ProcessEngineService.svc is a web service implemented in the Creatio service model to run business processes. Use the web service to integrate external apps with Creatio.
Run a business process from an external app
The ProcessEngineService.svc web service grants access to Creatio objects.
The Terrasoft.Core.Service.Model.ProcessEngineService class implements the ProcessEngineService.svc functionality. The primary methods of the ProcessEngineService.svc service are as follows:
- Execute(). Runs a business process. Passes a set of incoming business process parameters and returns the process execution result.
- ExecProcElByUId(). Executes a specific business process element. Can only execute an element of a running process.
Run a business process from the front-end
You can run a business process from the front-end in the following ways:
- executeProcess() method of the PrcessModuleUtilities module
- execute() method of the Terrasoft.RunProcessRequest class
Run a business process using the executeProcess() method
To run a business process from the front-end, use the executeProcess() method of the ProcessModuleUtilities module in the NUI package. This module provides a convenient interface for executing requests to the ProcessEngineService.svc service.
To run a business process from a client module schema:
- Add the ProcessModuleUtilities module as a dependency to the module of the page that calls the service.
- Call the executeProcess(args) method of the ProcessModuleUtilities module. Set the args object as a parameter.
The properties of the args object are as follows:
- sysProcessName. The name of the called process (optional if the sysProcessId property is used).
- sysProcessId. The ID of the called process (optional if the sysProcessName property is used).
- parameters. The object whose properties are the incoming parameters of the called process.
Run a business process using the execute() method
-
Create the RunProcessRequest class. Set the properties of the process to run and the needed resulting parameters in this class.
- schemaName. The name of the called process ( the Code field of the Process Designer).
- schemaUId. The ID of the called process.
- Parameters. The object whose properties are the incoming parameters of the called process.
- resultParameterNames. The array of parameters whose values must be received when executing the process.
- Call the execute() method of the RunProcessRequest class.
Run a business process from the back-end
To run a business process from the back-end, call the Execute() method of the Terrasoft.Core.Process.IProcessExecutor interface. The IProcessExecutor interface provides a set of the Execute() method overloads for solving custom tasks. View the description of the IProcessExecutor interface in the .NET class library.
Run a business process without passing or receiving parameters
View the ways to run a business process without passing or receiving parameters in the table below.
|
|
|
---|---|---|
|
|
processSchemaName. The name of the business process schema. |
|
|
processSchemaUId. The ID of the business process schema. |
Run a business process with passing incoming parameters
View the ways to run a business process with passing incoming parameters in the table below.
|
|
|
---|---|---|
|
|
processSchemaName. The name of the business process schema. parameterValues. The key-value collection of incoming parameters. In the parameter collection, the key is the name of the business process parameter, the value is the parameter value converted to a string type. |
|
|
processSchemaUId. The ID of the business process schema. parameterValues. The key-value collection of incoming parameters. |
View the examples that run a business process below.
Run a business process using a received single outgoing parameter
View ways to run a business process using a received single outgoing parameter in the table below.
|
|
|
---|---|---|
|
|
processSchemaName. The name of the business process schema. resultParameterName. The name of the outgoing parameter. |
|
|
processSchemaName. The name of the business process schema. resultParameterName. The name of the outgoing parameter. parameterValues. The key-value collection of incoming parameters. |
|
|
processSchemaUId. The ID of the business process schema. resultParameterName. The name of the outgoing parameter. |
|
|
processSchemaUId. The ID of the business process schema. resultParameterName. The name of the outgoing parameter. parameterValues. The key-value collection of incoming parameters. |
View the examples that run a business process below.
Run a business process with receiving multiple outgoing parameters
View the ways to run a business process with receiving multiple outgoing parameters in the table below.
|
|
|
---|---|---|
|
|
processSchemaName. The name of the business process schema. resultParameterNames. The collection of outgoing parameters. |
|
|
processSchemaName. The name of the business process schema. parameterValues. The key-value collection of incoming parameters. resultParameterNames. The collection of outgoing parameters. |
|
|
processSchemaUId. The ID of the business process schema. resultParameterNames. The collection of outgoing parameters. |
|
|
processSchemaUId. The ID of the business process schema. parameterValues. The key-value collection of incoming parameters. resultParameterNames. The collection of outgoing parameters. |
View the examples that run a business process below.
