The back-end core components provide the following database access options:
- access using the ORM model
- direct access
This article covers database queries that use the ORM model.
ORM (Object-relational mapping) is a technology that lets you use object-oriented programming languages to manage data retrieved from the database. The purpose of ORM is to bind objects implemented in the code to the database table records.
The ORM data model implements data management via the following classes:
- The Terrasoft.Core.Entities.EntitySchemaQuery class builds queries to retrieve the database table records. The queries apply the access permissions of the current user.
- The Terrasoft.Core.Entities.Entity class accesses entities that represent records in the database table.
We recommend using the ORM model to access data, though the direct access to the database is also implemented in the back-end core components. Learn more about running direct database queries: Access data directly.
Configure a column path relative to the root schema
Base a selection query that uses EntitySchemaQuery on the root schema. The root schema is the database table relative to which to build paths to the query columns, including the columns of joinable tables. To use a table column in a query, set the path to the column correctly.
Follow the principle of connection by lookup fields when building the column paths. Build the name of a column to add to the query as a chain of interconnected links. Each link must represent the context of the schema connected to the previous schema by a lookup column.
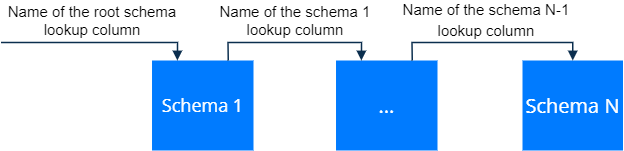
The template for configuring the path to a column in the N schema: 1SchemaContext.
Specify the column path using the direct connections
The template for configuring the column path using the direct connections: LookupColumnName.
Use direct connections if the connection lookup column is located in the main schema and links to the joinable schema. For example, a [City] root schema contains the [Country] lookup column. The column is connected to the [Country] lookup schema by the [Id] column.

The path to the column that contains the name of the country connected to the city, built using the direct connections: Country.Name. Where:
- Country is the name of the lookup column in the [City] root schema. Links to the [Country] schema.
- Name is the name of the column in the [Country] lookup schema.
Specify the column path using the reverse connections
Unlike direct connections, reverse connections require the lookup field to join to be located in the joinable entity, not the main entity.
The template for configuring the column path using the reverse connections: [JoinableSchemaName:
The path to the name column of the account whose [City] field contains the [City] record selected in the query, built using the reverse connections: [Account:City:Id].Name. Where:
- Account is the name of the joinable schema.
- City is the name of the [Account] schema's column to connect the joinable schema.
- Id is the name of the [City] schema's lookup column to connect the current schema.
- Name is the value of the [Account] schema's lookup column.
If the schema's lookup column to connect the current schema is [Id], you do not have to specify it: [JoinableSchemaName:
Retrieve data from the database
Use the following classes to retrieve data from the database:
- Terrasoft.Core.DB.Select retrieves data by accessing the database directly. Learn more: Access data directly.
- Terrasoft.Core.Entities.EntitySchemaQuery retrieves database data using the ORM model.
The purpose of the Terrasoft.Core.Entities.EntitySchemaQuery class is to build selection queries for database table records. By default, you can retrieve up to 20 000 records in a query. This value can be modified in the MaxEntityRowCount setting in the ...\Terrasoft.WebApp\Web.config file.
After you create and configure the class instance, Creatio will build a SELECT query to the application database. You can add columns, filters, and restriction conditions to the query. You can also set the parameters to output the query results in pagination mode. If needed, use the Terrasoft.Core.Entities.EntitySchemaQueryOptions class to set the parameters of a hierarchical query. Pass the same EntitySchemaQueryOptions instance as the GetEntityCollection() method parameter of the corresponding query to retrieve the results of various queries.
The Terrasoft.Core.Entities.EntitySchemaQuery class major features:
- The resulting query applies the access permissions of the current user.
- You can manage the current user's access permissions to the tables that JOIN SQL operator joined to the query.
- The class lets you manage data of a cache repository or any repository you specify.
Creatio will cache the data retrieved from the database after running the query. Any repository that implements the Terrasoft.Core.Store.ICacheStore interface can serve as the query cache. By default, Creatio uses the session level cache with local storage. Define the query cache in the Cache property of the EntitySchemaQuery class instance. Set the key to access the query cache in the CacheItemName property. Learn more about Creatio repository levels: The object model of Creatio repositories.
As result of the query, is the Terrasoft.Nui.ServiceModel.DataContract.EntityCollection instance or the Terrasoft.Core.Entities.Entity class instance collection. Each Entity collection instance represents a string of data returned by the query.
Manage joined tables
You can specify the schema join type of a query using the EntitySchemaQuery class. Use the JOIN operator to add the joinable schema column to the query.
The template for configuring the join type of the joinable schema column: SpecialCharacterOfTheJoinType
|
|
|
---|---|---|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
By default, Creatio uses the LEFT OUTER JOIN type.
See the example that adds columns to a query using various join types below.
As a result, Creatio will generate the SQL query.
If the query contains the root schema and joinable schemas that use record permissions, you will be able to apply the access permissions of the current user. The Terrasoft.Core.DB.QueryJoinRightLevel enumeration lists the ways to apply the record access permissions to the joinable schemas.
The ways to apply the access permissions to the joinable schema queries are as follows:
- Apply always.
- Apply only if the joinable schema query uses columns other than the primary column and primary display column. Usually, these are [Id] and [Name] columns.
- Do not apply.
The JoinRightState query property determines the order to apply the access permissions. The Joined objects administering (QueryJoinRightLevel code) system setting determines the default value of the property. If the setting is empty, Creatio will apply the access permissions if the joinable schema query uses columns other than the primary column and the primary display column.
Manage the query filters
Filter is sets of conditions applied when displaying the query data. In SQL terms, a filter is a separate predicate (condition) of the WHERE operator.
To create a simple filter in EntitySchemaQuery, use the CreateFilter() method that returns the instance of the EntitySchemaQueryFilter type. EntitySchemaQuery implements a series of overloads for this method. This lets you create filters with different incoming parameters. EntitySchemaQuery implements methods that create special kinds of filters.
The EntitySchemaQuery instance contains the Filters property that represents the filter collection of the query (the EntitySchemaQueryFilterCollection class instance). The EntitySchemaQueryFilterCollection class instance is a typed collection of the IEntitySchemaQueryFilterItem elements.
Follow this procedure to add a filter to the query:
- Create a filter instance for the query (CreateFilter() methods, methods that create special kinds of filters).
- Add the filter instance to the query filter collection (the Add() collection method).
