Implement a custom web service that uses cookie-based authentication
To implement the example, set up a custom web service. Read more >>>
Implement the UsrReceiveContactDataService
custom web service that uses cookie-based authentication and returns the contact information by the specified name. Creatio must return the following data:
Implement a custom web service
-
Open the Customer 360 app in the No-Code Designer.
-
Open the Advanced settings tab in the No-Code Designer. To do this, click
in the top right → "Application management" → "Application Hub" → Customer 360 app → "Advanced settings."
-
Create a user-made package to add the schema. To do this, click
→ Create new package → fill out the package properties → Save.
For this example, create the
sdkReceiveContactData
user-made package. -
Create the source code schema. To do this, click Add → Source code.
-
Fill out the schema properties.
For this example, use the schema properties as follows.
Property
Property value
Code
UsrReceiveContactDataService
Title
ReceiveContactDataService
-
Apply the changes.
-
Create a service class.
- Add the
Terrasoft.Configuration
namespace in the Schema Designer. You can use an arbitrary name. For example,UsrReceiveContactDataServiceNamespace
. - Add the namespaces the data types of which to utilize in the class using the
using
directive. - Add a class name that matches the schema name (the Code property).
- Specify the
Terrasoft.Nui.ServiceModel.WebService.BaseService
class as a parent class. - Add the
[ServiceContract]
and[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required)]
attributes to the class.
- Add the
-
Implement a class method.
Add the
public string GetContactIdByName(string Name)
method that implements the endpoint of the custom web service. The method executes database queries usingEntitySchemaQuery
. Depending on the value of theName
parameter in the query string, the response body will contain:- The contact ID (
string
type) if the contact is found. - The ID of the first contact (
string
type) if several contacts are found. - The empty string if no contacts are found.
View the source code of the
UsrReceiveContactDataService
custom web service below.UsrReceiveContactDataServicenamespace Terrasoft.Configuration.UsrReceiveContactDataServiceNamespace {
using System;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using Terrasoft.Core;
using Terrasoft.Web.Common;
using Terrasoft.Core.Entities;
[ServiceContract]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required)]
public class UsrReceiveContactDataService: BaseService {
/* The method that returns the contact ID by the contact name. */
[OperationContract]
[WebInvoke(Method = "GET", RequestFormat = WebMessageFormat.Json, BodyStyle = WebMessageBodyStyle.Wrapped, ResponseFormat = WebMessageFormat.Json)]
public string GetContactIdByName(string Name) {
/* The default result. */
var result = "";
/* The EntitySchemaQuery instance that accesses the "Contact" database table. */
var esq = new EntitySchemaQuery(UserConnection.EntitySchemaManager, "Contact");
/* Add columns to the query. */
var colId = esq.AddColumn("Id");
var colName = esq.AddColumn("Name");
/* Filter the query data. */
var esqFilter = esq.CreateFilterWithParameters(FilterComparisonType.Equal, "Name", Name);
esq.Filters.Add(esqFilter);
/* Retrieve the query results. */
var entities = esq.GetEntityCollection(UserConnection);
/* If the service receives data. */
if (entities.Count > 0) {
/* Return the "Id" column value of the first query result record. */
result = entities[0].GetColumnValue(colId.Name).ToString();
/* You can also use the following option.
result = entities[0].GetTypedColumnValue<string>(colId.Name); */
}
/* Return the results. */
return result;
}
}
} - The contact ID (
-
Publish the schema.
As a result, Creatio will add the custom UsrReceiveContactDataService
REST web service that has the GetContactIdByName
endpoint.
View the result
To view the outcome of the example without preauthorization:
-
Access the
GetContactIdByName
endpoint of theUsrReceiveContactDataService
web service from the browser address bar. -
Pass the contact name in the
Name
parameter. For example, "Mary King."Request stringCreatioURL/0/rest/UsrReceiveContactDataService/GetContactIdByName?Name=Mary%20King
As a result, the Creatio instance will return the 401 Unauthorized error.