Run a business process using a received collection of outgoing parameters returns an object of the ProcessDescriptor type. To receive the values of outgoing parameters, use the ResultParameterValues property of the IReadOnlyDictionary<string, object> type in the Terrasoft.Core.Process.ProcessDescriptor class. View the description of the ProcessDescriptor class in the .NET class library.
1. Create a process that adds a contact
- Open the Configuration section and select a custom package to add the business process.
- Open the Process Designer.
-
Fill out the business process properties.
- Set Name to "Add New External Contact."
- Set Code to "UsrAddNewExternalContact."
Use default values for other properties.
-
Add business process parameters.
The process uses parameters to pass the data of the new contact: name and phone number.
View the process parameter values to fill out in the table below.
Process parameter valuesTitle Code Data type ContactName parameter "Contact Name" "ContactName" "Text (50 characters)" ContactPhone parameter "Contact Phone" "ContactPhone" "Text (50 characters)" -
Add the ScriptTask element.
-
Fill out the element properties.
- Set Name to "Add contact."
- Set Code to "ScriptTaskAddContact."
-
Implement the mechanism that adds a new contact.
To edit the script code, double-click a diagram element. This opens a window on the element setup area. Enter and edit the source code in the window.
-
- Click Save.
2. Create a process that reads all Creatio contacts
The business process that creates the index of all Creatio contacts includes a single ScriptTask element as well.
To create a process that reads all Creatio contacts:
- Open the Configuration section and select a custom package to add the business process.
- Open the Process Designer.
-
Fill out the business process properties.
- Set Name to "Get All Contacts."
- Set Code to "UsrGetAllContacts."
Use default values for other properties.
-
Add the business process parameter.
Fill out the values of the business process parameter.
- Set Title to "Contact List."
- Set Code to "ContactList."
- Set Data type to "Unlimited length text."
The parameter returns the JSON index of all Creatio contacts.
-
Add the ScriptTask element.
-
Fill out the element properties.
- Set Name to "Get all contacts."
- Set Code to "ScriptTaskGetAllContacts."
-
Implement the mechanism that reads the Creatio contacts.
To edit the script code, double-click the diagram. This opens a window on the element setup area. Enter and edit the source code in the window.
-
- Click Save.
Outcome of the example
-
Run business processes from the browser address bar.
-
Run the process that adds a contact. To do this, enter the following URL in the browser address bar.
As a result, the process will add a contact to Creatio.
-
Run the process that reads all Creatio contacts. To do this, enter the following URL in the browser address bar.
As a result, the browser window will display the JSON object that contains the contact collection.
-
-
Run business processes from the console app.
View the complete source code of the custom console app that runs business processes via the ProcessEngineService.svc service on GitHub.
- Perform the authentication. To do this, use the AuthService.svc authentication service. Use the example available in a separate article: Implement authentication using C#.
-
Add a string field that contains the base service URL to the source code of the Program class.
-
Add a GET method that runs the business process that creates a contact to the source code of the Program class.
-
Add GET method that runs the business process that reads all Creatio contacts to the Program class source code.
-
Call the added methods in the main app method after a successful authentication.
1. Implement the Holding a meeting custom business process
- Create the Holding a meeting business process. To do this, implement the example from a separate article: Send emails via business processes.
-
Add the business process parameter.
Fill out the values of the business process parameter.
- Set Title to "Meeting contact."
- Set Code to "ProcessSchemaContactParameter."
- Set Data type to "Unique identifier."
-
Add the "[#Meeting contact#]" incoming parameter to the Contact property of the Call customer process action.
2. Implement the custom action on the account page
- Create a replacing view model schema of the account page To do this, follow the guide in a separate article: Add an action to the record page.
-
Add a localizable string that contains the menu item caption.
- Click the
button in the context menu of the Localizable strings node.
-
Fill out the localizable string properties.
- Set Code to "CallProcessCaption."
- Set Value to "Schedule a meeting."
- Click Add to add a localizable string.
- Click the
-
Implement the menu item behavior.
- Add the ProcessModuleUtilities module as a dependency.
-
Implement the following methods in the methods property:
- callCustomProcess(). Action handler method. Implements the executeProcess() method of the ProcessModuleUtilities module. Pass the name of the business process and object that contains the initialized incoming process parameters to the module as a parameter.
