Run the business process from the C# console app using the business process service
To implement the example:
- Implement a business process that adds a contact. Read more >>>
- Implement a business process that retrieves the list of contacts. Read more >>>
- Implement the C# console app. Read more >>>
Run the following custom business processes from the C# console app using the ProcessEngineService.svc
business process service:
1. Implement a business process that adds a contact
1. Create a business process
-
Open the Configuration section. Instructions: Open the Configuration section.
-
Create a package. Instructions: Create a user-made package using Configuration section.
For this example, create the
sdkWorkWithBusinessProcessService
package. -
Change the current package. Instructions: Change the current package.
For this example, change the current package to
sdkWorkWithBusinessProcessService
user-made package. -
Create the business process schema. To do this, click Add → Business process.
-
Open the Settings tab.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Title
Add external contact
Code
UsrAddExternalContactProcess
-
Save the changes.
As a result:
- The "Add external contact" business process will be created.
- Creatio will add the "Add external contact" business process to the Process library section.
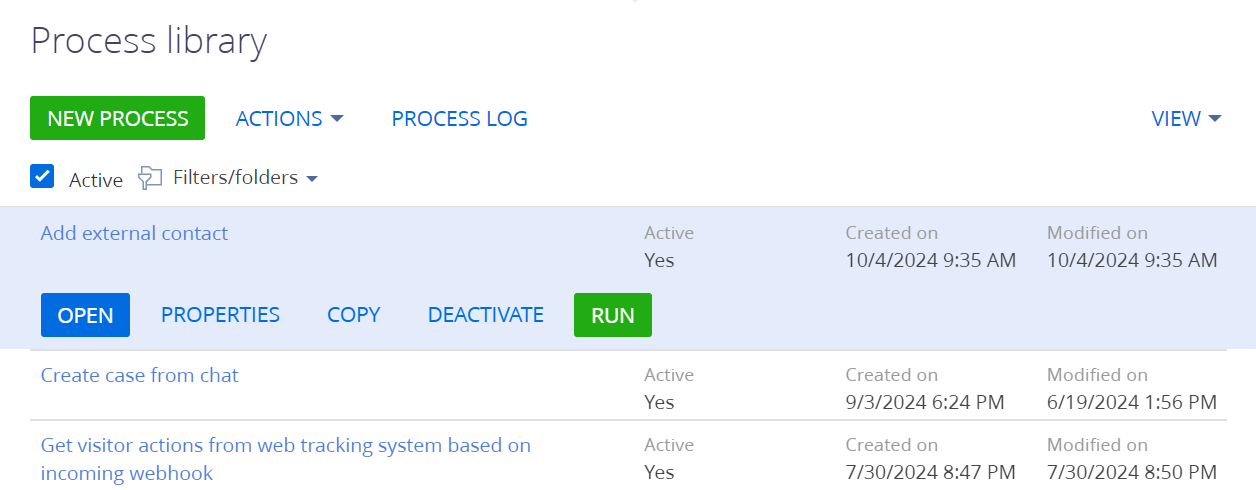
2. Set up the business process parameters
-
Open the properties of the business process schema. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Parameters tab.
-
Add the business process parameters.
For this example, add the following parameters:
- parameter that contains the contact name
- parameter that contains the mobile phone of the contact
To do this:
-
Click Add parameter and select a parameter of the needed type.
-
Fill out the parameter properties.
Element
Element type
Property
Property value
Parameter that contains the contact name
Text
Title
Contact name
Code
ContactName
Data type
Text (50 characters)
Parameter that contains the mobile phone of the contact
Text
Title
Mobile phone
Code
MobilePhone
Data type
Text (50 characters)
As a result, the Parameters tab of the "Add external contact" business process will be as follows.