By default, Creatio uses the logical AND operation to combine the filters added to the Filters collection. To implement the logical OR operation, use the LogicalOperation property of the Filters collection. This property takes the values of the LogicalOperationStrict enumeration and lets you specify the logical operation to combine the filters.
You can manage the filters that build the resulting dataset in the EntitySchemaQuery queries. Each element of the Filters collection includes the IsEnabled property that determines whether the element takes part in building the resulting query (true/false). Creatio defines the similar IsEnabled property for the Filters collection. If you set this property to false, the query filtering will be disabled, yet the query filters collection will remain unchanged. Thus, you can create a query filters collection and use various combinations without changing the collection itself.
Manage the database entity
Terrasoft.Core.Entities.Entity is a class that implements the database entity management.
The purpose of the Terrasoft.Core.Entities.Entity class is to access the object that represents a database table record. You can also use the class for CRUD operations on the corresponding records.
EntitySchemaQuery class
Terrasoft.Core.Entities namespace.
The Terrasoft.Core.Entities.EntitySchemaQuery class is used to build queries for selecting records in Creatio database tables. As a result of creating and configuring the instance of this class, the SELECT SQL-expression query to the application database will be built. You can add the needed columns, filters, and restriction conditions to the query.
Constructors
Creates a class instance, where the EntitySchema passed instance is set as a root schema. The manager of the passed-in instance of the root schema is set as the schema manager.
Creates a class instance with the specified EntitySchemaManager and the root schema passed as an argument.
Creates a class instance that is a clone of the instance passed as an argument.
Properties
The query cache.
Name of the cache item.
Determines whether the query results will include the data for which the transaction is not completed.
Title.
The number of strings in one chunk.
Collection of columns of the current entity schema query.
Manager of the data type values.
Entity schema manager.
Collection of filters of the current entity schema query.
Determines whether the values of the encrypted columns will be hidden.
Determines whether the displayed column values will be used in the query.
Indicates whether duplicates in the resulting data set should be removed.
Indicates whether the query is inherited.
Determines the conditions for applying permissions when using related tables if the schema is managed by records.
Schema manager.
Manager element.
Name.
A collection of queries to which the current request to the object schema belongs.
Parent schema of the query.
The column created from the primary column of the root schema. Initialized during the first access.
Allows using query optimization.
The root schema.
Number of rows that are returned by the query.
The prefix used to create schema alias.
Number of rows to skip when returning the query result.
The parameter that defines whether permissions will be taken into account when constructing a data acquisition request.
Determines whether localizable data will be used.
Determines whether the per-page returning of the query result is available.
Determines whether data will be excluded from filtering.
Methods
The object schema adds all the columns of the root schema in the column collection of the current query.
Creates and inserts a column in the current entity schema query.
column |
Path to the schema column in relation to the root schema. |
aggregation |
The type of the aggregate function. The enumeration type values of the Terrasoft.Common.AggregationTypeStrict aggregate function are passed as a parameter. |
sub |
Reference to the created subquery placed in the column. |
query |
An EntitySchemaQueryColumn instance to be added to the column collection of the current query. |
function | An EntitySchemaQueryFunction function instance. |
parameter |
The value of the parameter added to the query as a column. |
parameter |
The type of parameter value added to the query as a column. |
Clears the cache of the current query.
Removes the item with the specified cacheItemName name from the cache of the query.
Creates a clone of the current EntitySchemaQuery instance.
Returns expression of the aggregated function with the specified aggregation type from the Terrasoft.Common.AggregationTypeStrict enumeration for the column, located at the leftExprColumnPath specified path.
Creates an expression for the query parameter.
parameter |
The value of the parameter. |
value |
The type of the parameter value. |
display |
Parameter displayed value. |
Creates an expression collection for the query parameters with a certain DataValueType type of data.
Returns the expression of the entity schema column.
parent |
The entity schema query for which the column expression is created. |
root |
The root schema. |
column |
The path to column in relation to the root schema. |
use |
Indicates whether to use the COALESCE function for the column of the lookup type. An optional parameter, set to true by default. |
use |
Indicates whether to use the displayed value for the column. An optional parameter, set to false by default. |
Returns a collection of column expressions of the entity schema query by the specified columnPaths collection of paths to columns.
Returns the collection of column expressions of the entity schema query by the specified array of paths to columns. If it is a column of the multilookup type, the COALESCE function is not used for its values.
Returns the expression of the entity schema query by the specified path to column, root schema and schema column instance. You can define which column value type to use in the expression – either the stored value or the displayed value.
Returns the expression of entity schema subquery for the column located at the specified leftExprColumnPath path.
Returns the EntitySchemaAggregationQueryFunction aggregation function instance with the specified type of aggregation from the Terrasoft.Common.AggregationTypeStrict enumeration for the column at the specified columnPath path in relation to the root schema.
Returns an instance of the CASE EntitySchemaCaseNotNullQueryFunction function for the specified EntitySchemaCaseNotNullQueryFunctionWhenItem array of condition expressions.
Returns an instance of an expression for the sql construct of the WHEN <Expression_1> IS NOT NULL THEN <Expression_2> form.
when |
Path to the column that contains the expression of the WHEN clause. |
then |
Path to the column that contains the expression of the THEN clause. |
Returns an instance of the CAST EntitySchemaCastQueryFunction function for the column expression located at the specified columnPath path relative to the root schema and the specified DBDataValueType target data type.
Returns the function instance returning the first expression that is other than null from the list of arguments for the specified columns.
column |
An array of paths to columns in relation to the root schema. |
parent |
A query to entity schema, for which the function instance is created. |
root |
The root schema. |
Returns a function instance for generating a string that is the result of combining the string values of function arguments for the specified EntitySchemaQueryExpression array of expressions.
Returns a DATEPART instance of the EntitySchemaDatePartQueryFunction function that determines the date interval specified by the EntitySchemaDatePartQueryFunctionInterval enumeration (month, day, hour, year, week day...) for the value of column located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that determines the Day date range for a column value located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that returns a part of the Hour date for a column value located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that returns a part of the Minute date for a column value located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that returns a part of the Month date for a column value located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that returns a part of the Week day date for a column value located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that returns a part of the Week date for a column value located at the specified path in relation to the root schema.
Returns an instance of the EntitySchemaDatePartQueryFunction function that returns a part of the Year date for a column value located at the specified path in relation to the root schema.
Returns the instance of the EntitySchemaIsNullQueryFunction function for columns with values to check and substitute located at specified paths in relation to the root schema.
Creating an instance of the LEN function (the function for returning the length of expression) for the column expression at the specified path in relation to the root schema or for the specified expression array.
