Send email using business process and explicit account credentials
To implement the example:
- Create a business process. Read more >>>
- Implement a separate page to fill out the email parameters. Read more >>>
- Set up the email template. Read more >>>
- Set up the business process parameters. Read more >>>
- Set up the business process methods. Read more >>>
Create a "Send emails using explicit account credentials" business process that generates a "Fill out parameters to send email" page. The page sends an email that has the following parameters using an existing account:
- Sender that contains the name of email sender. Required.
- Sender mailbox that contains the mailbox of email sender. Required.
- Mailbox password that contains the password to the mailbox of email sender. Required.
- Mail server that contains the mail server address of the email sender. Required.
- Type of mail server that contains the type of the email sender's mail server. Required.
- Port that contains the port number of the email sender's mail server
- Use SSL that contains information about using a cryptographic protocol to provide secure connection
- Recipient (many recipients separated by semicolon ";") that contains the list of the recipient mailboxes. Required.
- Subject that contains the email subject. Required.
- Body that contains the email body. Required.
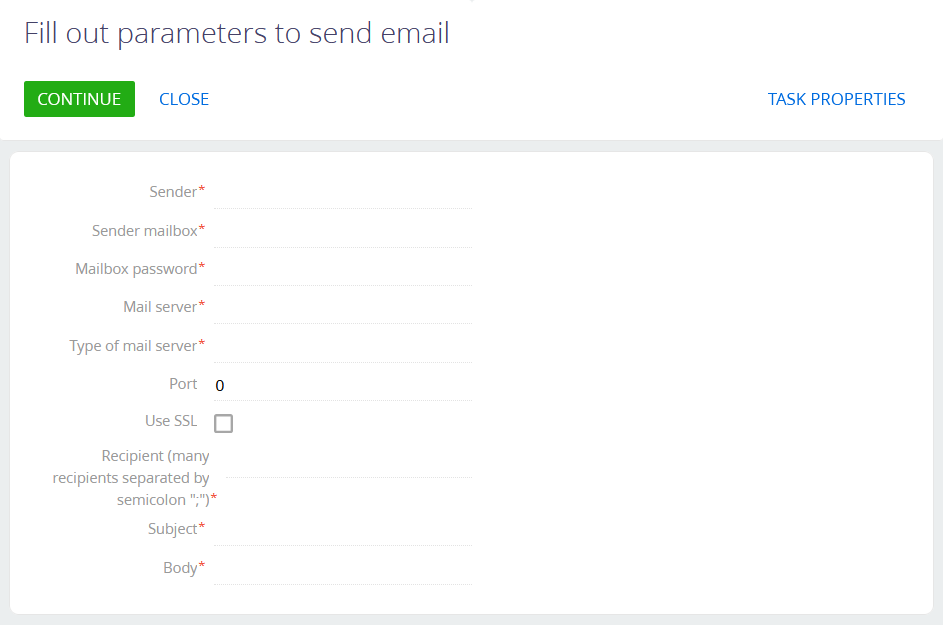
1. Create a business process
-
Open the Configuration section. Instructions: Open the Configuration section.
-
Create a package. Instructions: Create a user-made package using Configuration section.
For this example, create the
sdkSendEmailWithExplicitCredentials
package. -
Add the package properties.
- Open the package properties. To do this, click
→ Properties. This opens the Dependencies tab on the Package properties page.
- Click Add in the Depends on Packages block. This opens the Select package window.
- Select the checkbox for the
CrtCoreBase
package. TheCrtCoreBase
package includes theEmailContract.dll
external assembly. - Click Select.
- Apply the changes.
- Add the
CrtBaseConsts
package similarly. TheCrtBaseConsts
package includes theIntegrationApi.dll
external assembly.
- Open the package properties. To do this, click
-
Change the current package. Instructions: Change the current package.
For this example, change the current package to
sdkSendEmailWithExplicitCredentials
user-made package. -
Create the business process schema. To do this, click Add → Business process.
-
Open the Settings tab.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Title
Send emails using explicit account credentials
Code
UsrSendEmailUsingCredentialsProcess
-
Save the changes.
As a result:
- The "Send emails using explicit account credentials" business process will be created.
- Creatio will add the "Send emails using explicit account credentials" business process to the Process library section.
