Integrate the cell connection provider with Creatio for two-factor authentication via SMS
Two-factor authentication (2FA) functionality lets you secure your identity by adding a verification code sent via email/SMS or generated in an authenticator app during various actions in Creatio, most importantly when you log in or change your password. 2FA also lets you recover your password on your own. Learn more: Set up two-factor authentication, Use two-factor authentication (user documentation).
2FA via SMS sends the verification code to the phone specified in the Phone field of the system user via SMS. The method requires an additional integration with the cell connection provider. Before you set up 2FA via SMS, integrate the cell connection provider with Creatio.
Set up a cell connection provider
1. Add a cell connection provider to Creatio
-
Open the Configuration section. Instructions: Open the Configuration section.
-
Create a user-made package. Instructions: Create a user-made package using Configuration section.
-
Change the current package. Instructions: Change the current package.
-
Open the Lookups section. To do this, click
in the top right → System setup → Lookups.
-
Open the SMS providers lookup.
-
Add the lookup value. To do this, click New.
-
Fill out the properties of the lookup value.
View the example that adds the "Some SMS provider" cell connection provider below.
Property
Property value
Name
Some SMS provider
Code
UsrSomeSmsProvider
-
Save the changes.
As a result, the cell connection provider will be added to Creatio.
2. Implement the business logic that executes 2FA via SMS using the cell connection provider
-
Create the source code schema in a user-made package. To do this, click Add → Source code.
-
Fill out the schema properties. Specify the schema name (the Code property) based on the following template:
[DeveloperPrefix][NameOfSomeCellConnectionProvider]SmsSender
. For example,UsrCellcomSmsSender
. -
Apply the changes.
-
Create a service class.
- Add the
Terrasoft.Configuration
namespace. - Add the namespaces the data types of which to utilize in the class using the
using
directive. - Add a class name that matches the schema name (the Code property).
- Specify that the class implements the
ISmsSender
interface. - Add the
[DefaultBinding(typeof(ISmsSender), Name = nameof(UsrSomeSmsProvider))]
attribute to the class, whereUsrSomeSmsProvider
is the schema name (the Code property). - Add the
public string Code => nameof(UsrSomeProviderSmsSender);
property, whereUsrSomeProviderSmsSender
is the value of Code property specified in the SMS providers lookup.
UsrSomeProviderSmsSendernamespace Terrasoft.Configuration
{
using Common.Logging;
using Terrasoft.Common;
using Terrasoft.Common.Threading;
using Terrasoft.Core;
using Terrasoft.Core.Factories;
using Terrasoft.SmsIntegration;
#region Class: UsrSomeProviderSmsSender
[DefaultBinding(typeof(ISmsSender), Name = nameof(UsrSomeProviderSmsSender))]
public class UsrSomeProviderSmsSender : ISmsSender
{
...;
#region Properties: Public
public string Code => "UsrSomeSmsProvider";
#endregion
...
}
#endregion
} - Add the
-
Implement a class method.
-
Add the
public void Send(string phoneNumber, string message);
method that executes 2FA via SMS receives the following input parameters.Parameter
Parameter description
phoneNumber
User phone number specified in the
→ Users and administration → Users section → Business phone column.
message
One-time 6-digit verification code generated by a user phone.
If you use the API provided by the cell connection provider to send SMS:
- Store the API configuration settings in the app system settings. For example, service URL, sender name, sender phone number.
- Use the system setting values that store the API configuration settings in the class that implements the
ISmsSender
interface. - Send a request to the service URL provided by the cell connection provider using the
IHttpRequestClient
interface. - Implement handling, logging, and discarding exceptions that occur while sending the SMS (including failed delivery attempts) in subsequent message sends.
-
Add a localizable string that stores the error message if error occurs during the execution of 2FA via SMS. Instructions: Add a localizable string.
-
Implement asynchronous execution of the
Send()
method, if needed. To do this, use theAsyncPump()
method.