Creating an instance of the TRIM function (the function for removing all leading and trailing whitespace from expression) for the column expression at the specified path in relation to the root schema or for the specified expression array.
Returns an instance of the EntitySchemaUpperQueryFunction function that converts all the argument expression characters into upper case. The column expression located at the specified path relative to the root schema is specified as the argument.
Returns an instance of the EntitySchemaCurrentDateQueryFunction function that defines the current date.
Returns an instance of the EntitySchemaCurrentDateTimeQueryFunction function that returns the current date and time.
Returns the instance of the EntitySchemaCurrentTimeQueryFunction function that defines the current time.
Returns the instance of the EntitySchemaCurrentUserAccountQueryFunction function that defines the account Id of the current user.
Returns the instance of the EntitySchemaCurrentUserContactQueryFunction function that defines the contact Id of the current user.
Returns the instance of the EntitySchemaCurrentUserQueryFunction function that defines the current user.
Creates the Exists by the specified condition type comparison filter and sets the column expression located at the rightExpressionColumnPath specified path as the testing value.
Creates a query filter for selecting records according to specified conditions.
comparison |
A comparison type from the Terrasoft.Core.Entities.FilterComparisonType enumeration. |
left |
A path to the column that contains the filter's left side expression. |
left |
An expression in the filter's left side. |
left |
The type of the aggregate function. |
left |
A parameter that returns a subquery for the expression on the left side of the filter (if it is not null) or a subquery for the first expression on the right side of the filter (if the left-hand side of the filter is null). |
right |
An array of paths that contain the filter's right side expressions. |
right |
The expression in the filter's right side. |
right |
An instance of the expression function on the right side of the filter (the EntitySchemaQueryFunction parameter type) or a subquery expression on the right side of the filter (the EntitySchemaQuery parameter type). |
right |
The value processed by the macro in the filter's right side. |
right |
The value of the parameter to which the filter's right side aggregate function is applied. |
macros |
A type of macro from the Terrasoft.Core.Entities.EntitySchemaQueryMacrosType enumeration. |
days |
The value to which the filter's right side macro is applied. An optional parameter, default value is 0. |
Creates a parameter filter for selecting records according to specified conditions.
parent |
The parent query for which the filter is being created. |
root |
The root schema. |
comparison |
A comparison type from the Terrasoft.Core.Entities.FilterComparisonType enumeration. |
use |
Whether the column value type used in the filter:true – for the displayed value; false – for the stored value. |
left |
A path to the column that contains the filter's left side expression. |
right |
The collection of parameter expressions on the right side of the filter. |
Creates the comparison filter of the Is not “null” in database type and, as the tested value, sets the column expression located at the path specified in the leftExpressionColumnPath parameter.
Creates the comparison filter of the Is “null” in database type and, as the testing condition, sets the column expression located at the path specified in the leftExpressionColumnPath parameter.
Creates the Does not exist by the specified condition type comparison filter and sets the column expression located at the rightExpressionColumnPath specified path as the tested value.
Returns the result of the current query execution to the object schema as a data table in memory using UserConnection.
Returns the sequence number of the week day for the System.DayOfWeek entity taking into account local settings.
Returns an Entity instance by the primaryColumnValue primary key using UserConnection.
Returns the Entity instance collection representing the results of executing the current query using UserConnection and the specified additional EntitySchemaQueryOptions query settings.
Returns the EntitySchema entity schema instance of the current EntitySchemaQuery instance.
Returns a data selection query instance using UserConnection and the specified additional settings of the EntitySchemaQueryOptions query.
Returns the collection of expressions of the query columns for which total values are calculated.
Returns an Entity instance for the result returned by the total value selection query.
user |
The user connection. |
summary |
The collection of query columns for which totals are selected. |
column |
A collection of the column names. |
Builds a query for the selection of total values for a specified column collection of the EntitySchemaQuery current instance.
user |
The user connection. |
summary |
The collection of query columns for which totals are selected. |
column |
A collection of the column names. |
Returns the typed column value with columnName from the Entity passed instance.
Loads the result of executing the current query to the entity schema into the System.Data.DataTable entity using UserConnection and the EntitySchemaQueryOptions specified additional query settings.
Removes the columnName column from the current query column collection.
Clears the schema of the EntitySchemaQuery current instance.
Clears the select query for the current entity schema query.
Sets the identifier of the local culture.
Terrasoft.Core.Entities namespace.
The Terrasoft.Core.Entities.Entity class provides access to an entity that represents a record in the database table.
Constructors
Creates a new Entity class instance for the set UserConnection user connection.
Creates a new Entity class instance for the set UserConnection user connection and the schema set by the schemaUId identifier.
Creates a class instance that is a clone of the instance passed as an argument.
Properties
The type of entity status change (added, modified, deleted, unchanged).
An instance of the entity schema manager.
The name of the entity schema manager.
Whether an entity has at least one column.
The value of the column that binds the parent record, for hierarchical entities.
The object instance identifier.
Whether the object is deleted from the database.
Whether to handle the ColumnValueChanged event.
Whether to handle the ColumnValueChanging event.
Whether to handle the DefColumnValuesSet event.
Whether to handle the Deleted event.
Whether to handle the Deleting event.
Whether to handle the Inserted event.
Whether to handle the Inserting event.
Whether to hanlde the Loaded event.
Whether to handle the Loading event.
Whether to handle the Saved event.
Whether to handle the SaveError event.
Whether to handle the Saving event.
Whether to handle the Updated event.
Whether to handle the Updating event.
Whether to handle the Validating event.
Whether the entity schema is initialized.
The prefix of the operation to license.
The object load state.
The ID of the initial column.
The value to display in the initial column.
The nested process of the entity.
An instance of the entity schema.
The entity schema name.
The entity status (modified, added, deleted, unchanged).
Whether to apply permissions when inserting, updating, deleting, and retrieving data.
Whether to use the default entity permissions.
Whether to use the lazy initial loading of entity data.
The user connection.
The message collection displayed when validating an entity.
An instance of the entity enumerations manager.
The name of the entity enumerations manager.
Methods
Sets the default permissions for the entity.
primary |
The ID of the access permission value. |
primary |
An array of IDs of the access permission values. |
Creates a clone of the current Entity instance.
Creates a query to add data to the database.
skip |
Whether to take the lookup columns into account while adding data. By default, false. |
Creates a query to update data in the database.
skip |
Whether to add the columns of the lookup type to the database. If set to true, Creatio will not add the columns of the lookup type to the database. By default, false. |
Deletes the entity record from the database.
key |
The key field value. |
Deletes the entity record from the database and cancels the started process.