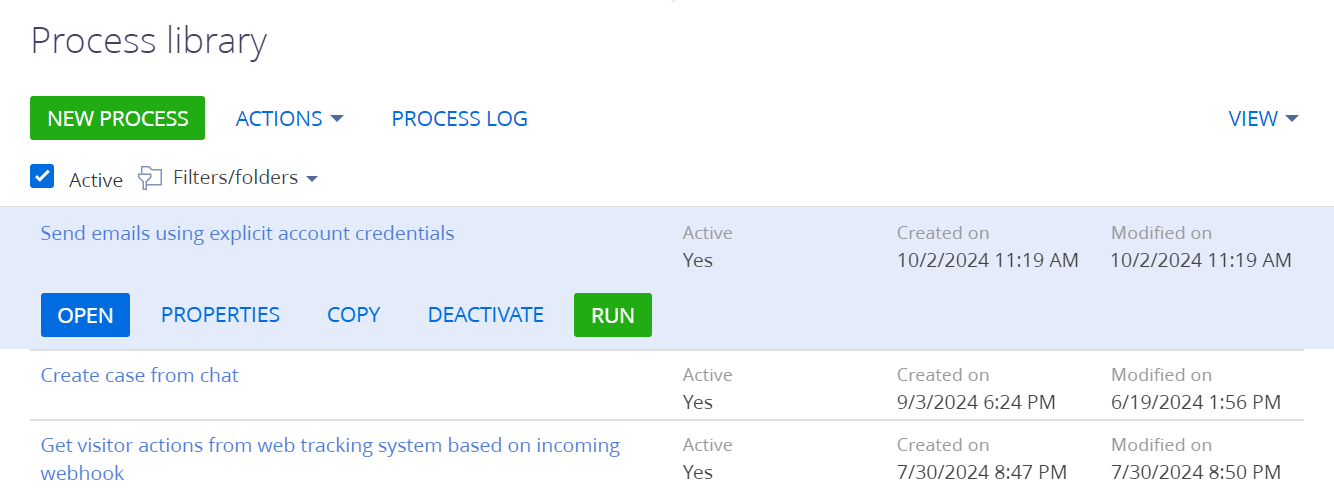
2. Implement a separate page to fill out the email parameters
-
Add an auto-generated page.
-
Click
→ place the Auto-generated page element between the Simple and Terminate page elements in the working area of the Process Designer.
-
Fill out the element properties.
Property
Property value
Title
Fill out the email parameters
Page title
Fill out parameters to send email
Creatio populates the Contact property using the "[#System variable.Current user contact#]" value.
-
-
Add a button.
For this example, add a button that sends the email. To do this:
-
Go to the Buttons block.
-
Click
and fill out the button properties.
Property
Property value
Caption
Continue
Code
ContinueButton
Style
Green
-
Save the changes.
As a result, the Buttons property block of the "Fill out the email parameters" auto-generated page will be as follows.
-
-
Add the email parameters.
For this example, add the following parameters:
- parameter that contains the name of email sender
- parameter that contains the mailbox of email sender
- parameter that contains the password to the mailbox of email sender
- parameter that contains the mail server address of the email sender
- parameter that contains the type of the email sender's mail server
- parameter that contains the port number of the email sender's mail server
- parameter that contains information about using a cryptographic protocol to provide secure connection
- parameter that contains the list of the recipient mailboxes
- parameter that contains the email subject
- parameter that contains the email body
To do this:
-
Go to the Page Items block.
-
Click
and select a parameter of the needed type.
-
Fill out the parameter properties.
Element
Element type
Property
Property value
Parameter that contains the name of email sender
Text field
Title
Sender
Code
Sender
Required
Select the checkbox
Parameter that contains the mailbox of email sender
Text field
Title
Sender mailbox
Code
SenderMailbox
Required
Select the checkbox
Parameter that contains the password to the mailbox of email sender
Text field
Title
Mailbox password
Code
MailboxPassword
Required
Select the checkbox
Parameter that contains the mail server address of the email sender
Text field
Title
Mail server
Code
MailServer
Required
Select the checkbox
Parameter that contains the type of the email sender's mail server
Selection field
Title
Type of mail server
Code
MailServerType
Required
Select the checkbox
Data source
Mail service provider type
View
Drop down list
Parameter that contains the port number of the email sender's mail server
Integer
Title
Port
Code
Port
Parameter that contains information about using a cryptographic protocol to provide secure connection
Boolean
Title
Use SSL
Code
UseSsl
Parameter that contains the list of the recipient mailboxes
Text field
Title
Recipient (many recipients separated by semicolon ";")
Code
Recipient
Required
Select the checkbox
Parameter that contains the email subject
Text field
Title
Subject
Code
Subject
Required
Select the checkbox
Parameter that contains the email body
Text field
Title
Body
Code
Body
Required
Select the checkbox
As a result, the Page Items property block of the "Fill out the email parameters" auto-generated page will be as follows.
3. Implement sending emails
-
Add a Script task element. To do this, click
→ place the Script task element between the Auto-generated page user action and Terminate page element in the working area of the Process Designer.
-
Fill out the element properties.
Property
Property value
Title
Send email
-
Implement the logic of working with process parameters. To do this, go to the element setup area and add the source code.
