Implement email template using custom macro
To implement the example:
- Implement a custom macro. Read more >>>
- Register the custom macro in the database. Read more >>>
- Set up the email template. Read more >>>
Implement the custom "Notification. New documentation release" email template. The email template must include the following text:
Greetings! I hope you're doing well. At Creatio, we are committed to empowering our customers with industry-leading product innovations for workflow automation, no-code app development, and CRM. We are introducing updated documentation. You can access the documentation here: Creatio documentation. Best regards, Creatio |
1. Implement a custom macro
-
Open the Configuration section. Instructions: Open the Configuration section.
-
Create a package. Instructions: Create a user-made package using Configuration section.
For this example, create the
sdkAddCustomEmailMacro
package. -
Create the source code schema. To do this, click Add → Source code.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Code
UsrCompanyName
Title
CompanyName
-
Apply the changes.
-
Implement the business logic of the email macro.
UsrCompanyNamenamespace Terrasoft.Configuration
{
using System;
using Terrasoft.Core;
/* Class that implements the "IMacrosInvokable" interface. */
public class UsrCompanyName : IMacrosInvokable
{
/* A user connection. */
public UserConnection UserConnection
{
get;
set;
}
/* Implement the "GetMacrosValue()" method of the "IMacrosInvokable" interface. */
public string GetMacrosValue(object arguments)
{
/* Method that returns string value for macros. */
return "Creatio";
}
}
} -
Publish the schema.
2. Register the custom macro in the database
To do this, execute the SQL query to the EmailTemplateMacros
database table that stores the email templates.
INSERT INTO EmailTemplateMacros(Name, Parentid, ColumnPath)
VALUES (
'UsrCompanyName',
(SELECT TOP 1 Id
FROM EmailTemplateMacros
WHERE Name = '@Invoke'),
'Terrasoft.Configuration.UsrCompanyName'
)
3. Set up the email template
-
Open the Message templates section in Creatio.
-
Create a custom email template.
-
Click New → Email template.
-
Fill out the properties of the email template.
Property
Property value
Template name
Notification. New documentation release
Subject
New documentation release
Email template
- Click Edit.
- Set up the content of the email template. Instructions: Work with message templates (user documentation).
- Replace the "Creatio" company name using
[#@Invoke.UsrCompanyName#]
macro. - Save the changes.
-
As a result, the custom "Notification. New documentation release" email template will be as follows.
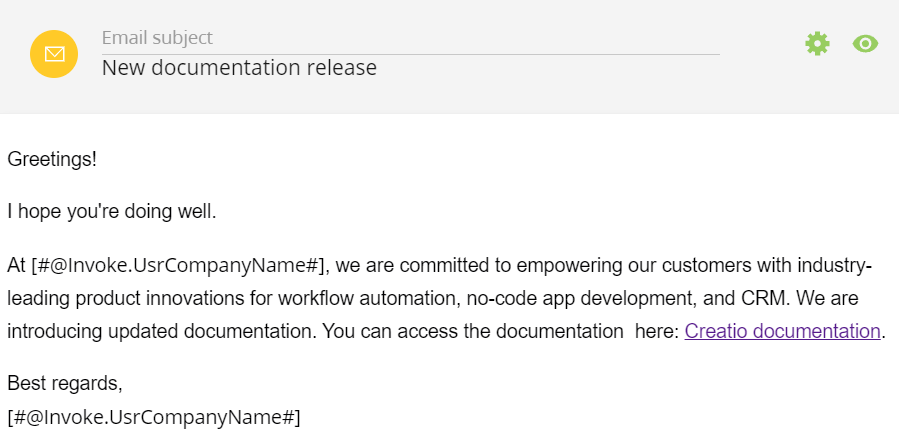
View the result
-
Open the Communication panel.
-
Open the Emails panel.
-
Click
. This opens the New Email window.
-
Insert the email template.
- Click
. This opens the Select: Message template window.
- Select the "Notification. New documentation release" email template.
- Click Select.
- Click
As a result, the email will include the custom "Notification. New documentation release" email template. The company name will be replaced by custom macro value. View the result >>>
Source code
- UsrCompanyName
- SQL query
namespace Terrasoft.Configuration
{
using System;
using Terrasoft.Core;
/* Class that implements the "IMacrosInvokable" interface. */
public class UsrCompanyName : IMacrosInvokable
{
/* A user connection. */
public UserConnection UserConnection
{
get;
set;
}
/* Implement the "GetMacrosValue()" method of the "IMacrosInvokable" interface. */
public string GetMacrosValue(object arguments)
{
/* Method that returns string value for macros. */
return "Creatio";
}
}
}
INSERT INTO EmailTemplateMacros(Name, Parentid, ColumnPath)
VALUES (
'UsrCompanyName',
(SELECT TOP 1 Id
FROM EmailTemplateMacros
WHERE Name = '@Invoke'),
'Terrasoft.Configuration.UsrCompanyName'
)