Creates an Entity type entity using the userConnection user connection and populates the entity field values from the specified jsonValue JSON string.
json |
A JSON string. |
user |
The user connection. |
Whether the database contains a record that matches the given condition of the conditionValue query to the conditionColumn entity schema column or with the specified keyValue initial key.
condition |
The column for which to specify the selection condition. |
condition |
The name of the column for which to specify the selection condition. |
condition |
The value of the condition column for the selected data. |
conditions | A set of conditions to filter the object record selection. |
key |
The key field value. |
Loads the object from the database by the specified condition.
condition |
The column for which to specify the selection condition. |
condition |
The name of the column for which to specify the selection condition. |
condition |
The value of the condition column for the selected data. |
columns |
The list of columns to select. |
column |
The list of column names to select. |
conditions | A set of conditions to filter the object record selection. |
key |
The key field value. |
use |
Indicates that the query returns the initial displayed values. If set to true, the query will return the initial displayed values. |
Loads the object with the initial column from the database by the set keyValue condition.
key |
The key field value. |
Loads a database object with initial columns, including the initial displayed column, by the set condition.
condition |
The column for which to specify the selection condition. |
condition |
The name of the column for which to specify the selection condition. |
condition |
The value of the condition column for the selected data. |
Returns the value of the specified entity column as a byte array.
value |
The name of the entity column. |
Returns the name collection of the object properties that were modified.
Returns the value for the display of the object property that matches the specified entity schema column.
column | A specific entity schema column. |
Returns the previous value of the specified entity property.
column | A specific entity schema column. |
value |
The name of the entity column. |
Returns the value of the entity column with the specified name that matches the passed entity schema column.
column | A specific entity schema column. |
value |
The name of the entity column. |
Returns the collection of entity column names.
Returns the flag that determines whether the specified entity property is loaded.
column | A specific entity schema column. |
value |
The name of the entity column. |
Returns the value of the passed entity schema column converted into the System.IO.MemoryStream type instance.
value |
The name of the entity column. |
Returns the typed value of the entity property that matches the specified column of the entity schema.
column | A specific entity schema column. |
Returns the typed previous value of the entity property that matches the specified column of the entity schema.
column | A specific entity schema column. |
value |
The name of the entity column. |
Adds a record of the current entity to the database.
skip |
Whether to add the columns of the lookup type to the database. If set to true, Creatio will not add the columns of the lookup type to the database. By default, false. |
validate |
Whether to validate the required values. By default, true. |
Whether the value of the entity property with the specified name is loaded.
column | A specific entity schema column. |
value |
The name of the entity column. |
Populates the entity with the passed data.
column |
The entity properties to populate with data. |
data |
A System.Data.DataRow instance from which to load the data to the entity. |
data |
A System.Data.IDataReader instance from which to load the data to the entity. |
data |
A System.Object instance from which to load the data to the entity. |
Loads the value from the passed instance for the property with the specified name.
binary |
The size of the loaded value. |
column | The entity schema column. |
column |
The entity property name. |
data |
A System.Data.IDataReader instance from which to load the property value. |
field |
The index of the field loaded from System.Data.IDataReader. |
value | The loaded value of the property. |
Returns the current property value of the Entity type from the input stream.
data |
A System.Data.IDataReader instance from which to load the property value. |
user |
The user connection. |
Reads data from the entity schema and saves the data to the specified object of the System.Data.IDataReader type.
reader | A System.Data.IDataReader instance to which to load the entity schema data. |
schema | The entity schema. |
Cancels the changes for all entity properties.
Reverts all entity properties to previous values.
Saves the entity to the database.
validate |
Whether to validate the required values. By default, true. |
Converts the entity object into a JSON string.
entity | The Entity instance. |
Sets the specified entity property to the passed value of the System.Byte type.
stream |
A value of the System.Byte type set for the specified entity column. |
value |
The name of the entity column. |
Sets the entity property that matches the specified schema column to passed value and displayValue.
column | The entity schema column. |
display |
The loaded value for display. |
display |
The name of the column that contains the value for display. |
value | The loaded value of the column. |
Sets the specified schema column to the passed value.
column | The entity schema column. |
value | The loaded value of the column. |
value |
The name of the entity column. |
Sets the property with the specified name to the default value.
column |
The name of the entity column. |
def |
The default value. |
Sets default values for all entity properties.
Sets the specified entity property to the passed value of the System.IO.Stream type.
value | The loaded value of the column. |
value |
The name of the entity column. |
Updates the entity record in the database.
validate |
Whether to validate the required values. By default, true. |
Verifies if the required fields are populated.
Writes the value of the Entity type to the output stream with the specified name.
could |
Allow converting for XML serialization. |
data |
An instance of the Terrasoft.Common.DataWriter class that provides methods for writing values to the output stream sequentially. |
entity | A value for a record of the Entity type. |
property |
The entity name. |
Writes the data to the output stream with the specified name.
data |
An instance of the Terrasoft.Common.DataWriter class that provides methods for writing values to the output stream sequentially. |
property |
The property name. |
Writes to the output stream for the specified or current entity schema.
schema | The entity schema. |
writer | An instance of the Terrasoft.Common.DataWriter class that provides methods for writing values to the output stream sequentially. |
Events
Event handler used after modifying the entity column value.
The event handler receives an argument of the EntityColumnAfterEventArgs type.
The EntityColumnAfterEventArgs properties that provide information relevant to the event:
- ColumnValueName
- DisplayColumnValueName
Event handler used before modifying the entity column value.
The event handler receives an argument of the EntityColumnBeforeEventArgs type.
The EntityColumnBeforeEventArgs properties that provide information relevant to the event:
- ColumnStreamValue
- ColumnValue
- ColumnValueName
- DisplayColumnValue
- DisplayColumnValueName
Event handler used after setting default entity field values.
Event handler used after deleting an entity.
The event handler receives an argument of the EntityAfterEventArgs type.
The EntityAfterEventArgs properties that provide information relevant to the event:
- ModifiedColumnValues
- PrimaryColumnValue
Event handler used before deleting an entity.
The event handler receives an argument of the EntityBeforeEventArgs type.
The EntityBeforeEventArgs properties that provide information relevant to the event:
- AdditionalCondition
- IsCanceled
- KeyValue
Event handler used after inserting an entity.
The event handler receives an argument of the EntityAfterEventArgs type.
The EntityAfterEventArgs properties that provide information relevant to the event:
- ModifiedColumnValues
- PrimaryColumnValue
Event handler used before inserting an entity.
The event handler receives an argument of the EntityBeforeEventArgs type.
