Send messages via WebSocket
WebSocket sends messages. Creatio broadcasts messages received via WebSocket to subscribers using the ClientMessageBridge
client module schema. Within Creatio, messages are sent using a sandbox
object. This is a broadcast message named SocketMessageReceived
. You can subscribe to the message and handle the received data.
Implement custom logic that sends a message
To implement custom logic that sends a message received via WebSocket:
- Create a replacing schema of the
ClientMessageBridge
client module schema. Learn more in a separate article: Client module. - Add the message to the
messages
property of a client schema. Learn more in a separate article: messages property. - Add the message received via WebSocket to the configuration object of schema messages. To do this, overload the
init()
parent method. - Trace the message sending. To do this, overload the
afterPublishMessage()
base method.
Save the message to history
Message history workflow is based on the Listener
handler that is a part of message publishing process in Creatio.
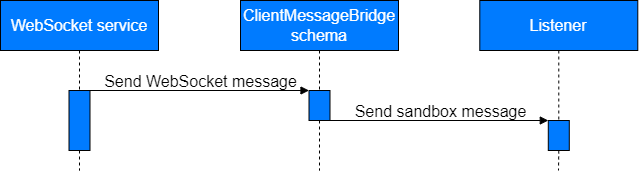
If the Listener
handler is not already loaded, Creatio executes the following actions:
- Save unhandled messages to history.
- Check availability of the
Listener
handler before publishing a message. - Publish all saved messages in their order of reception after the handler is loaded.
- Clear history after publishing messages from history.
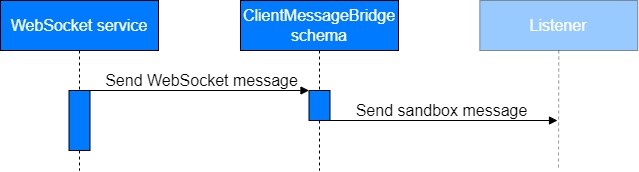
The following abstract methods of the BaseMessageBridge
class implement saving messages to history and working with them via localStorage
browser repository:
saveMessageToHistory()
. Saves a new message to the message collection.getMessagesFromHistory()
. Receives an array of messages by name.deleteSavedMessages()
. Deletes saved messages by name.
The ClientMessageBridge
schema implements the abstract methods of the BaseMessageBridge
parent class.
To implement saving messages to history, set the isSaveHistory
property to true
when adding a configuration object.
init: function() {
/* Call the parent init() method. */
this.callParent(arguments);
/* Add a new configuration object to the collection of configuration objects. */
this.addMessageConfig({
/* The name of the message received via WebSocket. */
sender: "OrderStepCalculated",
/* The name of the WebSocket message sent in Creatio via sandbox. */
messageName: "OrderStepCalculated",
/* Whether to save messages to history. */
isSaveHistory: true
});
},
To implement working with messages via another repository:
- Specify the
BaseMessageBridge
class as a parent class. - Implement custom
saveMessageToHistory()
,getMessagesFromHistory()
, anddeleteSavedMessages()
methods in the class that inherits from theBaseMessageBridge
class.