Data handling basics
Data handling in Creatio 8.x is based on the DAO (Data Access Object) pattern. Learn more about the DAO pattern: Data access object (Wikipedia).
The DAO pattern has the following layers:
BusinessObject
. Implements the business logic.DataAccessObject
. Enables Creatio to access data. The business logic and data source are separated from each other. The DataAccessObject layer can create a DTO (Data Transfer Object). The DTO can use theBusinessObject
layer to save DTOs and return them to the DataAccessObject layer. Learn more about the DTO pattern: Data transfer object (Wikipedia).DataStorage
. Organizes various data repositories.
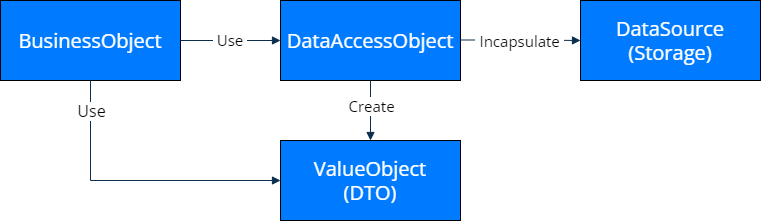
View the detailed data storage diagram in the figure below.
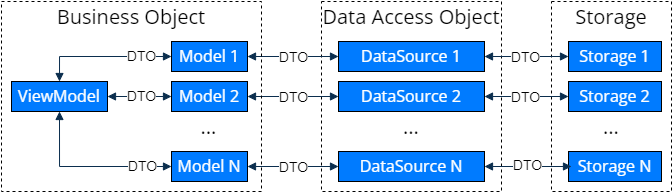
DataStorage layer
The DataStorage
layer is linked to a particular data source.
The types of storage are as follows:
- Creatio database
- web service
- file system
DataAccessObject layer
The DataAccessObject
layer contains data sources (Data Source). Each data source is linked to the corresponding model within the business logic layer.
The DataAccessObject
layer performs the following functions:
- Enable users to perform CRUD operations (
load
,save
,insert
,delete
). - Provide permissions for data operations (
canEdit
,canCreate
,canDelete
). - Provide data structure (
getSchema
).
Data schema describes the structure of data managed by Creatio.
Data schema includes the following components:
- name
- title
- attributes
Important structural elements of attributes are validators. Learn more about validators: Freedom UI page customization basics.
Learn more about the classes that describe the data schema and attribute structure below.
- Data schema structure
- Attribute structure
export class DataSchema {
public name: string;
public caption: LocalizableString;
public attributes: DataSchemaAttribute[];
public primaryAttributeName?: string;
public primaryDisplayAttributeName?: string;
}
export class DataSchemaAttribute {
public name: string;
public caption: string;
public path: string;
public dataValueType: DataValueType;
public validators: DataSchemaValidatorConfig;
public defaultValue: JsonData;
public isValueCloneable: boolean;
public attributeType: DataSchemaAttributeType;
public referenceSchemaName?: string;
}
BusinessObject layer
The BusinessObject
layer contains the view model (View model
), which, in turn, can contain several models (Model
). Learn more about the View model
and Model
layers: Creatio front-end architecture.
Model
The modelConfigDiff
section of the client schema describes data models. The model contains the data source description. Creatio implements EntityDataSource
that lets you manage the Creatio database.
Use the data source to perform various data actions, for example:
- Add new and existing data source elements to the canvas of the Freedom UI Designer.
- Edit the data source elements.
- Bind the controls to the data source elements on the canvas.
View an example that registers a model in the client schema below.
modelConfigDiff: /**SCHEMA_MODEL_CONFIG*/{
"dataSources": {
/* Unique names of data sources. */
"ContactDS": {
/* Data source type. */
"type": "crt.EntityDataSource",
"config": {
/* Data schema code. */
"entitySchemaName": "Contact"
}
}
}
}/**SCHEMA_MODEL_CONFIG*/
Learn more about the client schema structure: Client Schema.
View model
The view model handles the ViewModel
layer that contains the business logic of the interaction between the View
and Model
layers.
Configure the view model in the viewModelConfigDiff
section of the client schema. For each view model attribute, specify the corresponding model attribute in the modelConfigDiff
schema section.
View an example that describes a view model in the client schema below.
viewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
/* Bind the ContactName view model attribute to the Name attribute of the ContactDS model.*/
"ContactName": {
"modelConfigDiff": {
"path": "ContactDS.Name"
}
}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/
The view model can have the following attribute types:
- simple type columns
- collection columns
- direct link columns
Attributes for columns that contain simple data types
Simple data types can include the following data source elements:
- string
- number
- boolean
- date/time
To create an attribute for a column that contains a simple data type:
-
Register the data source in the schema of the Freedom UI page.
