Implement Word report using custom macros
To implement the example:
- Implement custom macros. Read more >>>
- Create a report. Read more >>>
- Set up the report columns. Read more >>>
- Set up the report template. Read more >>>
- Upload the file of report template to Creatio. Read more >>>
- Set up how to display the report. Read more >>>
Generate the custom "Account summary" Word report from the account page. The report must include the following account fields:
-
Name.
-
Type.
-
Primary contact.
-
Additional information.
- Display the annual revenue for "Customer" account type.
- Display the number of employees for "Partner" account type.
- Display an empty string for other account types.
-
Date of report generation.
-
Employee who generated the report.
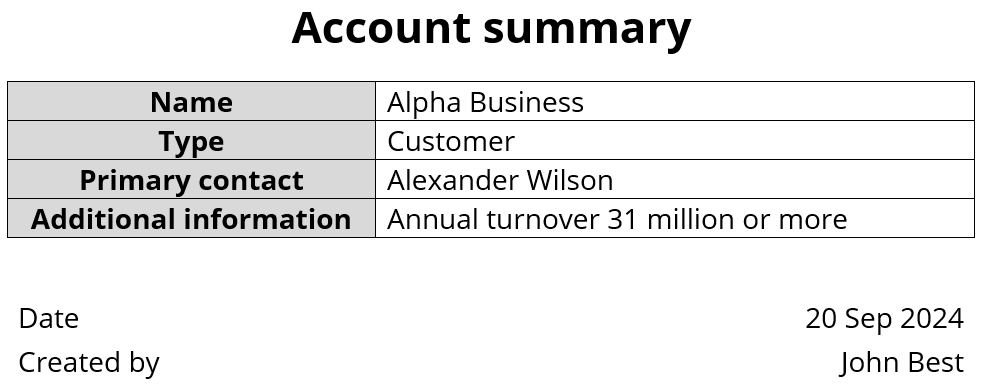
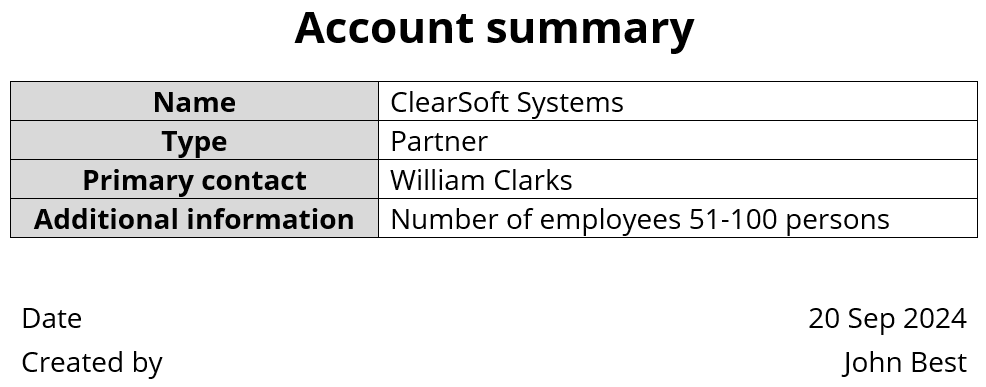
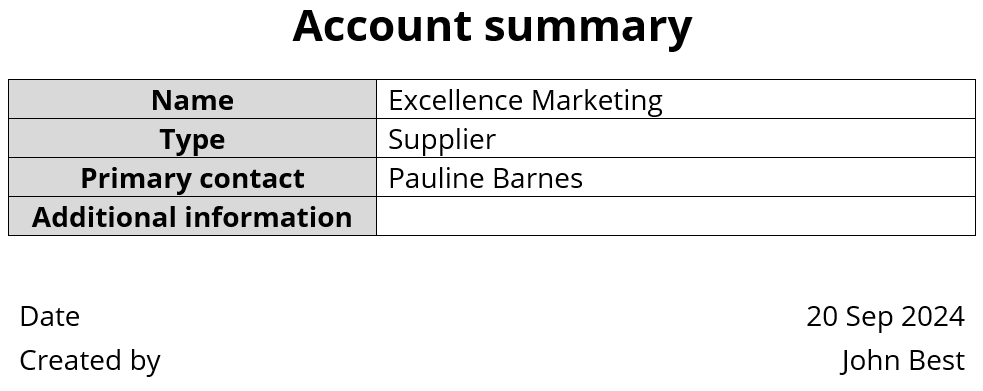
1. Implement custom macros
For this example, implement the following custom macros:
- Macro that receives additional information about the account depending on the account type.
