Introduction
Creatio enables implementing custom logic of receiving parameters for calculating case deadline. When calculating or recalculating a case deadline, a developer implemented strategy is used instead of one of the base calculation strategies.
You can select a specific calculation rule in the Case deadline calculation rules lookup. Follow these steps to add a new calculation rule:
1. Create an object schema and add columns necessary for storage of response and resolution deadlines, links to the calendar, service agreement and service.
2. Based on the created object schema, add a lookup and populate it with values needed to calculate the deadline parameters.
3. Add the source code schema and declare the class inherited from the BaseTermStrategy abstract class. Implement custom mechanism of receiving response and resolution deadline parameters in the class.
4. Add a new rule.
Case description
Add a custom rule for calculating case deadline parameters for the Lost data recovery service as per the 78 — Elite Systems agreement. Set the following values for the new rule:
- response time – 2 working hours
- resolution time – 1 working day
- used calendar – [Default calendar]
Source code of the case:
You can download the package with case implementation using the following link.
Case implementation algorithm
1. Creating an object schema containing the necessary columns for calculation
Perform the Add – Object action on the Schemas tab of the Configuration section.
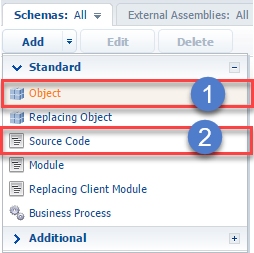
Set the following properties for the created object schema (Fig. 2):
- [Name] – “UsrServiceTestTerms”
- [Title] – “ServiceTestTerms”
- [Parent object] – the [Base object] schema
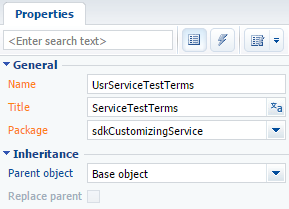
In the created schema, create a number of columns, whose primary properties are listed in table 1.
Name | Title | Type | Description |
---|---|---|---|
UsrReactionTimeUnit | Response time unit | The [Time unit] lookup | Specifies the time unit (calendar days, hours, etc.) that will be used for calculating the [Response time] parameter. |
UsrReactionTimeValue | Response time value | Integer | A column for storage the response time value. |
UsrSolutionTimeUnit | Response time unit | The [Time unit] lookup | Specifies the time unit (calendar days, hours, etc.) that will be used for calculating the [Response time] parameter. |
UsrSolutionTimeValue | Resolution time | Integer | A column for storage the response time value. |
UsrCalendarId | Calendar that is used | The [Calendar] lookup | The calendar used for calculating the case deadline. |
UsrServicePactId | Service agreement | The [Service agreement] lookup | Link to the [Service agreement] object. Added for enabling filtration. |
UsrServiceItemId | Service | The [Service] lookup | Link to the [Service] object. Added for enabling filtration. |
Publish the schema after adding the columns.
2. Adding a lookup and populating it with values needed to calculate the deadline parameters
Provide specific values to calculate the case response and resolution deadline. To do this, add a lookup with the following values based on the added schema (fig.3):
- [Name] – “Custom response and resolution deadlines”
- [Object] – ServiceTestTerms
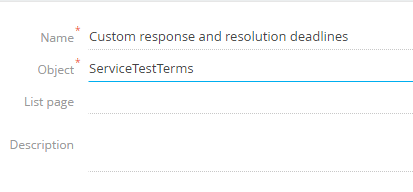
Add a record with the following data to the added lookup (as per the case conditions) (fig.4):
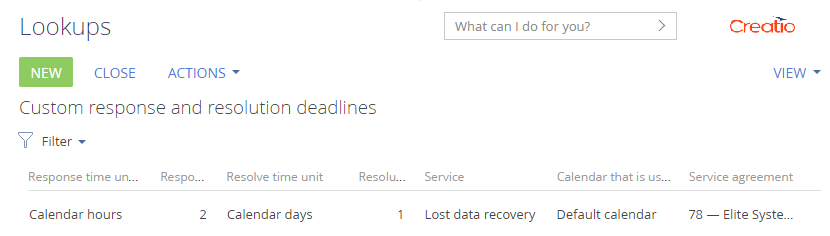
3. Implementing a class with the mechanism of receiving deadline parameters
Add the source code schema (fig.1, 2) Add the class inherited from the BaseTermStrategy abstract class (declared in the Calendar package) to the schema source code. Implement a parameterized constructor with the following parameters in the class:
- UserConnection userConnection – user current connection
- Dictionary
args – arguments that are the base of performing calculation
Implement the GetTermInterval() abstract method declared in the base class. This method accepts the mask of populated values as the incoming parameter, which is the base of taking a decision about populating the specific deadline parameters of the TermInterval returned class implementing the ITermInterval
The complete schema source code:
Publish the schema after adding the source code.
