On the previous step, we implemented population of the group class timetable.
Now, implement a web service that provides information about the number of classes in the timetable. To call a web service, add the Web service button to the record page.
Create a custom web service
- Go to the Configuration section.
- Select the "TryItPackage" package from the package list.
-
Click Add on the workspace toolbar and select the Source code configuration element type.
- Fill out the property fields as follows:
- Set Code to "UsrClassService".
- Set Title to "Class service".
-
Add the source code in the Schema Designer.
UsrClassService.cs - Click Publish to save the schema.
Modify the page source code
Add a class page button that calls the web service and displays the dialog box that contains the number of group classes in the timetable.
- Go to the Configuration section.
- Select the "TryItPackage" package from the package list.
-
The Wizards added schemas of various types to the package. Filter schemas by the Client module type.
- Double-click the UsrClass1Page schema to open it.
-
Add a new localizable string for the button name to the schema.
Click the
button in the Localizable strings block of the properties panel and fill out the localizable string properties:
- Set Code to "ServiceButtonCaption".
- Set Value to "Web service".
-
Modify the source code.
UsrClass1Page.js - Click Save to save the schema.
As a result, we implemented the web service that returns the number of group classes.
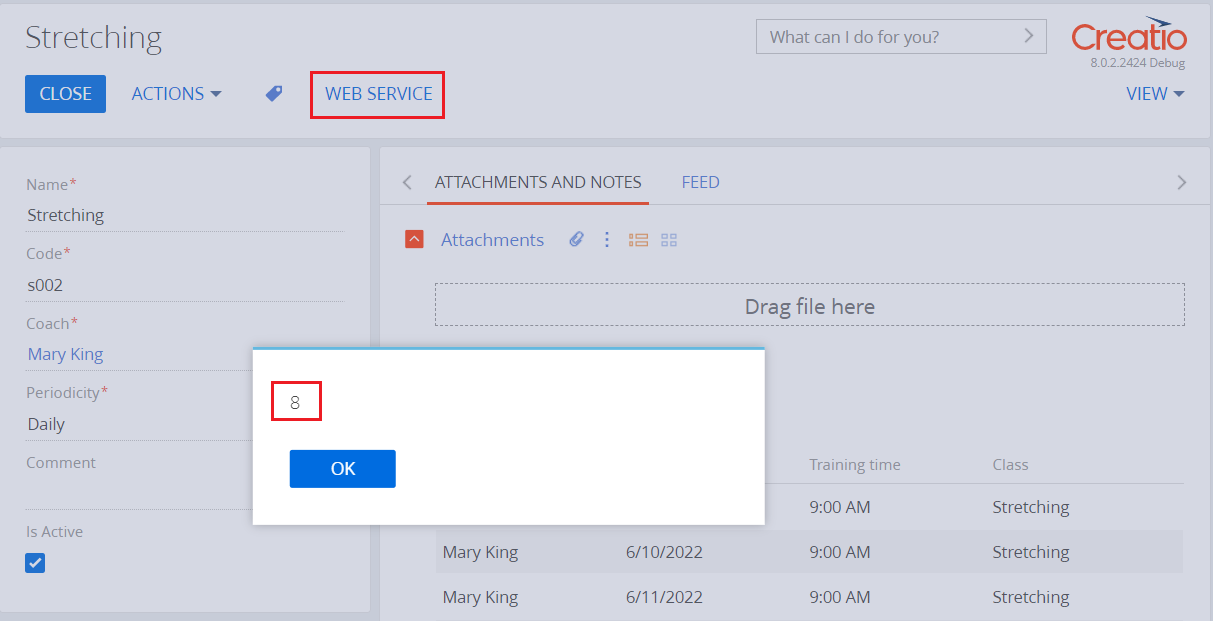