Register the data sourcemodelConfigDiff: /**SCHEMA_MODEL_CONFIG*/{
"dataSources": {
"ContactDS": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "Contact",
}
}
}
}/**SCHEMA_MODEL_CONFIG*/ -
Create an attribute in the
attributes
property of theviewModelConfigDiff
schema section.Create an attributeviewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
"StringAttribute_jghoo32": {
"modelConfigDiff": {
"path": "ContactDS.Name"
}
}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/,
Use a unique attribute name.
The base attribute property is modelConfigDiff
. This property describes the link to the data source element using the path
property. The path
property value must have the following format: [Data Source Name].[Column Code]
. For this example, the path
property value is ContactDS.Name
.
Attributes for collections
The view model lets you bind attributes that are data collections. To bind a collection type attribute, set the isCollection
attribute property to true
.
viewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
/* Bind a collection type attribute of the AccountList view model to the AccountDS model.*/
"AccountList": {
"isCollection": true,
"modelConfigDiff": {
"path": "AccountDS",
"pagingConfig": {},
"sortingConfig": {},
"filterAttributes": {}
},
"viewModelConfigDiff": {
"attributes": {
"value": {
"modelConfigDiff": {
"path": "AccountDS.Id"
}
},
"displayValue": {
"modelConfigDiff": {
"path": "AccountDS.Name"
}
}
}
},
"embeddedModel": {
"name": "AccountDS",
"config": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "Account"
}
}
}
}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/
The advanced settings of the collection type attribute let you configure data pagination and sorting.
viewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
"AccountList": {
"isCollection": true,
"modelConfigDiff": {
"path": "AccountDS",
/* Set up pagination.*/
"pagingConfig": {
"rowsOffset"?: "<OffsetAttributeName>",
"rowCount": 5 | "<RowCountAttributeName>"
},
/* Set up collection sorting.*/
"sortingConfig": {
"attributeName": "AccountsSorting",
"default": [{
"columnName": "Name",
"direction": "Asc"
}]
}
},
"viewModelConfigDiff": {...}
}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/
Attributes for direct link columns
Direct link columns are columns that contain objects linked to the current data source. For example, the [Contact]
object contains the [Account]
property, which is also an object. Register the attribute in the [Name]
column of the linked [Account]
object.
To create an attribute for a direct link column:
-
Register the data source in the page schema.
Example that registers a data sourcemodelConfigDiff: /**SCHEMA_MODEL_CONFIG*/{
"dataSources": {
"ContactDS": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "Contact",
}
}
}
}/**SCHEMA_MODEL_CONFIG*/ -
Create a page schema attribute in the
attributes
property of theviewModelConfigDiff
schema section.Example that creates an attributeviewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
"AccountName": {
"modelConfigDiff": {
"path": "ContactDS.AccountName"
}
}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/,The
path
property stores not the path to the column, but the path to the data source attribute that will be created on the next step. Thepath
property value must have the following format:[Data Source Name].[Data Source Attribute Name]
, for example,ContactDS.AccountName
. -
Create an attribute in the
modelConfigDiff
schema section.Example that creates a data source attributemodelConfigDiff: /**SCHEMA_MODEL_CONFIG*/{
"dataSources": {
"ContactDS": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "Contact",
"attributes": {
"AccountName": {
"path": "Account.Name",
"type": "ForwardReference"
}
}
}
}
}
}/**SCHEMA_MODEL_CONFIG*/,ImportantThe name of the data source attribute must match the attribute name specified in the
path
property of theviewModelConfigDiff
schema section on step 2.
You can create a direct link attribute that has multiple nesting levels. For example, you can display the city name of the account linked to the current contact. In this case, the data source attribute can look like this:
"dataSources": {
"ContactDS": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "Contact",
"attributes": {
"AccountCityName": {
"path": "Account.City.Name",
"type": "ForwardReference"
},
}
}
}
}
Embedded model
The Embedded Model is a data model embedded into the view model without being connected to the data source.
Creatio lets you use the embedded model for various collection type elements, for example:
- index of list values
- drop-down list generated from a lookup
Creatio does not display embedded data models in the Freedom UI Designer, nor does it let you bind embedded models to controls on the canvas.
viewModelConfigDiff: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
"LookupAttributeCityList": {
"isCollection": true,
"modelConfigDiff": {
"path": "EmbeddedDS"
},
"embeddedModel" {
"name": "EmbeddedDS",
"config": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "City"
}
}
}
"viewModelConfigDiff": {
"attributes": {
"value": {
"modelConfigDiff": {
"path": "EmbeddedDS.Id"
}
},
"displayValue": {
"modelConfigDiff": {
"path": "EmbeddedDS.Name"
}
}
}
}
}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/,