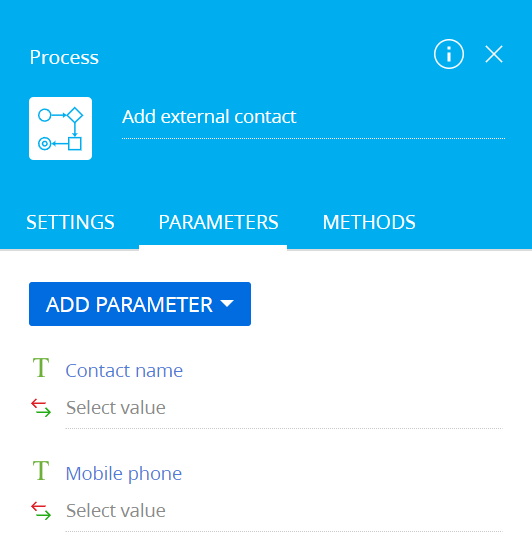
3. Implement adding a contact
-
Add a Script task element. To do this, click
→ place the Script task element between the Simple page element and Terminate page element in the working area of the Process Designer.
-
Fill out the element properties.
Property
Property value
Title
Add contact
-
Implement the logic of working with process parameters. To do this, go to the element setup area and add the source code.
Add contact script task// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an instance of the "Contact" object. */
var schema = UserConnection.EntitySchemaManager.GetInstanceByName("Contact");
/* Create an instance of new object. */
var entity = schema.CreateEntity(UserConnection);
/* Set object columns to default values. */
entity.SetDefColumnValues();
/* Contact name. */
string contactName = Get<string>("ContactName");
/* Mobile phone of the contact. */
string contactPhone = Get<string>("MobilePhone");
/* Set "Name" column to process parameter value. */
entity.SetColumnValue("Name", contactName);
/* Set "MobilePhone" column to process parameter value. */
entity.SetColumnValue("MobilePhone", contactPhone);
/* Save added contact. */
entity.Save();
return true; -
Save the changes.
-
Publish the changes.
As a result, the diagram of the "Add external contact" business process will be as follows.
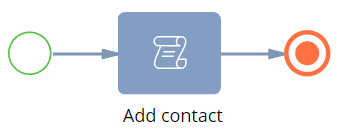
2. Implement a business process that retrieves the list of contacts
1. Create a business process
-
Select a user-made package to add the schema.
For this example, select the
sdkWorkWithBusinessProcessService
user-made package. -
Create the business process schema. To do this, click Add → Business process.
-
Open the Settings tab.
-
Fill out the schema properties.
For this example, use the following schema properties.
Property
Property value
Title
Retrieve the list of contacts
Code
UsrRetrieveContactListProcess
-
Save the changes.
As a result:
- The "Retrieve the list of contacts" business process will be created.
- Creatio will add the "Retrieve the list of contacts" business process to the Process library section.
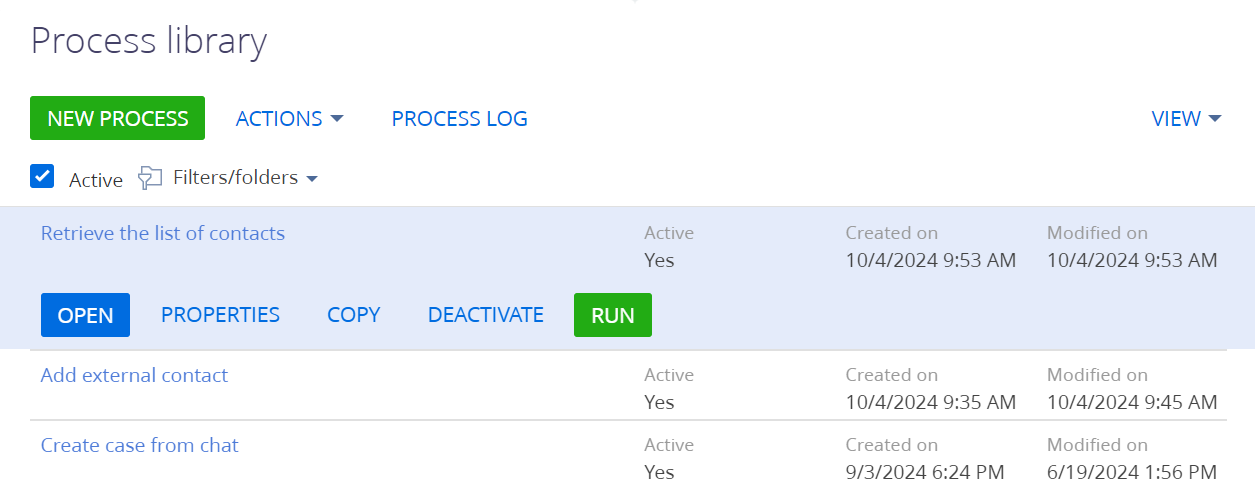
2. Set up the business process parameters
-
Open the properties of the business process schema. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Parameters tab.
