Create a mini page that adds records
Create a custom mini page that adds a new record to the Products section. The mini page must contain the base set of Name and Code fields.
1. Create a view model schema of the mini page
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Page view model on the section list toolbar.
-
Fill out the schema properties in the Schema Designer:
- Set Code to "UsrProductMiniPage."
- Set Title to "Product Mini Page."
- Set Parent object to "BaseMiniPage."
Click Apply to apply the properties.
2. Display the fields of the primary object
Add the needed source code in the Schema Designer.
-
Specify the
Product
schema as the object schema. -
Declare the
MiniPageModes
attribute. Assign the array that has the collection of the needed mini page operations to the attribute.noteIf you also need to display the mini page on the section page (see Create a custom mini page), add
this.Terrasoft.ConfigurationEnums.CardOperation.VIEW
to the array assigned to theMiniPageModes
attribute -
Add the needed modifications to the
diff
array of view model modifications.The view model elements of the base mini page are as follows:
MiniPage
is the page field.HeaderContainer
is the page heading. By default, the mini page places it in the first row of the field.
In this example, the
diff
modification array contains two new objects that configure the Name and Code fields.
View the source code of the view model schema below.
define("UsrProductMiniPage", ["UsrProductMiniPageResources"], function(resources) {
return {
entitySchemaName: "Product",
details: /**SCHEMA_DETAILS*/{}/**SCHEMA_DETAILS*/,
attributes: {
"MiniPageModes": {
"value": [this.Terrasoft.ConfigurationEnums.CardOperation.ADD]
}
},
diff: /**SCHEMA_DIFF*/[
{
"operation": "insert",
"parentName": "MiniPage",
"propertyName": "items",
"name": "Name",
"values": {
"isMiniPageModelItem": true,
"layout": {
"column": 0,
"row": 1,
"colSpan": 24
},
"controlConfig": {
"focused": true
}
}
},
{
"operation": "insert",
"parentName": "MiniPage",
"propertyName": "items",
"name": "Code",
"values": {
"isMiniPageModelItem": true,
"layout": {
"column": 0,
"row": 2,
"colSpan": 24
}
}
}
]/**SCHEMA_DIFF*/
};
});
3. Register the mini page in the database
Register new mini pages in the database. Run the following SQL query to do that.
DECLARE
-- The name of the mini page view schema.
@ClientUnitSchemaName NVARCHAR(100) = 'UsrProductMiniPage',
-- The name of the object schema to bind the mini page.
@EntitySchemaName NVARCHAR(100) = 'Product'
UPDATE SysModuleEdit
SET MiniPageSchemaUId = (
SELECT TOP 1 UId
FROM SysSchema
WHERE Name = @ClientUnitSchemaName
)
WHERE SysModuleEntityId = (
SELECT TOP 1 Id
FROM SysModuleEntity
WHERE SysEntitySchemaUId = (
SELECT TOP 1 UId
FROM SysSchema
WHERE Name = @EntitySchemaName
AND ExtendParent = 0
)
);
As a result of the query, Creatio will add the unique mini page identifier to the [MiniPageSchemaUId]
field of the [SysModuleEdit]
table record that corresponds to the Products section.

4. Add the system setting
Add the system setting that has the following properties to the System settings section of the System Designer:
- Set Name to "HasProductMiniPageAddMode."
- Set Code to "HasProductMiniPageAddMode."
- Set Type to "Boolean."
- Set Default value to selected checkbox.
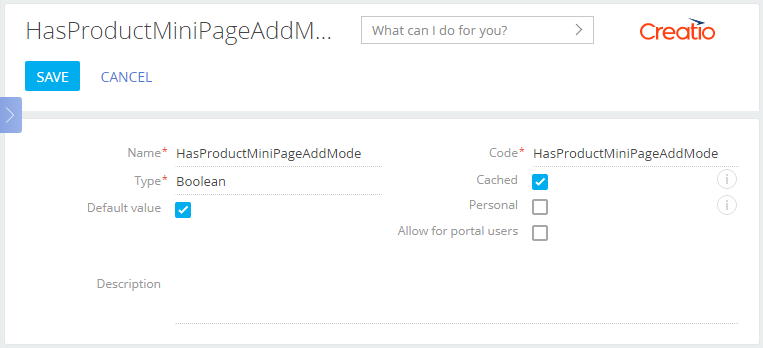
Outcome of the example
As a result, Creatio will display the mini page with two fields when you add a new product.
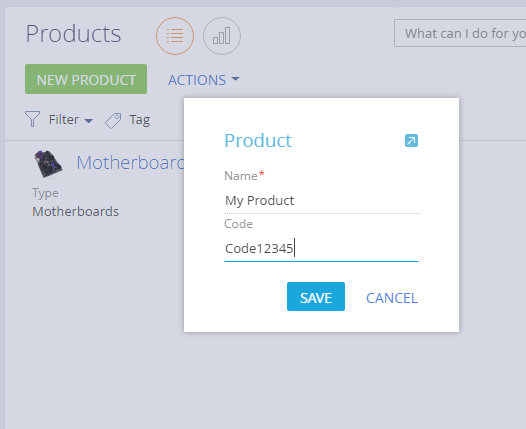
After you save the mini page, the corresponding record will appear in the section list.
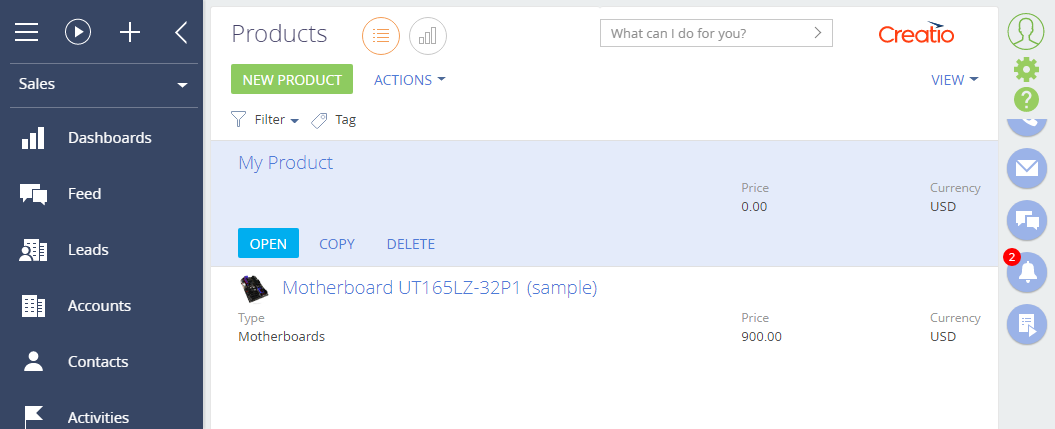
Creatio will display the record in the section list only after you refresh the browser page. If you want Creatio to display the record immediately after you save the mini page, add the corresponding functionality to the mini page and section page schemas via the message mechanism. Learn more in a separate article: Module message exchange.