Declare a module class
Class declaration is a function of the ExtJS JavaScript framework. To declare a class, use the define() method of the global Ext object. This is the standard library mechanism.
View the examples that create class instances below.
Inherit from a module class
In most cases, you need to inherit the module class from the BaseModule or BaseSchemaModule classes of the Terrasoft.configuration namespace.
The BaseModule and BaseSchemaModule classes implement the following methods:
- init(). Implements the logic that is executed when loading the module. The client core calls this method first automatically when loading the module. The init() method usually subscribes to events of other modules and initializes the module values.
- render(renderTo). Implements the module visualization logic. The client core calls this method automatically when loading the module. To ensure data is displayed correctly, trigger the mechanism that binds the view (View) and view model (ViewModel) before data visualization. Usually, this mechanism is initiated in the render() method by calling the bind() method in the view object. If you load the module into a container, pass the link to the container to the render() method as an argument. The render() method is required for visual modules.
- destroy(). Responsible for deleting the module view, deleting the view model, unsubscribing from previously subscribed messages, and deleting the module class object.
View the example of a module class that inherits from the Terrasoft.BaseModule class below. The module adds a button to DOM. When you click the button, Creatio displays a message and deletes the button from DOM.
Overload module class members
When you inherit from a module class, you can overload public and private properties and methods of a base module in an inheritor class.
Private class properties or methods are properties or methods whose names start with an underscore character, for example, _privateMemberName.
The purpose of tracking is to check if overloads of private properties or methods declared in parent classes are executed when declaring a custom class. The browser console displays an overload warning in debug mode. Learn more in a separate article: Front-end debugging.
To track overloads of private members of a module class, use the Terrasoft.PrivateMemberWatcher class.
For example, a custom package includes the UsrPrivateMemberWatcher module schema.
After Creatio loads the UsrPrivateMemberWatcher module, the browser console will display a warning about overloading the private members of the base classes.
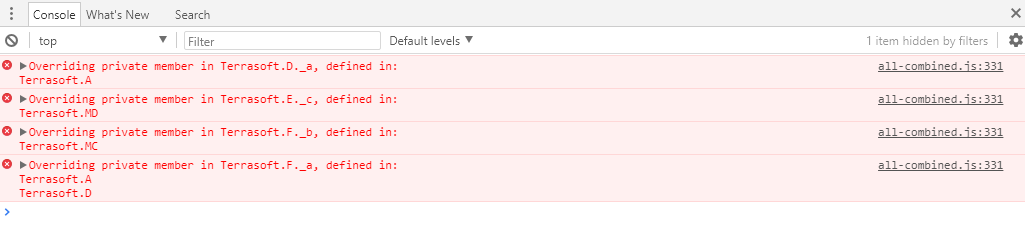
Initialize a module class instance
You can initialize a module class instance in the following ways:
- synchronous initialization
- asynchronous initialization
Initialize a module class instance synchronously
The module is initialized synchronously if the isAsync: true property of the configuration object that is passed as a parameter of the loadModule() method is not specified explicitly on load. For example, the module's class methods are loaded synchronously when the code below is executed.
Creatio calls the init() method first, then the render() method.
Initialize a module class instance asynchronously
The module is initialized asynchronously if the isAsync: true property of the configuration object that is passed as a parameter of the loadModule() method is specified explicitly on load. For example, the module class methods are loaded asynchronously when the code below is executed.
Creatio calls the init() method first. A callback function that has the scope of the current module is passed as a parameter of the init() method. When the callback function is called, Creatio executes the render() method. The view is added to DOM only after the render() method is executed.
Module chain
A module chain is a mechanism that lets you display a view of a model instead of a view of a different model. For example, to set the field value on the current page, display the SelectData page that enables users to select a lookup value. I. e., display the module view of the lookup selection page in place of the module container of the current page.
To create a chain, add the keepAlive property to the configuration object of the module to load.
View an example that calls the selectDataModule module from the CardModule current page module below. The CardModule module enables users to select a lookup value.
After the code is executed, Creatio generates a chain from the current page module and page module that lets you select a lookup value. If you add another element to the chain, users will be able to click the Add new record button to open a new page from the selectData current page module. You can add as many modules to a chain as needed.
An active module is the last chain element that is displayed on the page. If you use a module from the middle of a chain as the active module, Creatio deletes all elements after the active module from the chain. To activate a module in the chain, pass the module ID to the loadModule() function as a parameter.
The core will delete the elements of the chain, then call the init() and render() methods. The container that includes the previous active module is passed to the render() method. The presence of a module in a chain does not affect the module operability.
If you omit the keepAlive property or add it as keepAlive: false to a configuration object when calling the loadModule() method, Creatio deletes the module chain.