Authenticate external requests to Creatio from the C# console app
To implement the example:
- Create a C# console app. Read more >>>
- Implement the business logic of the console app. Read more >>>
1. Create a C# console app
-
Open an external IDE.
For this example, open Microsoft Visual Studio.
-
Create a project.
-
Go to the Get started block.
-
Click Create a new project. This opens the Create a new project window.
-
Select Console App (.NET Framework).
-
Click Next. This opens the Configure your new project window.
-
Fill out the project properties.
For this example, use the project properties as follows.
Property
Property value
Project name
AuthenticateExternalRequests
Framework
.NET Framework 4.7.2
Fill out other project properties based on your business goals.
-
Click Create.
-
As a result:
- The "AuthenticateExternalRequests" project of console app will be created.
- Microsoft Visual Studio will open the "AuthenticateExternalRequests" project of the console app.
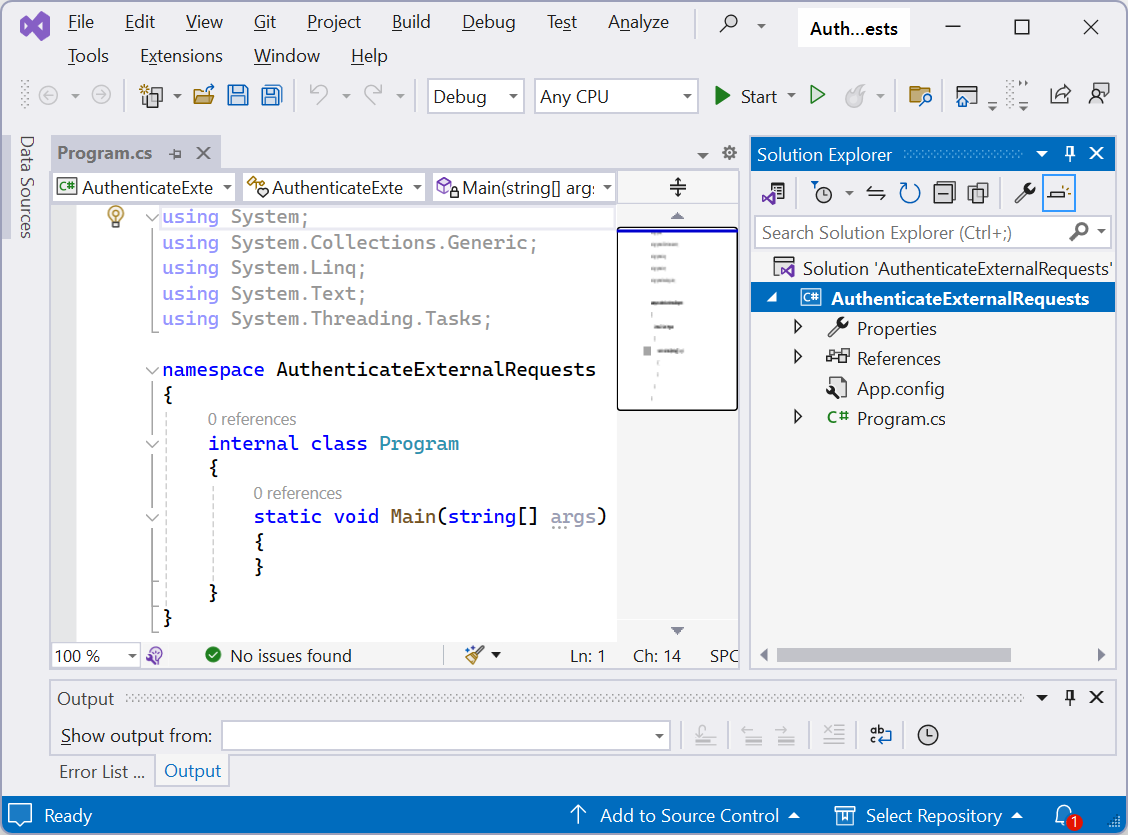
2. Implement the business logic of the console app
- Open the
Program.cs
file. - Implement the configuration object of the
AuthService.svc
service response body. - Specify data to implement the authentication of external requests.
- Implement the method that authenticates external requests.
- Authenticate external requests using user credentials.
- Save the changes.
using System;
using System.IO;
using System.Net;
namespace AuthenticateExternalRequests
{
/// <summary>
/// Configuration object of the "AuthService.svc" service response body.
/// </summary>
class ResponseStatus
{
public int Code { get; set; }
public string Message { get; set; }
public object Exception { get; set; }
public object PasswordChangeUrl { get; set; }
public object RedirectUrl { get; set; }
}
/// <summary>
/// Main program class.
