Feature toggle is a software development technique. The purpose of the feature toggle is to manage the additional feature status in a live application.
Feature toggle lets you use continuous integration while preserving the working capacity of the application and hiding features you are still developing. The application source code contains the additional feature block and the conditional operator that checks the feature status.
Learn more about the Feature toggle mechanism in Wikipedia.
Store the feature details
Creatio stores the feature details in the following database tables:
- [Feature]. Stores the list of features you can toggle. Empty by default.
- [AdminUnitFeatureState] (the [FeatureState] column). Stores the is the additional feature status (turned on/off). The [AdminUnitFeatureState] table connects the [Feature] and the [SysAdminUnit] database tables. [SysAdminUnit] is the additional feature status for Creatio users and user groups.
View the relationship chart between tables that store the additional feature details in the figure below.
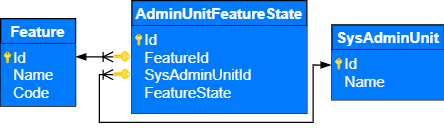
View the primary columns of the [Feature] database table below.
Column | Type | Description |
---|---|---|
[Id] | uniqueidentifier | The unique ID of the record. |
[Name] | nvarchar(250) | The name of the feature. |
[Code] | nvarchar( 50 ) | The code of the feature. |
View the primary columns of the [AdminUnitFeatureState] database table below.
Column | Type | Description |
---|---|---|
[Id] | uniqueidentifier | The unique ID of the record. |
[FeatureId] | uniqueidentifier | The unique ID of the record from the [Feature] table. |
[SysAdminUnitId] | uniqueidentifier | The unique ID of the user record. |
[FeatureState] | int | The status of the feature (1: turned on, 0: turned off). |
Turn on the additional feature
You can manage the additional feature status in several ways:
- for current Creatio user
- for all Creatio users
Turn on the feature for current Creatio user
-
Open the Features page that lets you use to turn the feature on selectively.
- Turn on the corresponding switch for the needed feature.
- Click Save changes.
The tooltip displays the status of the feature for the user group of the current user. Changing the feature status on the page does not change the feature status for the user group of the current user. In this case, Creatio adds an extra rule only for the current user. This rule has a higher priority than the rule for the user group.
View the example of the Features page in the figure below.
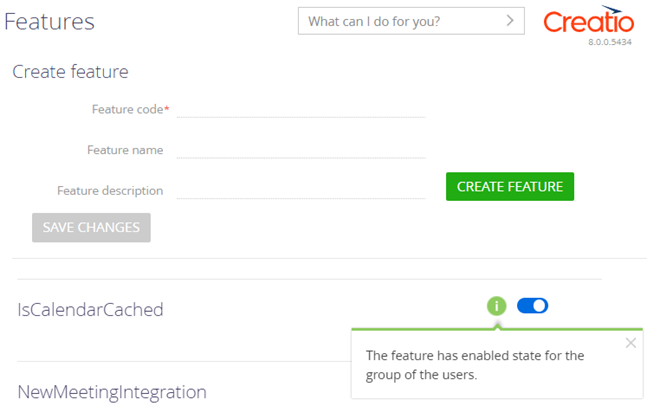
Turn on the feature for all Creatio users
To turn on the feature for all Creatio users, execute a database query. You can use one of example queries below.
featureCode specifies the name of the needed feature.
You can use SQL queries to turn on the feature for the user group, turn off the feature for current user and vice versa.
Run the SQL query that has 0 in the [FeatureState] column of the [AdminUnitFeatureState] table to turn off the feature for all Creatio users.
Implement a custom feature
-
Add a custom feature.
- Open the Features page that lets you turn the feature on selectively.
-
Fill out the properties of the feature to add:
- Enter the custom feature code in the Feature code property. Required.
- Enter the custom feature name in the Feature name property.
- Enter the custom feature description in the Feature description property.
- Click Create feature.
As a result, Creatio will add the custom feature to the Features page list.
-
Implement a custom feature in the source code. Implement the custom feature in the block of the conditional operator that checks the feature status (i. e. the [FeatureState] column value from the [AdminUnitFeatureState] database table).
You can implement a custom feature in several ways:
- In the front-end. To do this, follow the guide in a different section: Implement a custom feature in the front-end.
- In the back-end. To do this, follow the guide in a a different section: Implement a custom feature in the back-end.
Implement a custom feature in the front-end
- Create a view model schema. To do this, follow the guide in a separate article: Client module.
-
Add the source code in the Module Designer. You can use the template below.
The getIsFeatureEnabled method of the BaseSchemaViewModel base view model schema lets you streamline the workflow. As such, you can replace the Terrasoft.Features.getIsEnabled method with the this.getIsFeatureEnabled("FeatureCode") method.
- Click Save.
Refresh the page to add the custom feature to the client code and display the feature on the browser page.
Implement a custom feature in the back-end
- Create a Source code schema. To do this, follow the guide in a separate article: Source code (C#).
-
Add the source code in the Source Code Designer.
The Terrasoft.Configuration.FeatureUtilities class provides a set of extension methods to the UserConnection class. These methods let you use the Feature toggle functionality in the source code schemas of the Creatio back-end. You can use the template below.
-
Set the feature status by calling the SetFeatureState() method. You can use the template below.
The Terrasoft.Configuration namespace.
The Terrasoft.Configuration.FeatureUtilities class provides a set of extension methods to the UserConnectionclass. These methods let you use the Feature Toggle functionality in the source code schemas of Creatio back-end. The FeatureUtilities class also declares the enumeration of feature statuses (the [FeatureState] column of the [AdminUnitFeatureState] database table).
Methods
Returns the feature status.
source | The user connection. |
code | The feature code. |
Returns the feature status.
source | The user connection. |
code | The code of the feature. |
sysAdminUnitId | The unique record ID. |
Returns the feature status for any user.
source | The user connection. |
code | The code of the feature. |
Returns the feature status for all users.
source | The user connection. |
code | The code of the feature. |
Returns all feature statuses.
source | The user connection. |
Returns data about all features and their statuses.
source | The user connection. |
Sets the feature status.
source | The user connection. |
code | The code of the feature. |
state | The new status of the feature. |
forAllUsers | Sets the status of the feature for all users. |
Sets the feature status or creates the feature if it does not exist.
source | The user connection. |
code | The code of the feature. |
state | The new status of the feature. |
forAllUsers | Sets the status of the feature for all users. |
Creates the feature.
source | The user connection. |
code | The code of the feature. |
name | The name of the feature. |
description | The description of the feature. |
Checks if the feature is turned on.
source | The user connection. |
code | The code of the feature. |
Checks if the feature is turned off.
source | The user connection. |
code | The code of the feature. |
sysAdminUnitId | The unique record ID. |