Handle system-level data using Creatio AI in the front-end
The example is relevant to Case Management app.
To implement the example:
- Create a case. Read more >>>
- Develop AI Skill. Read more >>>
- Set up the page UI. Read more >>>
- Set up how to handle case resolution. Read more >>>
Minimize the resolution text of the custom "Creatio instance crashes while uploading files" case using Creatio AI. Support different tones of minimized text. Out of the box, use formal text tone. Limit the minimized text to 200 characters.
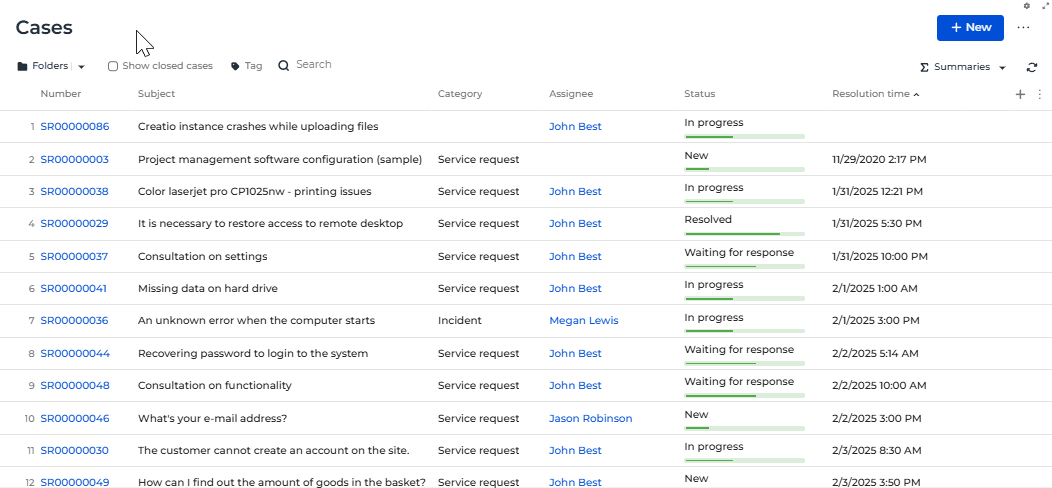
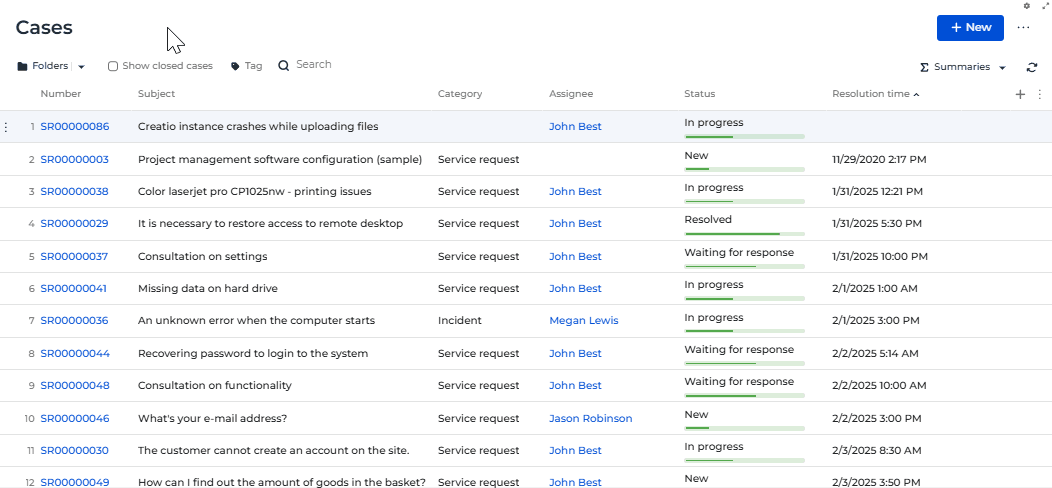
1. Create a case
-
Open the Case Management app in the No-Code Designer.
-
Open the Advanced settings tab in the No-Code Designer. To do this, click
in the top right → Application management → Application Hub → Case Management app → Advanced settings.
