Set up the condition that locks the field on a page
This example is implemented in the front-end. Learn more about the back-end implementation: Access management (user documentation).
To implement the example:
- Set up the page UI. Read more >>>
- Set up the condition that locks the field. Read more >>>
1. Set up the page UI
-
Create an app based on the Records & business processes template. Instructions: Create an app manually (user documentation).
For this example, create a Requests app.
-
Open the form page in the Freedom UI Designer.
For this example, open the Requests form page.
-
Add a field.
For this example, add the following fields:
- field that contains the applicant
- field that contains the request status
To do this:
-
Add a field of needed type to the working area of the Freedom UI Designer.
-
Click
and fill out the field properties.
Element
Element type
Property
Property value
Field that contains the applicant
Dropdown
Title
Applicant
Code
UsrApplicant
Lookup
Contact
Field that contains the request status
Dropdown
Title
Status
Code
UsrStatus
Lookup
Click
and fill out the lookup properties:
- Set Title to "Request status."
- Set Code to "UsrRequestStatusLookup."
-
Save the changes.
-
Fill out the lookup.
For this example, fill out the Request status lookup.
-
Open the Lookups section. To do this, click
in the top right → System setup → Lookups.
-
Register the lookup for Creatio version 8.0.0. To do this, click New lookup and fill out the lookup properties.
Property
Property value
Name
Request status
Object
Request status
The lookup is registered automatically in Creatio 8.0.1 and later.
-
Open the Request status lookup.
-
Add the lookup values. Instructions: Manage lookup values (user documentation).
For this example, add the following lookup values:
- New
- Under evaluation
- In progress
- Canceled
- Completed
-
2. Set up the condition that locks the field
Configure the business logic in the Client Module Designer. For this example, set up the condition that locks the field.
-
Open the source code of the Freedom UI page. To do this, click
.
-
Add an attribute.
- Go to the
viewModelConfig
schema section →attributes
configuration object. - Add an
IsApplicantReadonly
attribute that stores data about the contact permission to edit the Applicant field.
viewModelConfig schema sectionviewModelConfig: /**SCHEMA_VIEW_MODEL_CONFIG*/[
"attributes": {
...,
/* The attribute that stores the contact permission to edit the "Applicant" field. */
"IsApplicantReadonly": {}
},
...
]/**SCHEMA_VIEW_MODEL_CONFIG*/, - Go to the
-
Bind an attribute to the field.
- Go to the
viewConfigDiff
schema section →UsrApplicant
element. - Bind the
IsApplicantReadonly
attribute to thereadonly
property.
viewConfigDiff schema sectionviewConfigDiff: /**SCHEMA_VIEW_CONFIG_DIFF*/[
...,
/* Field that contains the applicant. */
{
"operation": "insert",
"name": "UsrApplicant",
"values": {
...,
/* The property that locks the field from editing. Bound to the "IsApplicantReadonly" attribute. */
"readonly": "$IsApplicantReadonly"
},
...
}
]/**SCHEMA_VIEW_CONFIG_DIFF*/, - Go to the
-
Implement the base request handler.
-
Go to the
handlers
schema section. -
Add a custom implementation of the
crt.HandleViewModelAttributeChangeRequest
base request handler.- Run the handler when the value of any attribute changes, including changes made after loading the attribute values from the data source.
- Find the ID of the "Completed" value in the Request status lookup. For this example, the ID is "dc326b2e-264d-45d0-bbf5-ea8ea1ce5e0c."
- Save the ID from the previous step to the
completedStatusId
constant. - Check the
IsApplicantReadonly
attribute value. If the new attribute value refers to the "Completed" request status, set theIsApplicantReadonly
attribute value totrue
, otherwise set it tofalse
. I. e., if the request is completed, the page locks the Applicant field. Otherwise, the page keeps the field editable for other request statuses.
handlers schema sectionhandlers: /**SCHEMA_HANDLERS*/[
{
request: "crt.HandleViewModelAttributeChangeRequest",
/* The custom implementation of the system request handler. */
handler: async (request, next) => {
/* Check the request status. */
if (request.attributeName === 'UsrStatus') {
const completedStatusId = 'dc326b2e-264d-45d0-bbf5-ea8ea1ce5e0c';
const selectedStatus = await request.$context.UsrStatus;
const selectedStatusId = selectedStatus?.value;
const isRequestCompleted = selectedStatusId === completedStatusId;
/* If the request status is "Completed", set the "IsApplicantReadonly" to "true." */
request.$context.IsApplicantReadonly = isRequestCompleted;
}
/* Call the next handler if it exists and return its result. */
return next?.handle(request);
}
}
]/**SCHEMA_HANDLERS*/, -
-
Save the changes.
View the result
- Open the Requests section.
- Create a request that has an arbitrary name. For example, "Request's name."
- Select an arbitrary applicant in the Applicant field. For example, "Bruce Clayton."
- Select "Completed" in the Status field.
As a result:
- Creatio will lock the Applicant field for completed requests. View the result >>>
- Creatio will keep the Applicant field editable for other request statuses, e. g., "New."