- Macro that receives date of report generation.
- Macro that receives the name of the employee who generated the report.
Additional account information
-
Open the Customer 360 app in the No-Code Designer.
-
Open the Advanced settings tab in the No-Code Designer. To do this, click
in the top right → "Application management" → "Application Hub" → Customer 360 app → "Advanced settings."
-
Create a user-made package to add the schema. To do this, click
→ Create new package → fill out the package properties → Save.
For this example, create the
sdkMsWordReportCustomMacros
user-made package. -
Add the package properties.
- Open the package properties. To do this, click
→ Properties. This opens the Dependencies tab on the Package properties page.
- Click Add in the Depends on Packages block. This opens the Select package window.
- Select the checkbox for the
CrtNUI
package. TheCrtNUI
package includes theExpressionConverterHelper
schema that implements the basicIExpressionConverter
interface. - Click Select.
- Apply the changes.
- Open the package properties. To do this, click
-
Change the current package. Instructions: Change the current package.
For this example, change the current package to
sdkMsWordReportCustomMacros
user-made package. -
Create the source code schema. To do this, click Add → Source code.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Code
UsrAccountInfoByAccountType
Title
AccountInfoByAccountType
-
Apply the changes.
-
Add localizable strings.
For this example, add the following localizable strings:
- Localizable string that stores the text to be displayed in the report for the "Customer" account type.
- Localizable string that stores the text to be displayed in the report for the "Partner" account type.
To do this:
-
Create a localizable string. To do this, go the Localizable strings additional property node and click
.
-
Fill out the localizable string properties.
Element
Property
Property value
Localizable string that stores the text to be displayed in the report for the "Customer" account type
Code
CustomerAccountType
Value
Annual turnover {0}
Localizable string that stores the text to be displayed in the report for the "Partner" account type.
Code
PartnerAccountType
Value
Number of employees {0} persons
-
Save the changes.
-
Implement the business logic of receiving additional account information depending on the account type.
UsrAccountInfoByAccountTypenamespace Terrasoft.Configuration
{
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Packages;
using Terrasoft.Core.Factories;
/* Attribute that stores the macro name. */
[ExpressionConverterAttribute("AccountInfoByAccountType")]
/* Class that implements the "IExpressionConverter" interface. */
class UsrAccountInfoByAccountType : IExpressionConverter
{
private UserConnection _userConnection;
private string _customerAdditional;
private string _partnerAdditional;
/* Call localizable string values. */
private void SetResources() {
string sourceCodeName = "UsrAccountInfoByAccountType";
_customerAdditional = new LocalizableString(_userConnection.ResourceStorage, sourceCodeName, "LocalizableStrings.CustomerAccountType.Value");
_partnerAdditional = new LocalizableString(_userConnection.ResourceStorage, sourceCodeName, "LocalizableStrings.PartnerAccountType.Value");
}
/* Implement the "Evaluate()" method of the "IExpressionConverter" interface. */
public string Evaluate(object value, string arguments = "")
{
try
{
_userConnection = (UserConnection)HttpContext.Current.Session["UserConnection"];
Guid accountId = new Guid(value.ToString());
return getAccountInfo(accountId);
}
catch (Exception err)
{
return err.Message;
}
}
/* Receive additional information based on the account type ID. */
private string getAccountInfo(Guid accountId)
{
SetResources();
try
{
/* Create an "EntitySchemaQuery" instance that has the "Account" root schema. */
EntitySchemaQuery esq = new EntitySchemaQuery(_userConnection.EntitySchemaManager, "Account");
/* Add columns to the query. */
var columnType = esq.AddColumn("Type.Name").Name;
var columnNumber = esq.AddColumn("EmployeesNumber.Name").Name;
var columnRevenue = esq.AddColumn("AnnualRevenue.Name").Name;
/* Filter records by the account ID. */
var accountFilter = esq.CreateFilterWithParameters(
FilterComparisonType.Equal,
"Id",
accountId
);
esq.Filters.Add(accountFilter);
/* Retrieve an entity collection. */
EntityCollection entities = esq.GetEntityCollection(_userConnection);
/* If the collection includes entities, the method returns data depending on the account type. */
if (entities.Count > 0)
{
Entity entity = entities[0];
var type = entity.GetTypedColumnValue<string>(columnType);
switch (type)
{
case "Customer":
return String.Format(_customerAdditional, entity.GetTypedColumnValue<string>(columnRevenue));
case "Partner":
return String.Format(_partnerAdditional, entity.GetTypedColumnValue<string>(columnNumber));
default:
return String.Empty;
}
}
return String.Empty;
}
catch (Exception err)
{
throw err;
}
}
}
} -
Publish the schema.