-
Add the business process parameters.
For this example, add the parameter that contains the list of contacts. To do this:
-
Click Add parameter and select a parameter of the needed type.
-
Fill out the parameter properties.
Element
Element type
Property
Property value
Parameter that contains the list of contacts
Text
Title
List of contacts
Code
ContactList
Data type
Unlimited length text
-
As a result, the Parameters tab of the "Retrieve the list of contacts" business process will be as follows.
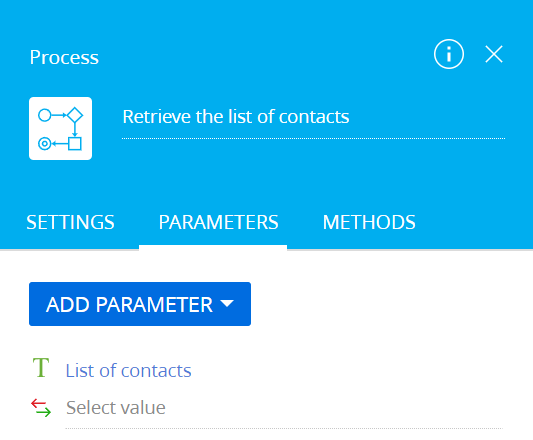
3. Implement retrieving the list of contacts
-
Add a Script task element. To do this, click
→ place the Script task element between the Simple page element and Terminate page element in the working area of the Process Designer.
-
Fill out the element properties.
Property
Property value
Title
Retrieve contacts
-
Implement the logic of working with process parameters. To do this, go to the element setup area and add the source code.
Retrieve contacts script task// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an "EntitySchemaQuery" instance. */
EntitySchemaQuery query = new EntitySchemaQuery(UserConnection.EntitySchemaManager, "Contact");
/* Flag the "Id" primary column as required to select. */
query.PrimaryQueryColumn.IsAlwaysSelect = true;
/* Add columns to the query. */
query.AddColumn("Name");
query.AddColumn("MobilePhone");
/* Retrieve an entity collection. */
var entities = query.GetEntityCollection(UserConnection);
/* Create the list of contacts to serialize in JSON. */
List<object> contacts = new List<object>();
foreach (var item in entities)
{
var contact = new
{
Id = item.GetTypedColumnValue<Guid>("Id"),
Name = item.GetTypedColumnValue<string>("Name"),
MobilePhone = item.GetTypedColumnValue<string>("MobilePhone")
};
contacts.Add(contact);
}
/* Save the contact collection that is serialized in JSON to the "ContactList" parameter. */
string contactList = JsonConvert.SerializeObject(contacts);
Set<string>("ContactList", contactList);
return true;
4. Set up the business process methods
-
Open the properties of the business process. To do this, click an arbitrary place in the working area of the Process Designer.
-
Open the Methods tab.
-
Add the business process methods.
-
Click
and fill out the method properties.
Property
Property value
Namespace
Newtonsoft.Json
-
Click Save.
-
-
Save the changes.
-
Publish the changes.
As a result:
-
The Methods tab of the "Retrieve the list of contacts" business process will be as follows.
-
The diagram of the "Retrieve the list of contacts" business process will be as follows.
3. Implement the C# console app
1. Create a C# console app
-
Open an external IDE.
For this example, open Microsoft Visual Studio.
-
Create a project.
