Create a custom mini page
Create a custom mini page for the Knowledge base section. The mini page must contain the base set of Name and Tags fields and let you download attachments.
1. Create a view model schema of the mini page
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Page view model on the section list toolbar.
-
Fill out the schema properties in the Schema Designer:
- Set Code to "UsrKnowledgeBaseArticleMiniPage."
- Set Title to "KnowledgeBase Mini Page."
- Set Parent object to "BaseMiniPage."
Click Apply to apply the properties.
2. Display the fields of the primary object
Add the needed source code in the Schema Designer.
-
Specify the
KnowledgeBase
schema as the object schema. -
Add the needed modifications to the
diff
array of view model modifications.The view model elements of the base mini page are as follows:
MiniPage
is the page field.HeaderContainer
is the page heading. By default, the mini page places it in the first row of the field.
In this example, the
diff
modification array contains two new objects that configure the Name and Keywords fields.
View the source code of the view model schema below.
define("UsrKnowledgeBaseArticleMiniPage", [], function() {
return {
entitySchemaName: "KnowledgeBase",
attributes: {
"MiniPageModes": {
"value": [this.Terrasoft.ConfigurationEnums.CardOperation.VIEW]
}
},
diff: /**SCHEMA_DIFF*/[
{
"operation": "insert",
"name": "Name",
"parentName": "HeaderContainer",
"propertyName": "items",
"index": 0,
"values": {
"labelConfig": {
"visible": false
},
"isMiniPageModelItem": true
}
},
{
"operation": "insert",
"name": "Keywords",
"parentName": "MiniPage",
"propertyName": "items",
"values": {
"labelConfig": {
"visible": false
},
"isMiniPageModelItem": true,
"layout": {
"column": 0,
"row": 1,
"colSpan": 24
}
}
}
]/**SCHEMA_DIFF*/
};
});
3. Add a functional button to the mini page
As per the example conditions, the mini page must let you download the files bound to the knowledge base article.
To implement the management of additional data, display the data as a drop-down list of a preconfigured button.
Modify the source code of the view model in the Schema Designer.
To add a button that selects the files of the knowledge base article:
- Add the
FilesButton
element, which is the button's description, to thediff
array. - Add the
Article
virtual column that connects the main and additional records to theattributes
property. - Add the
MiniPageModes
attribute that represents the array of the needed mini page operations to theattributes
property. - Add the button image to schema resources. For example, use the following image:
. Learn more about adding images to resources in a separate article: Add an image field to a record page.
- Add methods that manage the drop-down list of the file selection button to the
methods
property:init()
is an overridden base method.onEntityInitialized()
is an overridden base method.setArticleInfo()
sets the value of theArticle
attribute.getFiles(callback, scope)
retrieves the data about the files of the current knowledge base article.initFilesMenu(files)
populates the collection of the file selection button's drop-down list.fillFilesExtendedMenuData()
initiates the upload of files and the addition of them to the file selection button's drop-down list.downloadFile()
initiates the download of the selected file.
View the view model schema's source code that adds the functional button below.
define("UsrKnowledgeBaseArticleMiniPage", ["terrasoft", "KnowledgeBaseFile", "ConfigurationConstants"], function(Terrasoft, KnowledgeBaseFile, ConfigurationConstants) {
return {
entitySchemaName: "KnowledgeBase",
attributes: {
"MiniPageModes": {
"value": [this.Terrasoft.ConfigurationEnums.CardOperation.VIEW]
},
"Article": {
"type": Terrasoft.ViewModelColumnType.VIRTUAL_COLUMN,
"referenceSchemaName": "KnowledgeBase"
}
},
methods: {
/* Initialize the collection of the file selection button's dropdown list.*/
init: function() {
this.callParent(arguments);
this.initExtendedMenuButtonCollections("File", ["Article"], this.close);
},
/* Initialize the value of the attribute that binds the main and additional records.