4. Adding the new rule
Add a value to the Case deadline calculation schemas lookup. In the Handler column, specify the full qualified name of the created class (specifying the namespaces).
In the Alternative schema column you may specify the rule for calculating the deadline in case calculation by current rule is not possible. Take into considerations that if any of deadline parameters is not calculated by strategy class, a class instance of an alternative strategy will be created. In case the alternative strategy cannot calculate the deadline either, another alternative strategy will be created, thus forming a rule queue.
Select the Default checkbox for the added record.
See an example of an added record to the Case deadline calculation schemas lookup in fig.5.
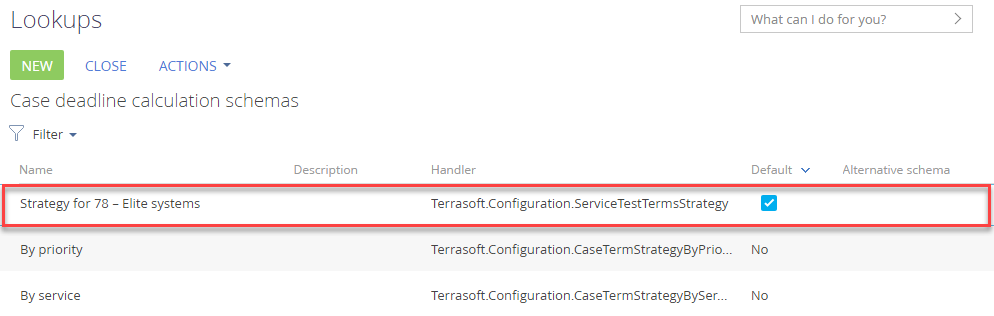
As a result, new response and resolution deadline calculation rules will be applied for cases per the 78 — Elite Systems agreement for the Lost data recovery service.
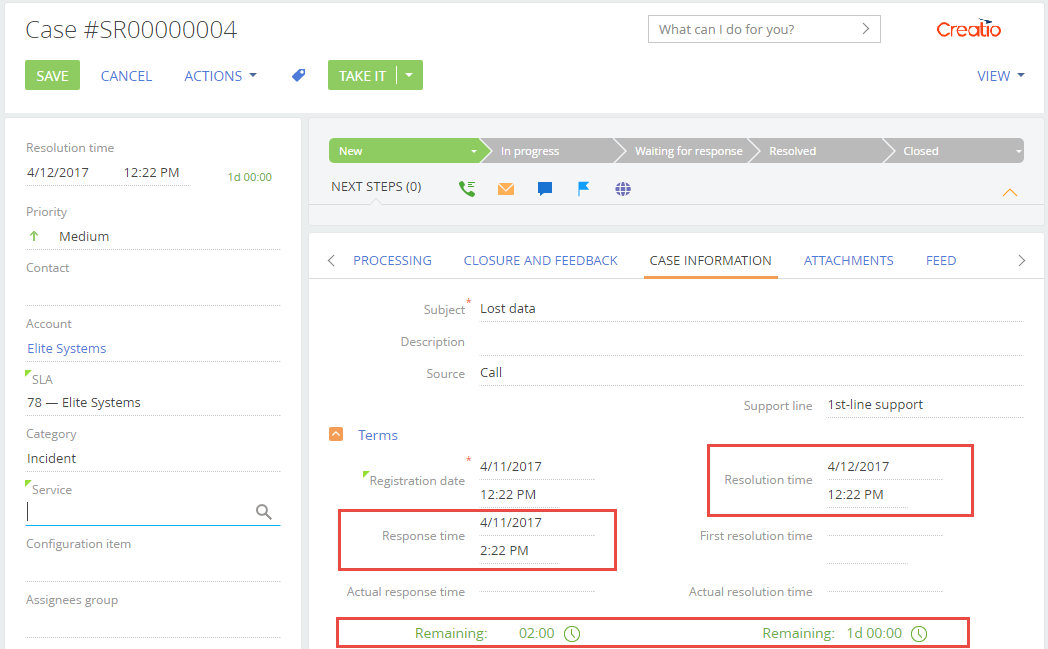