Add an action to the record page
The example is relevant to Sales Creatio products.
Add a Show execution date action to the order page. The action must bring up a notification box that contains the scheduled order execution date. The action must be active for orders at the In progress stage.
1. Create a replacing view model schema of the order page
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the following schema properties.
- Set Code to "OrderPageV2."
- Set Title to "Order edit page."
- Set Parent object to "OrderPageV2."
-
Add a localizable string that contains the menu item caption.
-
Implement the menu item logic.
To do this, implement the following methods in the
methods
property:isRunning()
– checks whether the order is at the In progress stage and the menu item is available.showOrderInfo()
– the action handler method. Displays the scheduled order execution date in the notification box. The record page action applies to a specific object opened on the page. To access the field values of the record page object in the action handler method, use theget()
(retrieve the value) andset()
(assign the value) view model methods.getActions()
– an overridden base method. Returns the action collection of the replacing page.
View the source code of the order page’s replacing view model below.
OrderPageV2define("OrderPageV2", ["OrderConfigurationConstants"], function(OrderConfigurationConstants) {
return {
/* The name of the record page object’s schema. */
entitySchemaName: "Order",
/* The methods of the record page’s view model. */
methods: {
/* Check the order stage to determine the menu item availability. */
isRunning: function() {
/* Return true if the order status is [In progress], return false otherwise. */
if (this.get("Status")) {
return this.get("Status").value === OrderConfigurationConstants.Order.OrderStatus.Running;
}
return false;
},
/* The action handler method. Displays the order execution date in the notification box. */
showOrderInfo: function() {
/* Retrieve the order execution date. */
var dueDate = this.get("DueDate");
/* Display the notification box. */
this.showInformationDialog(dueDate);
},
/* Override the base virtual method that returns the action collection of the record page. */
getActions: function() {
/* Call the parent method implementation to retrieve the collection of the base page’s initialized actions. */
var actionMenuItems = this.callParent(arguments);
/* Add a separator line. */
actionMenuItems.addItem(this.getButtonMenuItem({
Type: "Terrasoft.MenuSeparator",
Caption: ""
}));
/* Add a menu item to the action list of the record page. */
actionMenuItems.addItem(this.getButtonMenuItem({
/* Bind the menu item caption to the localizable schema string. */
"Caption": {bindTo: "Resources.Strings.InfoActionCaption"},
/* Bind the action handler method. */
"Tag": "showOrderInfo",
/* Bind the property of the menu item availability to the value returned by the isRunning() method. */
"Enabled": {bindTo: "isRunning"}
}));
return actionMenuItems;
}
}
};
}); -
Click Save on the Designer’s toolbar.
As a result, Creatio will add the Show execution date action to the page of the order at the In progress stage.
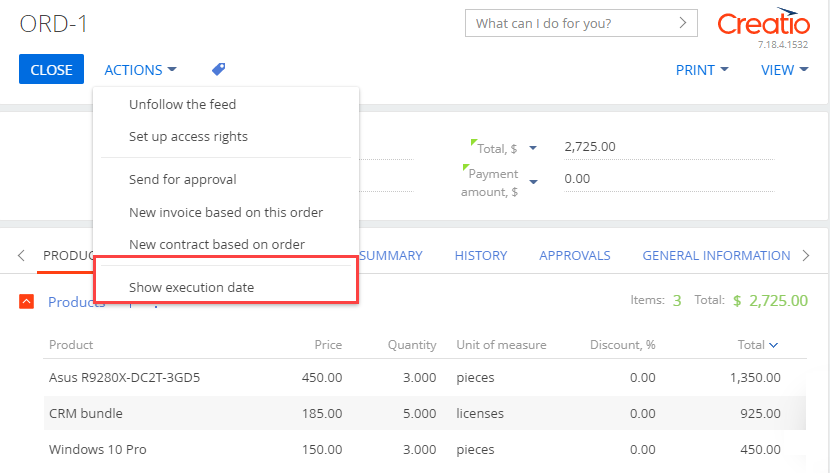
Creatio does not display the custom page action in the vertical list view.