-
Go to the Get started block.
-
Click Create a new project. This opens the Create a new project window.
-
Select Console App (.NET Framework).
-
Click Next. This opens the Configure your new project window.
-
Fill out the project properties.
For this example, use the project properties as follows.
Property
Property value
Project name
WorkWithBusinessProcessService
Framework
.NET Framework 4.7.2
Fill out other project properties based on your business goals.
-
Click Create.
-
As a result:
- The "WorkWithBusinessProcessService" project of console app will be created.
- Microsoft Visual Studio will open the "WorkWithBusinessProcessService" project of the console app.
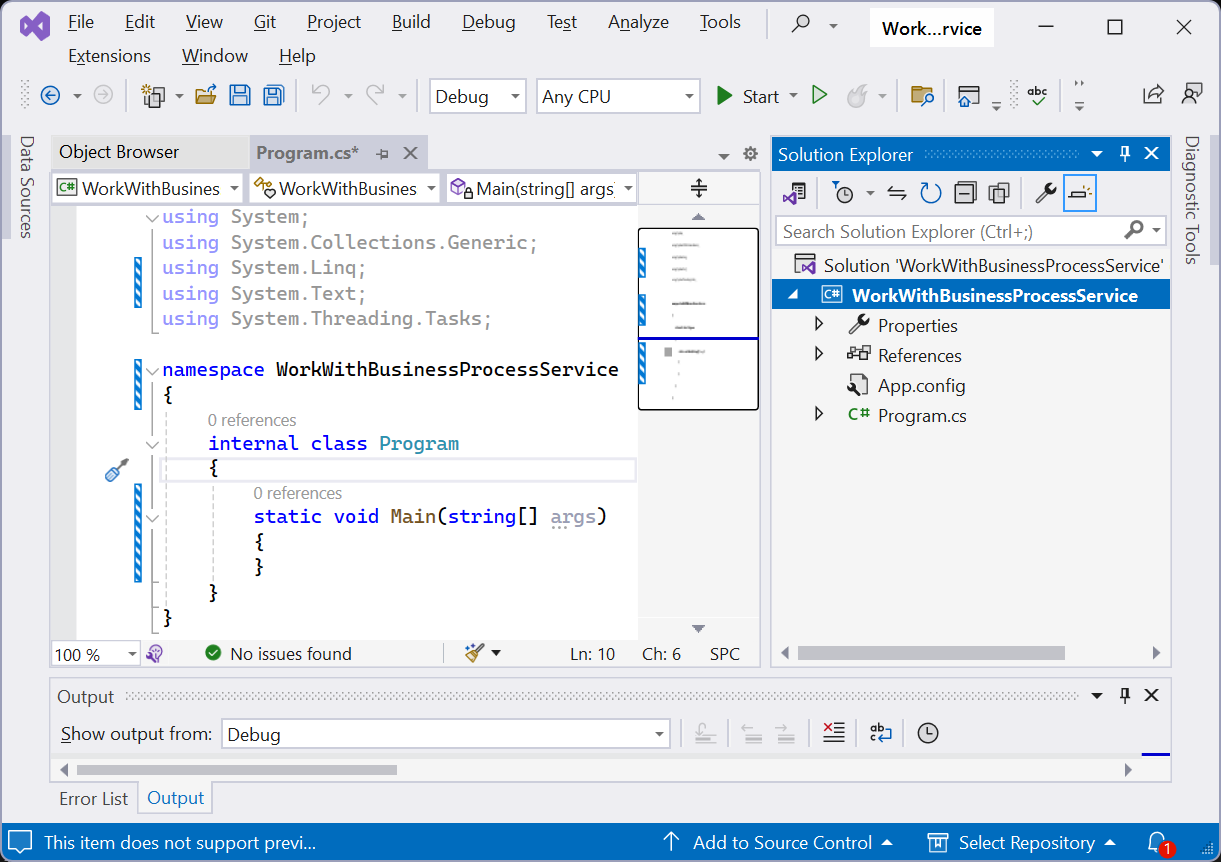
2. Implement the business logic of the console app
-
Implement the authentication of external requests to Creatio.
