Run the business process from the browser address bar using the business process service
To implement the example:
- Implement a business process that adds a contact. Read more >>>
- Implement a business process that retrieves the list of contacts. Read more >>>
Run the following custom business processes from the browser address bar using the ProcessEngineService.svc
business process service:
1. Implement a business process that adds a contact
1. Create a business process
-
Open the Configuration section. Instructions: Open the Configuration section.
-
Create a package. Instructions: Create a user-made package using Configuration section.
For this example, create the
sdkWorkWithBusinessProcessService
package. -
Change the current package. Instructions: Change the current package.
For this example, change the current package to
sdkWorkWithBusinessProcessService
user-made package. -
Create the business process schema. To do this, click Add → Business process.
-
Open the Settings tab.
-
Fill out the schema properties.
For this example, use the schema properties as follows.
Property
Property value
Title
Add external contact
Code
UsrAddExternalContactProcess
-
Save the changes.
As a result:
- The "Add external contact" business process will be created.
- Creatio will add the "Add external contact" business process to the Process library section.
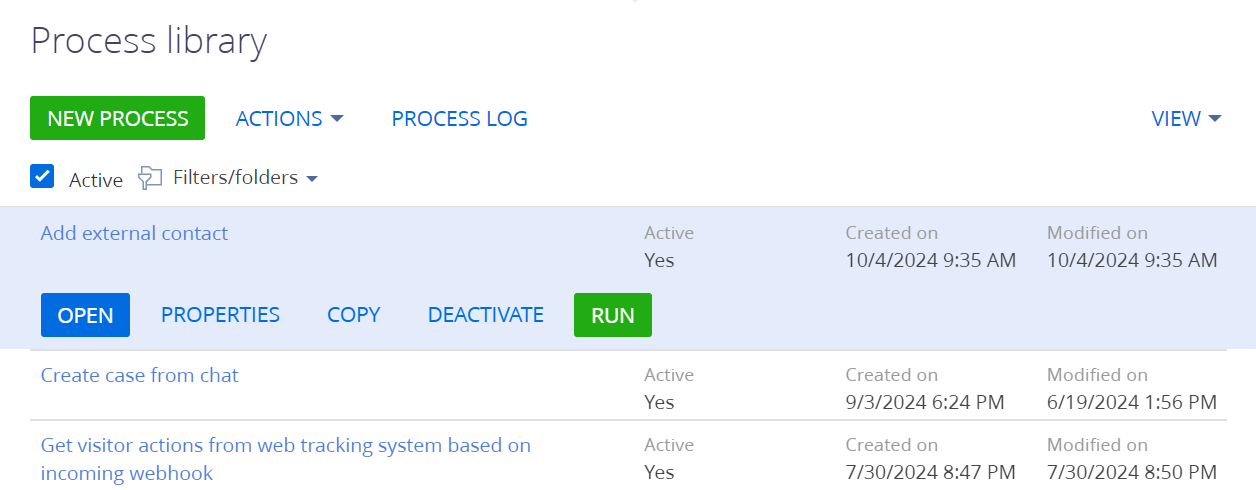
2. Set up the business process parameters
-
Open the properties of the business process schema. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Parameters tab.
-
Add the business process parameters.
For this example, add the following parameters:
- parameter that contains the contact name
- parameter that contains the mobile phone of the contact
To do this:
-
Click Add parameter and select a parameter of the needed type.
-
Fill out the parameter properties.
Element
Element type
Property
Property value
Parameter that contains the contact name
Text
Title
Contact name
Code
ContactName
Data type
Text (50 characters)
Parameter that contains the mobile phone of the contact
Text
Title
Mobile phone
Code
MobilePhone
Data type
Text (50 characters)
As a result, the Parameters tab of the "Add external contact" business process will be as follows.
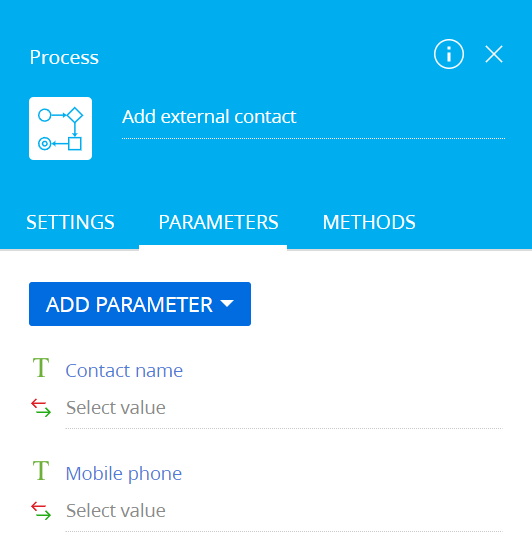
3. Implement adding a contact
-
Add a Script task element. To do this, click
→ place the Script task element between the Simple page element and Terminate page element in the working area of the Process Designer.
-
Fill out the element properties.
Property
Property value
Title
Add contact
-
Implement the logic of working with process parameters. To do this, go to the element setup area and add the source code.