Date of report generation
-
Select a user-made package to add the schema.
For this example, select the
sdkMsWordReportCustomMacros
user-made package. -
Create the source code schema. To do this, click Add → Source code.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Code
UsrCurrentDate
Title
CurrentDate
-
Apply the changes.
-
Implement the business logic of receiving date of report generation.
UsrCurrentDatenamespace Terrasoft.Configuration
{
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Packages;
using Terrasoft.Core.Factories;
/* Attribute that stores the macro name. */
[ExpressionConverterAttribute("CurrentDate")]
/* Class that implements the "IExpressionConverter" interface. */
class UsrCurrentDate : IExpressionConverter
{
private UserConnection _userConnection;
/* Implement the "Evaluate()" method of the "IExpressionConverter" interface. */
public string Evaluate(object value, string arguments = "")
{
try
{
_userConnection = (UserConnection)HttpContext.Current.Session["UserConnection"];
/* Return current date. */
return _userConnection.CurrentUser.GetCurrentDateTime().Date.ToString("dd MMM yyyy");
}
catch (Exception err)
{
return err.Message;
}
}
}
} -
Publish the schema.
Employee who generated the report
-
Select a user-made package to add the schema.
For this example, select the
sdkMsWordReportCustomMacros
user-made package. -
Create the source code schema. To do this, click Add → Source code.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Code
UsrCurrentUser
Title
CurrentUser
-
Apply the changes.
-
Implement the business logic of receiving the name of the employee who generated the report.
UsrCurrentUsernamespace Terrasoft.Configuration
{
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Packages;
using Terrasoft.Core.Factories;
/* Attribute that stores the macro name. */
[ExpressionConverterAttribute("CurrentUser")]
/* Class that implements the "IExpressionConverter" interface. */
class UsrCurrentUser : IExpressionConverter
{
private UserConnection _userConnection;
/* Implement the "Evaluate()" method of the "IExpressionConverter" interface. */
public string Evaluate(object value, string arguments = "")
{
try
{
_userConnection = (UserConnection)HttpContext.Current.Session["UserConnection"];
/* Return the contact of current user. */
return _userConnection.CurrentUser.ContactName;
}
catch (Exception err)
{
return err.Message;
}
}
}
} -
Publish the schema.
2. Create a report
-
Open the Report setup section. To do this, click
in the top right → System setup → Report setup.
-
Click New report.
-
Fill out the report properties.
Property
Property value
Report name
Account summary
Object
Account
Show in the list view
Clear the checkbox
Show in the record page
Select the checkbox
-
Apply the changes.
As a result, Creatio will add the "Account summary" report to the Report setup section.