The EntityBeforeEventArgs properties that provide information relevant to the event:
- AdditionalCondition
- IsCanceled
- KeyValue
Event handler used after loading an entity.
The event handler receives an argument of the EntityAfterLoadEventArgs type.
The EntityAfterLoadEventArgs properties that provide information relevant to the event:
- ColumnMap
- DataSource
Event handler used before loading an entity.
The event handler receives an argument of the EntityBeforeLoadEventArgs type.
The EntityBeforeLoadEventArgs properties that provide information relevant to the event:
- ColumnMap
- DataSource
- IsCanceled
Event handler used after saving an entity.
The event handler receives an argument of the EntityAfterEventArgs type.
The EntityAfterEventArgs properties that provide information relevant to the event:
- ModifiedColumnValues
- PrimaryColumnValue
Event handler used if an error occurs while saving an entity.
The event handler receives an argument of the EntitySaveErrorEventArgs type.
The EntitySaveErrorEventArgs properties that provide information relevant to the event:
- Exception
- IsHandled
Event handler used before saving an entity.
The event handler receives an argument of the EntityBeforeEventArgs type.
The EntityBeforeEventArgs properties that provide information relevant to the event:
- AdditionalCondition
- IsCanceled
- KeyValue
Event handler used after updating an entity.
The event handler receives an argument of the EntityAfterEventArgs type.
The EntityAfterEventArgs properties that provide information relevant to the event:
- ModifiedColumnValues
- PrimaryColumnValue
Event handler used before updating an entity.
The event handler receives an argument of the EntityBeforeEventArgs type.
The EntityBeforeEventArgs properties that provide information relevant to the event:
- AdditionalCondition
- IsCanceled
- KeyValue
Event handler used when validating an entity.
The event handler receives an argument of the EntityValidationEventArgs type.
The EntityValidationEventArgs properties that provide information relevant to the event:
- Messages
The Terrasoft.Configuration.EntityMapper class is a utility configuration class located in the FinAppLending package of the Lending product. EntityMapper lets you map data of one Entity to another according to the rules defined in the configuration file. Use this approach to avoid code monotony.
The Lending product has two objects that contain the same columns. These objects are Contact and AppForm. Also, several details of the Contact object are similar to details of the AppForm object. When filling out the app form, you might want to use the Id column of the Contact object to retrieve the list of all its columns and values, as well as required details with their columns and values, and map this data to the app form data. After that, Creatio can populate the app form fields using the mapped data. This helps to reduce the manual entry of the same data significantly.
The following classes implement the data mapping between different entities:
- EntityMapper – implements the mapping logic.
- EntityResult – defines the resulting type of the mapped entity.
- MapConfig – a set of mapping rules.
- DetailMapConfig – sets up a list of mapping rules for details and entities connected to them.
- RelationEntityMapConfig – contains rules for mapping connected entities.
- EntityFilterMap – a filter for database query.
EntityMapper class
The Terrasoft.Configuration namespace.
The class implements the mapping logic.
Methods
Returns the mapped data for two Entity objects.
rec |
The GUID records in the database. |
config | The instance of the MapConfig class, which is a set of mapping rules. |
Retrieves the main entity from the database and matches its columns and values according to the rules specified in the config object.
record |
The GUID records in the database. |
config | The instance of the MapConfig class, which is a set of mapping rules. |
result | The dictionary of columns and their values of the mapped entity. |
Retrieves the bound entities from the database and matches them to the main entities.
relations | The list of rules for retrieving bound records. |
dictionary |
The dictionary of columns and their values. |
column |
The parent column name. |
entity |
The object that contains the name and ID of the database record. |
EntityResult class
The Terrasoft.Configuration namespace.
The class defines the resulting type of the mapped entity.
Properties
The dictionary of main entity column names and their values.
The dictionary of detail names with the list of their columns and values.
MapConfig class
The Terrasoft.Configuration namespace.
The class is a set of mapping rules.
Properties
The entity name in the database.
The dictionary with the names of columns of one entity and mapped columns of another entity.
The list of configuration objects with rules for details.
The list of detail names. Required to clean their values.
The list of configuration objects with rules for mapping bound records to the main entity.
DetailMapConfig class
The Terrasoft.Configuration namespace.
The class sets up a list of mapping rules for the details and entities connected to them.
Properties
The detail name. Ensures the detail instance is unique.
The entity name in the database.
The dictionary with the names of columns of one entity and mapped columns of another entity.
The list of configuration objects with filtering rules for more accurate selections from the database.
The list of configuration objects with rules for mapping bound records to the main entity.
RelationEntityMapConfig class
The Terrasoft.Configuration namespace.
The class contains rules for mapping connected entities.
Properties
The parent column name. When Creatio finds the column the entity data retrieval and mapping logic activates.
The entity name in the database.
The dictionary with the names of columns of one entity and mapped columns of another entity.
The list of configuration objects with filtering rules for more accurate selections from the database.
The list of configuration objects with rules for mapping bound records to the main entity.
EntityFilterMap class
The Terrasoft.Configuration namespace.
The class is a filter for database queries.
The Terrasoft.Core.Entities.EntitySchemaQueryFunction class implements the expression function.
The expression function is implemented in the following classes:
- EntitySchemaQueryFunction – the base class of expression of the entity schema query.
- EntitySchemaAggregationQueryFunction – implements the aggregate function of the expression.
- EntitySchemaIsNullQueryFunction – replaces null values with the replacement experession.
- EntitySchemaCoalesceQueryFunction returns the first not null expression from the list of arguments.
- EntitySchemaCaseNotNullQueryFunctionWhenItem is a class that describes the condition expression of the CASE SQL operator.
- EntitySchemaCaseNotNullQueryFunctionWhenItems is a collection of condition expressions of the CASE SQL operator.
- EntitySchemaStartOfCurrentHourQueryFunction(EntitySchemaQuery parentQuery, EntitySchemaQueryExpression expression, int offset = 0) : this(parentQuery, offset)
- EntitySchemaCaseNotNullQueryFunction – returns one value from the set of possible values depending on the specified conditions.
- EntitySchemaSystemValueQueryFunction – returns the expression of the system value.
- EntitySchemaCurrentDateTimeQueryFunction – implements the function for current date and time expression.
- EntitySchemaBaseCurrentDateQueryFunction – base class of the expression function for the base date.
- EntitySchemaCurrentDateQueryFunction – implements the function for current date and time expression.
- EntitySchemaDateToCurrentYearQueryFunction implements a function that converts the date expression to the same date of the current year.
- EntitySchemaStartOfCurrentWeekQueryFunction implements a function for the date expression of the current week’s start.