- isAccountPrimaryContactSet(). Checks whether the primary contact of the account is filled out.
- getActions(). An overloaded base method. Returns the action collection of the replacing page.
View the source code of the replacing view model schema of the account page below.
- Click Save on the Module Designer’s toolbar.
3. Implement the custom action of the section page
- Create a replacing view model schema of the section. To do this, follow the guide in a separate article: Add an action to the record page.
- Add a localizable string that contains the menu item caption. To do this, take step 2 of the procedure to implement a custom action of the account page.
-
Implement the menu item behavior. To do this, implement the isAccountPrimaryContactSet() method in the methods property. The method checks whether the primary contact of the account is filled out.
View the source code of the replacing view model schema of the section page below.
- Click Save on the Module Designer’s toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Accounts section page.
As a result, Creatio will add the Schedule a meeting action to the account page. This action will be active if the primary contact of the account is filled out.
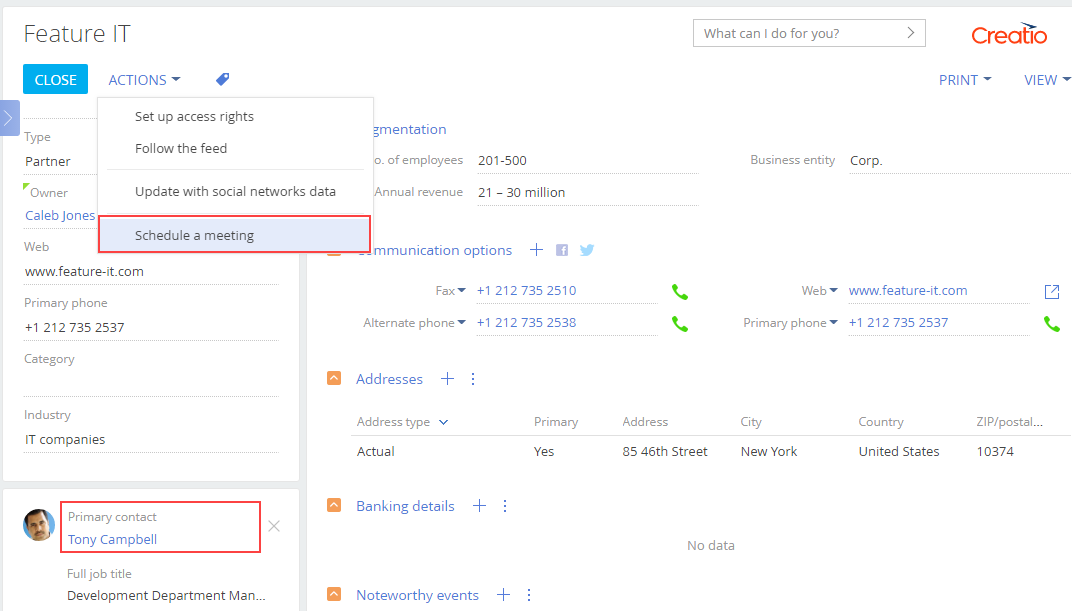
Select the action to run the Holding a meeting custom business process . The primary contact of the account will be passed to the business process as a parameter.