Add contact script task// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an instance of the "Contact" object. */
var schema = UserConnection.EntitySchemaManager.GetInstanceByName("Contact");
/* Create an instance of new object. */
var entity = schema.CreateEntity(UserConnection);
/* Set object columns to default values. */
entity.SetDefColumnValues();
/* Contact name. */
string contactName = Get<string>("ContactName");
/* Mobile phone of the contact. */
string contactPhone = Get<string>("MobilePhone");
/* Set "Name" column to process parameter value. */
entity.SetColumnValue("Name", contactName);
/* Set "MobilePhone" column to process parameter value. */
entity.SetColumnValue("MobilePhone", contactPhone);
/* Save added contact. */
entity.Save();
return true; -
Publish the changes.
As a result, the diagram of the "Add external contact" business process will be as follows.
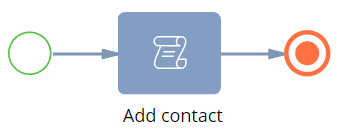
2. Implement a business process that retrieves the list of contacts
1. Create a business process
-
Select a user-made package to add the schema.
For this example, select the
sdkWorkWithBusinessProcessService
user-made package. -
Create the business process schema. To do this, click Add → Business process.
-
Open the Settings tab.
-
Fill out the schema properties.
For this example, use the schema properties as follows.
Property
Property value
Title
Retrieve the list of contacts
Code
UsrRetrieveContactListProcess
-
Save the changes.
As a result:
- The "Retrieve the list of contacts" business process will be created.
- Creatio will add the "Retrieve the list of contacts" business process to the Process library section.
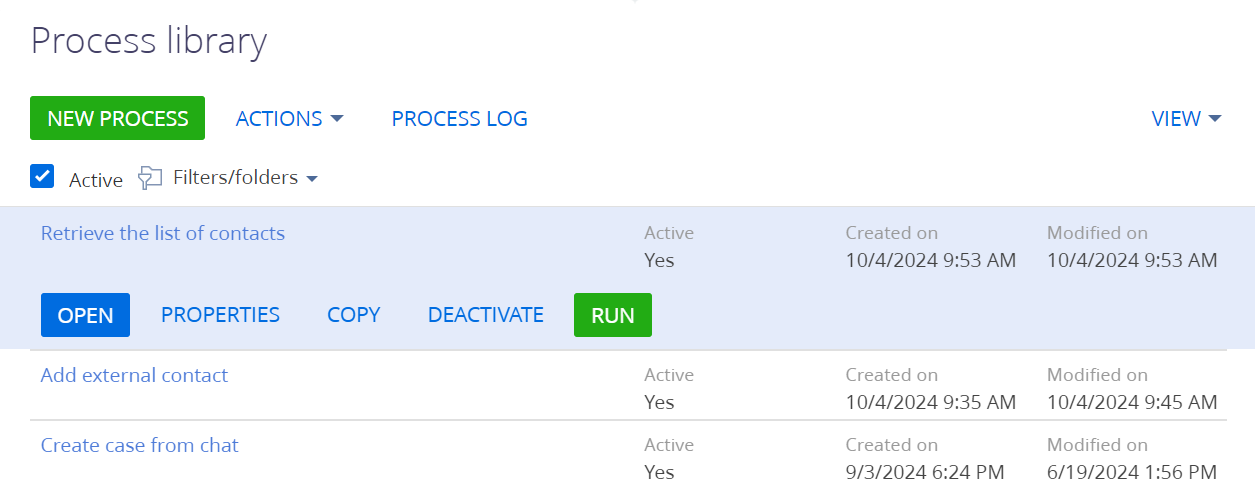
2. Set up the business process parameters
-
Open the properties of the business process schema. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Parameters tab.
-
Add the business process parameters.
For this example, add the parameter that contains the list of contacts. To do this:
-
Click Add parameter and select a parameter of the needed type.
-
Fill out the parameter properties.
Element
Element type
Property
Property value
Parameter that contains the list of contacts
Text
Title
List of contacts
Code
ContactList
Data type
Unlimited length text
-
As a result, the Parameters tab of the "Retrieve the list of contacts" business process will be as follows.
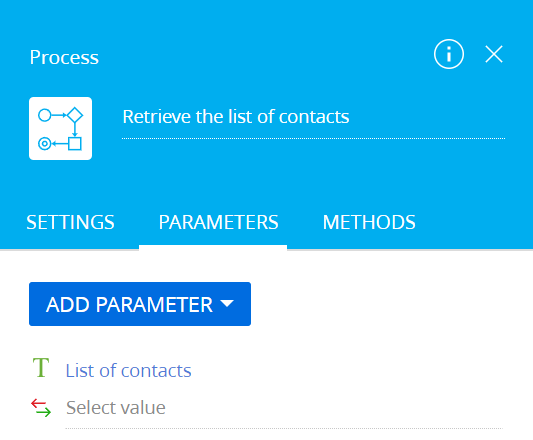
3. Implement retrieving the list of contacts
-
Add a Script task element. To do this, click
→ place the Script task element between the Simple page element and Terminate page element in the working area of the Process Designer.
