Implement a custom user task
Implement a custom User task of sum numbers user task. The task must calculate the sum of two integers that are specified in the task parameters. Display the calculation result on an auto-generated business process page.
1. Create a user task schema
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → User task on the section list toolbar.
-
Fill out the schema properties in the User Task Designer.
- Set Code to "UsrSumNumbersProcessUserTask."
- Set Title to "User task of sum numbers."
Click Apply to apply the properties.
-
Add a user task parameter that contains the first integer.
-
Add user task parameters that contain the following in a similar way:
- second integer
- integer sum
View the properties of the parameters to add in the table below.
Parameter
Property
Property value
Parameter that contains the second integer
Code
"SecondNumber"
Title
"Second number"
Type
Select "Integer"
Serializable checkbox
Set
Parameter that contains the integer sum
Code
"SumNumbers"
Title
"Sum of numbers"
Type
Select "Integer"
Serializable checkbox
Set
-
Implement the logic to add integers. To do this, implement the
InternalExecute()
method of the user task schema’s automatically generated source code.InternalExecute() methodprotected override bool InternalExecute(ProcessExecutingContext context) {
/* Execute operations with task parameters. */
SumNumbers = FirstNumber + SecondNumber;
/* Specify that the task was completed successfully. */
return true;
} -
Publish the schema.
2. Implement a business process
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Business process on the section list toolbar.
-
Fill out the properties of the business process:
- Set the Title property in the element setup area to "Sum of numbers process."
- Set the Code property on the Settings tab of the element setup area to "UsrProcessSumNumbers."
-
Implement the business process.
View the business process in the figure below.
-
Add the user task.
-
Click System actions in the Designer’s element area and place a User task element between the Simple start event and Terminate end event in the Process Designer’s working area.
-
Fill out the user task properties.
-
Select "User task of sum numbers" in the Which user task to perform? property.
-
Fill out the user task parameters.
- Set First number to "12."
- Set Second number to "23."
-
-
-
Add an auto-generated page.
-
Click User actions in the Designer’s element area and place an Auto-generated page element after the User task element in the Process Designer’s working area.
-
Fill out the auto-generated page properties.
- Set Title to "Sum of numbers page."
- Set Page title to "Sum of numbers page."
-
Add an auto-generated page.
-
-
-
Publish the schema.
Outcome of the example
To view the outcome of the example, run the Sum of numbers process
business process.
As a result, Creatio will open the Sum of numbers page auto-generated page. The page will display the sum of 2 integers that are specified in the business process parameters.
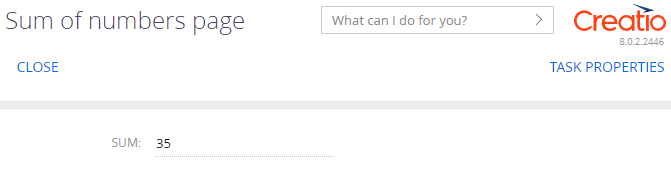
Source code
namespace Terrasoft.Core.Process.Configuration {
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Globalization;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.Configuration;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Process;
#region Class: UsrSumNumbersProcessUserTask
/// <exclude/>
public partial class UsrSumNumbersProcessUserTask {
#region Methods: Protected
protected override bool InternalExecute(ProcessExecutingContext context) {
/* Execute operations with task parameters. */
SumNumbers = FirstNumber + SecondNumber;
/* Specify that the task was completed successfully. */
return true;
}
#endregion
#region Methods: Public
public override bool CompleteExecuting(params object[] parameters) {
return base.CompleteExecuting(parameters);
}
public override void CancelExecuting(params object[] parameters) {
base.CancelExecuting(parameters);
}
public override string GetExecutionData() {
return string.Empty;
}
public override ProcessElementNotification GetNotificationData() {
return base.GetNotificationData();
}
#endregion
}
#endregion
}