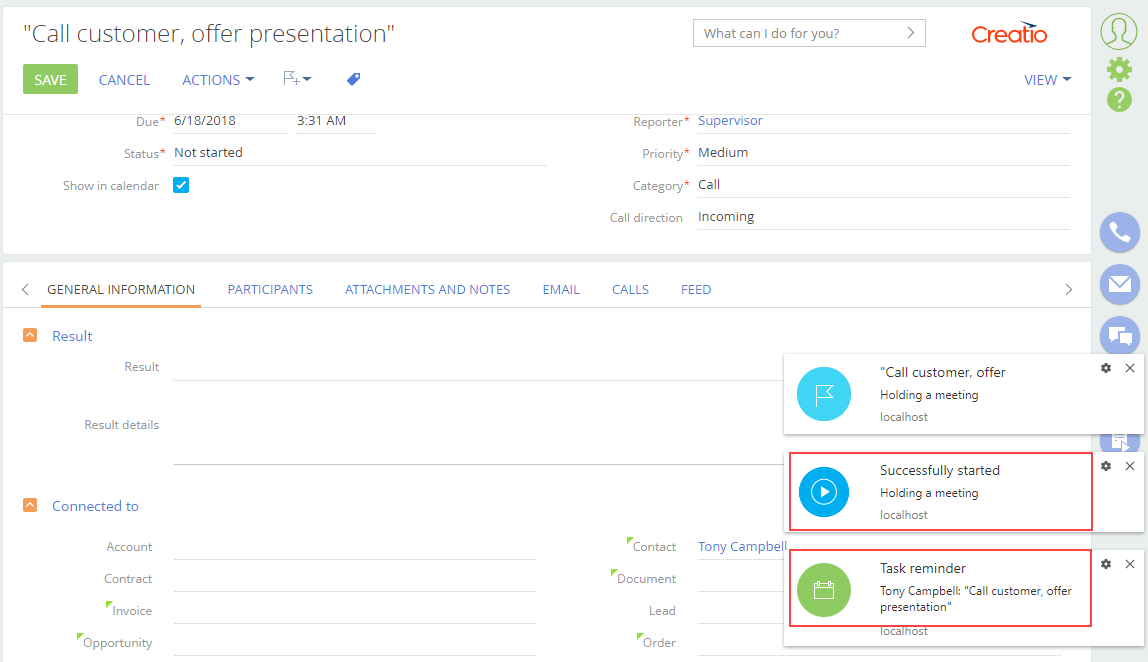
1. Implement the Result parameters custom business process
- Open the Configuration section and select a custom package to add the business process.
-
Click Add → Business process on the section list toolbar.
-
Fill out the business process properties.
- Set Title to "Result parameters."
- Set Code to "UsrParametersProcess."
Use default values for the other properties.
-
Add business process parameters.
View the process parameters values to fil out in the table below.
Process parameters valuesTitle Code Data type Direction "Primary contact" "Process Schema "Contact Parameter "Unique identifier" "Input" "Contact age" "Process Contact "Age "Integer" "Output" "Full job title" "Process Full "Job "Text (500 characters)" "Output" -
Implement data reading.
-
Add the Read data element to the Process Designer workspace.
-
Fill out the element properties.
- Set Which data read mode to use? to "Read the first record in the selection."
- Set Which object to read data from? to "Contact."
- Set the filter by the Id column in the How to filter records? property. Set the value of the "Primary contact" incoming parameter as the column value.
-
-
Add the values of the outgoing parameters.
- Go to the Parameters tab.
- Enter "[#Read data.First item of resulting collection.Full job title#]" in the Value field for the Full job title parameter.
- Enter "[#Read data.First item of resulting collection.Age#]" in the Value field for the Contact age parameter.
- Click Save on the Process Designer’s toolbar.
2. Create a replacing view model schema of the account page
- Open the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "AccountPageV2."
- Set Title to "Account edit page."
- Set Parent object to "AccountPageV2."
-
Add a localizable string that contains the menu item caption.
- Click the
button in the context menu of the Localizable strings) node.
-
Fill out the localizable string properties.
- Set Code to "CallProcessParametersCaption."
- Set Value to "Recieve parameters."
- Click Add to add a localizable string.
- Click the
-
Implement the menu item logic.
Implement the following methods in the methods property:
- isAccountPrimaryContactSet(). Checks whether the primary contact of the account is filled out and defines the visibility of the menu item.
- callCustomProcess(). Action handler method. Runs the business process and displays the values of the resulting parameters in the dialog.
- getActions(). An overloaded base method. Returns the action collection of the replacing page.
View the source code of the replacing view model schema of the account page below.
- Click Save on the Module Designer’s toolbar.
Creatio does not display the custom account page action in combined mode.
3. Create a replacing view model schema of the section page
- Open the Configuration section and select a custom package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the schema properties.
- Set Code to "AccountSectionV2."
- Set Title to "Accounts section."
- Set Parent object to "AccountSectionV2."
- Add a localizable string that contains the menu item caption. To do this, take step 4 of the procedure to create a replacing view model schema of the account page.
-
Implement the menu item behavior. To do this, implement the isAccountPrimaryContactSet() method in the methods property. The method checks whether the primary contact of the account is filled out and defines the visibility of the menu item.
View the source code of the replacing view model schema of the section page below.
- Click Save on the Module Designer’s toolbar.
Outcome of the example
To view the outcome of the example:
- Refresh the Accounts section page.