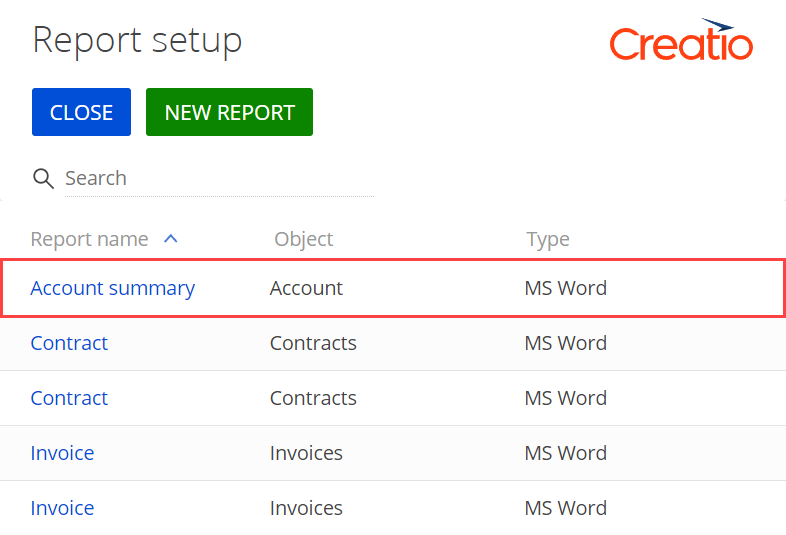
3. Set up the report columns
-
Add the report columns.
-
Go to the Set up report data block.
-
Add the column of account name. To do this, click
→ open the Column field → select Id → click Select.
The Id column is an input parameter for a custom macro.
-
Add the Id, Name, Type, Primary contact and Id columns similarly.
As a result, the "Account summary" report data will be as follows.
-
-
Add the macro tag to the column.
For this example, add the macro tags to the different Id columns. To do this:
-
Open the setting page of the Id column. To do this, go to the Set up report data block and double-click the column or click
in the column row.
-
Go to the Title property.
-
Add the
[#CurrentDate#]
macro tag to the Name column. The[#CurrentDate#]
macro receives date of report generation. -
Add the
[#CurrentUser#]
and[#AccountInfoByAccountType#]
macro tags to the different Id columns similarly.The
[#CurrentUser#]
macro receives the name of the employee who generated the report.The
[#AccountInfoByAccountType#]
macro receives additional information of the account depending on the account type. -
Save the changes.
-
-
Apply the changes.
As a result, the "Account summary" report data will be as follows.
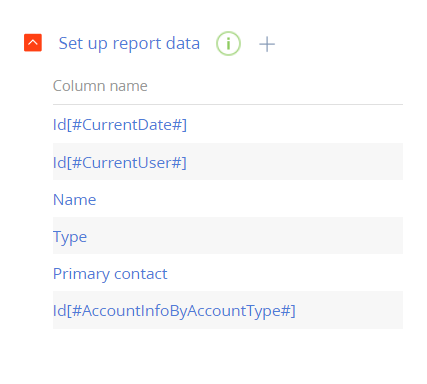
4. Set up the report template
-
Install the Creatio plug-in for Microsoft Word. Instructions: Install Creatio plug-in for Microsoft Word (user documentation). This is a one-time procedure.
-
Run the Microsoft Word app.
-
Connect to the Creatio instance that includes the created report.
-
Open the Creatio tab.
-
Click Connect. This opens the Login window.
-
Enter the Creatio user credentials.
-
Click
. This opens the Available Servers window.
-
Click New. This opens the Server Connection Setup window.
-
Fill out the server properties.
Property
Property value
Name
An arbitrary server name. For example, "Creatio."
Link
URL of the Creatio instance that includes the created report. For example,
https://mycreatio.com/
. -
Click OK. This closes the Server Connection Setup window.
-
Click OK. This closes the Available Servers window and adds the server name to the Server field of the Login window.
-
Click OK. This closes the Login window and connects to the Creatio instance.
-
-
Select the report to set up the template.
- Open the Creatio tab.
- Click Select report. This opens the Creatio Word reports window.
- Select "Account summary" report.
- Click OK. This closes the Creatio Word reports window and opens the Word report data panel that includes the "Account summary" report data set up in the Creatio instance.
-
Set up the template layout based on your business goals.
As a result, the template of the "Account summary" report will look as follows.
