoBootstrap, Injector, NgModule, ProviderToken } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { bootstrapCrtModule, CrtModule } from '@creatio-devkit/common';
import { VerifyRequestNameValidator, REQUEST_NAME_PREFIX } from "./verify-request-name.validator";
@CrtModule({
validators: [VerifyRequestNameValidator],
})
@NgModule({
declarations: [],
imports: [BrowserModule],
providers: [{
provide: REQUEST_NAME_PREFIX,
useValue: 'REQ-'
}],
})
export class AppModule implements DoBootstrap {
constructor(private _injector: Injector) { }
ngDoBootstrap(): void {
/* Bootstrap CrtModule definitions. */
bootstrapCrtModule('sdk_remote_module_package', AppModule, {
resolveDependency: (token) => this._injector.get(<ProviderToken<unknown>>token)
});
}
}