- EntitySchemaStartOfCurrentMonthQueryFunction implements a function for the date expression of the current month’s start.
- EntitySchemaStartOfCurrentQuarterQueryFunction implements a function for the date expression of the current quarter’a start.
- EntitySchemaStartOfCurrentHalfYearQueryFunction implements a function for the date expression of the current half-year’s start.
- EntitySchemaStartOfCurrentYearQueryFunction implements a function for the date expression of the current year’s start.
- EntitySchemaBaseCurrentDateTimeQueryFunction is a base class for a function for the base date and time expression.
- EntitySchemaStartOfCurrentHourQueryFunction implements a function for the time expression of the current hour.
- EntitySchemaCurrentTimeQueryFunction implements a function for the current time expression.
- EntitySchemaCurrentUserQueryFunction implements a function for the current user expression.
- EntitySchemaCurrentUserContactQueryFunction implements a function for the current user’s contact expression.
- EntitySchemaCurrentUserAccountQueryFunction implements a function for the current user’s account expression.
- EntitySchemaDatePartQueryFunction implements a function for a date part query.;
- EntitySchemaUpperQueryFunction – converts the argument expression characters to uppercase.
- EntitySchemaCastQueryFunction – casts the argument expression to the specified data type.
- EntitySchemaTrimQueryFunction – removes whitespaces from both ends of the expression.
- EntitySchemaLengthQueryFunction – returns the length of the expression.
- EntitySchemaConcatQueryFunction – returns a string resulting from merging the string arguments of the function.
- EntitySchemaWindowQueryFunction – implements an SQL window function.
EntitySchemaQueryFunction class
Terrasoft.Core.Entities namespace.
The base class of expression of the entity schema query.
Methods
For the current function, gets the query column expression that is generated taking into account the specified access rights.
db |
The Terrasoft.Core.DB.DBSecurityEngine object that defines the access rights. |
Gets the data type of the output returned by the function, using the passed-in data type manager.
data |
Data type manager. |
Indicates whether the output of the function has the specified data type.
data |
Data type. |
Gets the caption of the expression function.
Gets the collection of expressions of function arguments.
Verifies that the output of the function has the specified data type. Otherwise, an exception is thrown.
data |
Data type. |
EntitySchemaAggregationQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the aggregate expression function.
Constructors
Initializes the EntitySchemaAggregationQueryFunction instance of the specified aggregate function type for the specified query to the object schema.
parent |
Query against the schema of the entity that contains the function. |
Initializes the EntitySchemaAggregationQueryFunction instance of the specified aggregate function type for the specified query to the object schema.
aggregation |
The type of the aggregate function. |
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaAggregationQueryFunction instance for the specified type of aggregate function, expression, and query to the object schema.
aggregation |
The type of the aggregate function. |
expression | The query expression. |
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaAggregationQueryFunction instance that is a clone of the passed aggregate expression function instance.
source | Instance of the expression aggregate function whose clone is being created. |
Properties
The alias of the function in the SQL query.
The type of the aggregate function.
The scope of the aggregate function.
The expression of the aggregate function argument.
Methods
Serializes the aggregate function, using the specified Terrasoft.Common.DataWriter instance.
writer | A Terrasoft.Common.DataWriter instance used for serialization. |
For the aggregate function, gets the query column expression that is generated taking into account the specified access rights.
db |
The Terrasoft.Core.DB.DBSecurityEngine object that defines the access rights. |
Gets the collection of expressions of the aggregate function arguments.
Gets the data type of the output returned by the aggregate function, using the specified data type manager.
data |
Data type manager. |
Indicates whether the output of the aggregate function has the specified data type.
data |
Data type. |
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaAggregationQueryFunction instance.
Sets the To All Values scope for the current aggregate function.
Sets the To Unique Values scope for the current aggregate function.
EntitySchemaIsNullQueryFunction class
Terrasoft.Core.Entities namespace.
The class replaces null values with the replacement experession.
Constructors
Initializes a EntitySchemaIsNullQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaIsNullQueryFunction instance for the specified query to the object schema, validated expression and substitute expression.
parent |
Query against the schema of the entity that contains the function. |
check |
Expression to check for being equal to null. |
replacement |
The expression returned by the function if checkExpression is null. |
Initializes a new EntitySchemaIsNullQueryFunction instance that is a clone of the passed expression function.
source | An instance of the EntitySchemaIsNullQueryFunction function whose clone is being created. |
Properties
The alias of the function in the SQL query.
Expression of the function argument to check the null value.
Expression of the function argument that is returned if the check expression is equal to null.
Methods
Serializes the expression function, using the passed DataWriter instance.
writer | A DataWriter instance used for serializing the expression function. |
For the current function, gets the query column expression that is generated taking into account the specified access rights.
db |
The Terrasoft.Core.DB.DBSecurityEngine object that defines the access rights. |
Gets the collection of expressions of function arguments.
Gets the data type of the output returned by the function, using the passed-in data type manager.
data |
Data type manager. |
EntitySchemaCoalesceQueryFunction class
Terrasoft.Core.Entities namespace.
The class returns the first not null expression from the list of arguments.
Constructors
Initializes the new EntitySchemaCoalesceQueryFunction instance for the specified entity schema query.
aggregation |
The type of the aggregate function. |
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaCoalesceQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCoalesceQueryFunction function whose clone is being created. |
Properties
The alias of the function in the SQL query.
Collection of expressions of function arguments.
Indicates whether at least one item exists in the collection of expressions of the function arguments.
Methods
Indicates whether the output of the function has the specified data type.
data |
Data type. |
EntitySchemaCaseNotNullQueryFunctionWhenItem class
Terrasoft.Core.Entities namespace.
Class that describes the condition expression of the CASE SQL operator.
Constructors
Initializes a new instance of the EntitySchemaCaseNotNullQueryFunctionWhenItem class.
Initializes a EntitySchemaCaseNotNullQueryFunctionWhenItem instance for the specified expressions of the WHEN and THEN clauses.
when |
Expression of the WHEN condition clause. |
then |
Expression of the THEN condition clause. |
Initializes the EntitySchemaCaseNotNullQueryFunctionWhenItem instance that is a clone of the passed function.
source | The EntitySchemaCaseNotNullQueryFunctionWhenItem function whose clone is being created. |
EntitySchemaCaseNotNullQueryFunctionWhenItems class
Terrasoft.Core.Entities namespace.
The class implements a collection condition expressions of the CASE SQL operator.
Constructors
Initializes an EntitySchemaCaseNotNullQueryFunctionWhenItems instance.