Send email script task// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an instance of the "EmailClientFactory." */
var emailClientFactory = ClassFactory.Get<EmailClientFactory>(new ConstructorArgument("userConnection", UserConnection));
/* Set the connection settings for the mail server. */
var credentialConfig = new EmailContract.DTO.Credentials {
MailServer = Get<string>("MailServer"),
Port = Get<int>("Port"),
UseSsl = Get<bool>("UseSsl"),
Sender = Get<string>("Sender"),
MailboxPassword = Get<string>("MailboxPassword"),
MailServerType = Get<Guid>("MailServerType"),
SenderEmailAddress = Get<string>("SenderMailbox")
};
/* Create an instance of the "IEmailSender." */
var emailSender = ClassFactory.Get<IEmailSender>(new ConstructorArgument("emailClientFactory", emailClientFactory),
new ConstructorArgument("userConnection", UserConnection));
/* Fill out the email parameters. */
var message = new EmailContract.DTO.Email {
Sender = credentialConfig.SenderEmailAddress,
Recipients = Get<string>("Recipient").Split(';').ToList<string>(),
Subject = Get<string>("Subject"),
Body = Get<string>("Body"),
Importance = EmailContract.EmailImportance.Normal,
/* Whether the email body is in HTML format. */
IsHtmlBody = true,
/* List of the recipient mailboxes to send copy of the email. Optional.
CopyRecipients = new List<string> { "user@mail.service" },
List of the recipient mailboxes to send blind copy of the email. Optional.
BlindCopyRecipients = new List<string> { "user@mail.service" } */
};
/* If needed, attach file to the email. */
/* Create an attachment. */
var attachment = new EmailContract.DTO.Attachment {
/* Attachment ID. */
Id = Guid.NewGuid().ToString(),
/* Attachment title. For example, "Some file.txt." */
Name = "Some file.txt",
};
/* Attachment data. For example, "Some data." */
byte[] data = Encoding.ASCII.GetBytes("Some data");
/* Attach file to the email. */
attachment.SetData(data);
message.Attachments.Add(attachment);
/* Send the email. */
emailSender.Send(message, credentialConfig);
return true;
4. Set up the business process parameters
-
Open the properties of the business process schema. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Parameters tab.
-
Add the business process parameters.
For this example, add the following parameters:
- parameter that contains the name of email sender
- parameter that contains the mailbox of email sender
- parameter that contains the password to the mailbox of email sender
- parameter that contains the mail server address of the email sender
- parameter that contains the type of the email sender's mail server
- parameter that contains the port number of the email sender's mail server
- parameter that contains information about using a cryptographic protocol to provide secure connection
- parameter that contains the list of the recipient mailboxes
- parameter that contains the email subject
- parameter that contains the email body
To do this:
-
Click Add parameter and select a parameter of the needed type.
-
Fill out the parameter properties.
Element
Element type
Property
Property value
Parameter that contains the name of email sender
Text
Title
Sender
Code
Sender
Value
Click
→ Process parameter → Sender → Select. This populates the property using the "[#Fill out the email parameters.Sender#]" value.
Parameter that contains the mailbox of email sender
Text
Title
Sender mailbox
Code
SenderMailbox
Value
Click
→ Process parameter → Sender mailbox → Select. This populates the property using the "[#Fill out the email parameters.Sender mailbox#]" value.
Parameter that contains the password to the mailbox of email sender
Text
Title
Mailbox password
Code
MailboxPassword
Value
Click
→ Process parameter → Mailbox password → Select. This populates the property using the "[#Fill out the email parameters.Mailbox password#]" value.
Parameter that contains the mail server address of the email sender
Text
Title
Mail server
Code
MailServer
Value
Click
→ Process parameter → Mail server → Select. This populates the property using the "[#Fill out the email parameters.Mail server#]" value.
Parameter that contains the type of the email sender's mail server
Other → Unique identifier
Title
Type of mail server
Code
MailServerType
Value
Click
→ Process parameter → Type of mail server → Select. This populates the property using the "[#Fill out the email parameters.Type of mail server#]" value.
Parameter that contains the port number of the email sender's mail server
Integer
Title
Port
Code
Port
Value
Click
→ Process parameter → Port → Select. This populates the property using the "[#Fill out the email parameters.Port#]" value.
Parameter that contains information about using a cryptographic protocol to provide secure connection
Boolean
Title
Use SSL
Code
UseSsl
Value
Click
→ Process parameter → Use SSL → Select. This populates the property using the "[#Fill out the email parameters.Use SSL#]" value.
Parameter that contains the list of the recipient mailboxes
Text
Title
Recipient
Code
Recipient
Value
Click
→ Process parameter → Recipient (many recipients separated by semicolon ";") → Select. This populates the property using the "[#Fill out the email parameters.Recipient (many recipients separated by semicolon ";")#]" value.