-
Create a user-made package to develop AI Skill. To do this, click
→ Create new package → fill out the package properties → Save.
For this example, create the
sdkCreatioAIInFreedomUI
user-made package. -
Change the current package. Instructions: Change the current package.
For this example, change the current package to
sdkCreatioAIInFreedomUI
user-made package. -
Open the Cases section.
-
Add a new case. To do this, click New.
-
Fill out the case properties.
Property
Property value
Contact*
John Best
Account
Our company
General info tab
Subject*
Creatio instance crashes while uploading files
Description
The Creatio instance constantly crashes when trying to upload a file larger than 10 MB to the server. The issue seems to be with the file handling method not releasing resources after the upload is complete. In some cases, uploading a file also leads to a system timeout, with the connection closing before the process ends. It makes it difficult to determine the exact cause.
Closure and feedback tab
Resolution
Upon further analysis, it was identified that the file upload function is not properly disposing of memory stream objects after the file is uploaded. The memory stream was held in memory, resulting in excessive memory consumption and eventually leading to out-of-memory exceptions. Furthermore, due to improper exception handling in a multithreading implementation, multiple threads attempting to access shared resources during concurrent uploads caused deadlocks, which in turn triggered timeouts as connections waited indefinitely for resource locks to be released.
We solved this issue by implementing a more efficient memory management strategy, ensuring that all threads are explicitly disposed of after use. We implemented more efficient "try-finally
" blocks to ensure that the cleanup logic is executed even in the event of an unexpected failure. We also introduced a semaphore to limit the number of concurrent downloads, preventing the server from overloading due to insufficient resources. Finally, we added more detailed logging to capture thread state and memory usage during the upload process for future troubleshooting. -
Select the "In progress" status on the progress bar. This saves the case.
As a result, Creatio will add the "Creatio instance crashes while uploading files" case to the Cases section.
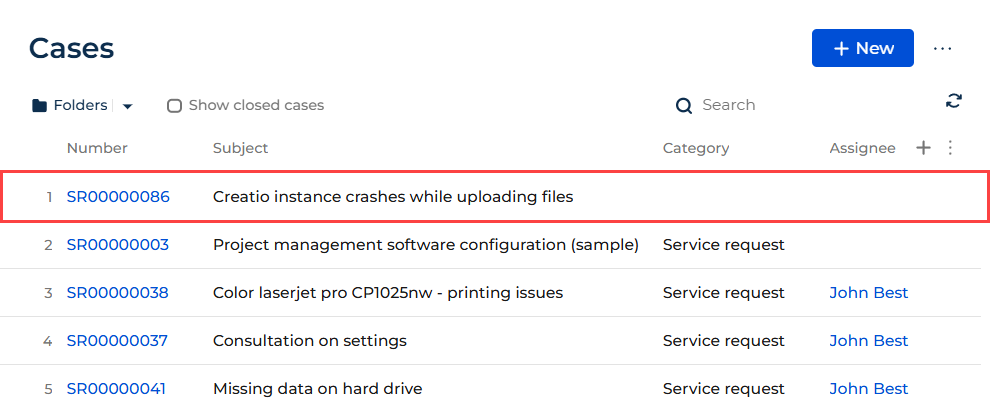
2. Develop AI Skill
-
Open the Copilot setup section. To do this, click
in the top right → System setup → Copilot setup.
-
Add an AI Skill. To do this, click New.
-
Fill out the properties of the AI Skill.
Property
Property value
Title*
Minimize the case resolution
Code*
UsrMinimizeTheCaseResolution
Description*
AI Skill that minimizes the case resolution
Status*
Active
Usage mode*
API
-
Specify the user's goal when using the AI Skill.
Property
Property value
Prompt
Your task is to minimize the following case resolution:
[# inputText #]
. Use the[# textTone #]
text tone and limit the minimized text to 200 characters. -
Save the changes.
As a result, Creatio will add the "Minimize the case resolution" AI Skill to the Copilot setup section.
