Execute operations in the background to run time-consuming operations without holding up the UI.
The Terrasoft.Core.Tasks.Task class implements the StartNew() and StartNewWithUserConnection() methods that start background operations. You can use base .NET data types (e. g., string, int, Guid, etc) or custom types as parameters. Unlike StartNew(), the StartNewWithUserConnection() method runs background operations that require the UserConnection user connection.
The MessagePack-CSharp module converts parameters accepted by the background operation to a byte array. View the implementation on the MessagePack-CSharp module on GitHub. If the module cannot serialize or deserialize a parameter value, the module will throw an exception.
Describe the execution of an asynchronous operation in an individual class that must implement the IBackgroundTask<in TParameters> interface.
If the action requires a user connection, implement the IUserConnectionRequired interface in the class as well.
Implement the methods of the Run and SetUserConnection interfaces in the class that implements the IBackgroundTask<in TParameters> and IUserConnectionRequired interfaces.
Good to know when implementing the methods:
- Do not pass UserConnection to the Run method.
- Do not call the SetUserConnection method from the Run method. The application core calls this method and initializes a UserConnection instance when the background operation starts.
- Pass structures that comprise only simple data types to the Run method. Complex class instances are highly likely to cause parameter serialization errors.
1. Create a class for the activity object
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Source code on the section list toolbar.
-
Go to the Schema Designer and fill out the schema properties:
- Set Code to "UsrActivityData."
- Set Title to "ActivityData."
Click Apply to apply the properties.
-
Add the source code in the Schema Designer.
- Click Save then Publish on the Designer’s toolbar.
2. Create a class that adds an activity
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Source code on the section list toolbar.
-
Go to the Schema Designer and fill out the schema properties:
- Set Code to "UsrBackgroundActivityCreator."
- Set Title to "BackgroundActivityCreator."
Click Apply to apply the properties.
-
Add the source code in the Schema Designer.
The UsrBackgroundActivityCreator class implements the IBackgroundTask<UsrActivityData> and IUserConnectionRequired interfaces. After a forced 30 seconds delay, the Run() method creates an instance of the Activities section object based on the given UsrActivityData instance.
- Click Save then Publish on the Designer’s toolbar.
3. Create a business process that starts the background operation
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Business process on the section list toolbar.
-
Go to the Process Designer and fill out the process properties:
- Set the Title property in the element setup area to "Background Task Example Process."
- Set the Code property on the Settings tab of the element setup area to "UsrBackgroundTaskExampleProcess."
-
Implement the business process.
-
Click System actions in the Designer’s element area and place a Script task element between the Simple start event and Terminate end event on the Process Designer’s working area.
-
Set the name of the Script task element to "Create background task."
-
Add the code of the Script task element.
-
Click the
button in the Usings block on the Methods tab of the Process Designer and add the Terrasoft.Configuration namespace. This is required for the business process to support the implementations of the class for the activity object and class that adds an activity.
-
- Click Save on the Designer’s toolbar. A pop-up box will appear after Creatio saves the process.
- Click Publish in the box to compile the code of the Script task element.
Outcome of the example
To run the Background Task Example Process business process, click Run on the Process Designer’s toolbar.
As a result, the Background Task Example Process business process will add the Activity created by background task record to the list view of the Activities section list.
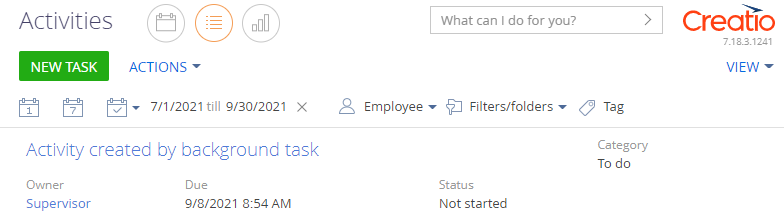