Medium
Create a replacing view model schema of the contact page
- Go to the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "ContactPageV2."
- Set Title to "Display schema - Contact card."
- Select "ContactPageV2" in the Parent object property.
-
Add a localizable string.
- Click the
button in the context menu of the Localizable strings node.
-
Fill out the localizable string properties.
- Set Code to "InvalidPhoneFormatMessage."
- Set Value to "Enter the number in format +44 XXX XXX XXXX.
- Click Add to add a localizable string.
- Click the
- Add the ConfigurationConstants module as a dependency to the declaration of the view model class.
-
Implement the validation of the String type field.
To do this, implement the following methods in the methods property:
- phoneValidator(). The validator method that checks whether the condition is true.
- setValidationConfig(). The overloaded base method that binds the validator method to the Phone column.
View the source code of the replacement view model of the contact page below.
- Click Save on the Designer's toolbar.
Outcome of the example
To view the outcome of the example:
- Refresh the Contacts section page.
- Enter a phone number that does not match the +44 XXX XXX XXXX pattern in the Business phone field.
As a result, Creatio will display the corresponding warning on the contact page.
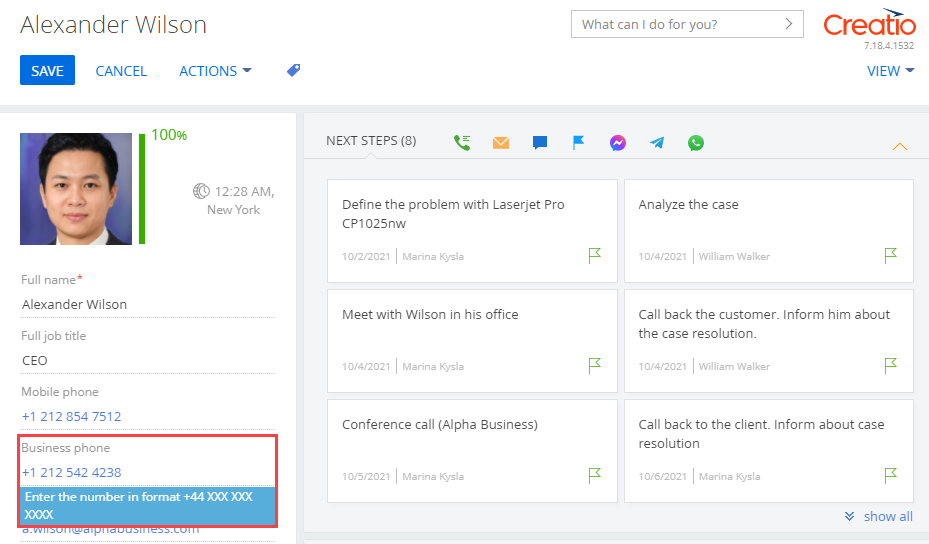
If you try to save a contact whose phone number does not match the +44 XXX XXX XXXX pattern, a message box will open.
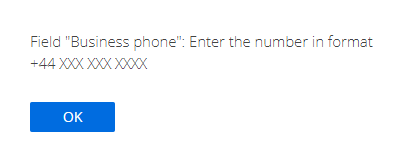
Resources