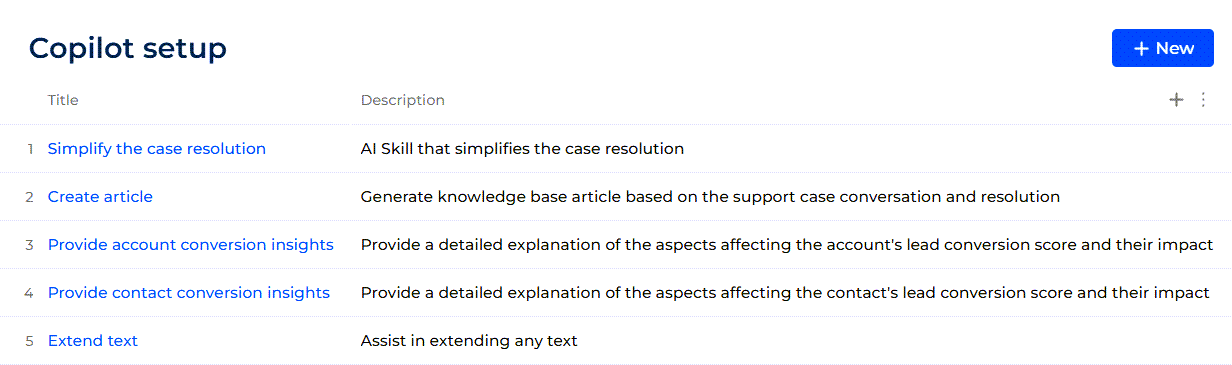
3. Set up the page UI
-
Open the Case Management app in the No-Code Designer.
-
Open the Cases form page.
-
Open the Closure and feedback tab.
-
Add a field.
For this example, add the field that contains the text tone to minimize the case resolution. To do this:
-
Add a field of needed type to the working area of the Freedom UI Designer.
-
Click
and fill out the field properties.
Element
Element type
Property
Property value
Field that contains the text tone to minimize the case resolution
Text
Title on page
Resolution tone
Placeholder
Formal
-
-
Add a button.
For this example, add the button that calls the "Minimize the case resolution" AI Skill.
To do this:
-
Add a Button type component to the toolbar of the Freedom UI Designer.
-
Click
and fill out the button properties.
Element
Property
Property value
Button that calls the "Minimize the case resolution" AI Skill
Title
Minimize the resolution
Style
Outline
-
-
Save the changes.
4. Set up how to handle case resolution
Configure the business logic in the Client Module Designer. For this example, set up how to handle case resolution.
-
Open the source code of the Freedom UI page. To do this, click
.
-
Add the dependencies. To do this, add
@creatio-devkit/common
library as a dependency.AMD module dependencies/* Declare the AMD module. */
define("Cases_FormPage", /**SCHEMA_DEPS*/["@creatio-devkit/common"] /**SCHEMA_DEPS*/, function/**SCHEMA_ARGS*/(sdk)/**SCHEMA_ARGS*/ {
return {
...
}
}); -
Add an attribute.
- Go to the
viewModelConfigDiff
schema section →values
configuration object. - Add a
IsCreatioAIElementsVisible
attribute that stores visibility data of the Resolution tone field and Minimize the resolution button. Out-of-the-box, the field and button are not visible. - Add a
ResolutionTone
attribute that stores data of the text tone to minimize the case resolution. Out-of-the-box, the text tone to minimize the case resolution is formal.
viewModelConfigDiff schema sectionviewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG_DIFF*/[
{
"operation": "merge",
"path": [
"attributes"
],
"values": {
...,
/* The attribute that stores visibility data of the "Resolution tone" field and "Minimize the resolution" button. */
"IsCreatioAIElementsVisible": {
"value": false
},
/* The attribute that stores data of the text tone to minimize the case resolution. */
"ResolutionTone": {
"value": 'Formal'
},
}
},
...
]/**SCHEMA_VIEW_MODEL_CONFIG_DIFF*/, - Go to the
-
Set up how to handle the action executed on button click.
- Go to the
viewConfigDiff
schema section →UsrMinimizeResolutionButton
element. - Bind the sending of the custom
usr.MinimizeText
request to theclicked
button event.
viewConfigDiff schema sectionviewConfigDiff: /**SCHEMA_VIEW_CONFIG_DIFF*/[
/* Button that calls the "Minimize the case resolution" AI Skill. */
{
"operation": "insert",
"name": "UsrMinimizeResolutionButton",
"values": {
...,
"clicked": {
/* Bind the sending of the custom request to the "clicked" button event. */
"request": "usr.MinimizeText"
}
},
...