- Open an account page, for example, Vertigo Systems.
As a result, Creatio will add the Receive parameters action to the account page.
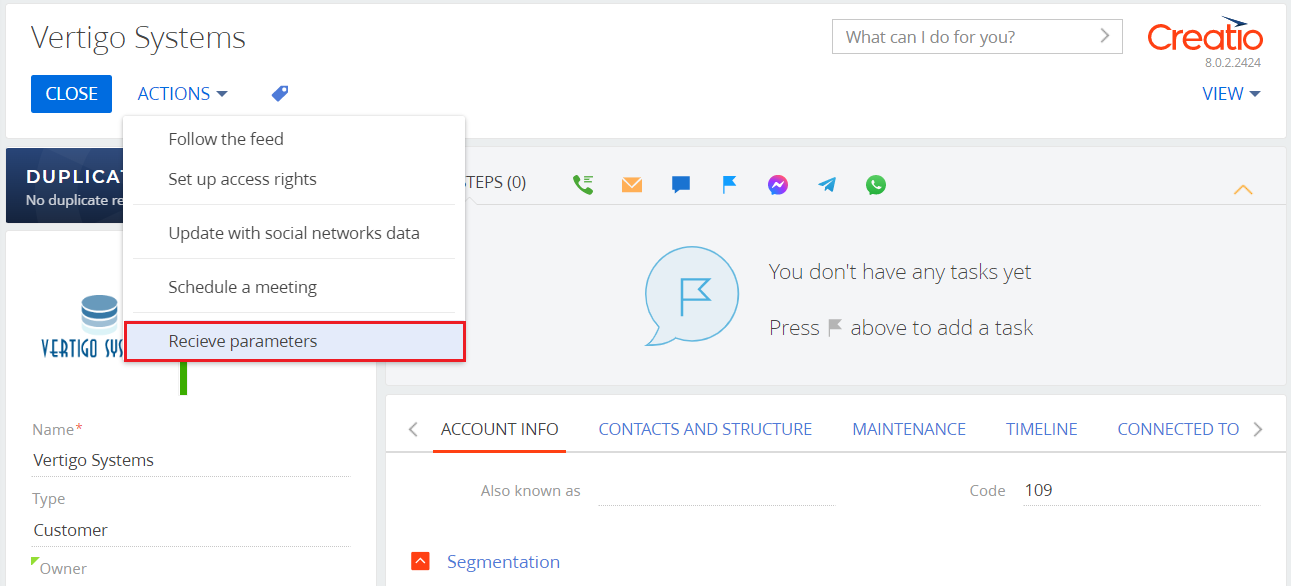
Click the Receive parameters action to run the Result parameters business process. The primary contact of the account will be passed to the business process as a parameter. The job title and age of the account’s primary contact will be displayed as the resulting parameters of the business process.
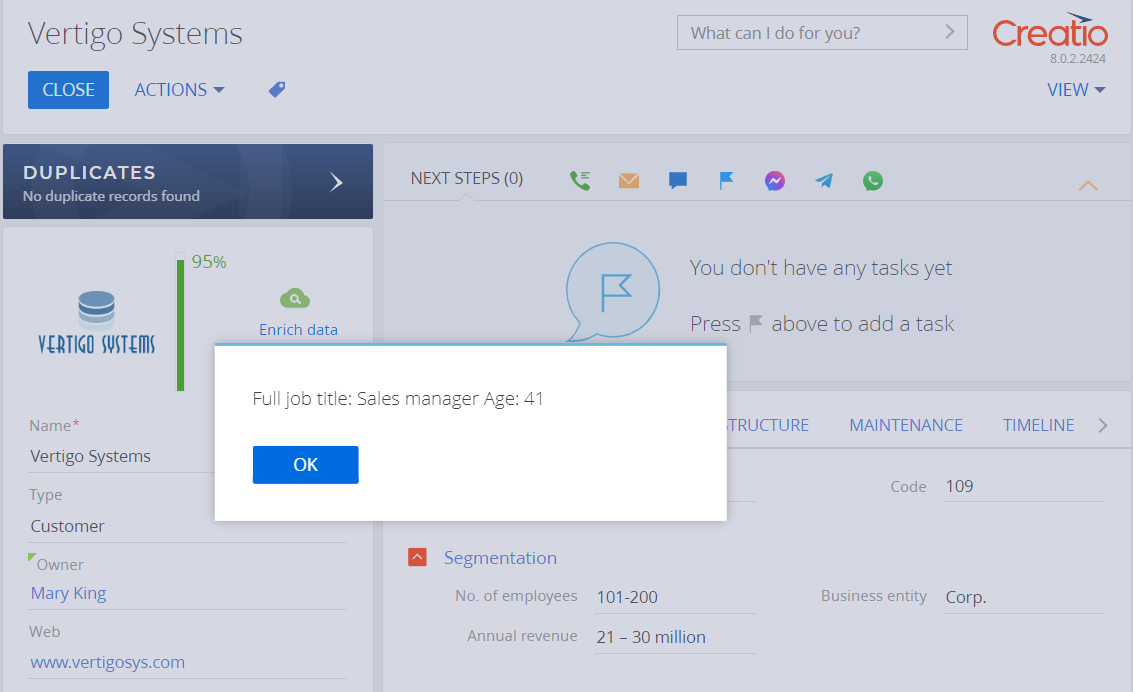
The action will be active if the account has a primary contact, i. e., the Primary contact field of the account page is filled out. For example, the action is inactive for the Wilson & Young account.