UsrSomeProviderSmsSendernamespace Terrasoft.Configuration
{
using System;
using global::Common.Logging;
using Terrasoft.Common;
using Terrasoft.Common.Threading;
using Terrasoft.Core;
using Terrasoft.Core.Factories;
using Terrasoft.Core.Requests;
using Terrasoft.SmsIntegration;
#region Class: UsrSomeProviderSmsSender
[DefaultBinding(typeof(ISmsSender), Name = nameof(UsrSomeProviderSmsSender))]
public class UsrSomeProviderSmsSender : ISmsSender
{
#region Fields: Private
private readonly ILog _logger = LogManager.GetLogger("UsrSomeProviderSms");
private readonly IHttpRequestClient _httpRequestClient;
private readonly UserConnection _userConnection;
#endregion
#region Constructors: Public
public UsrSomeProviderSmsSender(AppConnection appConnection, IHttpRequestClient httpRequestClient) {
appConnection.CheckArgumentNull(nameof(appConnection));
httpRequestClient.CheckArgumentNull(nameof(httpRequestClient));
_userConnection = appConnection?.SystemUserConnection;
_httpRequestClient = httpRequestClient;
}
#endregion
#region Properties: Public
public string Code => "UsrSomeSmsProvider";
#endregion
#region Methods: Public
/* Execute 2FA via SMS using user phone number and verification code. */
public void Send(string phoneNumber, string message) {
/* Receive some options for SMS sending from the system settings. */
var serviceUrl = Terrasoft.Core.Configuration.SysSettings.GetValue<string>(_userConnection, "SomeSystemSettingCode", string.Empty);
try {
/* Send the SMS to the phone number. */
IHttpResponse response = _httpRequestClient.SendWithJsonBody(new HttpRequestConfig() {
/* Implement the business logic. */
});
if (response.IsSuccessStatusCode) {
_logger.Info($"2FA via SMS log. Sends the following message: {message} to the recipient {phoneNumber}.");
return;
}
throw new Exception($"2FA via SMS log. SMS was not sent to the recipient {phoneNumber} due to the error:"
+ $"{response.ReasonPhrase}", response.Exception);
/* Send SMS asynchronously if needed. */
AsyncPump.Run(() => {
_logger.Info($"2FA via SMS log. Sends the following message: {message} to the recipient {phoneNumber}.");
});
} catch {
string codeOfLocalizableString = "CodeOfSomeLocalizableString";
/* Display the error message if error occurs during the execution of 2FA via SMS. */
string errorMessage = new LocalizableString(_userConnection.Workspace.ResourceStorage,
"UsrSomeProviderSmsSender", $"LocalizableStrings.{codeOfLocalizableString}.Value");
throw new InvalidOperationException(errorMessage, null);
}
}
#endregion
}
#endregion
} -
-
Publish the schema.
As a result, Creatio will be able to execute 2FA via SMS using the cell connection provider.
3. Select primary cell connection provider
-
Open the System settings section. To do this, click
→ System setup → System settings.
-
Open the SMS provider (
SmsProvider
code) system setting. -
Fill out the system setting properties.
Property
Property value
Default value
Select a cell connection provider. For this example, "Some SMS provider."
-
Save the changes.
-
Open the SMS sender name (
SmsSenderName
code) system setting. -
Fill out the system setting properties.
Property
Property value
Default value
An arbitrary name of the SMS sender.
-
Save the changes.
As a result, the cell connection provider will be configured.
To use 2FA via SMS as the main 2FA method, follow the instructions: Set up 2FA via SMS (user documentation).
Bind data of a cell connection provider to a package
Bind data of a cell connection provider to a package if you need to transfer the configured cell connection provider between Creatio instances.
1. Bind SMS providers lookup content
-
Create a Data schema type in a user-made package. To do this, click Add → Data.
-
Fill out the schema properties.
For this example, use the schema properties as follows.
Property
Property value
Object
SysSmsProvider
-
Select data to bind.
- Open the Columns setting tab.
- Select the Caption checkbox.
- Open the Bound data tab.
- Click Add. This opens the Select: SMS providers window.
- Select the required checkbox. For example, Some SMS provider.