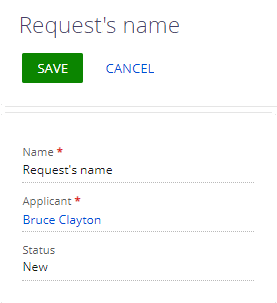
Source code
/* Declare the AMD module. */
define("UsrAppRequests_FormPage", /**SCHEMA_DEPS*/[]/**SCHEMA_DEPS*/, function/**SCHEMA_ARGS*/()/**SCHEMA_ARGS*/ {
return {
viewConfigDiff: /**SCHEMA_VIEW_CONFIG_DIFF*/[
{
"operation": "insert",
"name": "UsrName",
"values": {
"layoutConfig": {
"column": 1,
"row": 1,
"colSpan": 1,
"rowSpan": 1
},
"type": "crt.Input",
"label": "$Resources.Strings.UsrName",
"control": "$UsrName"
},
"parentName": "LeftAreaProfileContainer",
"propertyName": "items",
"index": 0
},
/* Field that contains the applicant. */
{
"operation": "insert",
"name": "UsrApplicant",
"values": {
"layoutConfig": {
"column": 1,
"row": 2,
"colSpan": 1,
"rowSpan": 1
},
"type": "crt.ComboBox",
"loading": false,
"control": "$UsrApplicant",
"label": "$Resources.Strings.UsrApplicant",
"items": "$UsrApplicant_List",
"attributeName": "UsrApplicant",
"isAddAllowed": true,
"showValueAsLink": true,
/* The property that locks the field from editing. Bound to the "IsApplicantReadonly" attribute. */
"readonly": "$IsApplicantReadonly"
},
"parentName": "LeftAreaProfileContainer",
"propertyName": "items",
"index": 1
},
/* Field that contains the request status. */
{
"operation": "insert",
"name": "UsrStatus",
"values": {
"layoutConfig": {
"column": 1,
"row": 3,
"colSpan": 1,
"rowSpan": 1
},
"type": "crt.ComboBox",
"loading": false,
"control": "$UsrStatus",
"label": "$Resources.Strings.UsrStatus",
"items": "$UsrStatus_List",
"attributeName": "UsrStatus",
"isAddAllowed": true,
"showValueAsLink": true
},
"parentName": "LeftAreaProfileContainer",
"propertyName": "items",
"index": 2
}
]/**SCHEMA_VIEW_CONFIG_DIFF*/,
viewModelConfig: /**SCHEMA_VIEW_MODEL_CONFIG*/{
"attributes": {
"UsrName": {
"modelConfig": {
"path": "PDS.UsrName"
}
},
"Id": {
"modelConfig": {
"path": "PDS.Id"
}
},
"UsrApplicant": {
"modelConfig": {
"path": "PDS.UsrApplicant"
}
},
"UsrApplicant_List": {
"isCollection": true,
"viewModelConfig": {
"attributes": {
"value": {
"modelConfig": {
"path": "UsrApplicant_List_DS.Id"
}
},
"displayValue": {
"modelConfig": {
"path": "UsrApplicant_List_DS.Name"
}
}
}
},
"modelConfig": {
"path": "UsrApplicant_List_DS",
"sortingConfig": {
"attributeName": "UsrApplicant_List_Sorting",
"default": [
{
"columnName": "Name",
"direction": "asc"
}
]
},
"pagingConfig": {
"rowCount": 50,
"rowsOffset": null
}
},
"embeddedModel": {
"name": "UsrApplicant_List_DS",
"config": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "Contact"
}
}
}
},
"UsrApplicant_List_Sorting": {
"isCollection": true,
"viewModelConfig": {
"attributes": {
"columnName": {},
"direction": {}
}
}
},
"UsrStatus": {
"modelConfig": {
"path": "PDS.UsrStatus"
}
},
"UsrStatus_List": {
"isCollection": true,
"viewModelConfig": {
"attributes": {
"value": {
"modelConfig": {
"path": "UsrStatus_List_DS.Id"
}
},
"displayValue": {
"modelConfig": {
"path": "UsrStatus_List_DS.Name"
}
}
}
},
"modelConfig": {
"path": "UsrStatus_List_DS",
"sortingConfig": {
"attributeName": "UsrStatus_List_Sorting",
"default": [
{
"columnName": "Name",
"direction": "asc"
}
]
},
"pagingConfig": {
"rowCount": 50,
"rowsOffset": null
}
},
"embeddedModel": {
"name": "UsrStatus_List_DS",
"config": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "UsrRequestStatusLookup"
}
}
}
},
"UsrStatus_List_Sorting": {
"isCollection": true,
"viewModelConfig": {
"attributes": {
"columnName": {},
"direction": {}
}
}
},
/* The attribute that stores the contact permission to edit the "Applicant" field. */
"IsApplicantReadonly": {}
}
}/**SCHEMA_VIEW_MODEL_CONFIG*/,
modelConfig: /**SCHEMA_MODEL_CONFIG*/{
"dataSources": {
"PDS": {
"type": "crt.EntityDataSource",
"config": {
"entitySchemaName": "UsrAppRequests"
}
}
}
}/**SCHEMA_MODEL_CONFIG*/,
handlers: /**SCHEMA_HANDLERS*/[
{
request: "crt.HandleViewModelAttributeChangeRequest",
/* The custom implementation of the system request handler. */
handler: async (request, next) => {
/* Check the request status. */
if (request.attributeName === 'UsrStatus') {
const completedStatusId = 'dc326b2e-264d-45d0-bbf5-ea8ea1ce5e0c';
const selectedStatus = await request.$context.UsrStatus;
const selectedStatusId = selectedStatus?.value;
const isRequestCompleted = selectedStatusId === completedStatusId;
/* If the request status is "Completed", set the "IsApplicantReadonly" to "true." */
request.$context.IsApplicantReadonly = isRequestCompleted;
}
/* Call the next handler if it exists and return its result. */
return next?.handle(request);
}
}
]/**SCHEMA_HANDLERS*/,
converters: /**SCHEMA_CONVERTERS*/{}/**SCHEMA_CONVERTERS*/,
validators: /**SCHEMA_VALIDATORS*/{}/**SCHEMA_VALIDATORS*/
};
});