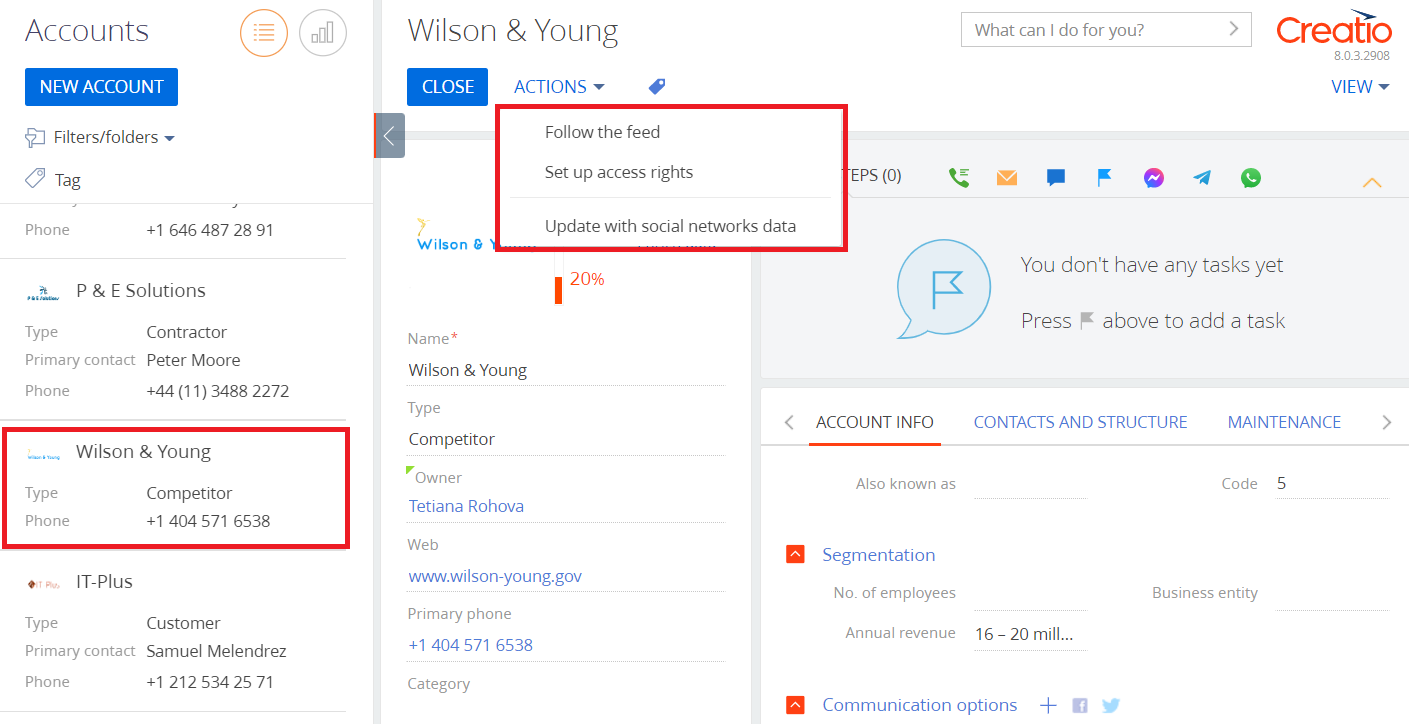
The Receive parameters custom action will be displayed on the Accounts section page and active for the selected account that has a primary contact.