}
]/**SCHEMA_VIEW_CONFIG_DIFF*/, - Go to the
-
Bind an attribute to the page elements.
- Go to the
viewConfigDiff
schema section →UsrResolutionToneField
element. - Bind the
$IsCreatioAIElementsVisible
attribute to thevisible
property. - Bind the
$ResolutionTone
attribute to thecontrol
property. - Go to the
UsrMinimizeResolutionButton
element. - Bind the
$IsCreatioAIElementsVisible
attribute to thevisible
property.
viewConfigDiff schema sectionviewConfigDiff: /**SCHEMA_VIEW_CONFIG_DIFF*/[
...,
/* Field that contains the text tone to minimize the case resolution. */
{
"operation": "insert",
"name": "UsrResolutionToneField",
"values": {
...,
"control": "$ResolutionTone",
/* The property that flags the field as visible. Bound to the "IsCreatioAIElementsVisible" attribute. */
"visible": "$IsCreatioAIElementsVisible",
},
...
},
/* Button that calls the "Minimize the case resolution" AI Skill. */
{
"operation": "insert",
"name": "UsrMinimizeResolutionButton",
"values": {
...,
/* The property that flags the button as visible. Bound to the "IsCreatioAIElementsVisible" attribute. */
"visible": "$IsCreatioAIElementsVisible",
},
...
},
...,
]/**SCHEMA_VIEW_CONFIG_DIFF*/, - Go to the
-
Implement the request handlers.
-
Go to the
handlers
schema section. -
Add a custom implementation of the
crt.HandleViewModelInitRequest
base request handler.- Wait for the rest of the initialization handlers to finish.
- Specify code of the "Minimize the case resolution" AI Skill.
- Retrieve the instance of the HTTP client from
@creatio-devkit/common
. - Send the
crt.CopilotGetAvailableIntentsRequest
base request that checks the accessibility of the AI Skill. - If the list of AI Skills includes the AI Skill whose code is
UsrMinimizeTheCaseResolution
, set theIsCreatioAIElementsVisible
attribute to the index of the corresponding AI Skill.
-
Implement the
usr.EditEventRequest
custom request handler.- Retrieve the instance of the HTTP client from
@creatio-devkit/common
. - Send the
crt.CopilotExecuteIntentRequest
base request that runs the AI Skill. - Specify code of the "Minimize the case resolution" AI Skill.
- Specify parameters of the "Minimize the case resolution" AI Skill. The
StringAttribute_qmmwoms
attribute stores data of the Resolution field. - If Creatio can run the AI Skill, AI Skill minimizes the case resolution. Otherwise, handle the status of the Creatio AI execution, display the error and warning messages if error or warning occur during the AI Skill execution.
- Call the next handler if it exists and return its result.