- Open the
Program.cs
file. - Implement the configuration object of the
AuthService.svc
service response body. - Specify data to implement the authentication of external requests.
- Implement the method that authenticates external requests.
- Authenticate external requests using user credentials.
- Open the
-
Implement running of business processes.
- Specify URL of the
ProcessEngineService.svc
business process service. - Implement the method that runs a business process of adding a contact.
- Run a business process that adds a contact.
- Implement the method that runs a business process of retrieving the list of contacts.
- Run a business process that retrieves the list of contacts.
- Specify URL of the
-
Save the changes.
using System;
using System.IO;
using System.Net;
namespace WorkWithBpmByWebServices
{
/// <summary>
/// Configuration object of the "AuthService.svc" service response body.
/// </summary>
class ResponseStatus
{
public int Code { get; set; }
public string Message { get; set; }
public object Exception { get; set; }
public object PasswordChangeUrl { get; set; }
public object RedirectUrl { get; set; }
}
/// <summary>
/// Main program class.
/// </summary>
internal class Program
{
/* URL of Creatio instance that has the corresponding business processes implemented. */
private const string baseUri = "https://mycreatio.com";
/* URL of "AuthService.svc" service. */
private const string authServiceUri = baseUri + @"/ServiceModel/AuthService.svc/Login";
/* URL of "ProcessEngineService.svc" service. */
private const string processServiceUri = baseUri + @"/0/ServiceModel/ProcessEngineService.svc/";
private static ResponseStatus status = null;
public static CookieContainer AuthCookie = new CookieContainer();
/// <summary>
/// Method that authenticates external requests using user credentials.
/// </summary>
/// <param name="userName">Login that user uses to log in to Creatio.</param>
/// <param name="userPassword">Password that user uses to log in to Creatio.</param>
/// <returns>If the request is authenticated, returns "true." Otherwise, "false."</returns>
public static bool TryLogin(string userName, string userPassword)
{
var authRequest = HttpWebRequest.Create(authServiceUri) as HttpWebRequest;
/* Request method. */
authRequest.Method = "POST";
/* Request header. */
authRequest.ContentType = "application/json";
authRequest.CookieContainer = AuthCookie;
using (var requestStream = authRequest.GetRequestStream())
{
using (var writer = new StreamWriter(requestStream))
{
writer.Write(@"{
""UserName"":""" + userName + @""",
""UserPassword"":""" + userPassword + @"""
}");
}
}
using (var response = (HttpWebResponse)authRequest.GetResponse())
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
string responseText = reader.ReadToEnd();
status = new System.Web.Script.Serialization.JavaScriptSerializer().Deserialize<ResponseStatus>(responseText);
}
}
if (status != null)
{
/* If the code contains a "0" value, the authentication is successful. Otherwise, it is failed. */
if (status.Code == 0)
{
return true;
}
Console.WriteLine(status.Message);
}
return false;
}
/// <summary>
/// Method that runs a business process of adding a contact.
/// </summary>
/// <param name="contactName">Parameter that contains the contact name.</param>
/// <param name="mobilePhone">Parameter that contains the mobile phone of the contact.</param>
public static void AddExternalContact(string contactName, string mobilePhone)
{
/* Request string.*/
string requestString = string.Format(processServiceUri + "UsrAddExternalContactProcess/Execute?ContactName={0}&MobilePhone={1}", contactName, mobilePhone);
HttpWebRequest request = HttpWebRequest.Create(requestString) as HttpWebRequest;
/* Request method. */
request.Method = "GET";
request.CookieContainer = AuthCookie;
using (var response = request.GetResponse())
{
Console.WriteLine(response.ContentLength);
Console.WriteLine(response.Headers.Count);
}
}
/// <summary>
/// Method that runs a business process of retrieving the list of contacts.