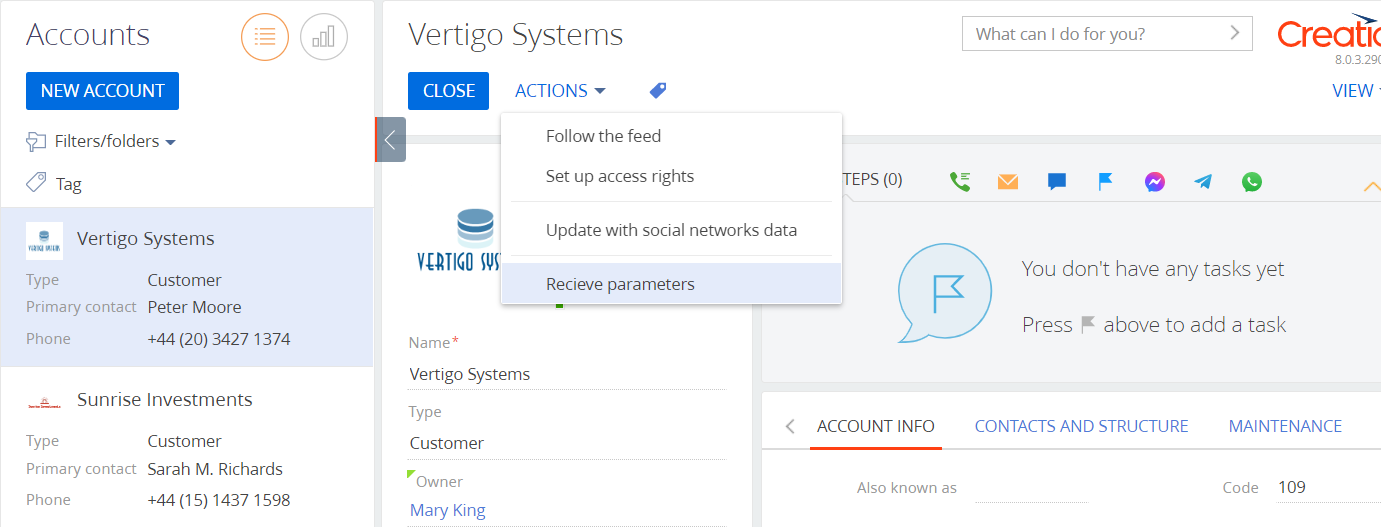
Request string
Method of the request to run business processes.
Creatio instance URL.
The path to the service that runs business processes. Unmodifiable part of the request.
The address of the web service that runs business processes. Unmodifiable part of the request.
The method of the web service that runs business processes.
Primary methods:
- Execute(). Runs a business process. Lets you pass a set of incoming parameters and return the outcome of the web service execution.
- ExecProcElByUId(). Executes a specific business process element. You can only execute an element of the running process. If Creatio has already executed the process element by the time the method is called, the element is not executed again.
Applicable for the Execute() method.
The name of the business process schema. You can find the name of the business process schema in the Configuration section.
Applicable for the Execute() method.
The variable of the process parameter code. Unmodifiable part of the request.
Applicable for the Execute() method.
The code of the process parameter that stores the outcome of the process execution. If you omit this parameter, the web service runs the business process without waiting for the outcome of the process execution. If the process lacks the parameter code, the web service returns null.
Applicable for the Execute() method.
The variable of the incoming process parameter code. If you pass multiple incoming parameters, combine them using the & character.
Applicable for the Execute() method.
The values of the incoming process parameters.
Applicable for the ExecProcElByUId() method.
The ID of the process element to run.
Response body
The object collection.
The collection object instances.
The name of the Id field.
The IDs of the collection object instances.
The names of the field1, field2, ... fields in the object1, object2, ... collection object instances.
The values of the field1, field2, ... fields in the object1, object2, ... collection object instances. Available only for GET and POST requests.