- Retrieve the instance of the HTTP client from
handlers schema sectionhandlers: /**SCHEMA_HANDLERS*/[
{
request: "crt.HandleViewModelInitRequest",
/* Custom implementation of a base request handler. */
handler: async (request, next) => {
/* Wait for the rest of the initialization handlers to finish. */
await next?.handle(request);
/* Code of the AI Skill. */
const skillName = 'UsrMinimizeTheCaseResolution';
/* Retrieve the instance of the HTTP client from "@creatio-devkit/common." */
const handlerChain = sdk.HandlerChainService.instance;
/* Send the base request that checks the accessibility of the AI Skill. */
var result = await handlerChain.process({
type: 'crt.CopilotGetAvailableIntentsRequest',
intentNames: [skillName],
$context: request.$context,
scopes: [...request.scopes]
});
/* If the list of AI Skills includes the AI Skill whose code is "UsrMinimizeTheCaseResolution," set the "IsCreatioAIElementsVisible" attribute to the index of the corresponding AI Skill. */
request.$context.IsCreatioAIElementsVisible = result.availableIntentNames.indexOf(skillName) > -1;
}
},
{
request: "usr.MinimizeText",
/* Implement the custom request handler. */
handler: async (request, next) => {
const context = request.$context;
/* Retrieve the instance of the HTTP client from "@creatio-devkit/common." */
const handlerChain = sdk.HandlerChainService.instance;
/* Send the base request that runs the AI Skill. */
const copilotCallResult = await handlerChain.process({
type: 'crt.CopilotExecuteIntentRequest',
/* Code of the AI Skill to run. */
intentName: 'UsrMinimizeTheCaseResolution',
/* Parameters of the AI Skill. */
parameters: {
inputText: await context.StringAttribute_qmmwoms,
textTone: await context.ResolutionTone
},
$context: request.$context,
scopes: [...request.scopes]
});
/* If Creatio can run the AI Skill, AI Skill minimizes the case resolution. */
if (copilotCallResult.isSuccess) {
request.$context.StringAttribute_qmmwoms = copilotCallResult.content;
} else {
/* If the status of the AI Skill execution is "FailedToExecute," the browser console displays the "Error occurs during the AI Skill execution" error message. Otherwise, implement a business logic that uses a predefined "errorMessage" value depending on your business goals. */
if(copilotCallResult.status == 'FailedToExecute'){
console.error(copilotCallResult.errorMessage);
alert("Error occurs during the AI Skill execution");
} else {
alert(copilotCallResult.errorMessage);
};
}
/* If warning occurs during the AI Skill execution, the browser console displays the list of warnings. Many messages are separated by comma. */
if(copilotCallResult.warnings.length > 0){
console.warn(copilotCallResult.warnings.join(', '));
}
/* Call the next handler if it exists and return its result. */
return next?.handle(request);
}
}
]/**SCHEMA_HANDLERS*/, -
-
Save the changes.
View the result
To view the result of the example that uses an out-of-the-box text tone to minimize the case resolution:
- Open the Cases section.
- Open the "Creatio instance crashes while uploading files" case.
- Click Minimize the resolution.
As a result, Creatio AI will minimize the case resolution using out-of-the-box text tone and add minimized text to the Resolution field. The minimized text is limited to 200 characters. View the result >>>
If you need, edit the Resolution field value provided by Creatio AI.
To view the result of the example that uses custom text tone to minimize the case resolution:
- Open the Cases section.
- Open the "Creatio instance crashes while uploading files" case.
- Change a text tone to minimize the case resolution. For example, "Friendly."
- Click Minimize the resolution.
As a result, Creatio AI will minimize the case resolution using custom text tone and add minimized text to the Resolution field. The minimized text is limited to 200 characters. View the result >>>
Source code
/* Declare the AMD module. */
define("Cases_FormPage", /**SCHEMA_DEPS*/["@creatio-devkit/common"]/**SCHEMA_DEPS*/, function/**SCHEMA_ARGS*/(sdk)/**SCHEMA_ARGS*/ {
return {
viewConfigDiff: /**SCHEMA_VIEW_CONFIG_DIFF*/[
/* Field that contains the text tone to minimize the case resolution. */
{
"operation": "insert",
"name": "UsrResolutionToneField",
"values": {
"layoutConfig": {
"column": 1,
"row": 2,
"colSpan": 1,
"rowSpan": 1
},
"type": "crt.Input",
"label": "#ResourceString(UsrResolutionToneField_caption)#",
"control": "$ResolutionTone",
"placeholder": "#ResourceString(UsrResolutionToneField_placeholder)#",
"tooltip": "",