Populate the collection of the file selection button's drop-down list.*/
onEntityInitialized: function() {
this.callParent(arguments);
this.setArticleInfo();
this.fillFilesExtendedMenuData();
},
/* Initiate the upload of files and the addition of them to the file selection button's drop-down list.*/
fillFilesExtendedMenuData: function() {
this.getFiles(this.initFilesMenu, this);
},
/* Set the value of the attribute that binds the main and additional records.*/
setArticleInfo: function() {
this.set("Article", {
value: this.get(this.primaryColumnName),
displayValue: this.get(this.primaryDisplayColumnName)
});
},
/* Retrieve the data about the files of the current knowledge base article.*/
getFiles: function(callback, scope) {
var esq = this.Ext.create("Terrasoft.EntitySchemaQuery", {
rootSchema: KnowledgeBaseFile
});
esq.addColumn("Name");
var articleFilter = >this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.EQUAL, "KnowledgeBase", this.get(this.primaryColumnName));
var typeFilter = this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.EQUAL, "Type", ConfigurationConstants.FileType.File);
esq.filters.addItem(articleFilter);
esq.filters.addItem(typeFilter);
esq.getEntityCollection(function(response) {
if (!response.success) {
return;
}
callback.call(scope, response.collection);
}, this);
},
/* Populate the collection of the file selection button's drop-down list.*/
initFilesMenu: function(files) {
if (files.isEmpty()) {
return;
}
var data = [];
files.each(function(file) {
data.push({
caption: file.get("Name"),
tag: file.get("Id")
});
}, this);
var recipientInfo = this.fillExtendedMenuItems("File", ["Article"]);
this.fillExtendedMenuData(data, recipientInfo, this.downloadFile);
},
/* Initiate the download of the selected file.*/
downloadFile: function(id) {
var element = document.createElement("a");
element.href = "../rest/FileService/GetFile/" + KnowledgeBaseFile.uId + "/" + id;
document.body.appendChild(element);
element.click();
document.body.removeChild(element);
}
},
diff: /**SCHEMA_DIFF*/[
{
"operation": "insert",
"name": "Name",
"parentName": "HeaderContainer",
"propertyName": "items",
"index": 0,
"values": {
"labelConfig": {
"visible": true
},
"isMiniPageModelItem": true
}
},
{
"operation": "insert",
"name": "Keywords",
"parentName": "MiniPage",
"propertyName": "items",
"values": {
"labelConfig": {
"visible": true
},
"isMiniPageModelItem": true,
"layout": {
"column": 0,
"row": 1,
"colSpan": 24
}
}
},
{
"operation": "insert",
"parentName": "HeaderContainer",
"propertyName": "items",
"name": "FilesButton",
"values": {
"itemType": Terrasoft.ViewItemType.BUTTON,
/* Set up the button image.*/
"imageConfig": {
/* Add the image to the mini page resources before you run the code.*/
"bindTo": "Resources.Images.FilesImage"
},
/* Set up the drop-down list.*/
"extendedMenu": {
/* The name of the drop-down list element.*/
"Name": "File",
/* The name of the mini page attribute that binds the main and additional records.*/
"PropertyName": "Article",
/* Set up the button click processor.*/
"Click": {
"bindTo": "fillFilesExtendedMenuData"
}
}
},
"index": 1
}
]/**SCHEMA_DIFF*/
};
});
4. Apply styles to the mini page
To add styles to the view model, create an individual style module and enable it in the view model schema.
-
Go to the Configuration section and select a user-made package to add the schema.
-
Click Add → Module on the section list toolbar.
-
Fill out the schema properties in the Schema Designer:
- Set Code to "UsrKnowledgeBaseArticleMiniPageCss."
- Set Title to "Knowledge base minipage styles."
Click Apply to apply the properties.
-
Specify the needed styles in the context menu of the LESS node.
UsrKnowledgeBaseArticleMiniPageCss.jsdiv[data-item-marker="UsrKnowledgeBaseArticleMiniPageContainer"] > div {
width: 250px;
} -
Add the module loading to the source code of the view model schema in the Schema Designer.
View the full source code of the mini page below:
UsrKnowledgeBaseArticleMiniPage.jsdefine("UsrKnowledgeBaseArticleMiniPage", ["terrasoft", "KnowledgeBaseFile", "ConfigurationConstants", "css!UsrKnowledgeBaseArticleMiniPageCss"], function(Terrasoft, KnowledgeBaseFile, ConfigurationConstants) {
return {
entitySchemaName: "KnowledgeBase",