Initializes a new EntitySchemaCaseNotNullQueryFunctionWhenItems instance that is a clone of the passed collection of conditions.
source | The collection of conditions whose clone is being created. |
EntitySchemaCaseNotNullQueryFunction class
Terrasoft.Core.Entities namespace.
The class returns one value from the set of possible values depending on the specified conditions.
Constructors
Initializes a new CurrentDateTimeQueryFunction instance.
Initializes the new EntitySchemaCaseNotNullQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaCaseNotNullQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCaseNotNullQueryFunction function whose clone is being created. |
Properties
The alias of the function in the SQL query.
Collection of conditions of the expression function.
Indicates whether the function has at least one condition.
Expression of the ELSE clause.
Methods
For the current expression function, defines the specified alias in the resulting SQL query.
query |
Alias to define for the current function. |
EntitySchemaSystemValueQueryFunction class
Terrasoft.Core.Entities namespace.
The class returns the expression of the system value.
EntitySchemaCurrentDateTimeQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for current date and time expression.
Constructors
Initializes a EntitySchemaCurrentDateTimeQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a EntitySchemaCurrentDateTimeQueryFunction instance that is a clone of the passed function.
source | An instance of the EntitySchemaCurrentDateTimeQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaCurrentDateTimeQueryFunction instance.
EntitySchemaBaseCurrentDateQueryFunction class
Terrasoft.Core.Entities namespace.
Base class of the expression function for the base date.
EntitySchemaCurrentDateQueryFunction class
Terrasoft.Core.Entities namespace.
EntitySchemaCurrentDateQueryFunction – implements the function for current date and time expression.
Constructors
Initializes a EntitySchemaCurrentDateQueryFunction instance with the specified offset from the base date for the request to the object schema.
parent |
Query against the schema of the entity that contains the function. |
offset | Day offset from the control date. By default, 0. |
expression | The query expression. |
Initializes a EntitySchemaCurrentDateQueryFunction instance that is a clone of the passed function.
source | An instance of the EntitySchemaCurrentDateQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaCurrentDateQueryFunction instance.
EntitySchemaDateToCurrentYearQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function that converts the date expression to the same date of the current year.
Constructors
Initializes the new EntitySchemaDateToCurrentYearQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes the new EntitySchemaDateToCurrentYearQueryFunction instance for the specified entity schema query and passed date expression.
parent |
Query against the schema of the entity that contains the function. |
expression | The query expression. |
Initializes a new EntitySchemaDateToCurrentYearQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaDateToCurrentYearQueryFunction function whose clone is being created. |
EntitySchemaStartOfCurrentWeekQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for current date expression.
Constructors
Initializes a EntitySchemaStartOfCurrentWeekQueryFunction instance with the specified offset from the base date for the request to the object schema.
parent |
Query against the schema of the entity that contains the function. |
offset | Day offset from the control date. By default, 0. |
expression | The query expression. |
Initializes a EntitySchemaStartOfCurrentWeekQueryFunction instance that is a clone of the passed-in expression function.
source | An instance of the EntitySchemaStartOfCurrentWeekQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaStartOfCurrentWeekQueryFunction instance.
EntitySchemaStartOfCurrentMonthQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for the current month start date expression.
Constructors
Initializes a EntitySchemaStartOfCurrentMonthQueryFunction instance with the specified offset from the base date for the request to the object schema.
parent |
Query against the schema of the entity that contains the function. |
offset | Day offset from the control date. By default, 0. |
expression | The query expression. |
Initializes an EntitySchemaStartOfCurrentMonthQueryFunction instance that is a clone of the passed-in expression function.
source | An instance of the EntitySchemaStartOfCurrentMonthQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaStartOfCurrentMonthQueryFunction instance.
EntitySchemaStartOfCurrentQuarterQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for the current month start date expression.
Constructors
Initializes a EntitySchemaStartOfCurrentQuarterQueryFunction instance with the specified offset from the base date for the request to the object schema.
parent |
Query against the schema of the entity that contains the function. |
offset | Day offset from the control date. By default, 0. |
expression | The query expression. |
Initializes an EntitySchemaStartOfCurrentQuarterQueryFunction instance that is a clone of the passed-in expression function.
source | An instance of the EntitySchemaStartOfCurrentQuarterQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaStartOfCurrentQuarterQueryFunction instance.
EntitySchemaStartOfCurrentHalfYearQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for the current half-year start date expression.
Constructors
Initializes a EntitySchemaStartOfCurrentHalfYearQueryFunction instance with the specified offset from the base date for the request to the object schema.
parent |
Query against the schema of the entity that contains the function. |
offset | Day offset from the control date. By default, 0. |
expression | The query expression. |
Initializes a EntitySchemaStartOfCurrentHalfYearQueryFunction instance that is a clone of the passed-in expression function.
source | An instance of the EntitySchemaStartOfCurrentHalfYearQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaStartOfCurrentHalfYearQueryFunction instance.
EntitySchemaStartOfCurrentYearQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for the current year start date expression.
Constructors
Initializes a EntitySchemaStartOfCurrentYearQueryFunction instance with the specified offset from the base date for the request to the object schema.
parent |
Query against the schema of the entity that contains the function. |
offset | Day offset from the control date. By default, 0. |
expression | The query expression. |
Initializes a EntitySchemaStartOfCurrentYearQueryFunction instance that is a clone of the passed-in expression function.
source | An instance of the EntitySchemaStartOfCurrentYearQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaStartOfCurrentHalfYearQueryFunction instance.
EntitySchemaBaseCurrentDateTimeQueryFunction class
Terrasoft.Core.Entities namespace.
Base class of the expression function for the base date and time.
EntitySchemaStartOfCurrentHourQueryFunction class
Terrasoft.Core.Entities namespace.
The class implement a function for the current hour expression.
Constructors
Initializes a EntitySchemaStartOfCurrentHourQueryFunction instance that is part of the parentQuery with the specified offset from the base date.
parent |
An EntitySchemaQuery instance. |
offset | Hour offset from the base date. |
Initializes a EntitySchemaStartOfCurrentHourQueryFunction instance that is part of the parentQuery with the specified expression and offset from the base date.
parent |
An EntitySchemaQuery instance. |
expression | The expression of the function argument. |
offset | Hour offset from the base date. |
Initializes a EntitySchemaStartOfCurrentHourQueryFunction instance that is a clone of the passed-in expression function.
source | An instance of the EntitySchemaStartOfCurrentHourQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaStartOfCurrentHourQueryFunction instance.
EntitySchemaCurrentTimeQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for current time expression.