-
Fill out the element properties.
Property
Property value
Title
Retrieve contacts
-
Implement the logic of working with process parameters. To do this, go to the element setup area and add the source code.
Retrieve contacts script task// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an "EntitySchemaQuery" instance. */
EntitySchemaQuery query = new EntitySchemaQuery(UserConnection.EntitySchemaManager, "Contact");
/* Flag the "Id" primary column as required to select. */
query.PrimaryQueryColumn.IsAlwaysSelect = true;
/* Add columns to the query. */
query.AddColumn("Name");
query.AddColumn("MobilePhone");
/* Retrieve an entity collection. */
var entities = query.GetEntityCollection(UserConnection);
/* Create the list of contacts to serialize in JSON. */
List<object> contacts = new List<object>();
foreach (var item in entities)
{
var contact = new
{
Id = item.GetTypedColumnValue<Guid>("Id"),
Name = item.GetTypedColumnValue<string>("Name"),
MobilePhone = item.GetTypedColumnValue<string>("MobilePhone")
};
contacts.Add(contact);
}
/* Save the contact collection that is serialized in JSON to the "ContactList" parameter. */
string contactList = JsonConvert.SerializeObject(contacts);
Set<string>("ContactList", contactList);
return true;
4. Set up the business process methods
-
Open the properties of the business process. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Methods tab.
-
Add the business process methods.
-
Click
and fill out the method properties.
Property
Property value
Namespace
Newtonsoft.Json
-
Click Save.
-
-
Publish the changes.
As a result:
-
The Methods tab of the "Retrieve the list of contacts" business process will be as follows.
-
The diagram of the "Retrieve the list of contacts" business process will be as follows.
View the result
To view the outcome of the example that adds a contact:
-
Access the
UsrAddExternalContactProcess
endpoint of theProcessEngineService.svc
service from the browser address bar. -
Pass the contact name in the
ContactName
parameter. For example, "James Bennett." -
Pass the mobile phone of the contact in the
MobilePhone
parameter. For example, "1 111 111 1111."Request stringCreatioURL/0/ServiceModel/ProcessEngineService.svc/UsrAddExternalContactProcess/Execute?ContactName=James%20Bennett&MobilePhone=1%20111%20111%201111
-
Click Enter.
As a result:
-
ProcessEngineService.svc
service will run an "Add external contact" business process. -
The "Add external contact" business process will add the contact whose fields are populated using the specified values to the Contacts section. View the result >>>
To view the outcome of the example that retrieves the list of contacts:
-
Access the
UsrRetrieveContactListProcess
endpoint of theProcessEngineService.svc
service from the browser address bar. -
Pass the
ContactList
parameter as a value of theResultParameterName
parameter.Request stringCreatioURL/0/ServiceModel/ProcessEngineService.svc/UsrRetrieveContactListProcess/Execute?ResultParameterName=ContactList
-
Click Enter.
As a result:
-
ProcessEngineService.svc
service will run a "Retrieve the list of contacts" business process. -
The "Retrieve the list of contacts" business process will retrieve the list of contacts from the Contacts section. View the result >>>
Source code
- Add contact
- Retrieve contacts
// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an instance of the "Contact" object. */
var schema = UserConnection.EntitySchemaManager.GetInstanceByName("Contact");
/* Create an instance of new object. */
var entity = schema.CreateEntity(UserConnection);
/* Set object columns to default values. */
entity.SetDefColumnValues();
/* Contact name. */
string contactName = Get<string>("ContactName");
/* Mobile phone of the contact. */
string contactPhone = Get<string>("MobilePhone");
/* Set "Name" column to process parameter value. */
entity.SetColumnValue("Name", contactName);
/* Set "MobilePhone" column to process parameter value. */
entity.SetColumnValue("MobilePhone", contactPhone);
/* Save added contact. */
entity.Save();
return true;
// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an "EntitySchemaQuery" instance. */
EntitySchemaQuery query = new EntitySchemaQuery(UserConnection.EntitySchemaManager, "Contact");
/* Flag the "Id" primary column as required to select. */
query.PrimaryQueryColumn.IsAlwaysSelect = true;
/* Add columns to the query. */
query.AddColumn("Name");
query.AddColumn("MobilePhone");
/* Retrieve an entity collection. */
var entities = query.GetEntityCollection(UserConnection);
/* Create the list of contacts to serialize in JSON. */
List<object> contacts = new List<object>();
foreach (var item in entities)
{
var contact = new
{
Id = item.GetTypedColumnValue<Guid>("Id"),
Name = item.GetTypedColumnValue<string>("Name"),
MobilePhone = item.GetTypedColumnValue<string>("MobilePhone")
};
contacts.Add(contact);
}
/* Save the contact collection that is serialized in JSON to the "ContactList" parameter. */
string contactList = JsonConvert.SerializeObject(contacts);
Set<string>("ContactList", contactList);
return true;