"readonly": false,
"multiline": false,
"labelPosition": "auto",
/* The property that flags the field as visible. Bound to the "IsCreatioAIElementsVisible" attribute. */
"visible": "$IsCreatioAIElementsVisible",
},
"parentName": "ClosureAndFeedbackFieldsContainer",
"propertyName": "items",
"index": 1
},
/* Button that calls the "Minimize the case resolution" AI Skill. */
{
"operation": "insert",
"name": "UsrMinimizeResolutionButton",
"values": {
"layoutConfig": {
"column": 2,
"row": 2,
"colSpan": 1,
"rowSpan": 1
},
"type": "crt.Button",
"caption": "#ResourceString(UsrMinimizeResolutionButton_caption)#",
"color": "outline",
"disabled": false,
"size": "large",
"iconPosition": "only-text",
/* The property that flags the button as visible. Bound to the "IsCreatioAIElementsVisible" attribute. */
"visible": "$IsCreatioAIElementsVisible",
"clicked": {
/* Bind the sending of the custom request to the "clicked" button event. */
"request": "usr.MinimizeText"
}
},
"parentName": "ClosureAndFeedbackFieldsContainer",
"propertyName": "items",
"index": 2
}
]/**SCHEMA_VIEW_CONFIG_DIFF*/,
viewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG_DIFF*/[
{
"operation": "merge",
"path": [
"attributes"
],
"values": {
/* The attribute that stores visibility data of the "Resolution tone" field and "Minimize the resolution" button. */
"IsCreatioAIElementsVisible": {
"value": false
},
/* The attribute that stores data of the text tone to minimize the case resolution. */
"ResolutionTone": {
"value": 'Formal'
},
}
}
]/**SCHEMA_VIEW_MODEL_CONFIG_DIFF*/,
modelConfigDiff: /**SCHEMA_MODEL_CONFIG_DIFF*/[]/**SCHEMA_MODEL_CONFIG_DIFF*/,
handlers: /**SCHEMA_HANDLERS*/[
{
request: "crt.HandleViewModelInitRequest",
/* Custom implementation of a base request handler. */
handler: async (request, next) => {
/* Wait for the rest of the initialization handlers to finish. */
await next?.handle(request);
/* Code of the AI Skill. */
const skillName = 'UsrMinimizeTheCaseResolution';
/* Retrieve the instance of the HTTP client from "@creatio-devkit/common." */
const handlerChain = sdk.HandlerChainService.instance;
/* Send the base request that checks the accessibility of the AI Skill. */
var result = await handlerChain.process({
type: 'crt.CopilotGetAvailableIntentsRequest',
intentNames: [skillName],
$context: request.$context,
scopes: [...request.scopes]
});
/* If the list of AI Skills includes the AI Skill whose code is "UsrMinimizeTheCaseResolution," set the "IsCreatioAIElementsVisible" attribute to the index of the corresponding AI Skill. */
request.$context.IsCreatioAIElementsVisible = result.availableIntentNames.indexOf(skillName) > -1;
}
},
{
request: "usr.MinimizeText",
/* Implement the custom request handler. */
handler: async (request, next) => {
const context = request.$context;
/* Retrieve the instance of the HTTP client from "@creatio-devkit/common." */
const handlerChain = sdk.HandlerChainService.instance;
/* Send the base request that runs the AI Skill. */
const copilotCallResult = await handlerChain.process({
type: 'crt.CopilotExecuteIntentRequest',
/* Code of the AI Skill to run. */
intentName: 'UsrMinimizeTheCaseResolution',
/* Parameters of the AI Skill. */
parameters: {
inputText: await context.StringAttribute_qmmwoms,
textTone: await context.ResolutionTone
},
$context: request.$context,
scopes: [...request.scopes]
});
/* If Creatio can run the AI Skill, AI Skill minimizes the case resolution. */
if (copilotCallResult.isSuccess) {
request.$context.StringAttribute_qmmwoms = copilotCallResult.content;
} else {
/* If the status of the AI Skill execution is "FailedToExecute," the browser console displays the "Error occurs during the AI Skill execution" error message. Otherwise, implement a business logic that uses a predefined "errorMessage" value depending on your business goals. */
if(copilotCallResult.status == 'FailedToExecute'){
console.error(copilotCallResult.errorMessage);
alert("Error occurs during the AI Skill execution");
} else {
alert(copilotCallResult.errorMessage);
};
}
/* If warning occurs during the AI Skill execution, the browser console displays the list of warnings. Many messages are separated by comma. */
if(copilotCallResult.warnings.length > 0){
console.warn(copilotCallResult.warnings.join(', '));
}
/* Call the next handler if it exists and return its result. */
return next?.handle(request);
}
}
]/**SCHEMA_HANDLERS*/,
converters: /**SCHEMA_CONVERTERS*/{}/**SCHEMA_CONVERTERS*/,
validators: /**SCHEMA_VALIDATORS*/{}/**SCHEMA_VALIDATORS*/
};
});