Constructors
Initializes the new EntitySchemaCurrentTimeQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaCurrentTimeQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCurrentTimeQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaCurrentTimeQueryFunction instance.
EntitySchemaCurrentUserQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for current user expression.
Constructors
Initializes the new EntitySchemaCurrentUserQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaCurrentUserQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCurrentUserQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaCurrentUserQueryFunction instance.
EntitySchemaCurrentUserContactQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements the function for the current user's contact.
Constructors
Initializes a new EntitySchemaCurrentUserContactQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaCurrentUserContactQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCurrentUserContactQueryFunction function whose clone is being created. |
Methods
Gets the caption of the expression function.
Creates a clone of the current EntitySchemaCurrentUserContactQueryFunction instance.
EntitySchemaCurrentUserAccountQueryFunction class
Terrasoft.Core.Entities namespace.
The class implments the expression function of the current user's account.
Constructors
Initializes the new EntitySchemaCurrentUserAccountQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaCurrentUserAccountQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCurrentUserAccountQueryFunction function whose clone is being created. |
EntitySchemaDatePartQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements a function for a date part query.
Constructors
Initializes a new EntitySchemaDatePartQueryFunction instance for the specified entity schema query.
parent |
An EntitySchemaQuery instance. |
Initializes a new EntitySchemaDatePartQueryFunction instance that is part of the parentQuery with the specified interval date part for requesting the entity schema and the query expression.
parent |
An EntitySchemaQuery instance. |
interval | Datepart. |
expression | The query expression. |
Initializes a new EntitySchemaDatePartQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaDatePartQueryFunction function whose clone is being created. |
Properties
The alias of the function in the SQL query.
The date part returned by the function.
The expression of the function argument.
Methods
Serializes the function using the specified Terrasoft.Common.DataWriter instance.
writer | A Terrasoft.Common.DataWriter instance used for serialization. |
For the current function, gets the query column expression that is generated taking into account the specified access rights.
db |
The Terrasoft.Core.DB.DBSecurityEngine object that defines the access rights. |
Gets the data type of the output returned by the function, using the passed-in data type manager.
data |
Data type manager. |
Indicates whether the output of the function has the specified data type.
data |
Data type. |
Gets the caption of the expression function.
Gets the collection of expressions of function arguments.
Creates a clone of the current EntitySchemaUpperQueryFunction instance.
EntitySchemaUpperQueryFunction class
Terrasoft.Core.Entities namespace.
The class converts the argument expression characters to uppercase.
Constructors
Initializes the new EntitySchemaUpperQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes the new EntitySchemaUpperQueryFunction instance for the specified entity schema query and passed date expression.
parent |
Query against the schema of the entity that contains the function. |
expression | The query expression. |
Initializes a new EntitySchemaUpperQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaUpperQueryFunction function whose clone is being created. |
EntitySchemaCastQueryFunction class
Terrasoft.Core.Entities namespace.
The class casts the argument expression to the specified data type.
Constructors
Initializes a new EntitySchemaCastQueryFunction instance for the specified query to the schema of the object with the specified target data type.
parent |
Query against the schema of the entity that contains the function. |
cast |
The target data type. |
Initializes a new EntitySchemaCastQueryFunction instance with the specified expression and target data type.
parent |
Query against the schema of the entity that contains the function. |
expression | The query expression. |
cast |
The target data type. |
Initializes a new EntitySchemaCastQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaCastQueryFunction function whose clone is being created. |
Properties
The alias of the function in the SQL query.
The expression of the function argument.
The target data type.
EntitySchemaTrimQueryFunction class
Terrasoft.Core.Entities namespace.
The class removes whitespaces from both ends of the expression.
Constructors
Initializes the new EntitySchemaTrimQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes the new EntitySchemaTrimQueryFunction instance for the specified entity schema query and passed date expression.
parent |
Query against the schema of the entity that contains the function. |
expression | The query expression. |
Initializes a new EntitySchemaTrimQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaTrimQueryFunction function whose clone is being created. |
EntitySchemaLengthQueryFunction class
Terrasoft.Core.Entities namespace.
The class returns the length of the expression.
Constructors
Initializes the new EntitySchemaLengthQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes the new EntitySchemaLengthQueryFunction instance for the specified entity schema query and passed date expression.
parent |
Query against the schema of the entity that contains the function. |
expression | The query expression. |
Initializes a new EntitySchemaLengthQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaLengthQueryFunction function whose clone is being created. |
EntitySchemaConcatQueryFunction class
Terrasoft.Core.Entities namespace.
The class returns a string resulting from merging the string arguments of the function.
Constructors
Initializes the new EntitySchemaConcatQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes a new EntitySchemaConcatQueryFunction instance for the specified array of expressions and entity schema query.
parent |
Query against the schema of the entity that contains the function. |
expressions | Array of expressions. |
Initializes a new EntitySchemaConcatQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaConcatQueryFunction function whose clone is being created. |
Properties
The alias of the function in the SQL query.
Collection of expressions of function arguments.
Indicates whether at least one item exists in the collection of expressions of the function arguments.
EntitySchemaWindowQueryFunction class
Terrasoft.Core.Entities namespace.
The class implements an SQL window function.
Constructors
Initializes the new EntitySchemaWindowQueryFunction instance for the specified entity schema query.
parent |
Query against the schema of the entity that contains the function. |
Initializes the new EntitySchemaWindowQueryFunction instance for the specified entity schema query.
function | Nested query function. |
esq | Query against the entity schema. |
Initializes the new EntitySchemaWindowQueryFunction instance for the specified entity schema query.
function | Nested query function. |
parent |
Query against the schema of the entity that contains the function. |
partition |
The expression for splitting the query. |
order |
The expression for ordering the query. |
Initializes a new EntitySchemaWindowQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaQueryFunction function whose clone is being created. |
Initializes a new EntitySchemaWindowQueryFunction instance that is a clone of the passed function.
source | The EntitySchemaWindowQueryFunction function whose clone is being created. |
The Terrasoft.Core.Entities namespace.
The Terrasoft.Core.Entities.EntitySchemaQueryOptions class configures entity schema queries.
Constructors
Initializes a class instance. By default, the PageableRowCount property is set to 14 in the constructor.
Properties
The number of page records in the resulting dataset that the query returns.
The direction of paged output.
Prior | Previous page. |
First | First page. |
Current | Current page. |
Next | Next page. |
The values of the paged output conditions.
The maximum nesting level of a hierarchical query.
The name of the column by which to build a hierarchical query.
The hierarchical column's seed value from which to build the hierarchy.