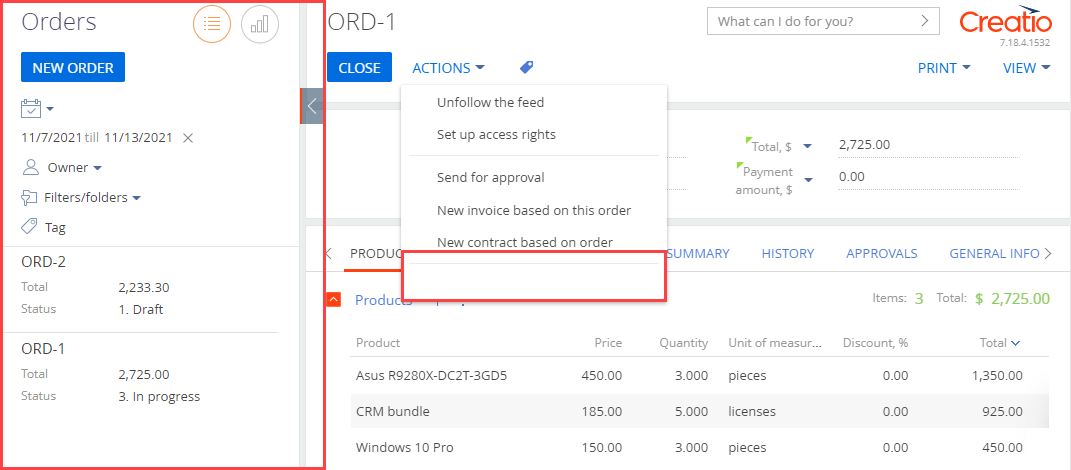
To ensure the page action is displayed correctly, add the following to the replacing view model schema of the section:
- The localizable string that contains the menu item caption.
- The method that determines the menu item availability.
2. Create a replacing view model schema of the section
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Replacing view model on the section list toolbar.
-
Fill out the following schema properties.
- Set Code to "OrderSectionV2."
- Set Title to "Order section."
- Set Parent object to "OrderSectionV2."
-
Add a localizable string that contains the menu item caption. To do this, take step 4 of the procedure to create a replacing view model schema of the order page.
-
Implement the menu item logic. To do this, implement the
isRunning()
method in themethods
property. The method checks if the order is at In progress stage and determines the menu item availability.View the source code of the section page’s replacing view model schema below.
OrderSectionV2define("OrderSectionV2", ["OrderConfigurationConstants"], function(OrderConfigurationConstants) {
return {
/* The name of the section page schema. */
entitySchemaName: "Order",
/* The methods of the section page’s view model. */
methods: {
/* Check the order stage to determine the menu item availability. */
isRunning: function(activeRowId) {
activeRowId = this.get("ActiveRow");
/* Retrieve the data collection of the section list’s list view. */
var gridData = this.get("GridData");
/* Retrieve the order model by the specified primary column value. */
var selectedOrder = gridData.get(activeRowId);
/* Retrieve the properties of the order status model. */
var selectedOrderStatus = selectedOrder.get("Status");
/* Return true if the order status is [In progress], return false otherwise. */
return selectedOrderStatus.value === OrderConfigurationConstants.Order.OrderStatus.Running;
}
}
};
}); -
Click Save on the Designer’s toolbar.
Outcome of the example
To view the outcome of the example:
- Clear the browser cache.
- Refresh the Orders section page.
As a result, Creatio will add the Show execution date action to the order page.
If the order is at the In progress stage, the Show execution date action will be active.