/// </summary>
public static void RetrieveContactList()
{
/* Request string.*/
string requestString = processServiceUri + "UsrRetrieveContactListProcess/Execute?ResultParameterName=ContactList";
HttpWebRequest request = HttpWebRequest.Create(requestString) as HttpWebRequest;
/* Request method. */
request.Method = "GET";
request.CookieContainer = AuthCookie;
using (var response = request.GetResponse())
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
string responseText = reader.ReadToEnd();
Console.WriteLine(responseText);
}
}
}
static void Main(string[] args)
{
/* Authenticate external requests using user credentials. */
if (!TryLogin("SomeCreatioLogin", "SomeCreatioPassword"))
{
Console.WriteLine("Wrong login or password. Application will be terminated.");
}
else
{
try
{
/* Run a business process that adds a contact. */
AddExternalContact("John Johanson", "+1 111 111 1111");
/* Run a business process that retrieves the list of contacts. */
RetrieveContactList();
}
catch (Exception)
{
/* Process exception here or throw it further. */
throw;
}
};
Console.WriteLine("Business processes are run. Press ENTER to exit...");
Console.ReadLine();
}
}
}
As a result, the "WorkWithBusinessProcessService" project of console app will be implemented.
View the result
To view the outcome of the example, run the project. To do this, click Start.
As a result:
-
ProcessEngineService.svc
service will run an "Add external contact" and "Retrieve the list of contacts" business processes. -
The "Add external contact" business process will add the contact whose fields are populated using the specified values to the Contacts section. View the result >>>
-
The "Retrieve the list of contacts" business process will retrieve the list of contacts from the Contacts section. View the result >>>
Source code
- Add contact
- Retrieve contacts
- Program.cs
// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an instance of the "Contact" object. */
var schema = UserConnection.EntitySchemaManager.GetInstanceByName("Contact");
/* Create an instance of new object. */
var entity = schema.CreateEntity(UserConnection);
/* Set object columns to default values. */
entity.SetDefColumnValues();
/* Contact name. */
string contactName = Get<string>("ContactName");
/* Mobile phone of the contact. */
string contactPhone = Get<string>("MobilePhone");
/* Set "Name" column to process parameter value. */
entity.SetColumnValue("Name", contactName);
/* Set "MobilePhone" column to process parameter value. */
entity.SetColumnValue("MobilePhone", contactPhone);
/* Save added contact. */
entity.Save();
return true;
// IMPORTANT: When implementing
// long-running operations,
// it is crucial to enable timely and
// responsive cancellation. To achieve
// this, ensure that your code
// is designed to respond appropriately
// to cancellation requests using
// the context.CancellationToken
// mechanism. For more detailed
// information and examples,
// please, refer to our documentation.
/* Create an "EntitySchemaQuery" instance. */
EntitySchemaQuery query = new EntitySchemaQuery(UserConnection.EntitySchemaManager, "Contact");
/* Flag the "Id" primary column as required to select. */
query.PrimaryQueryColumn.IsAlwaysSelect = true;
/* Add columns to the query. */
query.AddColumn("Name");
query.AddColumn("MobilePhone");
/* Retrieve an entity collection. */
var entities = query.GetEntityCollection(UserConnection);
/* Create the list of contacts to serialize in JSON. */
List<object> contacts = new List<object>();
foreach (var item in entities)
{
var contact = new
{
Id = item.GetTypedColumnValue<Guid>("Id"),
Name = item.GetTypedColumnValue<string>("Name"),
MobilePhone = item.GetTypedColumnValue<string>("MobilePhone")
};
contacts.Add(contact);
}
/* Save the contact collection that is serialized in JSON to the "ContactList" parameter. */
string contactList = JsonConvert.SerializeObject(contacts);
Set<string>("ContactList", contactList);
return true;
using System;
using System.IO;
using System.Net;
namespace WorkWithBpmByWebServices
{
/// <summary>
/// Configuration object of the "AuthService.svc" service response body.
/// </summary>
class ResponseStatus
{
public int Code { get; set; }
public string Message { get; set; }
public object Exception { get; set; }
public object PasswordChangeUrl { get; set; }
public object RedirectUrl { get; set; }
}
/// <summary>
/// Main program class.