- Click Select.
-
Save the changes.
As a result, the needed record of the SMS providers lookup will be bound to the package.
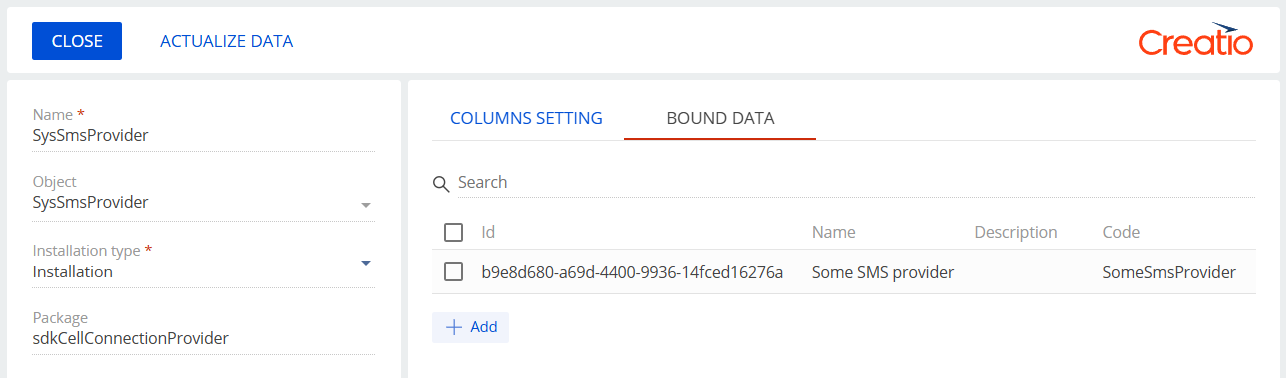
2. Bind SMS provider system setting
-
Bind code of the system setting.
-
Create a Data schema type in a user-made package. To do this, click Add → Data.
-
Fill out the schema properties.
Property
Property value
Object
SysSettings
-
Select data to bind.
- Open the Columns setting tab.
- Select the Caption checkbox.
- Open the Bound data tab.
- Click Add. This opens the Select: System setting window.
- Select the SMS provider checkbox.
- Click Select.
-
Save the changes.
As a result, a code of the SMS provider system setting will be bound to the package.
-
-
Bind value of the system setting.
-
Create a Data schema type in a user-made package. To do this, click Add → Data.
-
Fill out the schema properties.
Property
Property value
Object
SysSettingsValue
-
Select data to bind.
- Open the Columns setting tab.
- Select the Caption checkbox.
- Open the Bound data tab.
- Click Add. This opens the Select: System setting value window.
- Select the SMS provider checkbox.
- Click Select.
-
Save the changes.
As a result, a value of the SMS provider (
SmsProvider
code) system setting will be bound to the package. -
3. Bind SMS sender name system setting
-
Bind code of the system setting.
-
Create a Data schema type in a user-made package. To do this, click Add → Data.
-
Fill out the schema properties.
Property
Property value
Object
SysSettings
-
Select data to bind.
- Open the Columns setting tab.
- Select the Caption checkbox.
- Open the Bound data tab.
- Click Add. This opens the Select: System setting window.
- Select the SMS sender name checkbox.
- Click Select.
-
Save the changes.
As a result, a code of the SMS sender name system setting will be bound to the package.
-
-
Bind value of the system setting.
-
Create a Data schema type in a user-made package. To do this, click Add → Data.
-
Fill out the schema properties.
Property
Property value
Object
SysSettingsValue
-
Select data to bind.
- Open the Columns setting tab.
- Select the Caption checkbox.
- Open the Bound data tab.
- Click Add. This opens the Select: System setting value window.
- Select the SMS sender name checkbox.
- Click Select.
-
Save the changes.
As a result, a value of the SMS sender name (
SmsSenderName
code) system setting will be bound to the package. -
-
Bind system settings and system setting values that store the API configuration settings if needed.
See also
Set up two-factor authentication (user documentation)
Use two-factor authentication (user documentation)