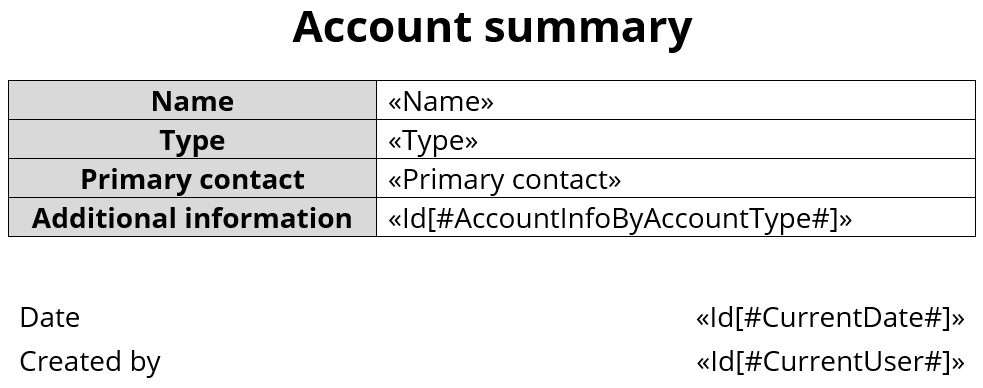
5. Upload the file of report template to Creatio
- Open the Creatio tab.
- Click Save to Creatio.
As a result, the template file of the "Account summary" report will be uploaded to the report page in Creatio.
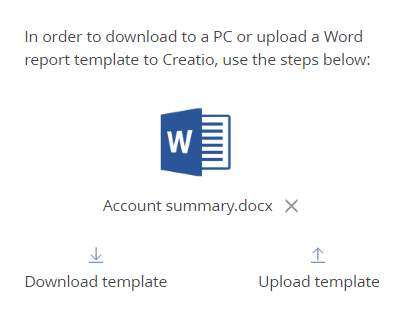
6. Set up how to display the report
Add the report to the account page. To do this:
-
Open the Accounts form page. To do this, click
in the top right → Application management → Application Hub → Customer 360 → Accounts form page.
-
Add a button that opens the "Account summary" report.
-
Add a Button type component to the toolbar of the Freedom UI Designer.
-
Click
and fill out the button properties.
Element
Property
Property value
Button that opens the "Account summary" report
Title
Print report
Action
Print report
Print settings
Print report for the current record
-
-
Save the changes.
As a result, Creatio will add the Print report button that lets you open the "Account summary" report from the account page.
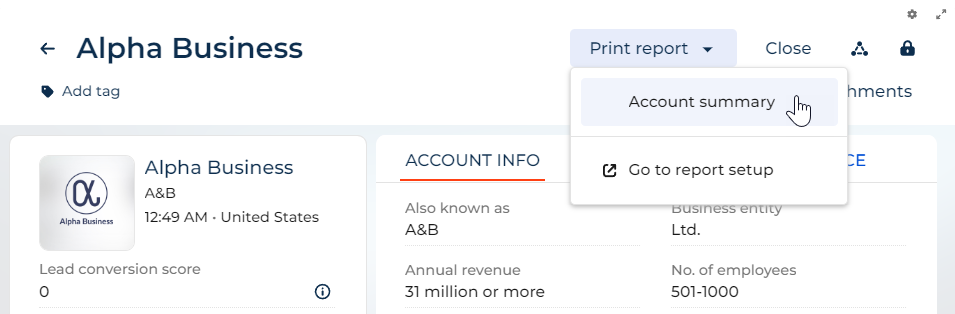
View the result
- Open the Accounts section.
- Open the page of an arbitrary account.
- Generate the report. To do this, click Print report → Account summary.
- Save the report file to your device.
- Open the report file.