/// </summary>
internal class Program
{
/* URL of Creatio instance that has the corresponding business processes implemented. */
private const string baseUri = "https://mycreatio.com";
/* URL of "AuthService.svc" service. */
private const string authServiceUri = baseUri + @"/ServiceModel/AuthService.svc/Login";
private static ResponseStatus status = null;
public static CookieContainer AuthCookie = new CookieContainer();
/// <summary>
/// Method that authenticates external requests using user credentials.
/// </summary>
/// <param name="userName">Login that user uses to log in to Creatio.</param>
/// <param name="userPassword">Password that user uses to log in to Creatio.</param>
/// <returns>If the request is authenticated, returns "true." Otherwise, "false."</returns>
public static bool TryLogin(string userName, string userPassword)
{
var authRequest = HttpWebRequest.Create(authServiceUri) as HttpWebRequest;
/* Request method. */
authRequest.Method = "POST";
/* Request header. */
authRequest.ContentType = "application/json";
authRequest.CookieContainer = AuthCookie;
using (var requestStream = authRequest.GetRequestStream())
{
using (var writer = new StreamWriter(requestStream))
{
writer.Write(@"{
""UserName"":""" + userName + @""",
""UserPassword"":""" + userPassword + @"""
}");
}
}
using (var response = (HttpWebResponse)authRequest.GetResponse())
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
string responseText = reader.ReadToEnd();
status = new System.Web.Script.Serialization.JavaScriptSerializer().Deserialize<ResponseStatus>(responseText);
}
}
if (status != null)
{
/* If the code contains a "0" value, the authentication is successful. Otherwise, it is failed. */
if (status.Code == 0)
{
return true;
}
Console.WriteLine(status.Message);
}
return false;
}
static void Main(string[] args)
{
/* Authenticate external requests using user credentials. */
f (!TryLogin("SomeCreatioLogin", "SomeCreatioPassword"))
{
Console.WriteLine("Wrong login or password. Application will be terminated.");
}
else
{
Console.WriteLine("The authentication is successful. External requests to Creatio will be authenticated.");
};
Console.WriteLine("Press ENTER to exit...");
Console.ReadLine();
}
}
}
As a result, the "AuthenticateExternalRequests" project of console app will be implemented.
View the result
To view the outcome of the example, run the project. To do this, click Start.
As a result, external requests to Creatio implemented using C# console app will be authenticated. View the result >>>
Source code
using System;
using System.IO;
using System.Net;
namespace AuthenticateExternalRequests
{
/// <summary>
/// Configuration object of the "AuthService.svc" service response body.
/// </summary>
class ResponseStatus
{
public int Code { get; set; }
public string Message { get; set; }
public object Exception { get; set; }
public object PasswordChangeUrl { get; set; }
public object RedirectUrl { get; set; }
}
/// <summary>
/// Main program class.
/// </summary>
internal class Program
{
/* URL of Creatio instance that has the corresponding business processes implemented. */
private const string baseUri = "https://mycreatio.com";
/* URL of "AuthService.svc" service. */
private const string authServiceUri = baseUri + @"/ServiceModel/AuthService.svc/Login";
private static ResponseStatus status = null;
public static CookieContainer AuthCookie = new CookieContainer();
/// <summary>
/// Method that authenticates external requests using user credentials.
/// </summary>
/// <param name="userName">Login that user uses to log in to Creatio.</param>
/// <param name="userPassword">Password that user uses to log in to Creatio.</param>
/// <returns>If the request is authenticated, returns "true." Otherwise, "false."</returns>
public static bool TryLogin(string userName, string userPassword)
{
var authRequest = HttpWebRequest.Create(authServiceUri) as HttpWebRequest;
/* Request method. */
authRequest.Method = "POST";
/* Request header. */
authRequest.ContentType = "application/json";
authRequest.CookieContainer = AuthCookie;
using (var requestStream = authRequest.GetRequestStream())
{
using (var writer = new StreamWriter(requestStream))
{
writer.Write(@"{
""UserName"":""" + userName + @""",
""UserPassword"":""" + userPassword + @"""
}");
}
}
using (var response = (HttpWebResponse)authRequest.GetResponse())
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
string responseText = reader.ReadToEnd();
status = new System.Web.Script.Serialization.JavaScriptSerializer().Deserialize<ResponseStatus>(responseText);
}
}
if (status != null)
{
/* If the code contains a "0" value, the authentication is successful. Otherwise, it is failed. */
if (status.Code == 0)
{
return true;
}
Console.WriteLine(status.Message);
}
return false;
}
static void Main(string[] args)
{
/* Authenticate external requests using user credentials. */
f (!TryLogin("SomeCreatioLogin", "SomeCreatioPassword"))
{
Console.WriteLine("Wrong login or password. Application will be terminated.");
}
else
{
Console.WriteLine("The authentication is successful. External requests to Creatio will be authenticated.");
};
Console.WriteLine("Press ENTER to exit...");
Console.ReadLine();
}
}
}