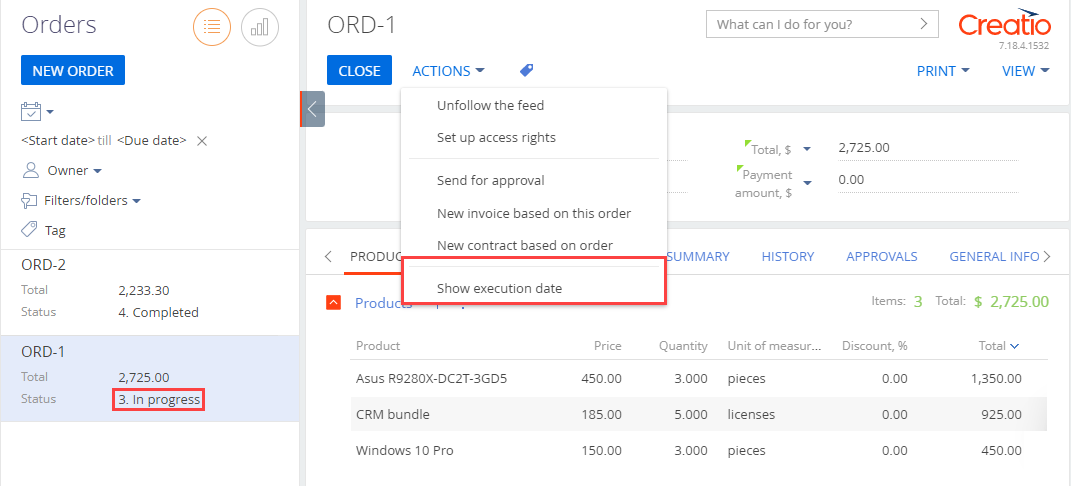
Run the Show execution date action to bring up the notification box that displays the scheduled order execution date.
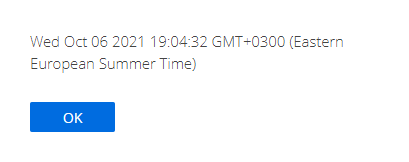
If the order is not at the In progress stage, the Show execution date action will be inactive.
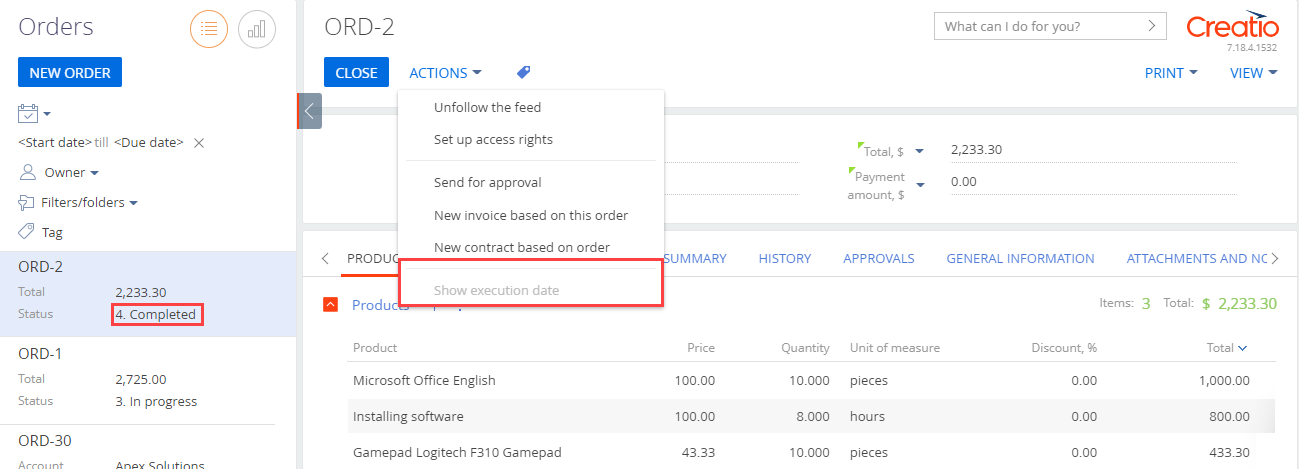