As a result, Creatio will generate the "Account summary" report from the account page. The Additional information field value differs for different account types:
- For the "Customer" account type, the field will include the annual revenue. View the result >>>
- For the "Partner" account type, the field will include the number of employees. View the result >>>
- For other account types, the field will include empty string. View the result >>>
Source code
- UsrAccountInfoByAccountType
- UsrCurrentDate
- UsrCurrentUser
namespace Terrasoft.Configuration
{
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Packages;
using Terrasoft.Core.Factories;
/* Attribute that stores the macro name. */
[ExpressionConverterAttribute("AccountInfoByAccountType")]
/* Class that implements the "IExpressionConverter" interface. */
class UsrAccountInfoByAccountType : IExpressionConverter
{
private UserConnection _userConnection;
private string _customerAdditional;
private string _partnerAdditional;
/* Call localizable string values. */
private void SetResources() {
string sourceCodeName = "UsrAccountInfoByAccountType";
_customerAdditional = new LocalizableString(_userConnection.ResourceStorage, sourceCodeName, "LocalizableStrings.CustomerAccountType.Value");
_partnerAdditional = new LocalizableString(_userConnection.ResourceStorage, sourceCodeName, "LocalizableStrings.PartnerAccountType.Value");
}
/* Implement the "Evaluate()" method of the "IExpressionConverter" interface. */
public string Evaluate(object value, string arguments = "")
{
try
{
_userConnection = (UserConnection)HttpContext.Current.Session["UserConnection"];
Guid accountId = new Guid(value.ToString());
return getAccountInfo(accountId);
}
catch (Exception err)
{
return err.Message;
}
}
/* Receive additional information based on the account type ID. */
private string getAccountInfo(Guid accountId)
{
SetResources();
try
{
/* Create an "EntitySchemaQuery" instance that has the "Account" root schema. */
EntitySchemaQuery esq = new EntitySchemaQuery(_userConnection.EntitySchemaManager, "Account");
/* Add columns to the query. */
var columnType = esq.AddColumn("Type.Name").Name;
var columnNumber = esq.AddColumn("EmployeesNumber.Name").Name;
var columnRevenue = esq.AddColumn("AnnualRevenue.Name").Name;
/* Filter records by the account ID. */
var accountFilter = esq.CreateFilterWithParameters(
FilterComparisonType.Equal,
"Id",
accountId
);
esq.Filters.Add(accountFilter);
/* Retrieve an entity collection. */
EntityCollection entities = esq.GetEntityCollection(_userConnection);
/* If the collection includes entities, the method returns data depending on the account type. */
if (entities.Count > 0)
{
Entity entity = entities[0];
var type = entity.GetTypedColumnValue<string>(columnType);
switch (type)
{
case "Customer":
return String.Format(_customerAdditional, entity.GetTypedColumnValue<string>(columnRevenue));
case "Partner":
return String.Format(_partnerAdditional, entity.GetTypedColumnValue<string>(columnNumber));
default:
return String.Empty;
}
}
return String.Empty;
}
catch (Exception err)
{
throw err;
}
}
}
}
namespace Terrasoft.Configuration
{
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Packages;
using Terrasoft.Core.Factories;
/* Attribute that stores the macro name. */
[ExpressionConverterAttribute("CurrentDate")]
/* Class that implements the "IExpressionConverter" interface. */
class UsrCurrentDate : IExpressionConverter
{
private UserConnection _userConnection;
/* Implement the "Evaluate()" method of the "IExpressionConverter" interface. */
public string Evaluate(object value, string arguments = "")
{
try
{
_userConnection = (UserConnection)HttpContext.Current.Session["UserConnection"];
/* Return current date. */
return _userConnection.CurrentUser.GetCurrentDateTime().Date.ToString("dd MMM yyyy");
}
catch (Exception err)
{
return err.Message;
}
}
}
}
namespace Terrasoft.Configuration
{
using System;
using System.CodeDom.Compiler;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.ServiceModel.Activation;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using Terrasoft.Common;
using Terrasoft.Core;
using Terrasoft.Core.DB;
using Terrasoft.Core.Entities;
using Terrasoft.Core.Packages;
using Terrasoft.Core.Factories;
/* Attribute that stores the macro name. */
[ExpressionConverterAttribute("CurrentUser")]
/* Class that implements the "IExpressionConverter" interface. */
class UsrCurrentUser : IExpressionConverter
{
private UserConnection _userConnection;
/* Implement the "Evaluate()" method of the "IExpressionConverter" interface. */
public string Evaluate(object value, string arguments = "")
{
try
{
_userConnection = (UserConnection)HttpContext.Current.Session["UserConnection"];
/* Return the contact of current user. */
return _userConnection.CurrentUser.ContactName;
}
catch (Exception err)
{
return err.Message;
}
}
}
}