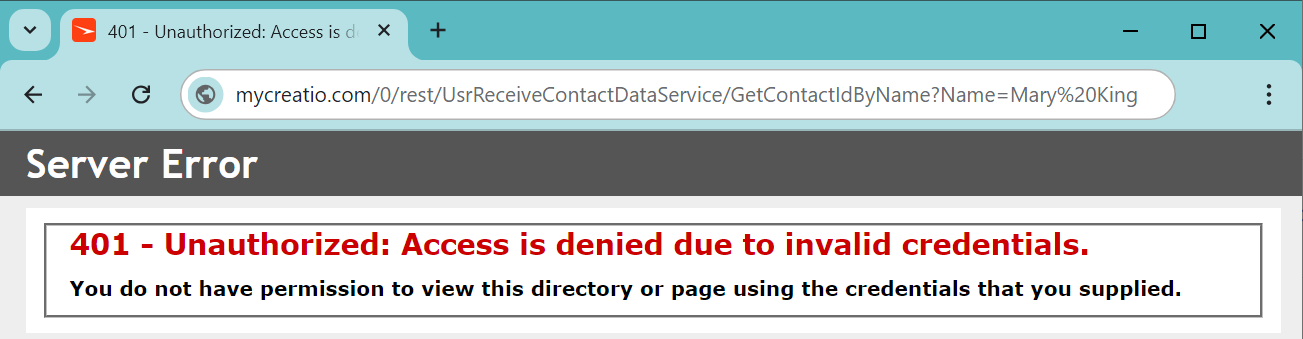
To view the outcome of the example that receives the name of a single contact:
-
Log in to Creatio.
-
Access the
GetContactIdByName
endpoint of theUsrReceiveContactDataService
web service from the browser address bar. -
Pass the contact name in the
Name
parameter. For example, "Mary King."Request stringCreatioURL/0/rest/UsrReceiveContactDataService/GetContactIdByName?Name=Mary%20King
As a result, the Creatio instance will return:
- The contact ID if the contact is found. View the result >>>
- The empty string if no contact is found. View the result >>>
To view the outcome of the example that receives the names of multiple contacts:
-
Log in to Creatio.
-
Access the
GetContactIdByName
endpoint of theUsrReceiveContactDataService
web service from the browser address bar. -
Pass the contact names in the
Name
parameter. For example, "Mary King" and "Andrew Wayne."Request stringCreatioURL/0/rest/UsrReceiveContactDataService/GetContactIdByName?Name=Mary%20King&Andrew%20Wayne
As a result, the Creatio instance will return:
- The ID of the first contact only if several contacts are found. View the result >>>
- The empty string if no contacts are found. View the result >>>
Source code
namespace Terrasoft.Configuration.UsrReceiveContactDataServiceNamespace {
using System;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using Terrasoft.Core;
using Terrasoft.Web.Common;
using Terrasoft.Core.Entities;
[ServiceContract]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Required)]
public class UsrReceiveContactDataService: BaseService {
/* The method that returns the contact ID by the contact name. */
[OperationContract]
[WebInvoke(Method = "GET", RequestFormat = WebMessageFormat.Json, BodyStyle = WebMessageBodyStyle.Wrapped, ResponseFormat = WebMessageFormat.Json)]
public string GetContactIdByName(string Name) {
/* The default result. */
var result = "";
/* The EntitySchemaQuery instance that accesses the "Contact" database table. */
var esq = new EntitySchemaQuery(UserConnection.EntitySchemaManager, "Contact");
/* Add columns to the query. */
var colId = esq.AddColumn("Id");
var colName = esq.AddColumn("Name");
/* Filter the query data. */
var esqFilter = esq.CreateFilterWithParameters(FilterComparisonType.Equal, "Name", Name);
esq.Filters.Add(esqFilter);
/* Retrieve the query results. */
var entities = esq.GetEntityCollection(UserConnection);
/* If the service receives data. */
if (entities.Count > 0) {
/* Return the "Id" column value of the first query result record. */
result = entities[0].GetColumnValue(colId.Name).ToString();
/* You can also use the following option.
result = entities[0].GetTypedColumnValue<string>(colId.Name); */
}
/* Return the results. */
return result;
}
}
}