/// </summary>
internal class Program
{
/* URL of Creatio instance that has the corresponding business processes implemented. */
private const string baseUri = "https://mycreatio.com";
/* URL of "AuthService.svc" service. */
private const string authServiceUri = baseUri + @"/ServiceModel/AuthService.svc/Login";
/* URL of "ProcessEngineService.svc" service. */
private const string processServiceUri = baseUri + @"/0/ServiceModel/ProcessEngineService.svc/";
private static ResponseStatus status = null;
public static CookieContainer AuthCookie = new CookieContainer();
/// <summary>
/// Method that authenticates external requests using user credentials.
/// </summary>
/// <param name="userName">Login that user uses to log in to Creatio.</param>
/// <param name="userPassword">Password that user uses to log in to Creatio.</param>
/// <returns>If the request is authenticated, returns "true." Otherwise, "false."</returns>
public static bool TryLogin(string userName, string userPassword)
{
var authRequest = HttpWebRequest.Create(authServiceUri) as HttpWebRequest;
/* Request method. */
authRequest.Method = "POST";
/* Request header. */
authRequest.ContentType = "application/json";
authRequest.CookieContainer = AuthCookie;
using (var requestStream = authRequest.GetRequestStream())
{
using (var writer = new StreamWriter(requestStream))
{
writer.Write(@"{
""UserName"":""" + userName + @""",
""UserPassword"":""" + userPassword + @"""
}");
}
}
using (var response = (HttpWebResponse)authRequest.GetResponse())
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
string responseText = reader.ReadToEnd();
status = new System.Web.Script.Serialization.JavaScriptSerializer().Deserialize<ResponseStatus>(responseText);
}
}
if (status != null)
{
/* If the code contains a "0" value, the authentication is successful. Otherwise, it is failed. */
if (status.Code == 0)
{
return true;
}
Console.WriteLine(status.Message);
}
return false;
}
/// <summary>
/// Method that runs a business process of adding a contact.
/// </summary>
/// <param name="contactName">Parameter that contains the contact name.</param>
/// <param name="mobilePhone">Parameter that contains the mobile phone of the contact.</param>
public static void AddExternalContact(string contactName, string mobilePhone)
{
/* Request string.*/
string requestString = string.Format(processServiceUri + "UsrAddExternalContactProcess/Execute?ContactName={0}&MobilePhone={1}", contactName, mobilePhone);
HttpWebRequest request = HttpWebRequest.Create(requestString) as HttpWebRequest;
/* Request method. */
request.Method = "GET";
request.CookieContainer = AuthCookie;
using (var response = request.GetResponse())
{
Console.WriteLine(response.ContentLength);
Console.WriteLine(response.Headers.Count);
}
}
/// <summary>
/// Method that runs a business process of retrieving the list of contacts.
/// </summary>
public static void RetrieveContactList()
{
/* Request string.*/
string requestString = processServiceUri + "UsrRetrieveContactListProcess/Execute?ResultParameterName=ContactList";
HttpWebRequest request = HttpWebRequest.Create(requestString) as HttpWebRequest;
/* Request method. */
request.Method = "GET";
request.CookieContainer = AuthCookie;
using (var response = request.GetResponse())
{
using (var reader = new StreamReader(response.GetResponseStream()))
{
string responseText = reader.ReadToEnd();
Console.WriteLine(responseText);
}
}
}
static void Main(string[] args)
{
/* Authenticate external requests using user credentials. */
if (!TryLogin("SomeCreatioLogin", "SomeCreatioPassword"))
{
Console.WriteLine("Wrong login or password. Application will be terminated.");
}
else
{
try
{
/* Run a business process that adds a contact. */
AddExternalContact("John Johanson", "+1 111 111 1111");
/* Run a business process that retrieves the list of contacts. */
RetrieveContactList();
}
catch (Exception)
{
/* Process exception here or throw it further. */
throw;
}
};
Console.WriteLine("Business processes are run. Press ENTER to exit...");
Console.ReadLine();
}
}
}