attributes: {
"MiniPageModes": {
"value": [this.Terrasoft.ConfigurationEnums.CardOperation.VIEW]
},
"Article": {
"type": Terrasoft.ViewModelColumnType.VIRTUAL_COLUMN,
"referenceSchemaName": "KnowledgeBase"
}
},
methods: {
init: function() {
this.callParent(arguments);
this.initExtendedMenuButtonCollections("File", ["Article"], this.close);
},
onEntityInitialized: function() {
this.callParent(arguments);
this.setArticleInfo();
this.fillFilesExtendedMenuData();
},
fillFilesExtendedMenuData: function() {
this.getFiles(this.initFilesMenu, this);
},
setArticleInfo: function() {
this.set("Article", {
value: this.get(this.primaryColumnName),
displayValue: this.get(this.primaryDisplayColumnName)
});
},
getFiles: function(callback, scope) {
var esq = this.Ext.create("Terrasoft.EntitySchemaQuery", {
rootSchema: KnowledgeBaseFile
});
esq.addColumn("Name");
var articleFilter = this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.EQUAL, "KnowledgeBase", this.get(this.primaryColumnName));
var typeFilter = this.Terrasoft.createColumnFilterWithParameter(
this.Terrasoft.ComparisonType.EQUAL, "Type", ConfigurationConstants.FileType.File);
esq.filters.addItem(articleFilter);
esq.filters.addItem(typeFilter);
esq.getEntityCollection(function(response) {
if (!response.success) {
return;
}
callback.call(scope, response.collection);
}, this);
},
initFilesMenu: function(files) {
if (files.isEmpty()) {
return;
}
var data = [];
files.each(function(file) {
data.push({
caption: file.get("Name"),
tag: file.get("Id")
});
}, this);
var recipientInfo = this.fillExtendedMenuItems("File", ["Article"]);
this.fillExtendedMenuData(data, recipientInfo, this.downloadFile);
},
downloadFile: function(id) {
var element = document.createElement("a");
element.href = "../rest/FileService/GetFile/" + KnowledgeBaseFile.uId + "/" + id;
document.body.appendChild(element);
element.click();
document.body.removeChild(element);
}
},
diff: /**SCHEMA_DIFF*/[
{
"operation": "insert",
"name": "Name",
"parentName": "HeaderContainer",
"propertyName": "items",
"index": 0,
"values": {
"labelConfig": {
"visible": true
},
"isMiniPageModelItem": true
}
},
{
"operation": "insert",
"name": "Keywords",
"parentName": "MiniPage",
"propertyName": "items",
"values": {
"labelConfig": {
"visible": true
},
"isMiniPageModelItem": true,
"layout": {
"column": 0,
"row": 1,
"colSpan": 24
}
}
},
{
"operation": "insert",
"parentName": "HeaderContainer",
"propertyName": "items",
"name": "FilesButton",
"values": {
"itemType": Terrasoft.ViewItemType.BUTTON,
"imageConfig": {
"bindTo": "Resources.Images.FilesImage"
},
"extendedMenu": {
"Name": "File",
"PropertyName": "Article",
"Click": {
"bindTo": "fillFilesExtendedMenuData"
}
}
},
"index": 1
}
]/**SCHEMA_DIFF*/
};
});
5. Register the mini page in the database
You must register new mini pages in the database. Run the following SQL query to modify the database:
DECLARE
-- The name of the mini page view schema.
@ClientUnitSchemaName NVARCHAR(100) = 'UsrKnowledgeBaseArticleMiniPage',
-- The name of the object schema to bind the mini page.
@EntitySchemaName NVARCHAR(100) = 'KnowledgeBase'
UPDATE SysModuleEdit
SET MiniPageSchemaUId = (
SELECT TOP 1 UId
FROM SysSchema
WHERE Name = @ClientUnitSchemaName
)
WHERE SysModuleEntityId = (
SELECT TOP 1 Id
FROM SysModuleEntity
WHERE SysEntitySchemaUId = (
SELECT TOP 1 UId
FROM SysSchema
WHERE Name = @EntitySchemaName
AND ExtendParent = 0
)
);
As a result of the query, Creatio will add a unique mini page identifier to the [MiniPageSchemaUId]
field of the [SysModuleEdit]
table record that corresponds to the Knowledge base section.

6. Add the system setting
Add the system setting that has the following properties to the System settings section of the System Designer:
- Set Name to "HasKnowledgeBaseMiniPageAddMode."
- Set Code to "HasKnowledgeBaseMiniPageAddMode."
- Set Type to "Boolean."
- Set Default value to selected checkbox.
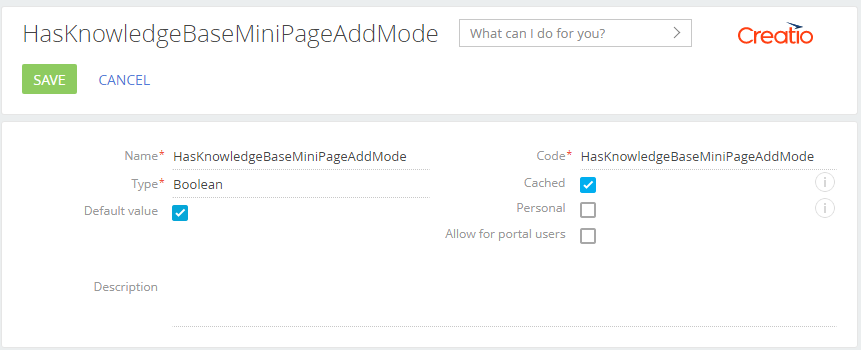
Outcome of the example
After you save the schema and refresh the Creatio web page, hover over the article name in the Knowledge base section to bring up a custom mini page that displays the files bound to the record. You will be able to download the files.
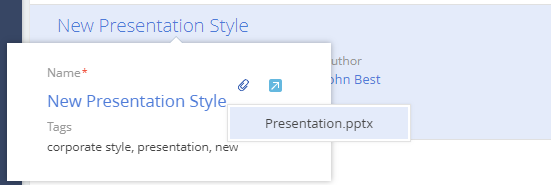