Parameter that contains the email subject
Text
Title
Subject
Code
Subject
Value
Click
→ Process parameter → Subject → Select. This populates the property using the "[#Fill out the email parameters.Subject#]" value.
Parameter that contains the email body
Text
Title
Body
Code
Body
Value
Click
→ Process parameter → Body → Select. This populates the property using the "[#Fill out the email parameters.Body#]" value.
As a result, the Parameters tab of the "Send emails using explicit account credentials" business process will be as follows.
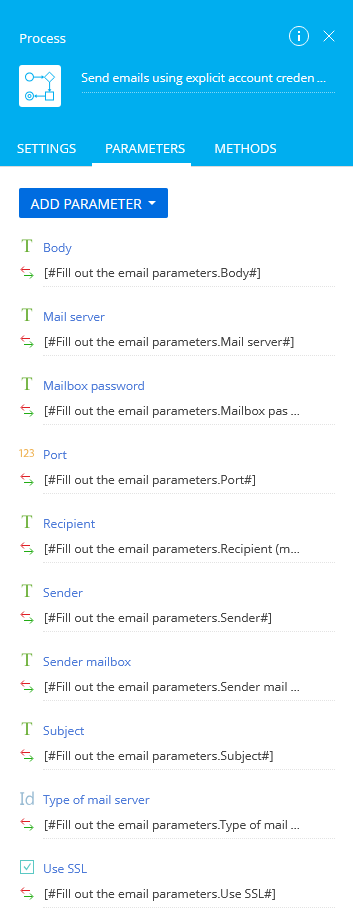
5. Set up the business process methods
-
Open the properties of the business process. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Methods tab.
-
Add the business process methods.
-
Click
and fill out the method properties.
Property
Property value
Namespace
Terrasoft.Configuration
-
Click Save.
-
Add the following methods similarly.
- Terrasoft.Mail.Sender
- Terrasoft.Core.Factories
- Terrasoft.Core
- Terrasoft.Mail
- System.Linq
- IntegrationApi
- EmailContract
-
-
Save the changes.
-
Publish the changes.
As a result:
-
The Methods tab of the "Send emails from existing account" business process will be as follows.
-
The diagram of the "Send emails using explicit account credentials" business process will be as follows.
View the result
- Open the Process library section. To do this, click
→ Processes → Process library.
- Select the "Send emails using explicit account credentials" business process in the section list.
- Run the business process. To do this, click Run.
As a result:
-
Creatio will run a "Send emails using explicit account credentials" business process.
-
The "Send emails using explicit account credentials" business process will generate a "Fill out parameters to send email" page.
-
The "Fill out parameters to send email" page will include the parameters to send the email using explicit account credentials. View the result >>>
To send an email, fill out the parameters and click Continue.
Source code
// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an instance of the "EmailClientFactory." */
var emailClientFactory = ClassFactory.Get<EmailClientFactory>(new ConstructorArgument("userConnection", UserConnection));
/* Set the connection settings for the mail server. */
var credentialConfig = new EmailContract.DTO.Credentials {
MailServer = Get<string>("MailServer"),
Port = Get<int>("Port"),
UseSsl = Get<bool>("UseSsl"),
Sender = Get<string>("Sender"),
MailboxPassword = Get<string>("MailboxPassword"),
MailServerType = Get<Guid>("MailServerType"),
SenderEmailAddress = Get<string>("SenderMailbox")
};
/* Create an instance of the "IEmailSender." */
var emailSender = ClassFactory.Get<IEmailSender>(new ConstructorArgument("emailClientFactory", emailClientFactory),
new ConstructorArgument("userConnection", UserConnection));
/* Fill out the email parameters. */
var message = new EmailContract.DTO.Email {
Sender = credentialConfig.SenderEmailAddress,
Recipients = Get<string>("Recipient").Split(';').ToList<string>(),
Subject = Get<string>("Subject"),
Body = Get<string>("Body"),
Importance = EmailContract.EmailImportance.Normal,
/* Whether the email body is in HTML format. */
IsHtmlBody = true,
/* List of the recipient mailboxes to send copy of the email. Optional.
CopyRecipients = new List<string> { "user@mail.service" },
List of the recipient mailboxes to send blind copy of the email. Optional.
BlindCopyRecipients = new List<string> { "user@mail.service" } */
};
/* If needed, attach file to the email. */
/* Create an attachment. */
var attachment = new EmailContract.DTO.Attachment {
/* Attachment ID. */
Id = Guid.NewGuid().ToString(),
/* Attachment title. For example, "Some file.txt." */
Name = "Some file.txt",
};
/* Attachment data. For example, "Some data." */
byte[] data = Encoding.ASCII.GetBytes("Some data");
/* Attach file to the email. */
attachment.SetData(data);
message.Attachments.Add(attachment);
/* Send the email. */
emailSender.Send(message, credentialConfig);
return true;